command-line-usage
Advanced tools
Comparing version 1.2.1 to 2.0.0
@@ -1,19 +0,37 @@ | ||
module.exports = { | ||
options: { | ||
title: "a typical app", | ||
description: "Generates something [italic]{very} important.", | ||
groups: { | ||
main: "Main options", | ||
_none: { | ||
title: "Misc", | ||
description: "Miscelaneous ungrouped options." | ||
} | ||
} | ||
}, | ||
definitions: [ | ||
{ name: "help", alias: "h", type: Boolean, description: "Display this usage guide.", group: "main" }, | ||
{ name: "src", type: String, multiple: true, defaultOption: true, description: "The input files to process", typeLabel: "[underline]{file} ...", group: "main" }, | ||
{ name: "timeout", alias: "t", type: Number, description: "Timeout value in ms", typeLabel: "[underline]{ms}", group: "main" }, | ||
{ name: "plugin", type: String, description: "A plugin path" } | ||
] | ||
}; | ||
const getUsage = require('../') | ||
const optionDefinitions = [ | ||
{ | ||
name: 'help', description: 'Display this usage guide.', | ||
alias: 'h', type: Boolean, | ||
group: 'main' | ||
}, | ||
{ | ||
name: 'src', description: 'The input files to process', | ||
type: String, multiple: true, defaultOption: true, typeLabel: '[underline]{file} ...', | ||
group: 'main' | ||
}, | ||
{ | ||
name: 'timeout', description: 'Timeout value in ms', | ||
alias: 't', type: Number, typeLabel: '[underline]{ms}', | ||
group: 'main' | ||
}, | ||
{ | ||
name: 'plugin', description: 'A plugin path', | ||
type: String | ||
} | ||
] | ||
const options = { | ||
title: 'a typical app', | ||
description: 'Generates something [italic]{very} important.', | ||
groups: { | ||
main: 'Main options', | ||
_none: { | ||
title: 'Misc', | ||
description: 'Miscelaneous ungrouped options.' | ||
} | ||
} | ||
} | ||
console.log(getUsage(optionDefinitions, options)) |
@@ -1,12 +0,24 @@ | ||
module.exports = { | ||
options: { | ||
title: "a typical app", | ||
description: "Generates something very important.", | ||
footer: "Project home: [underline]{https://github.com/me/example}" | ||
}, | ||
definitions: [ | ||
{ name: "help", alias: "h", type: Boolean, description: "Display this usage guide." }, | ||
{ name: "src", type: String, multiple: true, defaultOption: true, description: "The input files to process" }, | ||
{ name: "timeout", alias: "t", type: Number, description: "Timeout value in ms" } | ||
] | ||
}; | ||
const getUsage = require('../') | ||
const optionDefinitions = [ | ||
{ | ||
name: 'help', description: 'Display this usage guide.', | ||
alias: 'h', type: Boolean | ||
}, | ||
{ | ||
name: 'src', description: 'The input files to process', | ||
type: String, multiple: true, defaultOption: true | ||
}, | ||
{ | ||
name: 'timeout', description: 'Timeout value in ms', | ||
alias: 't', type: Number | ||
} | ||
] | ||
const options = { | ||
title: 'a typical app', | ||
description: 'Generates something very important.', | ||
footer: 'Project home: [underline]{https://github.com/me/example}' | ||
} | ||
console.log(getUsage(optionDefinitions, options)) |
@@ -1,18 +0,25 @@ | ||
"use strict"; | ||
var columnLayout = require("column-layout"); | ||
var o = require("object-tools"); | ||
var a = require("array-tools"); | ||
var util = require("util"); | ||
var ansi = require("ansi-escape-sequences"); | ||
var t = require("typical"); | ||
var UsageOptions = require("./usage-options"); | ||
var arrayify = require("array-back"); | ||
'use strict' | ||
var columnLayout = require('column-layout') | ||
var o = require('object-tools') | ||
var ansi = require('ansi-escape-sequences') | ||
var os = require('os') | ||
var t = require('typical') | ||
var UsageOptions = require('./usage-options') | ||
var arrayify = require('array-back') | ||
