Comparing version 3.2.3 to 3.3.0
import { ConsolaOptions, ConsolaInstance } from './core.js'; | ||
export { Consola, ConsolaReporter, FormatOptions, InputLogObject, LogLevel, LogLevels, LogObject, LogType, LogTypes } from './core.js'; | ||
export { ConfirmPromptOptions, Consola, ConsolaReporter, FormatOptions, InputLogObject, LogLevel, LogLevels, LogObject, LogType, LogTypes, MultiSelectOptions, PromptOptions, SelectPromptOptions, TextPromptOptions } from './core.js'; | ||
/** | ||
* Factory function to create a new Consola instance | ||
* | ||
* @param {Partial<ConsolaOptions & { fancy: boolean }>} [options={}] - Optional configuration options. See {@link ConsolaOptions}. | ||
* @returns {ConsolaInstance} A new Consola instance configured with the given options. | ||
*/ | ||
declare function createConsola(options?: Partial<ConsolaOptions & { | ||
fancy: boolean; | ||
}>): ConsolaInstance; | ||
/** | ||
* Creates and exports a standard instance of Consola with the default configuration. | ||
* This instance can be used directly for logging throughout the application. | ||
* | ||
* @type {ConsolaInstance} consola - The default instance of Consola. | ||
*/ | ||
declare const consola: ConsolaInstance; | ||
export { ConsolaInstance, ConsolaOptions, consola, createConsola, consola as default }; |
import { ConsolaOptions, ConsolaInstance } from './core.js'; | ||
export { Consola, ConsolaReporter, FormatOptions, InputLogObject, LogLevel, LogLevels, LogObject, LogType, LogTypes } from './core.js'; | ||
export { ConfirmPromptOptions, Consola, ConsolaReporter, FormatOptions, InputLogObject, LogLevel, LogLevels, LogObject, LogType, LogTypes, MultiSelectOptions, PromptOptions, SelectPromptOptions, TextPromptOptions } from './core.js'; | ||
/** | ||
* Creates a new Consola instance configured specifically for browser environments. | ||
* This function sets up default reporters and a prompt method tailored to the browser's dialogue APIs. | ||
* | ||
* @param {Partial<ConsolaOptions>} [options={}] - Optional configuration options. | ||
* The options can override the default reporter and prompt behaviour. See {@link ConsolaOptions}. | ||
* @returns {ConsolaInstance} A new Consola instance optimised for use in browser environments. | ||
*/ | ||
declare function createConsola(options?: Partial<ConsolaOptions>): ConsolaInstance; | ||
/** | ||
* A standard Consola instance created with browser-specific configurations. | ||
* This instance can be used throughout a browser-based project. | ||
* | ||
* @type {ConsolaInstance} consola - The default browser-configured Consola instance. | ||
*/ | ||
declare const consola: ConsolaInstance; | ||
export { ConsolaInstance, ConsolaOptions, consola, createConsola, consola as default }; |
@@ -6,43 +6,185 @@ type SelectOption = { | ||
}; | ||
type TextOptions = { | ||
declare const kCancel: unique symbol; | ||
type PromptCommonOptions = { | ||
/** | ||
* Specify how to handle a cancelled prompt (e.g. by pressing Ctrl+C). | ||
* | ||
* Default strategy is `"default"`. | ||
* | ||
* - `"default"` - Resolve the promise with the `default` value or `initial` value. | ||
* - `"undefined`" - Resolve the promise with `undefined`. | ||
* - `"null"` - Resolve the promise with `null`. | ||
* - `"symbol"` - Resolve the promise with a symbol `Symbol.for("cancel")`. | ||
* - `"reject"` - Reject the promise with an error. | ||
*/ | ||
cancel?: "reject" | "default" | "undefined" | "null" | "symbol"; | ||
}; | ||
type TextPromptOptions = PromptCommonOptions & { | ||
/** | ||
* Specifies the prompt type as text. | ||
* @optional | ||
* @default "text" | ||
*/ | ||
type?: "text"; | ||
/** | ||
* The default text value. | ||
* @optional | ||
*/ | ||
default?: string; | ||
/** | ||
* A placeholder text displayed in the prompt. | ||
* @optional | ||
*/ | ||
placeholder?: string; | ||
/** | ||
* The initial text value. | ||
* @optional | ||
*/ | ||
initial?: string; | ||
}; | ||
type ConfirmOptions = { | ||
type ConfirmPromptOptions = PromptCommonOptions & { | ||
/** | ||
* Specifies the prompt type as confirm. | ||
