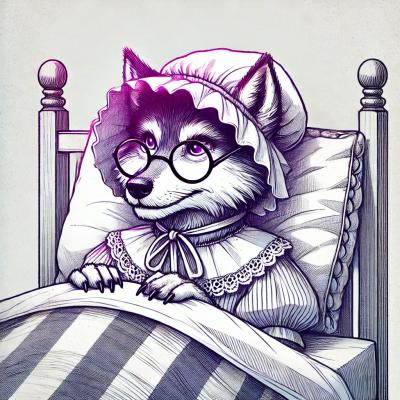
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The 'conf' npm package is a simple yet powerful configuration management tool for Node.js applications. It allows you to easily store and retrieve configuration data, manage defaults, and handle schema validation. The data is stored in a JSON file, making it easy to read and modify.
Store and Retrieve Configuration Data
This feature allows you to store and retrieve configuration data easily. The data is stored in a JSON file, and you can set and get values using simple methods.
const Conf = require('conf');
const config = new Conf();
// Set a value
config.set('unicorn', '🦄');
// Get a value
console.log(config.get('unicorn'));
//=> '🦄'
Manage Default Values
You can define default values for your configuration settings. If a key is not set, the default value will be returned.
const Conf = require('conf');
const config = new Conf({
defaults: {
foo: 'bar'
}
});
console.log(config.get('foo'));
//=> 'bar'
Schema Validation
You can define a schema for your configuration to ensure that the data meets certain criteria. This helps in maintaining data integrity and consistency.
const Conf = require('conf');
const schema = {
type: 'object',
properties: {
foo: {
type: 'string'
},
bar: {
type: 'number',
minimum: 0
}
}
};
const config = new Conf({ schema });
config.set('foo', 'baz');
config.set('bar', 42);
console.log(config.get('foo'));
//=> 'baz'
console.log(config.get('bar'));
//=> 42
Configstore is another package for managing configuration data in Node.js applications. It provides similar functionality to 'conf', such as storing and retrieving data, managing defaults, and handling schema validation. However, 'conf' offers a more modern API and better TypeScript support.
Node-persist is a simple, zero-dependency, key-value storage library for Node.js. It provides persistent storage for configuration data, similar to 'conf'. However, 'conf' offers more advanced features like schema validation and default values management.
Nconf is a hierarchical configuration manager for Node.js. It supports multiple configuration sources such as command-line arguments, environment variables, and JSON files. While 'nconf' is more flexible in terms of configuration sources, 'conf' is simpler and easier to use for most use cases.
Simple config handling for your app or module
All you have to care about is what to persist. This module will handle all the dull details like where and how.
$ npm install --save conf
const Conf = require('conf');
const config = new Conf();
config.set('unicorn', '🦄');
console.log(config.get('unicorn'));
//=> '🦄'
// Use dot-notation to access nested properties
config.set('foo.bar', true);
console.log(config.get('foo'));
//=> {bar: true}
config.delete('unicorn');
console.log(config.get('unicorn'));
//=> undefined
Returns a new instance.
Type: Object
Default config.
Type: string
Default: config
Name of the config file (without extension).
Useful if you need multiple config files for your app or module. For example, different config files between two major versions.
Type: string
Default: The name
field in the package.json closest to where conf
is imported.
You only need to specify this if you don't have a package.json file in your project.
Type: string
Default: System default user config directory
You most likely don't need this. Please don't use it unless you really have to.
Overrides projectName
.
The only use-case I can think of is having the config located in the app directory or on some external storage.
You can use dot-notation in a key
to access nested properties.
The instance is iterable
so you can use it directly in a for…of
loop.
Set an item.
Set multiple items at once.
Get an item or defaultValue
if the item does not exist.
Check if an item exists.
Delete an item.
Delete all items.
Get the item count.
Get all the config as an object or replace the current config with an object:
conf.store = {
hello: 'world'
};
Get the path to the config file.
configstore
?I'm also the author of configstore
. While it's pretty good, I did make some mistakes early on that are hard to change at this point. This module is the result of everything I learned from making configstore
. Mainly where config is stored. In configstore
, the config is stored in ~/.config
(which is mainly a Linux convention) on all systems, while conf
stores config in the system default user config directory. The ~/.config
directory, it turns out, often have an incorrect permission on macOS and Windows, which has caused a lot of grief for users.
MIT © Sindre Sorhus
FAQs
Simple config handling for your app or module
The npm package conf receives a total of 0 weekly downloads. As such, conf popularity was classified as not popular.
We found that conf demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.