Comparing version 1.1.4 to 2.0.0-next-0370fc
{ | ||
"version": "1.1.4", | ||
"version": "2.0.0-next-0370fc", | ||
"license": "MIT", | ||
"main": "dist/index.js", | ||
"typings": "dist/index.d.ts", | ||
"files": [ | ||
"dist", | ||
"src" | ||
], | ||
"engines": { | ||
"node": ">=10" | ||
}, | ||
"main": "./dist/cjs/context.js", | ||
"types": "./types/context.d.ts", | ||
"name": "context", | ||
"author": "ealush", | ||
"scripts": { | ||
"start": "tsdx watch", | ||
"build": "tsdx build", | ||
"test": "tsdx test", | ||
"lint": "tsdx lint", | ||
"prepare": "tsdx build" | ||
"test": "vx test", | ||
"build": "vx build", | ||
"release": "vx release" | ||
}, | ||
"peerDependencies": {}, | ||
"husky": { | ||
"hooks": { | ||
"pre-commit": "tsdx lint" | ||
} | ||
"module": "./dist/es/context.production.js", | ||
"exports": { | ||
".": { | ||
"development": { | ||
"types": "./types/context.d.ts", | ||
"browser": "./dist/es/context.development.js", | ||
"umd": "./dist/umd/context.development.js", | ||
"import": "./dist/es/context.development.js", | ||
"require": "./dist/cjs/context.development.js", | ||
"node": "./dist/cjs/context.development.js", | ||
"module": "./dist/es/context.development.js", | ||
"default": "./dist/cjs/context.development.js" | ||
}, | ||
"types": "./types/context.d.ts", | ||
"browser": "./dist/es/context.production.js", | ||
"umd": "./dist/umd/context.production.js", | ||
"import": "./dist/es/context.production.js", | ||
"require": "./dist/cjs/context.js", | ||
"node": "./dist/cjs/context.js", | ||
"module": "./dist/es/context.production.js", | ||
"default": "./dist/cjs/context.js" | ||
}, | ||
"./package.json": "./package.json", | ||
"./": "./" | ||
}, | ||
"prettier": { | ||
"printWidth": 80, | ||
"semi": true, | ||
"singleQuote": true, | ||
"trailingComma": "es5" | ||
}, | ||
"name": "context", | ||
"author": "ealush", | ||
"module": "dist/context.esm.js", | ||
"devDependencies": { | ||
"husky": "^4.2.5", | ||
"tsdx": "^0.13.2", | ||
"tslib": "^2.0.1", | ||
"typescript": "^4.0.2" | ||
}, | ||
"repository": { | ||
"type": "git", | ||
"url": "git+https://github.com/ealush/context.git" | ||
"url": "https://github.com/ealush/vest.git", | ||
"directory": "packages/context" | ||
}, | ||
"bugs": { | ||
"url": "https://github.com/ealush/vest.git/issues" | ||
} | ||
} |
451
README.md
# Context | ||
Simple utility that creates a multi-layered context singleton. | ||
It allows you to keep reference for shared variables, and access them later down in your function call even if not declared in the same scope. | ||
Simple utility for context propagation within Javascript applications and libraries. Loosely based on the ideas behind React's context, allows you to achieve the same goals (and more) without actually using react. | ||
It allows you to keep reference for shared variables, and access them down in your function call even if not declared in the same scope. | ||
Originally built for [vest](https://github.com/ealush/vest) validation framework. | ||
## How Context Works? | ||
The way context works is quite simple. Creating a context initializes a closure with a context storage object. When you run your context call, it takes your values, and places them in the context storage object. When your function finishes running, the context is cleared. | ||
# The Concepts | ||
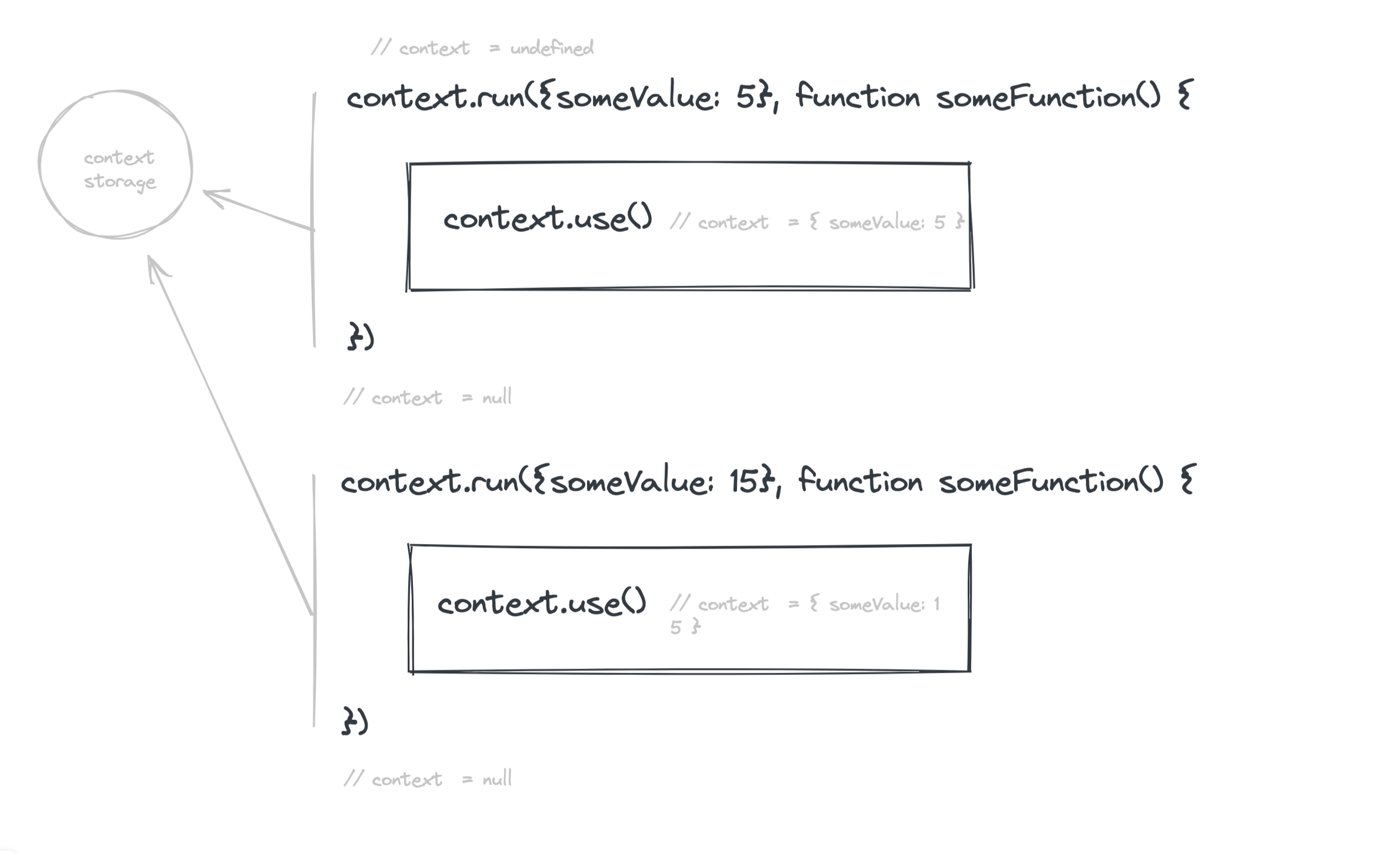 | ||