/** | ||
Exports a single function to generate a usage guide using [column-layout](http://github.com/75lb/column-layout). | ||
@module command-line-usage | ||
*/ | ||
module.exports = getUsage; | ||
module.exports = getUsage | ||
class Lines extends Array { | ||
add (content) { | ||
arrayify(content).forEach(line => this.push(ansi.format(line))) | ||
} | ||
emptyLine () { | ||
this.push('') | ||
} | ||
} | ||
/** | ||
@@ -22,3 +29,3 @@ @param {optionDefinition[]} - an array of [option definition](https://github.com/75lb/command-line-args#exp_module_definition--OptionDefinition) objects. In addition to the regular definition properties, command-line-usage will look for: | ||
- `description` - a string describing the option. | ||
- `typeLabel` - a string to replace the default type string (e.g. `<string>`). It's often more useful to set a more descriptive type label, like `<ms>`, `<files>`, `<command>` etc. | ||
- `typeLabel` - a string to replace the default type string (e.g. `<string>`). It's often more useful to set a more descriptive type label, like `<ms>`, `<files>`, `<command>` etc. | ||
@@ -28,143 +35,161 @@ @param options {module:usage-options} - see [UsageOptions](#exp_module_usage-options--UsageOptions). | ||
@alias module:command-line-usage | ||
@example @lang off | ||
Some example usage output: | ||
*/ | ||
function getUsage (definitions, options) { | ||
options = new UsageOptions(options) | ||
definitions = definitions || [] | ||
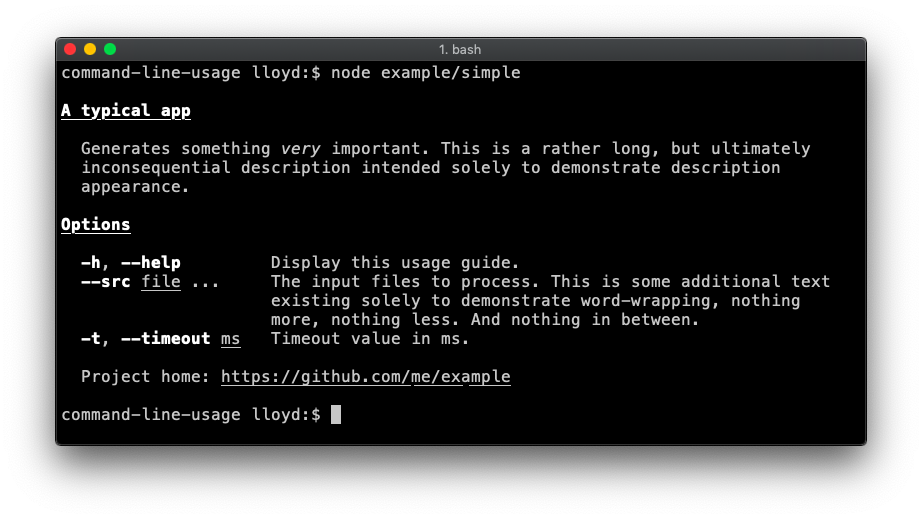 | ||
var output = new Lines() | ||
output.emptyLine() | ||
 | ||
/* filter out hidden definitions */ | ||
if (options.hide && options.hide.length) { | ||
definitions = definitions.filter(definition => options.hide.indexOf(definition.name) === -1) | ||
} | ||
*/ | ||
function getUsage(definitions, options){ | ||
options = new UsageOptions(options); | ||
definitions = definitions || []; | ||
if (options.header) { | ||
output.add(renderSection('', options.header)) | ||
} | ||
/* filter out hidden options */ | ||
if (options.hide && options.hide.length){ | ||
definitions = definitions.filter(function(option){ | ||
return !a(options.hide).contains(option.name); | ||
}); | ||
} | ||
if (options.title || options.description) { | ||
output.add(renderSection(options.title, options.description)) | ||
} | ||
var lines = new Lines(options); | ||
lines.addEmpty(); | ||
if (options.title) lines.addLine(getText(options.title, [ "underline", "bold" ])); | ||
if (options.description) lines.addLine(getText(options.description)); | ||
if (options.title || options.description) lines.addEmpty(); | ||
if (options.synopsis) { | ||
output.add(renderSection('Synopsis', options.synopsis)) | ||
} | ||
if (options.synopsis){ | ||
options.synopsis = arrayify(options.synopsis); | ||
lines.addLine(ansi.format("Synopsis", [ "underline", "bold" ])); | ||
options.synopsis.forEach(function(form){ | ||
lines.addLine(getText(form)); | ||
}); | ||
lines.addEmpty(); | ||
} | ||
if (definitions.length){ | ||
if (options.groups){ | ||
o.each(options.groups, function(val, group){ | ||
var title, description; | ||
if (t.isObject(val)){ | ||
title = val.title; | ||
description = val.description; | ||
} else if (typeof val === "string"){ | ||
title = val; | ||
} else { | ||
throw ("Unexpected group config structure"); | ||
} | ||
lines.addLine(getText(title, [ "underline", "bold" ])); | ||
if (description){ | ||
lines.addLine(getText(description)); | ||
lines.addEmpty(); | ||
} | ||
if (group === "_none"){ | ||
a(definitions).where({ group: undefined }).forEach(lines.addRow); | ||
} else { | ||
a(definitions).where({ "+group": group }).forEach(lines.addRow); | ||
} | ||
lines.addEmpty(); | ||
}); | ||
