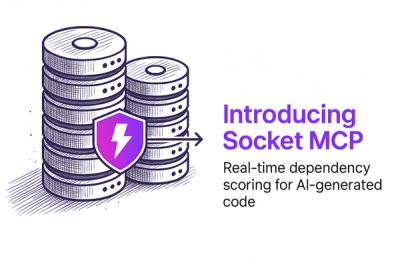
Product
Secure Your AI-Generated Code with Socket MCP
Socket MCP brings real-time security checks to AI-generated code, helping developers catch risky dependencies before they enter the codebase.
color-space
Advanced tools
The color-space npm package provides a comprehensive set of tools for color space conversion and manipulation. It supports a wide range of color spaces and allows for easy conversion between them.
RGB to HSL Conversion
This feature allows you to convert colors from the RGB color space to the HSL color space. The code sample demonstrates converting a red color in RGB to its HSL representation.
const colorSpace = require('color-space');
const rgb = [255, 0, 0];
const hsl = colorSpace.rgb.hsl(rgb);
console.log(hsl); // [0, 100, 50]
HSL to RGB Conversion
This feature allows you to convert colors from the HSL color space to the RGB color space. The code sample demonstrates converting a red color in HSL to its RGB representation.
const colorSpace = require('color-space');
const hsl = [0, 100, 50];
const rgb = colorSpace.hsl.rgb(hsl);
console.log(rgb); // [255, 0, 0]
CMYK to RGB Conversion
This feature allows you to convert colors from the CMYK color space to the RGB color space. The code sample demonstrates converting a red color in CMYK to its RGB representation.
const colorSpace = require('color-space');
const cmyk = [0, 1, 1, 0];
const rgb = colorSpace.cmyk.rgb(cmyk);
console.log(rgb); // [255, 0, 0]
XYZ to LAB Conversion
This feature allows you to convert colors from the XYZ color space to the LAB color space. The code sample demonstrates converting a color in XYZ to its LAB representation.
const colorSpace = require('color-space');
const xyz = [41.24, 21.26, 1.93];
const lab = colorSpace.xyz.lab(xyz);
console.log(lab); // [53.23288178584245, 80.10930952982204, 67.22006831026425]
The color-convert package provides similar functionality for converting between different color spaces. It supports a wide range of color spaces and offers a simple API for conversions. Compared to color-space, color-convert is more focused on providing a straightforward conversion API without additional manipulation features.
Chroma.js is a powerful library for color manipulation and conversion. It supports a wide range of color spaces and provides additional features for color interpolation, blending, and more. Compared to color-space, Chroma.js offers more advanced color manipulation capabilities and a more user-friendly API.
TinyColor is a small, fast library for color manipulation and conversion. It supports a variety of color spaces and provides a simple API for common color operations. Compared to color-space, TinyColor is more lightweight and focused on providing essential color manipulation features.
Open collection of color spaces.
Demo.
import space from 'color-space';
//convert lab to lch
var result = space.lab.lch([80,50,60]);
Spaces can be imported separately:
import rgb from 'color-space/rgb.js';
import hsl from 'color-space/hsl.js';
//convert rgb to hsl
rgb.hsl([200, 230, 100]);
<fromSpace>.<toSpace>(array);
<space>.name //space name
<space>.channel //channel names
<space>.min //channel minimums
<space>.max //channel maximums
A complete collection of color spaces with minimal consistent and clean API, verified formulas and cases. While alternatives focus on digital color spaces, this project takes broader perspective, including historical / multidisciplinary spaces. A side effect is verifying and correcting papers.
Thanks to all who contribute to color science – researchers, scientists, color theorists, specifiers, implementors, developers, and users.
FAQs
Collection of color space conversions
The npm package color-space receives a total of 356,854 weekly downloads. As such, color-space popularity was classified as popular.
We found that color-space demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket MCP brings real-time security checks to AI-generated code, helping developers catch risky dependencies before they enter the codebase.
Security News
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
Research
Security News
Socket’s Threat Research Team has uncovered 60 npm packages using post-install scripts to silently exfiltrate hostnames, IP addresses, DNS servers, and user directories to a Discord-controlled endpoint.