Lets take a quick look at how this would work | ||
The reason the context is cleared after its run is so that items can't override one another. Assume you have two running the same async function twice, and it writes to the context, expecting to read the value somewhere down the line. Now you have two competing consumers of this single resource, and one eventually get the wrong value since they both read from and write to the same place. | ||
```js | ||
import createContext from 'context'; | ||
## API Reference | ||
const context = createContext(); | ||
Context tries to mimic the behavior of Javascript scopes without actually being in the same scopes, and the API is built around context initialization, setting of values within the context and retrieving values. | ||
context.run({ name: 'Sam' }, sayMyName); | ||
context.run({ name: 'David' }, sayMyName); | ||
### createContext() | ||
function sayMyName() { | ||
const { name } = context.use(); | ||
console.log(`My Name is ${name}`); | ||
} | ||
``` | ||
Context's default export, it creates a context singleton object that can later be referenced. | ||
This will print: | ||
My Name is Sam | ||
My Name is David | ||
So as we see 'run' allows us to run a function and access the specific variable we assigned to the object. | ||
Why is this useful? Why not just do | ||
```js | ||
sayMyName('Sam'); | ||
sayMyName('David'); | ||
// ctx.js | ||
import { createContext } from 'context'; | ||
function sayMyName(name) { | ||
console.log(`My Name is ${name}`); | ||
} | ||
export default createContext(); // { run: ƒ, bind: ƒ, use: ƒ, useX: ƒ } | ||
``` | ||
We will be getting the same results. Right? | ||
createContext also accepts an initialization function that's run every time ctx.run is called. It allows intercepting the context initialization and adding custom logic, or default values. | ||
The init function is passed the context object run was called with, and the parent context if it was called within a previously created context. The init function needs to return the desired context object. | ||
It's the next feature that illustrates context's power. | ||
Instead of using 'run'. we will use 'bind' | ||
```js | ||
// getNames.js | ||
createContext((ctx, parentContext) => { | ||
if (parentContext === null) { | ||
// we're at the top level | ||
// so let's add a default cart object | ||
return Object.assign(ctx, { | ||
cart: [], | ||
}); | ||
} | ||
import createContext from 'context'; | ||
const context = createContext(); | ||
const samName = context.bind({ name: 'Sam' }, sayMyName); | ||
const davidName = context.bind({ name: 'David' }, sayMyName); | ||
function sayMyName() { | ||
const { name } = context.use(); | ||
console.log(`My Name is ${name}`); | ||
} | ||
export { samName, davidName }; | ||
//index.js | ||
samName(); | ||
davidName(); | ||
``` | ||
This will once again print | ||
My Name is Sam | ||
My Name is David | ||
Except now, because context is a singleton anywhere we run these functions they will print those values. | ||
What if we want to share a value between these functions? | ||
We can initialize createContext with a value | ||
```js | ||
// getNames.js | ||
import createContext from 'context'; | ||
const context = createContext((ctx, parentCtx) => { | ||
return Object.assign({}, ctx, { lastName: 'Smith' }); | ||
// we're in a sub context, so we already have those default values. | ||