*/ | ||
type: "confirm"; | ||
/** | ||
* The initial value for the confirm prompt. | ||
* @optional | ||
*/ | ||
initial?: boolean; | ||
}; | ||
type SelectOptions = { | ||
type SelectPromptOptions = PromptCommonOptions & { | ||
/** | ||
* Specifies the prompt type as select. | ||
*/ | ||
type: "select"; | ||
/** | ||
* The initial value for the select prompt. | ||
* @optional | ||
*/ | ||
initial?: string; | ||
/** | ||
* The options to select from. See {@link SelectOption}. | ||
*/ | ||
options: (string | SelectOption)[]; | ||
}; | ||
type MultiSelectOptions = { | ||
type MultiSelectOptions = PromptCommonOptions & { | ||
/** | ||
* Specifies the prompt type as multiselect. | ||
*/ | ||
type: "multiselect"; | ||
initial?: string; | ||
options: string[] | SelectOption[]; | ||
/** | ||
* The options to select from. See {@link SelectOption}. | ||
*/ | ||
initial?: string[]; | ||
/** | ||
* The options to select from. See {@link SelectOption}. | ||
*/ | ||
options: (string | SelectOption)[]; | ||
/** | ||
* Whether the prompt requires at least one selection. | ||
*/ | ||
required?: boolean; | ||
}; | ||
type PromptOptions = TextOptions | ConfirmOptions | SelectOptions | MultiSelectOptions; | ||
type inferPromptReturnType<T extends PromptOptions> = T extends TextOptions ? string : T extends ConfirmOptions ? boolean : T extends SelectOptions ? T["options"][number] : T extends MultiSelectOptions ? T["options"] : unknown; | ||
declare function prompt<_ = any, __ = any, T extends PromptOptions = TextOptions>(message: string, opts?: PromptOptions): Promise<inferPromptReturnType<T>>; | ||
/** | ||
* Defines a combined type for all prompt options. | ||
*/ | ||
type PromptOptions = TextPromptOptions | ConfirmPromptOptions | SelectPromptOptions | MultiSelectOptions; | ||
type inferPromptReturnType<T extends PromptOptions> = T extends TextPromptOptions ? string : T extends ConfirmPromptOptions ? boolean : T extends SelectPromptOptions ? T["options"][number] extends SelectOption ? T["options"][number]["value"] : T["options"][number] : T extends MultiSelectOptions ? T["options"] : unknown; | ||
type inferPromptCancalReturnType<T extends PromptOptions> = T extends { | ||
cancel: "reject"; | ||
} ? never : T extends { | ||
cancel: "default"; | ||
} ? inferPromptReturnType<T> : T extends { | ||
cancel: "undefined"; | ||
} ? undefined : T extends { | ||
cancel: "null"; | ||
} ? null : T extends { | ||
cancel: "symbol"; | ||
} ? typeof kCancel : inferPromptReturnType<T>; | ||
/** | ||
* Asynchronously prompts the user for input based on specified options. | ||
* Supports text, confirm, select and multi-select prompts. | ||
* | ||
* @param {string} message - The message to display in the prompt. | ||
* @param {PromptOptions} [opts={}] - The prompt options. See {@link PromptOptions}. | ||
* @returns {Promise<inferPromptReturnType<T>>} - A promise that resolves with the user's response, the type of which is inferred from the options. See {@link inferPromptReturnType}. | ||
*/ | ||
declare function prompt<_ = any, __ = any, T extends PromptOptions = TextPromptOptions>(message: string, opts?: PromptOptions): Promise<inferPromptReturnType<T> | inferPromptCancalReturnType<T>>; | ||
/** | ||
* Defines the level of logs as specific numbers or special number types. | ||
* | ||
* @type {0 | 1 | 2 | 3 | 4 | 5 | (number & {})} LogLevel - Represents the log level. | ||
* @default 0 - Represents the default log level. | ||
*/ | ||
type LogLevel = 0 | 1 | 2 | 3 | 4 | 5 | (number & {}); | ||
/** | ||
* A mapping of `LogType` to its corresponding numeric log level. | ||
* | ||
* @type {Record<LogType, number>} LogLevels - key-value pairs of log types to their numeric levels. See {@link LogType}. | ||
*/ | ||
declare const LogLevels: Record<LogType, number>; | ||
/** | ||
* Lists the types of log messages supported by the system. | ||
* | ||
* @type {"silent" | "fatal" | "error" | "warn" | "log" | "info" | "success" | "fail" | "ready" | "start" | "box" | "debug" | "trace" | "verbose"} LogType - Represents the specific type of log message. | ||
