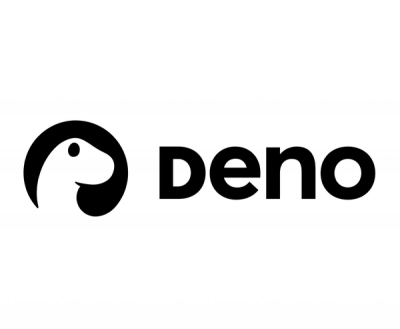
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
The connect npm package is a middleware layer for Node.js, designed to be used as a part of the 'http' module. It allows developers to create a series of middleware functions to handle requests and responses in a sequential manner. Connect is often used to set up middleware that can perform various tasks such as logging, parsing, session handling, and more.
Logging
This feature allows you to log every request that comes into the server with the method and URL.
const connect = require('connect');
const app = connect();
// Middleware for logging
function logger(req, res, next) {
console.log('%s %s', req.method, req.url);
next();
}
app.use(logger);
app.listen(3000);
Static File Serving
This feature serves static files from a specified directory, in this case, 'public'.
const connect = require('connect');
const serveStatic = require('serve-static');
const app = connect();
app.use(serveStatic('public'));
app.listen(3000);
Body Parsing
This feature allows you to parse the body of incoming requests in middleware before handling them.
const connect = require('connect');
const bodyParser = require('body-parser');
const app = connect();
app.use(bodyParser.json());
app.use(function(req, res) {
res.end(JSON.stringify(req.body));
});
app.listen(3000);
Cookie Parsing
This feature allows you to parse cookies attached to the client request object.
const connect = require('connect');
const cookieParser = require('cookie-parser');
const app = connect();
app.use(cookieParser());
app.use(function(req, res) {
res.end(JSON.stringify(req.cookies));
});
app.listen(3000);
Express is a web application framework for Node.js, built on top of connect. It extends connect's middleware model with additional functionality like routing, template engine support, and more. Express is more feature-rich and is considered a de facto standard for Node.js web applications.
Koa is a web framework designed by the creators of Express, aiming to be a smaller, more expressive, and more robust foundation for web applications and APIs. Koa uses async functions to eliminate callbacks and greatly increase error-handling capabilities. It does not bundle any middleware within its core, and it provides an elegant suite of methods that make writing servers fast and enjoyable.
Hapi is a rich framework for building applications and services. It enables developers to focus on writing reusable application logic instead of spending time building infrastructure. Hapi is known for its powerful plugin system and comprehensive API.
Restify is a Node.js web service framework optimized for building semantically correct RESTful web services ready for production use at scale. It is somewhat similar to Express but is more focused on enabling the building of correct REST web services.
Connect is an extensible HTTP server framework for node, providing high performance "plugins" known as middleware.
Connect is bundled with over 20 commonly used middleware, including a logger, session support, cookie parser, and more. Be sure to view the 2.x documentation.
var connect = require('connect')
, http = require('http');
var app = connect()
.use(connect.favicon('public/favicon.ico'))
.use(connect.logger('dev'))
.use(connect.static('public'))
.use(connect.directory('public'))
.use(connect.cookieParser())
.use(connect.session({ secret: 'my secret here' }))
.use(function(req, res){
res.end('Hello from Connect!\n');
});
http.createServer(app).listen(3000);
first:
$ npm install -d
then:
$ npm test
https://github.com/senchalabs/connect/graphs/contributors
Connect < 1.x
is compatible with node 0.2.x
Connect 1.x
is compatible with node 0.4.x
Connect 2.x
is compatible with node 0.8.x
Connect 3.x
is compatible with node 0.10.x
FAQs
High performance middleware framework
The npm package connect receives a total of 5,967,479 weekly downloads. As such, connect popularity was classified as popular.
We found that connect demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.