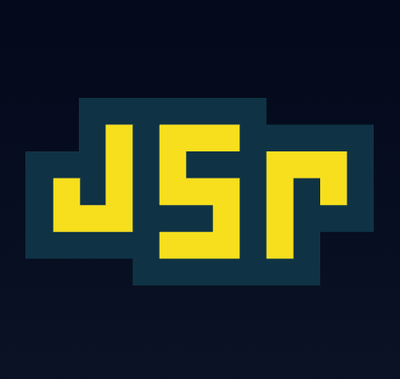
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
concurrently
Advanced tools
The 'concurrently' npm package is a utility that allows you to run multiple commands concurrently. It is often used to run multiple processes during development, such as a server and a client application at the same time. It can handle the output of these processes, terminate them all together, and more.
Running multiple commands
This feature allows you to run multiple commands at the same time. Each command is quoted and separated by a space.
concurrently "command1 arg" "command2 arg"
Customizing the prefix for command output
This feature allows you to customize the prefix shown in the output for each command. In this example, 'API' and 'UI' are custom prefixes for the two npm scripts.
concurrently --names "API,UI" "npm:api" "npm:start"
Killing all commands when one of them exits
This feature ensures that if one command exits, all other commands are also terminated. This is useful for cleaning up processes if one fails.
concurrently --kill-others "command1" "command2"
Running commands sequentially
This feature allows you to run commands sequentially instead of concurrently. The --success option determines which command's exit code will be used as the exit code for concurrently.
concurrently "command1" "command2" --success first
A CLI tool to run multiple npm-scripts in parallel or sequential. It is similar to concurrently but specifically designed for npm scripts. It provides a simpler interface for running scripts in series or parallel.
PM2 is a production process manager for Node.js applications with a built-in load balancer. It is more feature-rich than concurrently, providing a daemon process that helps keep applications alive forever, facilitates common system admin tasks, and can be used in production environments.
Foreman is a manager for Procfile-based applications. Its focus is on easing the development of applications by allowing you to run multiple processes with a single command. It is not limited to Node.js and can be used with any language or markup.
Run multiple commands concurrently.
Like npm run watch-js & npm run watch-less
but better.
Table of contents
I like task automation with npm
but the usual way to run multiple commands concurrently is
npm run watch-js & npm run watch-css
. That's fine but it's hard to keep
on track of different outputs. Also if one process fails, others still keep running
and you won't even notice the difference.
Another option would be to just run all commands in separate terminals. I got tired of opening terminals and made concurrently.
Features:
--kill-others
switch, all commands are killed if one diesThe tool is written in Node.js, but you can use it to run any commands.
npm install -g concurrently
or if you are using it from npm scripts:
npm install concurrently --save
Remember to surround separate commands with quotes:
concurrently "command1 arg" "command2 arg"
Otherwise concurrently would try to run 4 separate commands:
command1
, arg
, command2
, arg
.
In package.json, escape quotes:
"start": "concurrently \"command1 arg\" \"command2 arg\""
NPM run commands can be shortened:
concurrently "npm:watch-js" "npm:watch-css" "npm:watch-node"
# Equivalent to:
concurrently -n watch-js,watch-css,watch-node "npm run watch-js" "npm run watch-css" "npm run watch-node"
NPM shortened commands also support wildcards. Given the following scripts in package.json:
{
//...
"scripts": {
// ...
"watch-js": "...",
"watch-css": "...",
"watch-node": "...",
// ...
},
// ...
}
concurrently "npm:watch-*"
# Equivalent to:
concurrently -n js,css,node "npm run watch-js" "npm run watch-css" "npm run watch-node"
# Any name provided for the wildcard command will be used as a prefix to the wildcard
# part of the script name:
concurrently -n w: npm:watch-*
# Equivalent to:
concurrently -n w:js,w:css,w:node "npm run watch-js" "npm run watch-css" "npm run watch-node"
Good frontend one-liner example here.
Help:
concurrently [options] <command ...>
General
-m, --max-processes How many processes should run at once.
New processes only spawn after all restart tries of a
process. [number]
-n, --names List of custom names to be used in prefix template.
Example names: "main,browser,server" [string]
--name-separator The character to split <names> on. Example usage:
concurrently -n "styles|scripts|server" --name-separator
"|" [default: ","]
-r, --raw Output only raw output of processes, disables prettifying
and concurrently coloring. [boolean]
-s, --success Return exit code of zero or one based on the success or
failure of the "first" child to terminate, the "last
child", or succeed only if "all" child processes succeed.
[choices: "first", "last", "all"] [default: "all"]
--no-color Disables colors from logging [boolean]
Prefix styling
-p, --prefix Prefix used in logging for each process.
Possible values: index, pid, time, command, name,
none, or a template. Example template: "{time}-{pid}"
[string] [default: index or name (when --names is set)]
-c, --prefix-colors Comma-separated list of chalk colors to use on
prefixes. If there are more commands than colors, the
last color will be repeated.
- Available modifiers: reset, bold, dim, italic,
underline, inverse, hidden, strikethrough
- Available colors: black, red, green, yellow, blue,
magenta, cyan, white, gray, or any hex values for
colors, eg #23de43
- Available background colors: bgBlack, bgRed,
bgGreen, bgYellow, bgBlue, bgMagenta, bgCyan, bgWhite
See https://www.npmjs.com/package/chalk for more
information. [string] [default: "reset"]
-l, --prefix-length Limit how many characters of the command is displayed
in prefix. The option can be used to shorten the
prefix when it is set to "command"
[number] [default: 10]
-t, --timestamp-format Specify the timestamp in moment/date-fns format.