*/ | ||
type LogType = "silent" | "fatal" | "error" | "warn" | "log" | "info" | "success" | "fail" | "ready" | "start" | "box" | "debug" | "trace" | "verbose"; | ||
/** | ||
* Maps `LogType` to a `Partial<LogObject>`, primarily defining the log level. | ||
* | ||
* @type {Record<LogType, Partial<LogObject>>} LogTypes - key-value pairs of log types to partial log objects, specifying log levels. See {@link LogType} and {@link LogObject}. | ||
*/ | ||
declare const LogTypes: Record<LogType, Partial<LogObject>>; | ||
interface ConsolaOptions { | ||
/** | ||
* An array of ConsolaReporter instances used to handle and output log messages. | ||
*/ | ||
reporters: ConsolaReporter[]; | ||
/** | ||
* A record mapping LogType to InputLogObject, defining the log configuration for each log type. | ||
* See {@link LogType} and {@link InputLogObject}. | ||
*/ | ||
types: Record<LogType, InputLogObject>; | ||
/** | ||
* The minimum log level to output. See {@link LogLevel}. | ||
*/ | ||
level: LogLevel; | ||
/** | ||
* Default properties applied to all log messages unless overridden. See {@link InputLogObject}. | ||
*/ | ||
defaults: InputLogObject; | ||
/** | ||
* The maximum number of times a log message can be repeated within a given timeframe. | ||
*/ | ||
throttle: number; | ||
/** | ||
* The minimum time in milliseconds that must elapse before a throttled log message can be logged again. | ||
*/ | ||
throttleMin: number; | ||
/** | ||
* The Node.js writable stream for standard output. See {@link NodeJS.WriteStream}. | ||
* @optional | ||
*/ | ||
stdout?: NodeJS.WriteStream; | ||
/** | ||
* The Node.js writeable stream for standard error output. See {@link NodeJS.WriteStream}. | ||
* @optional | ||
*/ | ||
stderr?: NodeJS.WriteStream; | ||
/** | ||
* A function that allows you to mock log messages for testing purposes. | ||
* @optional | ||
*/ | ||
mockFn?: (type: LogType, defaults: InputLogObject) => (...args: any) => void; | ||
/** | ||
* Custom prompt function to use. It can be undefined. | ||
* @optional | ||
*/ | ||
prompt?: typeof prompt | undefined; | ||
/** | ||
* Configuration options for formatting log messages. See {@link FormatOptions}. | ||
*/ | ||
formatOptions: FormatOptions; | ||
@@ -54,26 +196,100 @@ } | ||
interface FormatOptions { | ||
/** | ||
* The maximum number of columns to output, affects formatting. | ||
* @optional | ||
*/ | ||
columns?: number; | ||
/** | ||
* Whether to include timestamp information in log messages. | ||
* @optional | ||
*/ | ||
date?: boolean; | ||
/** | ||
* Whether to use colors in the output. | ||
* @optional | ||
*/ | ||
colors?: boolean; | ||
/** | ||
* Specifies whether or not the output should be compact. Accepts a boolean or numeric level of compactness. | ||
* @optional | ||
*/ | ||
compact?: boolean | number; | ||
/** | ||
* Error cause level. | ||
*/ | ||
errorLevel?: number; | ||
/** | ||
* Allows additional custom formatting options. | ||
*/ | ||
[key: string]: unknown; | ||
} | ||
interface InputLogObject { | ||
/** | ||
* The logging level of the message. See {@link LogLevel}. | ||
* @optional | ||
*/ | ||
level?: LogLevel; | ||
/** | ||
* A string tag to categorise or identify the log message. | ||
* @optional | ||
*/ | ||
tag?: string; | ||
/** | ||
* The type of log message, which affects how it's processed and displayed. See {@link LogType}. | ||
* @optional | ||
*/ | ||
type?: LogType; | ||
/** | ||
* The main log message text. | ||
* @optional | ||
*/ | ||
message?: string; | ||
/** | ||
* Additional text or texts to be logged with the message. | ||
* @optional | ||
*/ | ||
additional?: string | string[]; | ||
/** | ||
* Additional arguments to be logged with the message. | ||
* @optional | ||
*/ | ||
args?: any[]; | ||
/** | ||
* The date and time when the log message was created. | ||
* @optional | ||
*/ | ||
date?: Date; | ||
} | ||
interface LogObject extends InputLogObject { | ||
/** | ||
* The logging level of the message, overridden if required. See {@link LogLevel}. | ||
*/ | ||
level: LogLevel; | ||
/** | ||