if (definitions.length) { | ||
if (options.groups) { | ||
o.each(options.groups, (val, group) => { | ||
var title | ||
var description | ||
if (t.isObject(val)) { | ||
title = val.title | ||
description = val.description | ||
} else if (t.isString(val)) { | ||
title = val | ||
} else { | ||
definitions.forEach(lines.addRow); | ||
lines.addEmpty(); | ||
throw new Error('Unexpected group config structure') | ||
} | ||
} | ||
var output = lines.render(); | ||
if (options.examples){ | ||
lines = new Lines(options); | ||
lines.addLine(ansi.format("Examples", [ "bold", "underline" ])); | ||
arrayify(options.examples).forEach(lines.addLine.bind(lines)); | ||
lines.addEmpty(); | ||
output += lines.render(); | ||
output.add(renderSection(title, description)) | ||
let optionList = getUsage.optionList(definitions, group) | ||
output.add(renderSection(null, optionList, true)) | ||
}) | ||
} else { | ||
output.add(renderSection('Options', getUsage.optionList(definitions), true)) | ||
} | ||
} | ||
if (options.footer){ | ||
lines = new Lines(options); | ||
arrayify(options.footer).forEach(lines.addLine.bind(lines)); | ||
lines.addEmpty(); | ||
output += lines.render(); | ||
} | ||
/* the footer adds an empty line - remove it if one-too-many */ | ||
output = output.replace(/\n\s*\n$/, "\n"); | ||
return output; | ||
if (options.examples) { | ||
output.add(renderSection('Examples', options.examples)) | ||
} | ||
if (options.footer) { | ||
output.add(renderSection('', options.footer)) | ||
} | ||
return output.join(os.EOL) | ||
} | ||
function getOptionNames(cliOption, optionNameStyles){ | ||
var names = []; | ||
var type = cliOption.type ? cliOption.type.name.toLowerCase() : ""; | ||
var multiple = cliOption.multiple ? "[]" : ""; | ||
if (type) type = type === "boolean" ? "" : "[underline]{" + type + multiple + "}"; | ||
type = ansi.format(cliOption.typeLabel || type); | ||
function getOptionNames (definition, optionNameStyles) { | ||
var names = [] | ||
var type = definition.type ? definition.type.name.toLowerCase() : '' | ||
var multiple = definition.multiple ? '[]' : '' | ||
if (type) type = type === 'boolean' ? '' : `[underline]{${type}${multiple}}` | ||
type = ansi.format(definition.typeLabel || type) | ||
if (cliOption.alias) names.push(ansi.format("-" + cliOption.alias, optionNameStyles)); | ||
names.push(ansi.format("--" + cliOption.name, optionNameStyles) + " " + type); | ||
return names.join(", "); | ||
if (definition.alias) names.push(ansi.format('-' + definition.alias, optionNameStyles)) | ||
names.push(ansi.format(`--${definition.name}`, optionNameStyles) + ' ' + type) | ||
return names.join(', ') | ||
} | ||
function getText(text, styleArray){ | ||
if (t.isString(text)){ | ||
return ansi.format(text, styleArray) | ||
} else if (t.isPlainObject(text)){ | ||
return ansi.format(text.text, text.format || styleArray); | ||
function renderSection (title, content, skipIndent) { | ||
var lines = new Lines() | ||
if (title) { | ||
lines.add(ansi.format(title, [ 'underline', 'bold' ])) | ||
lines.emptyLine() | ||
} | ||
if (!content) { | ||
return lines | ||
} else { | ||
if (t.isString(content)) { | ||
lines.add(indentString(content)) | ||
} else if (Array.isArray(content) && content.every(t.isString)) { | ||
lines.add(skipIndent ? content : indentArray(content)) | ||
} else if (Array.isArray(content) && content.every(t.isPlainObject)) { | ||
lines.add(columnLayout.lines(content, { | ||
padding: { left: ' ', right: ' ' } | ||
})) | ||
} else if (t.isPlainObject(content)) { | ||
if (!content.options || !content.data) { | ||
throw new Error('must have an "options" or "data" property\n' + JSON.stringify(content)) | ||
} | ||
content.options = o.extend({ | ||
