What is command-line-usage?
The command-line-usage npm package is used to generate usage guides for command-line applications. It helps in creating structured and styled help text that can be displayed when users need assistance with the command-line interface (CLI) of your application.
What are command-line-usage's main functionalities?
Defining Sections
This feature allows you to define different sections of the usage guide, such as headers and options. The code sample demonstrates how to create a usage guide with a description and options.
const commandLineUsage = require('command-line-usage');
const sections = [
{
header: 'My App',
content: 'This is a description of my app.'
},
{
header: 'Options',
optionList: [
{
name: 'help',
typeLabel: '{underline boolean}',
description: 'Display this usage guide.'
},
{
name: 'src',
typeLabel: '{underline file}',
description: 'The input file to process.'
}
]
}
];
const usage = commandLineUsage(sections);
console.log(usage);
Customizing Option Descriptions
This feature allows you to customize the descriptions of the options in your CLI application. The code sample shows how to define options with aliases and types, along with their descriptions.
const commandLineUsage = require('command-line-usage');
const sections = [
{
header: 'Options',
optionList: [
{
name: 'verbose',
alias: 'v',
type: Boolean,
description: 'Enable verbose mode.'
},
{
name: 'timeout',
alias: 't',
type: Number,
description: 'Set the timeout value in ms.'
}
]
}
];
const usage = commandLineUsage(sections);
console.log(usage);
Adding Examples
This feature allows you to add examples to your usage guide, making it easier for users to understand how to use your CLI application. The code sample demonstrates how to add concise and detailed examples.
const commandLineUsage = require('command-line-usage');
const sections = [
{
header: 'Examples',
content: [
{
desc: '1. A concise example.',
example: '$ app -v'
},
{
desc: '2. A long example.',
example: '$ app --timeout 1000'
}
]
}
];
const usage = commandLineUsage(sections);
console.log(usage);
Other packages similar to command-line-usage
yargs
Yargs is a popular library for building command-line interfaces. It provides extensive functionality for parsing arguments, generating usage information, and handling commands. Compared to command-line-usage, yargs offers more comprehensive features for argument parsing and command handling.
commander
Commander is another widely-used library for building command-line interfaces. It allows you to define commands, options, and usage information. While it provides similar functionality to command-line-usage, it is more focused on command and option parsing rather than generating usage guides.
oclif
Oclif is a framework for building command-line tools. It provides a robust set of features for creating complex CLI applications, including argument parsing, command handling, and generating help text. Oclif is more comprehensive and suited for larger CLI projects compared to command-line-usage.

command-line-usage
Exports a single function to generate a usage guide using column-layout.
getUsage(definitions, options) ⇒ string
⏏
Kind: Exported function
Param | Type | Description |
---|
definitions | Array.<optionDefinition> | an array of option definition objects. In addition to the regular definition properties, command-line-usage will look for: - description - a string describing the option. - typeLabel - a string to replace the default type string (e.g. <string> ). It's often more useful to set a more descriptive type label, like <ms> , <files> , <command> etc. |
options | usage-options | see UsageOptions. |
Example
Some example usage output:
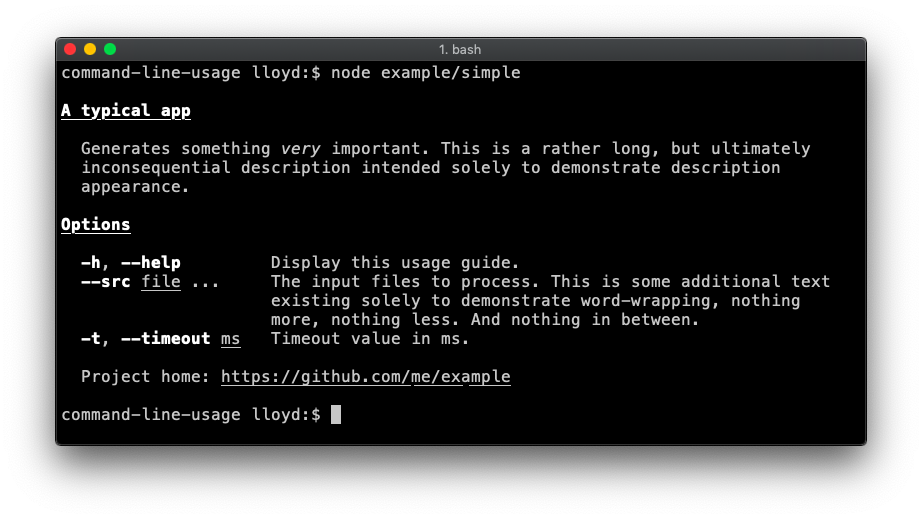

UsageOptions ⏏
The class describes all valid options for the getUsage
function. Inline formatting can be used within any text string supplied using valid ansi-escape-sequences formatting syntax.
Kind: Exported class
options.title : string
The title line at the top of the usage, typically the name of the app. By default it is underlined but this formatting can be overridden by passing a module:usage-options~textObject.
Kind: instance property of UsageOptions
Example
{
title: "my-app"
}
Example
{
title: {
text: "my-app",
format: [ "bold", "underline" ]
}
}
options.description : string
A description to go underneath the title. For example, some words about what the app is for.
Kind: instance property of UsageOptions
options.synopsis : Array.<string>
An array of strings highlighting the main usage forms of the app.
Kind: instance property of UsageOptions
options.groups : object
Specify which groups to display in the output by supplying an object of key/value pairs, where the key is the name of the group to include and the value is a string or textObject. If the value is a string it is used as the group title. Alternatively supply an object containing a title
and description
string.
Kind: instance property of UsageOptions
Example
{
main: {
title: "Main options",
description: "This group contains the most important options."
},
misc: "Miscellaneous"
}
options.examples : Array.<string>
| Array.<object>
Examples
Kind: instance property of UsageOptions
Displayed at the foot of the usage output.
Kind: instance property of UsageOptions
Example
{
footer: "Project home: [underline]{https://github.com/me/my-app}"
}
options.hide : string
| Array.<string>
If you want to hide certain options from the output, specify their names here. This is sometimes used to hide the defaultOption
.
Kind: instance property of UsageOptions
Example
{
hide: "files"
}
More examples
You can see output from the examples in the examples folder using the test harness. To install:
$ npm install -g command-line-usage
Usage:
$ cat example/typical-formatted.js | command-line-usage
© 2015 Lloyd Brookes <75pound@gmail.com>. Documented by jsdoc-to-markdown.