* The type of log message, overridden if required. See {@link LogType}. | ||
*/ | ||
type: LogType; | ||
/** | ||
* A string tag to categorise or identify the log message, overridden if necessary. | ||
*/ | ||
tag: string; | ||
/** | ||
* Additional arguments to be logged with the message, overridden if necessary. | ||
*/ | ||
args: any[]; | ||
/** | ||
* The date and time the log message was created, overridden if necessary. | ||
*/ | ||
date: Date; | ||
/** | ||
* Allows additional custom properties to be set on the log object. | ||
*/ | ||
[key: string]: unknown; | ||
} | ||
interface ConsolaReporter { | ||
/** | ||
* Defines how a log message is processed and displayed by this reporter. | ||
* @param logObj The LogObject containing the log information to process. See {@link LogObject}. | ||
* @param ctx An object containing context information such as options. See {@link ConsolaOptions}. | ||
*/ | ||
log: (logObj: LogObject, ctx: { | ||
@@ -84,2 +300,8 @@ options: ConsolaOptions; | ||
/** | ||
* Consola class for logging management with support for pause/resume, mocking and customisable reporting. | ||
* Provides flexible logging capabilities including level-based logging, custom reporters and integration options. | ||
* | ||
* @class Consola | ||
*/ | ||
declare class Consola { | ||
@@ -95,22 +317,129 @@ options: ConsolaOptions; | ||
_mockFn?: ConsolaOptions["mockFn"]; | ||
/** | ||
* Creates an instance of Consola with specified options or defaults. | ||
* | ||
* @param {Partial<ConsolaOptions>} [options={}] - Configuration options for the Consola instance. | ||
*/ | ||
constructor(options?: Partial<ConsolaOptions>); | ||
/** | ||
* Gets the current log level of the Consola instance. | ||
* | ||
* @returns {number} The current log level. | ||
*/ | ||
get level(): LogLevel; | ||
/** | ||
* Sets the minimum log level that will be output by the instance. | ||
* | ||
* @param {number} level - The new log level to set. | ||
*/ | ||
set level(level: LogLevel); | ||
prompt<T extends PromptOptions>(message: string, opts?: T): Promise<T extends TextOptions ? string : T extends ConfirmOptions ? boolean : T extends SelectOptions ? T["options"][number] : T extends MultiSelectOptions ? T["options"] : unknown>; | ||
/** | ||
* Displays a prompt to the user and returns the response. | ||
* Throw an error if `prompt` is not supported by the current configuration. | ||
* | ||
* @template T | ||
* @param {string} message - The message to display in the prompt. | ||
* @param {T} [opts] - Optional options for the prompt. See {@link PromptOptions}. | ||
* @returns {promise<T>} A promise that infer with the prompt options. See {@link PromptOptions}. | ||
*/ | ||
prompt<T extends PromptOptions>(message: string, opts?: T): Promise<(T extends TextPromptOptions ? string : T extends ConfirmPromptOptions ? boolean : T extends SelectPromptOptions ? T["options"][number] extends { | ||
label: string; | ||
value: string; | ||
hint?: string; | ||
} ? T["options"][number]["value"] : T["options"][number] : T extends MultiSelectOptions ? T["options"] : unknown) | (T extends { | ||
cancel: "reject"; | ||
} ? never : T extends { | ||
cancel: "default"; | ||
} ? T extends infer T_1 ? T_1 extends T ? T_1 extends TextPromptOptions ? string : T_1 extends ConfirmPromptOptions ? boolean : T_1 extends SelectPromptOptions ? T_1["options"][number] extends { | ||
label: string; | ||
value: string; | ||
hint?: string; | ||
} ? T_1["options"][number]["value"] : T_1["options"][number] : T_1 extends MultiSelectOptions ? T_1["options"] : unknown : never : never : T extends { | ||
cancel: "undefined"; | ||
} ? undefined : T extends { | ||
cancel: "null"; | ||
} ? null : T extends { | ||
cancel: "symbol"; | ||
} ? typeof kCancel : T extends TextPromptOptions ? string : T extends ConfirmPromptOptions ? boolean : T extends SelectPromptOptions ? T["options"][number] extends { | ||
label: string; | ||
value: string; | ||
hint?: string; | ||
} ? T["options"][number]["value"] : T["options"][number] : T extends MultiSelectOptions ? T["options"] : unknown)>; | ||