[string] [default: "yyyy-MM-dd HH:mm:ss.SSS"]
Input handling
-i, --handle-input Whether input should be forwarded to the child
processes. See examples for more information.[boolean]
--default-input-target Identifier for child process to which input on stdin
should be sent if not specified at start of input.
Can be either the index or the name of the process.
[default: 0]
Killing other processes
-k, --kill-others kill other processes if one exits or dies [boolean]
--kill-others-on-fail kill other processes if one exits with non zero status
code [boolean]
Restarting
--restart-tries How many times a process that died should restart.
Negative numbers will make the process restart forever.
[number] [default: 0]
--restart-after Delay time to respawn the process, in milliseconds.
[number] [default: 0]
Options:
-h, --help Show help [boolean]
-v, -V, --version Show version number [boolean]
Examples:
- Output nothing more than stdout+stderr of child processes
$ concurrently --raw "npm run watch-less" "npm run watch-js"
- Normal output but without colors e.g. when logging to file
$ concurrently --no-color "grunt watch" "http-server" > log
- Custom prefix
$ concurrently --prefix "{time}-{pid}" "npm run watch" "http-server"
- Custom names and colored prefixes
$ concurrently --names "HTTP,WATCH" -c "bgBlue.bold,bgMagenta.bold"
"http-server" "npm run watch"
- Send input to default
$ concurrently --handle-input "nodemon" "npm run watch-js"
rs # Sends rs command to nodemon process
- Send input to specific child identified by index
$ concurrently --handle-input "npm run watch-js" nodemon
1:rs
- Send input to specific child identified by name
$ concurrently --handle-input -n js,srv "npm run watch-js" nodemon
srv:rs
- Shortened NPM run commands
$ concurrently npm:watch-node npm:watch-js npm:watch-css
- Shortened NPM run command with wildcard
$ concurrently npm:watch-*
For more details, visit https://github.com/open-cli-tools/concurrently
concurrently can be used programmatically by using the API documented below:
concurrently(commands[, options])
commands
: an array of either strings (containing the commands to run) or objects
with the shape { command, name, prefixColor, env, cwd }
.
options
(optional): an object containing any of the below:
cwd
: the working directory to be used by all commands. Can be overriden per command.
Default: process.cwd()
.defaultInputTarget
: the default input target when reading from inputStream
.
Default: 0
.handleInput
: when true
, reads input from process.stdin
.inputStream
: a Readable
stream
to read the input from. Should only be used in the rare instance you would like to stream anything other than process.stdin
. Overrides handleInput
.pauseInputStreamOnFinish
: by default, pauses the input stream (process.stdin
when handleInput
is enabled, or inputStream
if provided) when all of the processes have finished. If you need to read from the input stream after concurrently
has finished, set this to false
. (#252).killOthers
: an array of exitting conditions that will cause a process to kill others.
Can contain any of success
or failure
.maxProcesses
: how many processes should run at once.outputStream
: a Writable
stream
to write logs to. Default: process.stdout
.prefix
: the prefix type to use when logging processes output.
Possible values: index
, pid
, time
, command
, name
, none
, or a template (eg [{time} process: {pid}]
).
Default: the name of the process, or its index if no name is set.prefixLength
: how many characters to show when prefixing with command
. Default: 10
raw
: whether raw mode should be used, meaning strictly process output will
be logged, without any prefixes, colouring or extra stuff.successCondition
: the condition to consider the run was successful.
If first
, only the first process to exit will make up the success of the run; if last
, the last process that exits will determine whether the run succeeds.
Anything else means all processes should exit successfully.restartTries
: how many attempts to restart a process that dies will be made. Default: 0
.restartDelay
: how many milliseconds to wait between process restarts. Default: 0
.timestampFormat
: a date-fns format
to use when prefixing with time
. Default: yyyy-MM-dd HH:mm:ss.ZZZ
Returns: a
Promise
that resolves if the run was successful (according tosuccessCondition
option), or rejects, containing an array of objects with information for each command that has been run, in the order that the commands terminated. The objects have the shape{ command, index, exitCode, killed }
, wherecommand
is the object passed in thecommands
array,index
its index there andkilled
indicates if the process was killed as a result ofkillOthers
. Default values (empty strings or objects) are returned for the fields that were not specified.
Example:
const concurrently = require('concurrently');
concurrently([
'npm:watch-*',
{ command: 'nodemon', name: 'server' },
{ command: 'deploy', name: 'deploy', env: { PUBLIC_KEY: '...' } },
{ command: 'watch', name: 'watch', cwd: path.resolve(__dirname, 'scripts/watchers')}
], {
prefix: 'name',
killOthers: ['failure', 'success'],
restartTries: 3,
cwd: path.resolve(__dirname, 'scripts'),
}).then(success, failure);
Process exited with code null?
From Node child_process documentation, exit
event:
This event is emitted after the child process ends. If the process terminated normally, code is the final exit code of the process, otherwise null. If the process terminated due to receipt of a signal, signal is the string name of the signal, otherwise null.
So null means the process didn't terminate normally. This will make concurrent to return non-zero exit code too.
Does this work with the npm-replacements yarn or pnpm?
Yes! In all examples above, you may replace "npm
" with "yarn
" or "pnpm
".
FAQs
Run commands concurrently
The npm package concurrently receives a total of 4,816,031 weekly downloads. As such, concurrently popularity was classified as popular.
We found that concurrently demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.