return ctx; | ||
}); | ||
const samName = context.bind({ name: 'Sam' }, sayMyName); | ||
const davidName = context.bind({ name: 'David' }, sayMyName); | ||
function sayMyName() { | ||
const { name, lastName } = context.use(); | ||
console.log(`My Name is ${name} ${lastName}`); | ||
} | ||
export { samName, davidName }; | ||
//index.js | ||
samName(); | ||
davidName(); | ||
``` | ||
will print | ||
### run() | ||
My Name is Sam Smith | ||
My Name is David Smith | ||
Runs a callback function within the context. It takes an object referencing the data you want to store within your context, and a callback function to run. | ||
These example are pretty contrived | ||
Lets try something a little more real world. | ||
# Real World Example | ||
What if we wanted to keep track of how many times a function was called. | ||
We want to create an environment where we pass the function we want to track and receive exactly the same function. | ||
The only difference is that the function is going to have a count property which we will be able to call at any time. | ||
So lets start | ||
```js | ||
//counter.js | ||
import createContext from 'context'; | ||
// myFunction.js | ||
import ctx from './ctx'; | ||
import sayUsername from './sayUsername'; | ||
const context = createContext(); | ||
``` | ||
We've seen this before, we are creating the context object. | ||
Now I want to have a main context that will store the values of all the functions that are running. | ||
in context world it will look like this. | ||
Just a parent function called counter. | ||
```js | ||
//counter.js | ||
import createContext from 'context'; | ||
const context = createContext(); | ||
function counter() {} | ||
``` | ||
In this function I am going to want to store the function name and the count. | ||
I add a couple of functions add and getVals. | ||
```js | ||
//counter.js | ||
import createContext from 'context'; | ||
const context = createContext(); | ||
function counter() { | ||
const values = {}; | ||
function add(val) { | ||
if (!values[val]) { | ||
values[val] = 0; | ||
} | ||
values[val] += 1; | ||
} | ||
function getVals() { | ||
return values; | ||
} | ||
function myFunction(username) { | ||
return ctx.run({ username }, sayUsername); | ||
} | ||
``` | ||
At this stage it won't accomplish much. | ||
What I want counter to return is a function that holds 'values' in its context. We will use context.bind for that. | ||
So imagine a box and counter is the outer most box. In it is the values object and anything I put into the box will have access to that object. | ||
and what do I want to put in the box? The function I want to count. | ||
If `run` is called within a different `run` call, the context values will be merged when the callback is run. When we exit our callback, the context will be reset to the values it had before the call. | ||
I add an argument func to counter. | ||
This will be the function we want to count. | ||
I create a ref object which I will then pass to the function. | ||
This object is accessible to the the function thanks to context. | ||
### use() and useX() | ||
```js | ||
//counter.js | ||
import createContext from 'context'; | ||
These are the main ways to access the context within your code. They return the current object that stored within the context, and differ in the way they handle calls outside of the context. | ||
const context = createContext(); | ||