/** | ||
* Creates a new instance of Consola, inheriting options from the current instance, with possible overrides. | ||
* | ||
* @param {Partial<ConsolaOptions>} options - Optional overrides for the new instance. See {@link ConsolaOptions}. | ||
* @returns {ConsolaInstance} A new Consola instance. See {@link ConsolaInstance}. | ||
*/ | ||
create(options: Partial<ConsolaOptions>): ConsolaInstance; | ||
/** | ||
* Creates a new Consola instance with the specified default log object properties. | ||
* | ||
* @param {InputLogObject} defaults - Default properties to include in any log from the new instance. See {@link InputLogObject}. | ||
* @returns {ConsolaInstance} A new Consola instance. See {@link ConsolaInstance}. | ||
*/ | ||
withDefaults(defaults: InputLogObject): ConsolaInstance; | ||
/** | ||
* Creates a new Consola instance with a specified tag, which will be included in every log. | ||
* | ||
* @param {string} tag - The tag to include in each log of the new instance. | ||
* @returns {ConsolaInstance} A new Consola instance. See {@link ConsolaInstance}. | ||
*/ | ||
withTag(tag: string): ConsolaInstance; | ||
/** | ||
* Adds a custom reporter to the Consola instance. | ||
* Reporters will be called for each log message, depending on their implementation and log level. | ||
* | ||
* @param {ConsolaReporter} reporter - The reporter to add. See {@link ConsolaReporter}. | ||
* @returns {Consola} The current Consola instance. | ||
*/ | ||
addReporter(reporter: ConsolaReporter): this; | ||
/** | ||
* Removes a custom reporter from the Consola instance. | ||
* If no reporter is specified, all reporters will be removed. | ||
* | ||
* @param {ConsolaReporter} reporter - The reporter to remove. See {@link ConsolaReporter}. | ||
* @returns {Consola} The current Consola instance. | ||
*/ | ||
removeReporter(reporter: ConsolaReporter): ConsolaReporter[] | this; | ||
/** | ||
* Replaces all reporters of the Consola instance with the specified array of reporters. | ||
* | ||
* @param {ConsolaReporter[]} reporters - The new reporters to set. See {@link ConsolaReporter}. | ||
* @returns {Consola} The current Consola instance. | ||
*/ | ||
setReporters(reporters: ConsolaReporter[]): this; | ||
wrapAll(): void; | ||
restoreAll(): void; | ||
/** | ||
* Overrides console methods with Consola logging methods for consistent logging. | ||
*/ | ||
wrapConsole(): void; | ||
/** | ||
* Restores the original console methods, removing Consola overrides. | ||
*/ | ||
restoreConsole(): void; | ||
/** | ||
* Overrides standard output and error streams to redirect them through Consola. | ||
*/ | ||
wrapStd(): void; | ||
_wrapStream(stream: NodeJS.WriteStream | undefined, type: LogType): void; | ||
/** | ||
* Restores the original standard output and error streams, removing the Consola redirection. | ||
*/ | ||
restoreStd(): void; | ||
_restoreStream(stream?: NodeJS.WriteStream): void; | ||
/** | ||
* Pauses logging, queues incoming logs until resumed. | ||
*/ | ||
pauseLogs(): void; | ||
/** | ||
* Resumes logging, processing any queued logs. | ||
*/ | ||
resumeLogs(): void; | ||
/** | ||
* Replaces logging methods with mocks if a mock function is provided. | ||
* | ||
* @param {ConsolaOptions["mockFn"]} mockFn - The function to use for mocking logging methods. See {@link ConsolaOptions["mockFn"]}. | ||
*/ | ||
mockTypes(mockFn?: ConsolaOptions["mockFn"]): void; | ||
@@ -126,4 +455,10 @@ _wrapLogFn(defaults: InputLogObject, isRaw?: boolean): (...args: any[]) => false | undefined; | ||
type ConsolaInstance = Consola & Record<LogType, LogFn>; | ||
/** | ||
* Utility for creating a new Consola instance with optional configuration. | ||
* | ||
* @param {Partial<ConsolaOptions>} [options={}] - Optional configuration options for the new Consola instance. See {@link ConsolaOptions}. | ||
* @returns {ConsolaInstance} A new instance of Consola. See {@link ConsolaInstance}. | ||
*/ | ||
declare function createConsola(options?: Partial<ConsolaOptions>): ConsolaInstance; | ||