padding: { left: ' ', right: ' ' } | ||
}, content.options) | ||
lines.add(columnLayout.lines( | ||
content.data.map(row => formatRow(row)), | ||
content.options | ||
)) | ||
} else { | ||
var message = `invalid input - 'content' must be a string, array of strings, or array of plain objects:\n\n${JSON.stringify(content)}` | ||
throw new Error(message) | ||
} | ||
lines.emptyLine() | ||
return lines | ||
} | ||
} | ||
function Lines(options){ | ||
var lines = []; | ||
this.addLine = function(line){ | ||
if (t.isPlainObject(line)){ | ||
lines.push(line); | ||
} else { | ||
lines.push((typeof line === "string" ? getText(line) : "")); | ||
} | ||
}; | ||
this.addRow = function(definition){ | ||
lines.push({ | ||
col1: getOptionNames(definition, "bold"), | ||
col2: getText(definition.description) | ||
}); | ||
}; | ||
this.addEmpty = function(){ | ||
lines.push(""); | ||
}; | ||
this.render = function(){ | ||
return columnLayout(lines, { | ||
viewWidth: options.viewWidth || process.stdout.columns, | ||
padding: { | ||
left: " ", | ||
right: " " | ||
}, | ||
columns: [ | ||
{ name: "col1", nowrap: true } | ||
] | ||
}); | ||
} | ||
function indentString (string) { | ||
return ' ' + string | ||
} | ||
function indentArray (array) { | ||
return array.map(indentString) | ||
} | ||
function formatRow (row) { | ||
o.each(row, (val, key) => { | ||
row[key] = ansi.format(val) | ||
}) | ||
return row | ||
} | ||
/** | ||
* A helper for getting a column-format list of options and descriptions. Useful for inserting into a custom usage template. | ||
* | ||
* @param {optionDefinition[]} - the definitions to Display | ||
* @param [group] {string} - if specified, will output the options in this group. The special group `'_none'` will return options without a group specified. | ||
* @returns {string[]} | ||
*/ | ||
getUsage.optionList = function (definitions, group) { | ||
if (!definitions || (definitions && !definitions.length)) { | ||
throw new Error('you must pass option definitions to getUsage.optionList()') | ||
} | ||
var columns = [] | ||
if (group === '_none') { | ||
definitions = definitions.filter(def => !t.isDefined(def.group)) | ||
} else if (group) { | ||
definitions = definitions.filter(def => arrayify(def.group).indexOf(group) > -1) | ||
} | ||
definitions | ||
.forEach(def => { | ||
columns.push({ | ||
option: getOptionNames(def, 'bold'), | ||
description: def.description | ||
}) | ||
}) | ||
return columnLayout.lines(columns, { | ||
padding: { left: ' ', right: ' ' }, | ||
columns: [ | ||
{ name: 'option', nowrap: true }, | ||
{ name: 'description', maxWidth: 80 } | ||
] | ||
}) | ||
} |
@@ -1,4 +0,3 @@ | ||
"use strict"; | ||
var arrayify = require("array-back"); | ||
var o = require("object-tools"); | ||
'use strict' | ||
var arrayify = require('array-back') | ||
@@ -8,3 +7,3 @@ /** | ||
*/ | ||
module.exports = UsageOptions; | ||
module.exports = UsageOptions | ||
@@ -17,74 +16,80 @@ /** | ||
*/ | ||
function UsageOptions(options){ | ||
options = options || {}; | ||
function UsageOptions (options) { | ||
options = options || {} | ||
/** | ||
* The title line at the top of the usage, typically the name of the app. By default it is underlined but this formatting can be overridden by passing a {@link module:usage-options~textObject}. | ||
* | ||
* @type {string} | ||
* @example | ||
* { | ||
* title: "my-app" | ||
* } | ||
* @example | ||
* { | ||
* title: { | ||
* text: "my-app", | ||
* format: [ "bold", "underline" ] | ||
* } | ||
* } | ||
*/ | ||
this.title = options.title; | ||
/** | ||
* Use this field to display a banner or header above the main body. | ||
* @type {string} | ||
*/ | ||
this.header = options.header | ||
/** | ||
