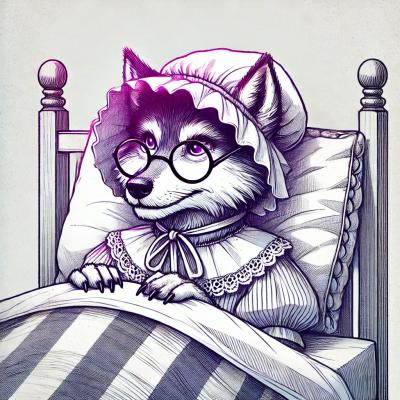
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
comment-parser
Advanced tools
The comment-parser npm package is a tool designed to parse comments from source code files into a structured format. It primarily focuses on extracting JSDoc-like comment blocks and converting them into a JSON object, making it easier to analyze and manipulate documentation embedded within code.
Parsing JSDoc comments
This feature allows the extraction of JSDoc comments from a string of source code. The output is an array of objects, each representing a parsed comment block with details about parameters, return types, and descriptions.
const parse = require('comment-parser');
const sourceCode = `/**
* This is a description of the function
* @param {string} name - The name of the person
* @return {string} - Greeting message
*/
function greet(name) { return 'Hello ' + name; }`;
const parsedComments = parse(sourceCode);
console.log(parsedComments);
Custom tags parsing
This feature supports parsing custom tags within comments. Users can configure the parser to handle specific tag formats and preserve spacing, allowing for more flexible documentation styles.
const parse = require('comment-parser');
const options = { dotted_names: false, spacing: 'preserve' };
const sourceCode = `/**
* @customTag Here is some custom information
*/`;
const parsedComments = parse(sourceCode, options);
console.log(parsedComments);
JSDoc is a popular tool similar to comment-parser but with a broader scope. It not only parses comments but also generates entire websites of documentation from the parsed data. JSDoc supports a wide range of tags and offers more extensive configuration options compared to comment-parser.
Doctrine is another npm package that parses JSDoc comments. It is somewhat similar to comment-parser but focuses more on the syntactic structure of the comments rather than converting them into a structured format. Doctrine is often used for linting tools and other development utilities.
Generic JSDoc-like comment parser. This library is not intended to be documentation generator, but rather composite unit for it.
npm install comment-parser
Module provides parse(s:String[, opts:Object]):Object
function which takes /** ... */
comment string and returns array of objects with parsed data.
It is not trying to detect relations between tags or somehow recognize their meaning. Any tag can be used, as long as it satisfies the format.
/**
* Singleline or multiline description text. Line breaks are preserved.
*
* @some-tag {Type} name Singleline or multiline description text
* @some-tag {Type} name.subname Singleline or multiline description text
* @some-tag {Type} name.subname.subsubname Singleline or
* multiline description text
* @another-tag
*/
this would be parsed into following
[{
"tags": [{
"tag": "some-tag",
"type": "Type",
"name": "name",
"optional": false,
"description": "Singleline or multiline description text",
"line": 3,
"source": "@some-tag {Type} name Singleline or multiline description text"
}, {
"tag": "some-tag",
"type": "Type",
"name": "name.subname",
"optional": false,
"description": "Singleline or multiline description text",
"line": 4,
"source": "@some-tag {Type} name.subname Singleline or multiline description text"
}, {
"tag": "some-tag",
"type": "Type",
"name": "name.subname.subsubname",
"optional": false,
"description": "Singleline or\nmultiline description text",
"line": 5,
"source": "@some-tag {Type} name.subname.subsubname Singleline or\nmultiline description text"
}, {
"tag": "another-tag",
"name": "",
"optional": false,
"type": "",
"description": "",
"line": 7,
"source": "@another-tag"
}],
"line": 0,
"description": "Singleline or multiline description text. Line breaks are preserved.",
"source": "Singleline or multiline description text. Line breaks are preserved.\n\n@some-tag {Type} name Singleline or multiline description text\n@some-tag {Type} name.subname Singleline or multiline description text\n@some-tag {Type} name.subname.subsubname Singleline or\nmultiline description text\n@another-tag"
}]
By default dotted names like name.subname.subsubname
will be expanded into nested sections, this can be prevented by passing opts.dotted_names = false
.
Invalid comment blocks are skipped. Comments starting with /*
and /***
are considered not valid.
Also you can parse entire file with parse.file('path/to/file', callback)
or acquire an instance of Transform stream with parse.stream()
.
In case you need to parse tags in different way you can pass opts.parsers = [parser1, ..., parserN]
, where each parser is function name(str:String, data:Object):{source:String, data:Object}
.
Each parser function takes string left after previous parsers applied and data produced by them. And returns null
or {source: '', data:{}}
where source
is consumed substring and data
is a payload with tag node fields.
Tag node data is build by merging result bits from all parsers. Here is some example that is not doing actual parsing but is demonstrating the flow:
/**
* Source to be parsed below
* @tag {type} name Description
*/
parse(source, {parsers: [
// takes entire string
function parse_tag(str, data) {
return {source: ' @tag', data: {tag: 'tag'}};
},
// parser throwing exception
function check_tag(str, data) {
if (allowed_tags.indexOf(data.tag) === -1) {
throw new Error('Unrecognized tag "' + data.tag + '"');
}
},
// takes the rest of the string after ' @tag''
function parse_name1(str, data) {
return {source: ' name', data: {name: 'name'}};
},
// alternative name parser
function parse_name2(str, data) {
return {source: ' name', data: {name: 'name'}};
}
]});
This would produce following:
[{
"tags": [{
"tag": "tag",
"type": "type",
"name": "name",
"optional": false,
"description": "Description",
"line": 2,
"source": "@tag {type} name Description"
}],
"line": 0,
"description": "Source to be parsed below",
"source": "Source to be parsed below\n@tag {type} name Description"
}]
Happy coding :)
v0.3.0
feature
allow to use custom parsersfeature
always include source, no raw_value
option neededbugfix
always provide optional
tag propertyrefactor
clean up testsFAQs
Generic JSDoc-like comment parser
The npm package comment-parser receives a total of 1,222,005 weekly downloads. As such, comment-parser popularity was classified as popular.
We found that comment-parser demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.