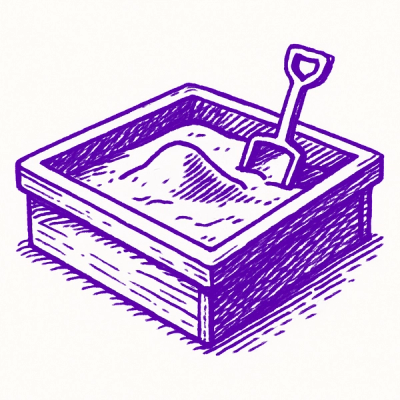
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
compute-indexspace
Advanced tools
Generates a linearly spaced index array from a subsequence string.
Generates a linearly spaced index array from a subsequence string.
$ npm install compute-indexspace
For use in the browser, use browserify.
var indexspace = require( 'compute-indexspace' );
Generates a linearly spaced index array
from a subsequence string
. len
specifies the reference array
length, which is needed to properly interpret the subsequence string
. If len = 0
, the function returns an empty array
.
var arr = indexspace( ':', 5 );
// returns [ 0, 1, 2, 3, 4 ]
arr = indexspace( ':', 0 );
// returns []
The subsequence string
syntax is similar to Python's slice notation.
var str = '<start>:<stop>:<increment>';
Notes about the notation:
increment
is not specified, the default increment is 1
. An increment
of zero is not allowed.start
index is inclusive, while the stop
index is exclusive.start
and stop
indices are optional. If not provided, start
and stop
default to index extremes.start
and stop
can be negative, in which case the index is subtracted from len
.var arr = indexspace( '-3:', 5 );
// returns [ 2, 3, 4 ];
arr = indexspace( ':-2', 5 );
// returns [ 0, 1, 2 ]
The function also recognizes the end
keyword, which refers to the last index; i.e., len-1
. If specified as the stop
index, end
is inclusive and equivalent to <start>::<increment>
.
var arr = indexspace( 'end::-1', 5 );
// returns [ 4, 3, 2, 1, 0 ]
arr = indexspace( ':end', 5 );
// returns [ 0, 1, 2, 3, 4 ]
Basic arithmetic (subtraction and division) may be performed on the end
keyword. The result from division is rounded up to the next integer.
var arr = indexspace( 'end-2::-1', 5 );
// returns [ 2, 1, 0 ];
arr = indexspace( ':end/2', 5 );
// returns [ 0, 1 ]
arr = indexspace( 'end/2:', 5 );
// returns [ 2, 3, 4 ]
arr = indexspace( 'end/3::-1', 5 );
// returns [ 2, 1, 0 ];
arr = indexspace( '1:end:2', 5 );
// returns [ 1, 3 ];
Note: unlike Matlab, but like Python, the subsequence string
is upper-bound exclusive. For example, in Python, 0:2
corresponds to the index array [0,1]
. In Matlab, 1:3
corresponds to [1,2,3]
.
This implementation chooses to follow the Python convention such that :n
combined with n:
is equivalent to :
. Using the Matlab convention, the two subsequences would overlap by one element.
var indexspace = require( 'compute-indexspace' );
var arr = indexspace( ':', 5 );
// returns [ 0, 1, 2, 3, 4 ]
arr = indexspace( '2:', 5 );
// returns [ 2, 3, 4 ]
arr = indexspace( ':3', 5 );
// returns [ 0, 1, 2 ]
arr = indexspace( '2:4', 5 );
// returns [ 2, 3 ]
arr = indexspace( '1:4:2', 5 );
// returns [ 1, 3 ]
arr = indexspace( '2::2', 5 );
// returns [ 2, 4 ]
arr = indexspace( ':10:3', 20 );
// returns [ 0, 3, 6, 9 ]
arr = indexspace( ':-2', 5 );
// returns [ 0, 1, 2 ]
arr = indexspace( ':-1:2', 5 );
// returns [ 0, 2 ]
arr = indexspace( '-4:-1:2', 5 );
// returns [ 1, 3 ]
arr = indexspace( '-5:-1', 5 );
// returns [ 0, 1, 2, 3 ]
arr = indexspace( '::-1', 5 );
// returns [ 4, 3, 2, 1, 0 ]
arr = indexspace( ':0:-1', 5 );
// returns [ 4, 3, 2, 1 ]
arr = indexspace( '3:0:-1', 5 );
// returns [ 3, 2, 1 ]
arr = indexspace( '-1:-4:-2', 5 );
// returns [ 4, 2 ]
arr = indexspace( ':end', 5 );
// returns [ 0, 1, 2, 3, 4 ]
arr = indexspace( ':end-1', 5 );
// returns [ 0, 1, 2, 3 ]
arr = indexspace( ':end/2', 5 );
// returns [ 0, 1 ]
arr = indexspace( 'end-2::-1', 5 );
// returns [ 2, 1, 0 ]
arr = indexspace( 'end/2:', 5 );
// returns [ 2, 3, 4 ]
To run the example code from the top-level application directory,
$ node ./examples/index.js
The motivation for this module stems from wanting to create an API for arrays
similar to Python and Matlab; e.g., A = B[1:6:2];
. JavaScript only supports basic indexing; e.g., A = B[3];
.
The workaround provided by this module is to express the subsequence syntax as a string
, which, when provided with a reference array
length, is parsed and then converted into an index array
. A consumer can then iterate through the index array
to extract the desired elements.
var indexspace = require( 'compute-indexspace' );
// Create an array...
var len = 10,
arr;
arr = new Array( len );
for ( var i = 0; i < len; i++ ) {
arr[ i ] = i;
}
// Create an index array...
var idx = indexspace( '::-1', len );
// From the original array, create a reversed array...
var rev = new Array( len );
for ( var j = 0; j < len; j++ ) {
rev[ j ] = arr[ idx[j] ];
}
console.log( arr.join( ',' ) );
console.log( rev.join( ',' ) );
Unit tests use the Mocha test framework with Chai assertions. To run the tests, execute the following command in the top-level application directory:
$ make test
All new feature development should have corresponding unit tests to validate correct functionality.
This repository uses Istanbul as its code coverage tool. To generate a test coverage report, execute the following command in the top-level application directory:
$ make test-cov
Istanbul creates a ./reports/coverage
directory. To access an HTML version of the report,
$ make view-cov
Copyright © 2015. Athan Reines.
FAQs
Generates a linearly spaced index array from a subsequence string.
The npm package compute-indexspace receives a total of 2,681 weekly downloads. As such, compute-indexspace popularity was classified as popular.
We found that compute-indexspace demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.