A description to go underneath the title. For example, some words about what the app is for. | ||
@type {string} | ||
*/ | ||
this.description = options.description; | ||
/** | ||
* The title line at the top of the usage, typically the name of the app. By default it is underlined but this formatting can be overridden by passing a {@link module:usage-options~textObject}. | ||
* | ||
* @type {string} | ||
* @example | ||
* { | ||
* title: "my-app" | ||
* } | ||
* @example | ||
* { | ||
* title: { | ||
* text: "my-app", | ||
* format: [ "bold", "underline" ] | ||
* } | ||
* } | ||
*/ | ||
this.title = options.title | ||
/** | ||
* An array of strings highlighting the main usage forms of the app. | ||
* @type {string[]} | ||
*/ | ||
this.synopsis = options.synopsis || (options.usage && options.usage.forms) || options.forms; | ||
/** | ||
A description to go underneath the title. For example, some words about what the app is for. | ||
@type {string} | ||
*/ | ||
this.description = options.description | ||
/** | ||
* Specify which groups to display in the output by supplying an object of key/value pairs, where the key is the name of the group to include and the value is a string or textObject. If the value is a string it is used as the group title. Alternatively supply an object containing a `title` and `description` string. | ||
* @type {object} | ||
* @example | ||
* { | ||
* main: { | ||
* title: "Main options", | ||
* description: "This group contains the most important options." | ||
* }, | ||
* misc: "Miscellaneous" | ||
* } | ||
*/ | ||
this.groups = options.groups; | ||
/** | ||
Examples | ||
@type {string[] | object[]} | ||
*/ | ||
this.examples = options.examples; | ||
/** | ||
* An array of strings highlighting the main usage forms of the app. | ||
* @type {string[]} | ||
*/ | ||
this.synopsis = options.synopsis || (options.usage && options.usage.forms) || options.forms | ||
/** | ||
* Displayed at the foot of the usage output. | ||
* @type {string} | ||
* @example | ||
* { | ||
* footer: "Project home: [underline]{https://github.com/me/my-app}" | ||
* } | ||
*/ | ||
this.footer = options.footer; | ||
/** | ||
* Specify which groups to display in the output by supplying an object of key/value pairs, where the key is the name of the group to include and the value is a string or textObject. If the value is a string it is used as the group title. Alternatively supply an object containing a `title` and `description` string. | ||
* @type {object} | ||
* @example | ||
* { | ||
* main: { | ||
* title: "Main options", | ||
* description: "This group contains the most important options." | ||
* }, | ||
* misc: "Miscellaneous" | ||
* } | ||
*/ | ||
this.groups = options.groups | ||
/** | ||
* If you want to hide certain options from the output, specify their names here. This is sometimes used to hide the `defaultOption`. | ||
* @type {string|string[]} | ||
* @example | ||
* { | ||
* hide: "files" | ||
* } | ||
*/ | ||
this.hide = arrayify(options.hide); | ||
/** | ||
Examples | ||
@type {string[] | object[]} | ||
*/ | ||
this.examples = options.examples | ||
/** | ||
* Displayed at the foot of the usage output. | ||
* @type {string} | ||
* @example | ||
* { | ||
* footer: "Project home: [underline]{https://github.com/me/my-app}" | ||
* } | ||
*/ | ||
this.footer = options.footer | ||
/** | ||
* If you want to hide certain options from the output, specify their names here. This is sometimes used to hide the `defaultOption`. | ||
* @type {string|string[]} | ||
* @example | ||
* { | ||
* hide: "files" | ||
* } | ||
*/ | ||
this.hide = arrayify(options.hide) | ||
} |
{ | ||
"name": "command-line-usage", | ||
"author": "Lloyd Brookes <75pound@gmail.com>", | ||
"version": "1.2.1", | ||