export { Consola, ConsolaInstance, ConsolaOptions, ConsolaReporter, FormatOptions, InputLogObject, LogLevel, LogLevels, LogObject, LogType, LogTypes, createConsola }; | ||
export { type ConfirmPromptOptions, Consola, type ConsolaInstance, type ConsolaOptions, type ConsolaReporter, type FormatOptions, type InputLogObject, type LogLevel, LogLevels, type LogObject, type LogType, LogTypes, type MultiSelectOptions, type PromptOptions, type SelectPromptOptions, type TextPromptOptions, createConsola }; |
import { ConsolaOptions, ConsolaInstance } from './core.js'; | ||
export { Consola, ConsolaReporter, FormatOptions, InputLogObject, LogLevel, LogLevels, LogObject, LogType, LogTypes } from './core.js'; | ||
export { ConfirmPromptOptions, Consola, ConsolaReporter, FormatOptions, InputLogObject, LogLevel, LogLevels, LogObject, LogType, LogTypes, MultiSelectOptions, PromptOptions, SelectPromptOptions, TextPromptOptions } from './core.js'; | ||
/** | ||
* Factory function to create a new Consola instance tailored for use in different environments. | ||
* It automatically adjusts logging levels based on environment variables and execution context. | ||
* | ||
* @param {Partial<ConsolaOptions & { fancy: boolean }>} [options={}] - Optional configuration options. See {@link ConsolaOptions}. | ||
* @returns {ConsolaInstance} A new Consola instance with configurations based on the given options and the execution environment. | ||
*/ | ||
declare function createConsola(options?: Partial<ConsolaOptions & { | ||
fancy: boolean; | ||
}>): ConsolaInstance; | ||
/** | ||
* A default instance of Consola, created and configured for immediate use. | ||
* This instance is configured based on the execution environment and the options provided. | ||
* | ||
* @type {ConsolaInstance} consola - The default Consola instance, ready to use. | ||
*/ | ||
declare const consola: ConsolaInstance; | ||
export { ConsolaInstance, ConsolaOptions, consola, createConsola, consola as default }; |
@@ -107,2 +107,8 @@ type BoxBorderStyle = { | ||
}; | ||
/** | ||
* Creates a styled box with text content, customisable via options. | ||
* @param {string} text - The text to display in the box. | ||
* @param {BoxOpts} [_opts={}] - Optional settings for the appearance and behaviour of the box. See {@link BoxOpts}. | ||
* @returns {string} The formatted box as a string, ready for printing or logging. | ||
*/ | ||
declare function box(text: string, _opts?: BoxOpts): string; | ||
@@ -160,12 +166,72 @@ | ||
type ColorFunction = (text: string | number) => string; | ||
declare const colors: Record<"reset" | "bold" | "dim" | "italic" | "underline" | "inverse" | "hidden" | "strikethrough" | "black" | "red" | "green" | "yellow" | "blue" | "magenta" | "cyan" | "white" | "gray" | "bgBlack" | "bgRed" | "bgGreen" | "bgYellow" | "bgBlue" | "bgMagenta" | "bgCyan" | "bgWhite" | "blackBright" | "redBright" | "greenBright" | "yellowBright" | "blueBright" | "magentaBright" | "cyanBright" | "whiteBright" | "bgBlackBright" | "bgRedBright" | "bgGreenBright" | "bgYellowBright" | "bgBlueBright" | "bgMagentaBright" | "bgCyanBright" | "bgWhiteBright", ColorFunction>; | ||
/** | ||
* An object containing functions for colouring text. Each function corresponds to a terminal colour. See {@link ColorName} for available colours. | ||
*/ | ||
declare const colors: Record<ColorName, ColorFunction>; | ||
/** | ||
* Gets a colour function by name, with an option for a fallback colour if the requested colour is not found. | ||
* @param {ColorName} color - The name of the colour function to get. See {@link ColorName}. | ||
* @param {ColorName} [fallback="reset"] - The name of the fallback colour function if the requested colour is not found. See {@link ColorName}. | ||
* @returns {ColorFunction} The colour function that corresponds to the requested colour, or the fallback colour function. See {@link ColorFunction}. | ||
*/ | ||
declare function getColor(color: ColorName, fallback?: ColorName): ColorFunction; | ||
/** | ||
* Applies a specified colour to a given text string or number. | ||
* @param {ColorName} color - The colour to apply. See {@link ColorName}. | ||
