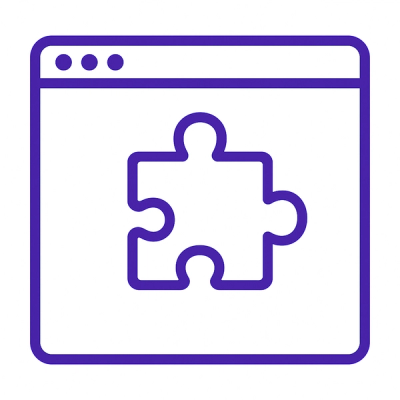
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
The concat-map package is a simple utility that applies a function to each item in an array and concatenates the results into a new array. It is similar to the Array.prototype.map function combined with Array.prototype.concat, but it is implemented in a way that is compatible with older versions of JavaScript and can be used in various Node.js environments.
Mapping and concatenating
This feature allows you to map each element of an array to a new array and then concatenate those arrays into a single array. In the code sample, each number is mapped to an array containing the number itself and its double, resulting in a new array [1, 2, 2, 4, 3, 6].
[1, 2, 3].concatMap(function (x) { return [x, x * 2]; })
The flat-map package offers similar functionality to concat-map, allowing you to map and flatten arrays in a single operation. It is a more modern alternative that may use Array.prototype.flatMap if available, providing better performance in environments that support it.
This package is a polyfill for the Array.prototype.flatMap method, which is part of the ES2019 specification. It provides similar functionality to concat-map but is intended to mimic the native implementation as closely as possible, including adherence to the ECMAScript specification.
Concatenative mapdashery.
var concatMap = require('concat-map');
var xs = [ 1, 2, 3, 4, 5, 6 ];
var ys = concatMap(xs, function (x) {
return x % 2 ? [ x - 0.1, x, x + 0.1 ] : [];
});
console.dir(ys);
[ 0.9, 1, 1.1, 2.9, 3, 3.1, 4.9, 5, 5.1 ]
var concatMap = require('concat-map')
Return an array of concatenated elements by calling fn(x, i)
for each element
x
and each index i
in the array xs
.
When fn(x, i)
returns an array, its result will be concatenated with the
result array. If fn(x, i)
returns anything else, that value will be pushed
onto the end of the result array.
With npm do:
npm install concat-map
MIT
This module was written while sitting high above the ground in a tree.
v0.0.2 - 2022-10-12
7015b7b
e31ccb4
95b4764
tape
a068899
e965df9
auto-changelog
eee5dc2
npmignore
to autogenerate an npmignore file 4a8e034
c39fa6c
a321524
safe-publish-latest
bd95e66
aud
in posttest
075e7f9
ac5bd7a
funding
in package.json 717b783
FAQs
concatenative mapdashery
The npm package concat-map receives a total of 55,267,813 weekly downloads. As such, concat-map popularity was classified as popular.
We found that concat-map demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.