"version": "2.0.0", | ||
"description": "Generates command-line usage information", | ||
"repository": "https://github.com/75lb/command-line-usage.git", | ||
"license": "MIT", | ||
"main": "./lib/command-line-usage.js", | ||
"bin": { | ||
"command-line-usage": "bin/cli.js" | ||
}, | ||
"main": "index", | ||
"keywords": [ | ||
@@ -23,3 +20,4 @@ "terminal", | ||
"docs": "jsdoc2md -t jsdoc2md/README.hbs --no-gfm lib/*.js > README.md; echo", | ||
"test": "tape test/*.js" | ||
"test": "tape test/*.js", | ||
"es5": "babel --no-comments lib --out-dir es5" | ||
}, | ||
@@ -29,4 +27,4 @@ "dependencies": { | ||
"array-back": "^1.0.2", | ||
"array-tools": "^2", | ||
"column-layout": "^1.0.0", | ||
"column-layout": "^2", | ||
"feature-detect-es6": "^1.0.0", | ||
"object-tools": "^2", | ||
@@ -38,3 +36,8 @@ "typical": "^2.3.1" | ||
"tape": "^4.0.0" | ||
}, | ||
"standard": { | ||
"ignore": [ | ||
"es5" | ||
] | ||
} | ||
} |
123
README.md
[](https://www.npmjs.org/package/command-line-usage) | ||
[](https://www.npmjs.org/package/command-line-usage) | ||
[](https://www.npmjs.org/package/command-line-usage) | ||
[](https://travis-ci.org/75lb/command-line-usage) | ||
[](https://david-dm.org/75lb/command-line-usage) | ||
[](https://github.com/feross/standard) | ||
<a name="module_command-line-usage"></a> | ||
## command-line-usage | ||
Exports a single function to generate a usage guide using [column-layout](http://github.com/75lb/column-layout). | ||
# command-line-usage | ||
A simple template to create a usage guide. It was extracted from [command-line-args](https://github.com/75lb/command-line-args) to faciliate arbitrary use. | ||
```js | ||
var getUsage = require("command-line-usage"); | ||
var usage = getUsage(definitions, options) | ||
``` | ||
Inline ansi formatting can be used anywhere within the usage template using the formatting syntax described [here](https://github.com/75lb/ansi-escape-sequences#module_ansi-escape-sequences.format). | ||
## Examples | ||
### Simple | ||
A `description` field is added to each option definition. A `title`, `description` and simple `footer` are set in the getUsage options. [Code](https://github.com/75lb/command-line-usage/blob/master/example/simple.js). | ||
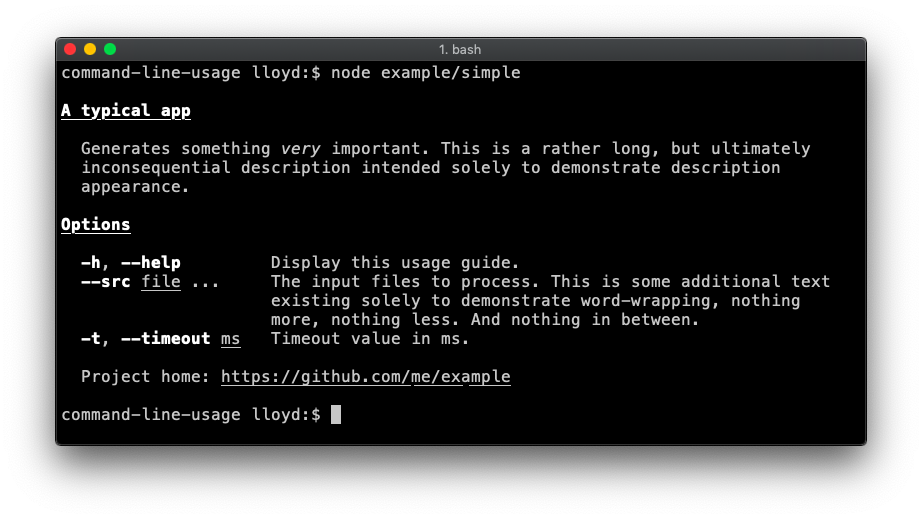 | ||
### Groups | ||
Demonstrates breaking the options up into groups. This example also sets a `typeLabel` on each option definition (e.g. a `typeLabel` value of `files` is more meaningful than the default `string[]`). [Code](https://github.com/75lb/command-line-usage/blob/master/example/groups.js). | ||
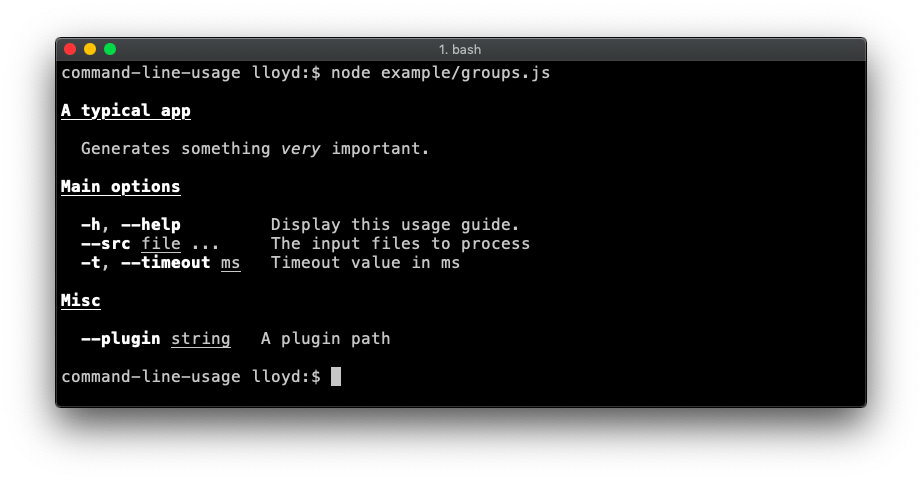 | ||
### Header | ||
Here, the `title` is replaced with a `header` banner. This example also adds a `synopsis` list. [Code](https://github.com/75lb/command-line-usage/blob/master/example/header.js). | ||
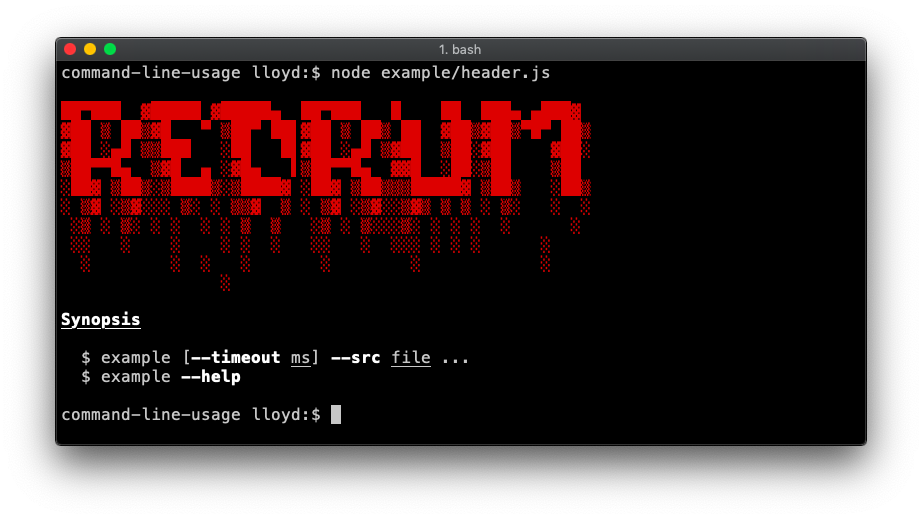 | ||
### Footer | ||
The footer is displayed at the end of the template. [Code](https://github.com/75lb/command-line-usage/blob/master/example/footer.js). | ||
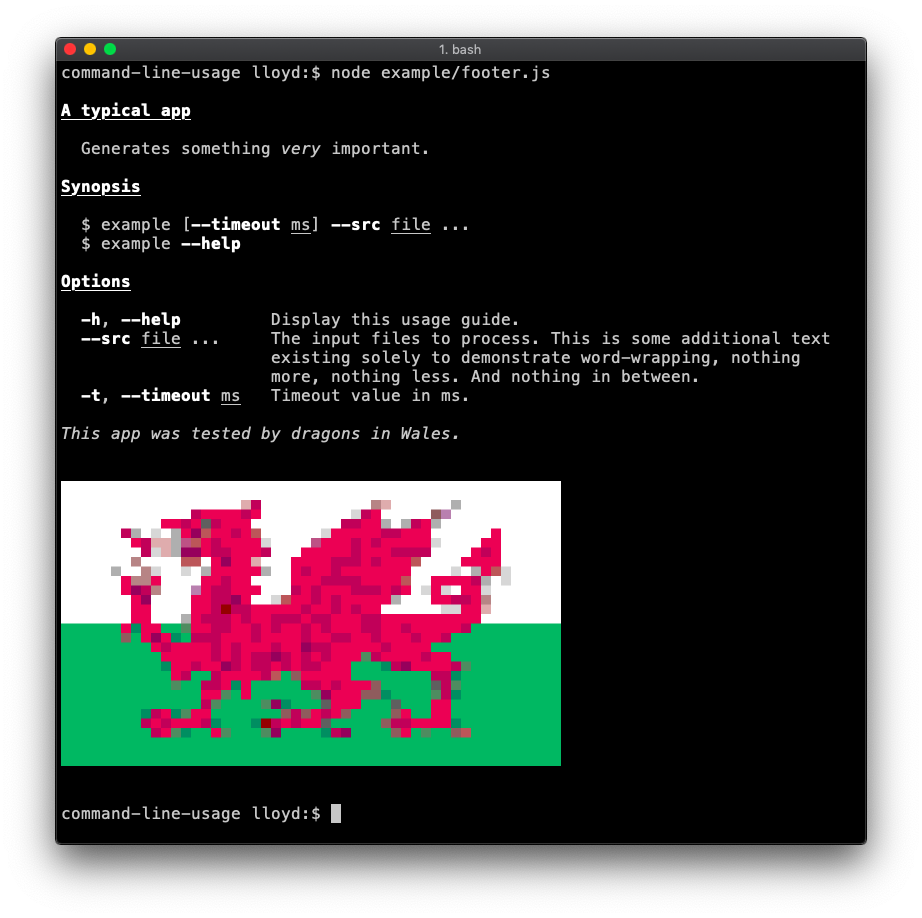 | ||
### Examples (column layout) | ||
A list of `examples` is added. In this case the example list is defined as an array of objects (each with consistently named properties) so will be formatted by [column-layout](https://github.com/75lb/column-layout). [Code](https://github.com/75lb/command-line-usage/blob/master/example/examples.js). | ||
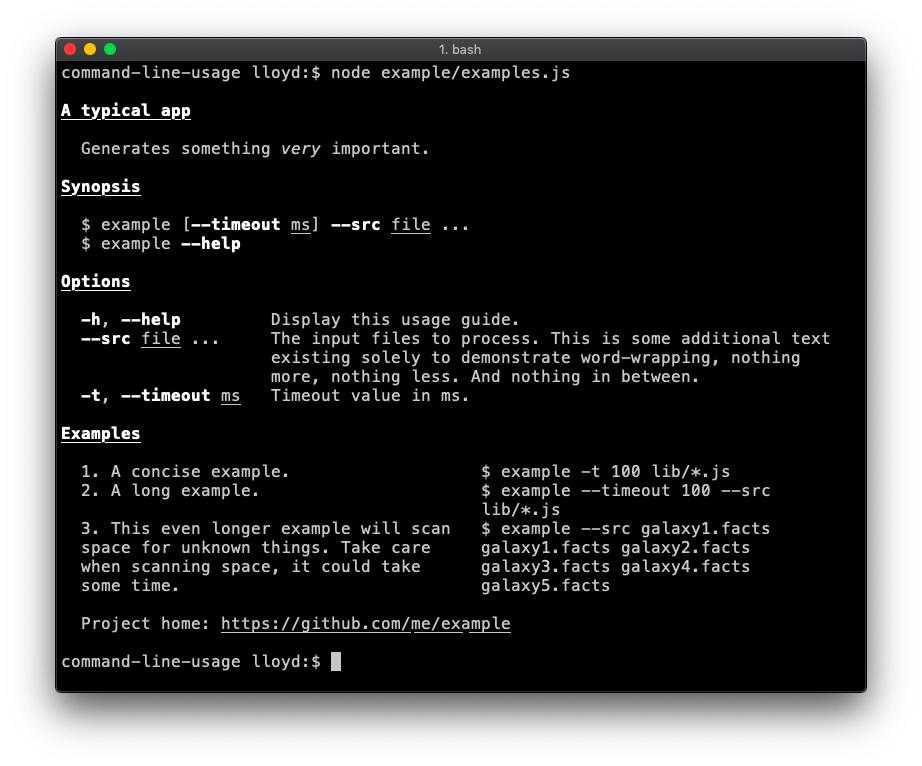 | ||
### Description (column layout) | ||
Demonstrates usage of custom column layout in the description. In this case the second column (containing the hammer and sickle) has `nowrap` disabled, as the input is already formatted as desired. [Code](https://github.com/75lb/command-line-usage/blob/master/example/description-columns.js). | ||