* @param {string | number} text - The text to colour. | ||
* @returns {string} The coloured text. | ||
*/ | ||
declare function colorize(color: ColorName, text: string | number): string; | ||
/** | ||
* Removes ANSI escape codes from a given string. This is particularly useful for | ||
* processing text that contains formatting codes, such as colours or styles, so that the | ||
* the raw text without any visual formatting. | ||
* | ||
* @param {string} text - The text string from which to strip the ANSI escape codes. | ||
* @returns {string} The text without ANSI escape codes. | ||
*/ | ||
declare function stripAnsi(text: string): string; | ||
/** | ||
* Centers a string within a specified total width, padding it with spaces or another specified character. | ||
* If the string is longer than the total width, it is returned as is. | ||
* | ||
* @param {string} str - The string to centre. | ||
* @param {number} len - The total width in which to centre the string. | ||
* @param {string} [space=" "] - The character to use for padding. Defaults to a space. | ||
* @returns {string} The centred string. | ||
*/ | ||
declare function centerAlign(str: string, len: number, space?: string): string; | ||
/** | ||
* Right-justifies a string within a given total width, padding it with whitespace or another specified character. | ||
* If the string is longer than the total width, it is returned as is. | ||
* | ||
* @param {string} str - The string to right-justify. | ||
* @param {number} len - The total width to align the string. | ||
* @param {string} [space=" "] - The character to use for padding. Defaults to a space. | ||
* @returns {string} The right-justified string. | ||
*/ | ||
declare function rightAlign(str: string, len: number, space?: string): string; | ||
/** | ||
* Left-aligns a string within a given total width, padding it with whitespace or another specified character on the right. | ||
* If the string is longer than the total width, it is returned as is. | ||
* | ||
* @param {string} str - The string to align left. | ||
* @param {number} len - The total width to align the string. | ||
* @param {string} [space=" "] - The character to use for padding. Defaults to a space. | ||
* @returns {string} The left-justified string. | ||
*/ | ||
declare function leftAlign(str: string, len: number, space?: string): string; | ||
/** | ||
* Aligns a string (left, right, or centre) within a given total width, padding it with spaces or another specified character. | ||
* If the string is longer than the total width, it is returned as is. This function acts as a wrapper for individual alignment functions. | ||
* | ||
* @param {"left" | "right" | "centre"} alignment - The desired alignment of the string. | ||
* @param {string} str - The string to align. | ||
* @param {number} len - The total width in which to align the string. | ||
* @param {string} [space=" "] - The character to use for padding. Defaults to a space. | ||
* @returns {string} The aligned string, according to the given alignment. | ||
*/ | ||
declare function align(alignment: "left" | "right" | "center", str: string, len: number, space?: string): string; | ||
export { BoxBorderStyle, BoxOpts, BoxStyle, ColorFunction, ColorName, align, box, centerAlign, colorize, colors, getColor, leftAlign, rightAlign, stripAnsi }; | ||
export { type BoxBorderStyle, type BoxOpts, type BoxStyle, type ColorFunction, type ColorName, align, box, centerAlign, colorize, colors, getColor, leftAlign, rightAlign, stripAnsi }; |
{ | ||
"name": "consola", | ||
"version": "3.2.3", | ||
"version": "3.3.0", | ||
"description": "Elegant Console Wrapper", | ||
@@ -31,3 +31,4 @@ "keywords": [ | ||
"types": "./dist/browser.d.ts", | ||
"import": "./dist/browser.mjs" | ||
"import": "./dist/browser.mjs", | ||
"require": "./dist/browser.cjs" | ||
} | ||
@@ -37,3 +38,4 @@ }, | ||
"types": "./dist/browser.d.ts", | ||
"import": "./dist/browser.mjs" | ||
"import": "./dist/browser.mjs", | ||
"require": "./dist/browser.cjs" | ||
}, | ||
@@ -48,3 +50,4 @@ "./basic": { | ||
