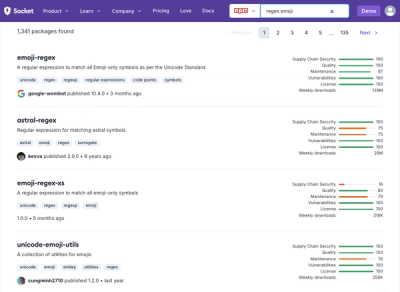
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
connect.io
Advanced tools
Real-time bidirectional event-based and Promise friendly communication in Chrome extensions or Apps inspired by Socket.IO.
Real-time bidirectional event-based and Promise friendly communication in Chrome extensions/apps inspired by Socket.IO.
If you build your project with Webpack, you can install connect.io via npm:
npm install connect.io
Then you can import it in your project:
// es6
import { createClient, createServer, send } from 'connect.io'
// commonjs
const { createClient, createServer, send } = require('connect.io')
Download chrome-connect.js from unpkg(min version).
Then reference it in your html:
<script src="path/to/chrome-connect.js"></script>
<!-- now you will get a global variable named chromeConnect -->
<script>
var createClient = chromeConnect.createClient
var createServer = chromeConnect.createServer
var send = chromeConnect.send
</script>
First, create a server in background page(or other pages like popup, options):
import { createServer } from 'connect.io'
const server = createServer()
Second, listen connect
event on server:
server.on('connect', client => {
// client is a Client object
})
Finally, create a client in content script:
import { createClient } from 'connect.io'
// client is an Client object
const client = createClient()
For more information about the Client
object please read the API below.
Only different with the above steps is: when you create client in background, you must specify the tab id in createClient
.
For example, in your background:
import { createClient } from 'connect.io'
const clientInBackground = createClient(1001) // the tab id you want to connect
Server can optional has a namespace:
import { createServer } from 'connect.io'
const serverTweets = createServer('tweets')
serverTweets.on('connect', client => {
client.on('tweet', tween => {
postTweet(tween)
})
})
const serverNotification = createServer('notification')
serverNotification.on('connect', client => {
client.on('notice', () => {
showNotification()
})
})
You can connect to different server in client:
import { createClient } from 'connect.io'
const clientTweets = createClient({
namespace: 'tweets'
})
clientTweets.send('tweet', 'connect.io is awesome')
const clientNotification = createClient({
namespace: 'notification'
})
clientNotification.send('notice')
You can send response from server to the client. For example:
// in server
import { createServer } from 'connect.io'
const server = createServer()
server.on('connect', client => {
client.on('Do I have enough money?', (data, resolve, reject) => {
if (data > 100) {
resolve('Yes you do.')
} else {
reject('You need more money.')
}
})
})
// in client
import { createClient } from 'connect.io'
const client = createClient()
// pass true as the last params to get response
client.send('Do I have enough money?', 50, true).catch(msg => {
console.log(msg) // 'You need more money.'
})
client.send('Do I have enough money?', 10000, true).then(msg => {
console.log(msg) // 'Yes you do.'
})
If you want to send one-time message and don't want to create a client, you can use send
method:
import { send } from 'connect.io'
send({
name: 'Do I have enough money?',
data: 10000,
needResponse: true
}).then(msg => {
console.log(msg) // 'Yes you do.'
})
Return a Server
object.
Note: same namespace get the same Server
object.
import { createServer } from 'connect.io'
createServer() === createServer() // true
createServer('namespace') === createServer('namespace') // true
createServer('foo') === createServer('bar') // false
Create from createServer
method.
Send message to all clients that connect to this server.
Listen event. For now there is only one event: connect
.
Return a Client
object.
If you want to connect to content script from background, then id
is necessary and must be a tab id.
options
has these property:
namespace
: which server you want connectframeId
: if you are connect to content script from background, you can specify a frameId to connect only this frame.Create from createClient
, or provided in server.on('connect')
event.
The native chrome Port object.
If this client is connect from webpage or other extension, then this property is true
.
Send message to server or client.
Receive message from server or client. Use resolve
or reject
method to response this message to server or client.
Note: this method only exist in server.
Sending a message to everyone else except for the connection that starts it.
Disconnect the connection.
Send one-time message.
The options
has these property:
id
: optional. If you want to connect to content script from background, then id
is necessary and must be a tab id.frameId
: optional. See createClient([id, options])
API.namespace
: optional. See createClient([id, options])
API.name
: the message name.data
: the data you want send.needResponse
: if you need response, then set this to true
MIT
FAQs
Real-time bidirectional event-based and Promise friendly communication in Chrome extensions or Apps inspired by Socket.IO.
We found that connect.io demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.