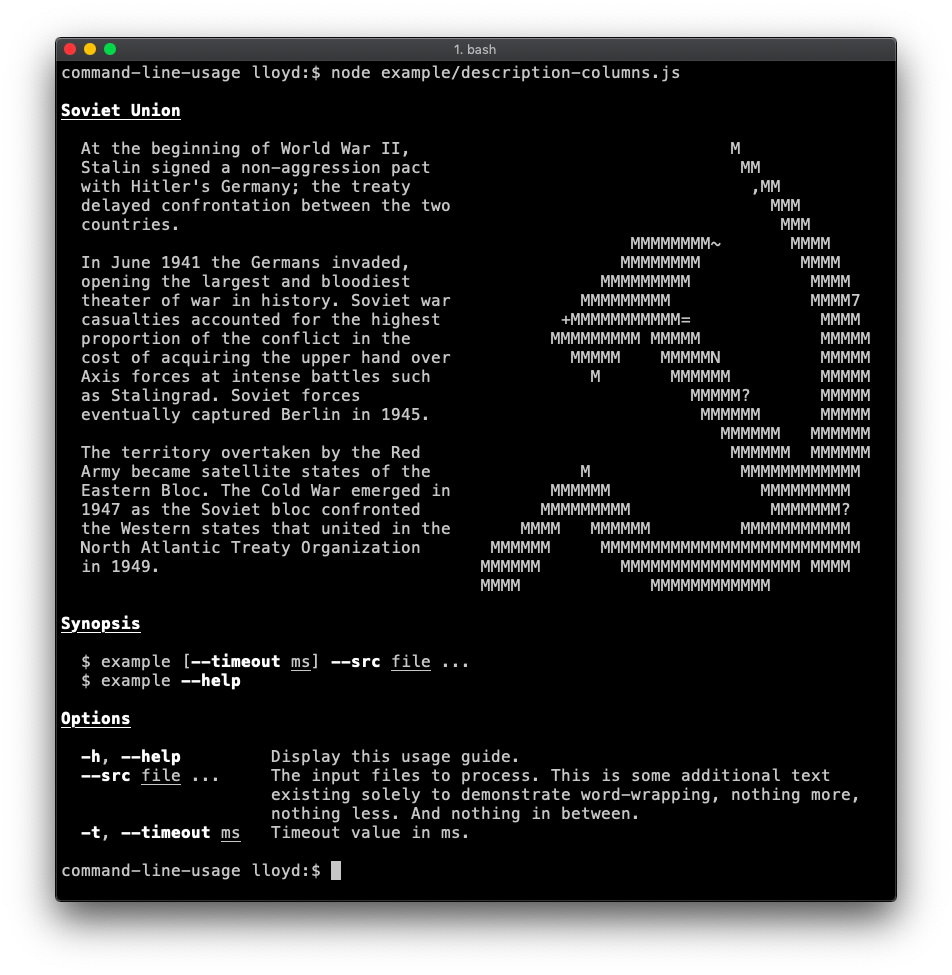 | ||
### Custom | ||
Demonstrates a custom template. The `getUsage.optionList()` method exists for users that want the option list and nothing else. [Code](https://github.com/75lb/command-line-usage/blob/master/example/custom.js). | ||
 | ||
# API Reference | ||
* [command-line-usage](#module_command-line-usage) | ||
* [getUsage(definitions, options)](#exp_module_command-line-usage--getUsage) ⇒ <code>string</code> ⏏ | ||
* [.optionList(definitions, [group])](#module_command-line-usage--getUsage.optionList) ⇒ <code>Array.<string></code> | ||
<a name="exp_module_command-line-usage--getUsage"></a> | ||
### getUsage(definitions, options) ⇒ <code>string</code> ⏏ | ||
**Kind**: Exported function | ||
<table> | ||
<thead> | ||
<tr> | ||
<th>Param</th><th>Type</th><th>Description</th> | ||
</tr> | ||
</thead> | ||
<tbody> | ||
<tr> | ||
<td>definitions</td><td><code>Array.<optionDefinition></code></td><td><p>an array of <a href="https://github.com/75lb/command-line-args#exp_module_definition--OptionDefinition">option definition</a> objects. In addition to the regular definition properties, command-line-usage will look for:</p> | ||
<ul> | ||
<li><code>description</code> - a string describing the option.</li> | ||
<li><code>typeLabel</code> - a string to replace the default type string (e.g. <code><string></code>). It's often more useful to set a more descriptive type label, like <code><ms></code>, <code><files></code>, <code><command></code> etc.</li> | ||
</ul> | ||
</td> | ||
</tr><tr> | ||
<td>options</td><td><code><a href="#module_usage-options">usage-options</a></code></td><td><p>see <a href="#exp_module_usage-options--UsageOptions">UsageOptions</a>.</p> | ||
</td> | ||
</tr> </tbody> | ||
</table> | ||
| Param | Type | Description | | ||
| --- | --- | --- | | ||
| definitions | <code>Array.<optionDefinition></code> | an array of [option definition](https://github.com/75lb/command-line-args#exp_module_definition--OptionDefinition) objects. In addition to the regular definition properties, command-line-usage will look for: - `description` - a string describing the option. - `typeLabel` - a string to replace the default type string (e.g. `<string>`). It's often more useful to set a more descriptive type label, like `<ms>`, `<files>`, `<command>` etc. | | ||
| options | <code>[usage-options](#module_usage-options)</code> | see [UsageOptions](#exp_module_usage-options--UsageOptions). | | ||
<a name="module_command-line-usage--getUsage.optionList"></a> | ||
#### getUsage.optionList(definitions, [group]) ⇒ <code>Array.<string></code> | ||
A helper for getting a column-format list of options and descriptions. Useful for inserting into a custom usage template. | ||
**Example** | ||
Some example usage output: | ||
**Kind**: static method of <code>[getUsage](#exp_module_command-line-usage--getUsage)</code> | ||
<table> | ||
<thead> | ||
<tr> | ||
<th>Param</th><th>Type</th><th>Description</th> | ||
</tr> | ||
</thead> | ||
<tbody> | ||
<tr> | ||
<td>definitions</td><td><code>Array.<optionDefinition></code></td><td><p>the definitions to Display</p> | ||
</td> | ||
</tr><tr> | ||
<td>[group]</td><td><code>string</code></td><td><p>if specified, will output the options in this group. The special group <code>'_none'</code> will return options without a group specified.</p> | ||
</td> | ||
</tr> </tbody> | ||
</table> | ||
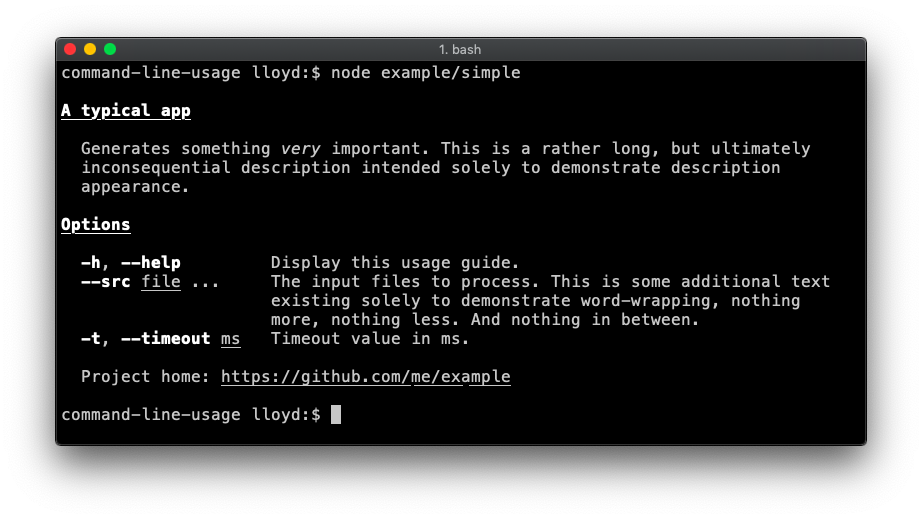 | ||
 | ||
<a name="exp_module_usage-options--UsageOptions"></a> | ||
@@ -33,2 +111,3 @@ ## UsageOptions ⏏ | ||
* [UsageOptions](#exp_module_usage-options--UsageOptions) ⏏ | ||