"types": "./dist/browser.d.ts", | ||
"import": "./dist/browser.mjs" | ||
"import": "./dist/browser.mjs", | ||
"require": "./dist/browser.cjs" | ||
} | ||
@@ -81,20 +84,20 @@ }, | ||
"devDependencies": { | ||
"@clack/core": "^0.3.2", | ||
"@types/node": "^20.3.3", | ||
"@vitest/coverage-v8": "^0.32.2", | ||
"changelogen": "^0.5.3", | ||
"defu": "^6.1.2", | ||
"eslint": "^8.44.0", | ||
"eslint-config-unjs": "^0.2.1", | ||
"is-unicode-supported": "^1.3.0", | ||
"jiti": "^1.18.2", | ||
"@clack/core": "^0.4.0", | ||
"@types/node": "^22.10.2", | ||
"@vitest/coverage-v8": "^2.1.8", | ||
"changelogen": "^0.5.7", | ||
"defu": "^6.1.4", | ||
"eslint": "^9.17.0", | ||
"eslint-config-unjs": "^0.4.2", | ||
"is-unicode-supported": "^2.1.0", | ||
"jiti": "^2.4.2", | ||
"lodash": "^4.17.21", | ||
"prettier": "^3.0.0", | ||
"prettier": "^3.4.2", | ||
"sentencer": "^0.2.1", | ||
"sisteransi": "^1.0.5", | ||
"std-env": "^3.3.3", | ||
"string-width": "^6.1.0", | ||
"typescript": "^5.1.6", | ||
"unbuild": "^1.2.1", | ||
"vitest": "^0.32.2" | ||
"std-env": "^3.8.0", | ||
"string-width": "^7.2.0", | ||
"typescript": "^5.7.2", | ||
"unbuild": "^3.0.1", | ||
"vitest": "^2.1.8" | ||
}, | ||
@@ -104,3 +107,3 @@ "engines": { | ||
}, | ||
"packageManager": "pnpm@8.6.5" | ||
} | ||
"packageManager": "pnpm@9.12.3" | ||
} |
@@ -67,3 +67,3 @@ # 🐨 Consola | ||
<img width="760" alt="image" src="https://user-images.githubusercontent.com/5158436/231029244-abc79f48-ca16-4eaa-b592-7abd271ecb1f.png"> | ||
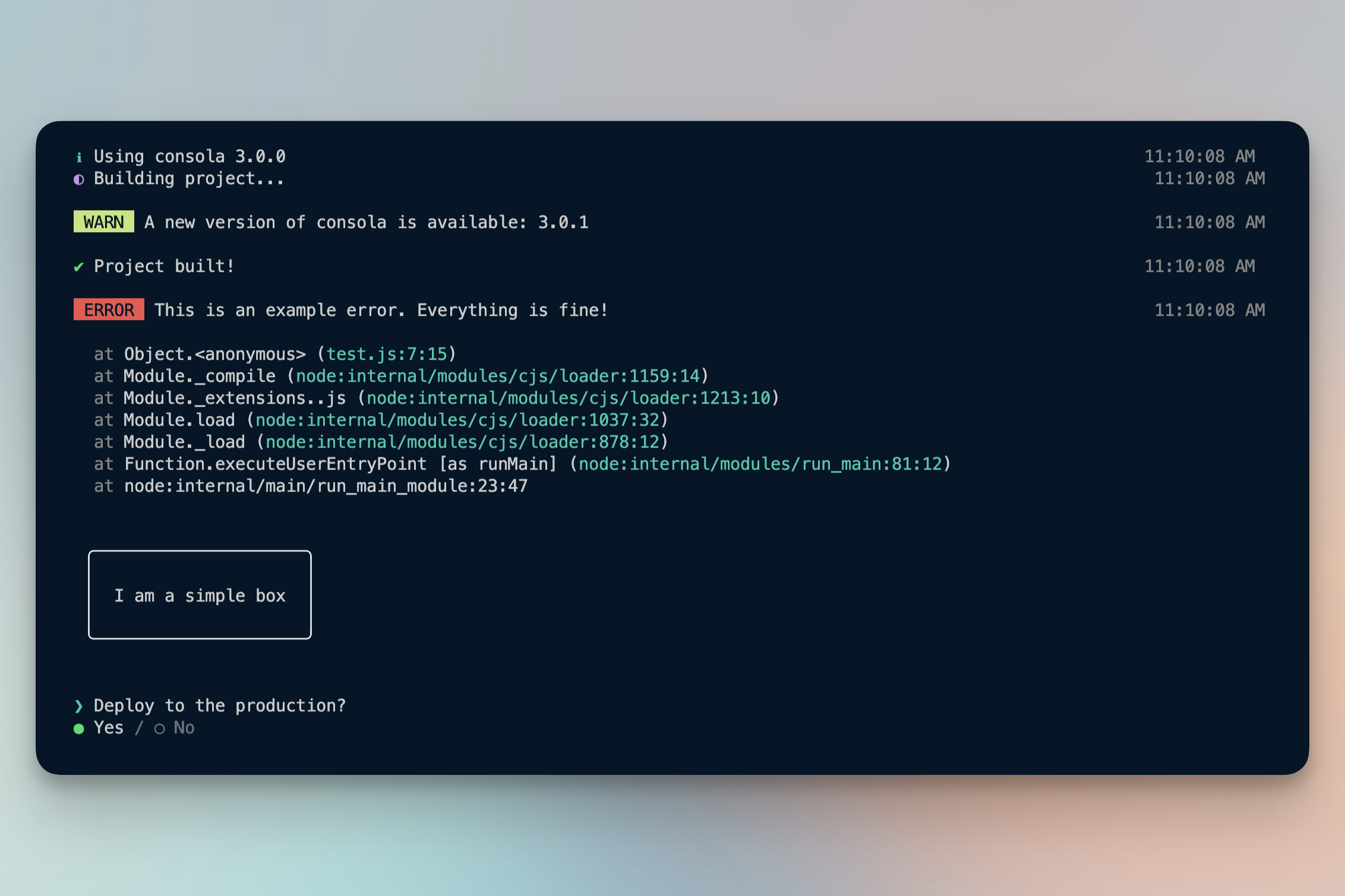 | ||
@@ -86,6 +86,14 @@ You can use smaller core builds without fancy reporter to save 80% of the bundle size: | ||
#### `await prompt(message, { type })` | ||
#### `await prompt(message, { type, cancel })` | ||
Show an input prompt. Type can either of `text`, `confirm`, `select` or `multiselect`. | ||
If prompt is canceled by user (with Ctrol+C), default value will be resolved by default. This strategy can be configured by setting `{ cancel: "..." }` option: | ||
- `"default"` - Resolve the promise with the `default` value or `initial` value. | ||
- `"undefined`" - Resolve the promise with `undefined`. | ||
- `"null"` - Resolve the promise with `null`. | ||
- `"symbol"` - Resolve the promise with a symbol `Symbol.for("cancel")`. | ||
- `"reject"` - Reject the promise with an error. | ||
See [examples/prompt.ts](./examples/prompt.ts) for usage examples. | ||
@@ -157,3 +165,6 @@ | ||
```js | ||
// Jest | ||
consola.mockTypes((typeName, type) => jest.fn()); | ||
// Vitest | ||
consola.mockTypes((typeName, type) => vi.fn()); | ||
``` | ||
@@ -164,3 +175,6 @@ | ||
```js | ||
// Jest | ||
const fn = jest.fn(); | ||
// Vitest | ||
const fn = vi.fn(); | ||
consola.mockTypes(() => fn); | ||
@@ -174,3 +188,6 @@ ``` | ||
```js | ||
// Jest | ||
consola.mockTypes((typeName) => typeName === "fatal" && jest.fn()); | ||
// Vitest | ||
consola.mockTypes((typeName) => typeName === "fatal" && vi.fn()); | ||
``` | ||
@@ -268,3 +285,6 @@ | ||
// calls from before | ||
// Jest | ||
consola.mockTypes(() => jest.fn()); | ||
// Vitest | ||
consola.mockTypes(() => vi.fn()); | ||
}); | ||
@@ -287,3 +307,3 @@ | ||
{ | ||
virtualConsole: new jsdom.VirtualConsole().sendTo(consola); | ||
new jsdom.VirtualConsole().sendTo(consola); | ||
} | ||
@@ -312,2 +332,16 @@ ``` | ||
## Raw logging methods | ||
Objects sent to the reporter could lead to unexpected output when object is close to internal object structure containing either `message` or `args` props. To enforce the object to be interpreted as pure object, you can use the `raw` method chained to any log type. | ||
**Example:** | ||
```js | ||
// Prints "hello" | ||
consola.log({ message: "hello" }); | ||
// Prints "{ message: 'hello' }" | ||
consola.log.raw({ message: "hello" }); | ||
``` | ||
## License | ||
@@ -314,0 +348,0 @@ |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Environment variable access
Supply chain riskPackage accesses environment variables, which may be a sign of credential stuffing or data theft.
Found 1 instance in 1 package
319982
41
5267
353
13
1