* [.header](#module_usage-options--UsageOptions+header) : <code>string</code> | ||
* [.title](#module_usage-options--UsageOptions+title) : <code>string</code> | ||
@@ -42,2 +121,7 @@ * [.description](#module_usage-options--UsageOptions+description) : <code>string</code> | ||
<a name="module_usage-options--UsageOptions+header"></a> | ||
### options.header : <code>string</code> | ||
Use this field to display a banner or header above the main body. | ||
**Kind**: instance property of <code>[UsageOptions](#exp_module_usage-options--UsageOptions)</code> | ||
<a name="module_usage-options--UsageOptions+title"></a> | ||
@@ -117,15 +201,4 @@ ### options.title : <code>string</code> | ||
## More examples | ||
You can see output from the examples in the [examples](https://github.com/75lb/command-line-usage/tree/master/example) folder using the test harness. To install: | ||
``` | ||
$ npm install -g command-line-usage | ||
``` | ||
Usage: | ||
``` | ||
$ cat example/typical-formatted.js | command-line-usage | ||
``` | ||
* * * | ||
© 2015 Lloyd Brookes \<75pound@gmail.com\>. Documented by [jsdoc-to-markdown](https://github.com/75lb/jsdoc-to-markdown). |
@@ -1,9 +0,50 @@ | ||
var test = require("tape"); | ||
var usage = require("../"); | ||
var cliOptions = require("../example/simple"); | ||
var test = require('tape') | ||
var getUsage = require('../') | ||
test("basic", function(t){ | ||
var result = usage(cliOptions.definitions, cliOptions.options); | ||
t.ok(/a typical app/.test(result)); | ||
t.end(); | ||
}); | ||
test('getUsage(definitions, options)', function (t) { | ||
var definitions = [ | ||
{ | ||
name: 'help', description: 'Display this usage guide.', | ||
alias: 'h', type: Boolean, group: 'one' | ||
}, | ||
{ | ||
name: 'src', description: 'The input files to process', | ||
type: String, multiple: true, defaultOption: true, group: 'one' | ||
}, | ||
{ | ||
name: 'timeout', description: 'Timeout value in ms', | ||
alias: 't', type: Number | ||
} | ||
] | ||
var options = { | ||
title: 'a typical app', | ||
description: 'Generates something very important.' | ||
} | ||
var result = getUsage(definitions, options) | ||
t.ok(/a typical app/.test(result)) | ||
t.end() | ||
}) | ||
test('getUsage.optionList()', function (t) { | ||
var definitions = [ | ||
{ name: 'one', description: 'one', group: 'one' }, | ||
{ name: 'two', description: 'two', group: 'one' }, | ||
{ name: 'three', description: 'three' } | ||
] | ||
t.deepEqual(getUsage.optionList(definitions), [ | ||
' \x1b[1m--one\x1b[0m one ', | ||
' \x1b[1m--two\x1b[0m two ', | ||
' \x1b[1m--three\x1b[0m three ' | ||
]) | ||
t.deepEqual(getUsage.optionList(definitions, 'one'), [ | ||
' \x1b[1m--one\x1b[0m one ', | ||
' \x1b[1m--two\x1b[0m two ' | ||
]) | ||
t.deepEqual(getUsage.optionList(definitions, '_none'), [ | ||
' \x1b[1m--three\x1b[0m three ' | ||
]) | ||
t.end() | ||
}) |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
Dynamic require
Supply chain riskDynamic require can indicate the package is performing dangerous or unsafe dynamic code execution.
Found 1 instance in 1 package
Filesystem access
Supply chain riskAccesses the file system, and could potentially read sensitive data.
Found 1 instance in 1 package
438058
30
765
202
1
1
+ Addedfeature-detect-es6@^1.0.0
- Removedarray-tools@^2
- Removedarray-tools@2.0.9(transitive)
- Removedcolumn-layout@1.3.0(transitive)
- Removedcommand-line-usage@2.0.5(transitive)
- Removedcore-js@1.2.7(transitive)
- Removedfilter-where@1.0.1(transitive)
- Removedreduce-extract@1.0.0(transitive)
- Removedreduce-flatten@1.0.1(transitive)
- Removedreduce-unique@1.0.0(transitive)
- Removedreduce-without@1.0.1(transitive)
- Removedsort-array@1.1.2(transitive)
Updatedcolumn-layout@^2