- use() returns the current object stored within the context, or null if we're not within a `run` call. | ||
- useX() returns the current object stored within the context, or throws an error if we're not within a `run` call. | ||
- useX also takes an optional argument that will be used as the error message if the context is not found. | ||
function counter(func) { | ||
const values = {}; | ||
function add(val) { | ||
if (!values[val]) { | ||
values[val] = 0; | ||
} | ||
values[val] += 1; | ||
} | ||
function getVals() { | ||
return values; | ||
} | ||
const ref = { add, getVals }; | ||
return context.bind({ ref }, func); | ||
} | ||
``` | ||
great! wait no, this function that is returned will run our function we passed and it does have access to values but in order to use values we have to modify our function! | ||
Not what we wanted... | ||
We want a function that will run our function, have access to values but won't require us to modify our existing function. | ||
so lets create a function called track. | ||
'track' will return a function that will modify values and run out function | ||
```js | ||
//counter.js | ||
function track(func) { | ||
const { ref } = context.use(); | ||
// sayUsername.js | ||
import ctx from './ctx'; | ||
function holder(args) { | ||
ref.add(func.name); | ||
holder.count = ref.getVals()[func.name]; | ||
holder.getAll = () => { | ||
return ref.getVals(); | ||
}; | ||
return func(args); | ||
} | ||
function sayUsername() { | ||
const context = ctx.use(); // { username: 'John Doe' } | ||
return holder; | ||
} | ||
``` | ||
In 'track' I am using context.use and I can do this because I am going to pass it to context.bind. If I didn't run it in either context.run or context.bind this will not work. It basically is looking for the outer box. | ||
I pull out ref which holds the reference to values. | ||
The function 'holder' is going to run 'add' from ref and since functions in javascript are object we are going to pass the resulting value into a 'count' property. | ||
We are also creating a getAll function to the function. | ||
Then our function will run and the result will return as if no change was ever made. | ||
```js | ||
//counter.js | ||
import createContext from 'context'; | ||
const context = createContext(); | ||
function track(func) { | ||
const { ref } = context.use(); | ||
function holder(args) { | ||
ref.add(func.name); | ||
holder.count = ref.getVals()[func.name]; | ||
holder.getAll = () => { | ||
return ref.getVals(); | ||
}; | ||
return func(args); | ||
if (!context) { | ||
// we're not within a `run` call. This function was called outside of a running context. | ||
return "Hey, I don't know you, and this is crazy!"; | ||
} | ||
return holder; | ||
return `Hello, ${context.username}!`; | ||
} | ||
function counter(tracker) { | ||
const values = {}; | ||
function add(val) { | ||
if (!values[val]) { | ||
values[val] = 0; | ||
} | ||
values[val] += 1; | ||
} | ||
function getVals() { | ||
return values; | ||
} | ||
const ref = { add, getVals }; | ||
return context.bind({ ref }, tracker); // | ||
} | ||
export { counter, track }; | ||
``` | ||
Lets see how we use this. | ||
```js | ||
// index.js | ||
import { counter, track } from './counter'; | ||
// handleCart.js | ||
import ctx from './ctx'; | ||
const countIt = counter(func => { | ||
return track(func); | ||
}); | ||
function handleCart() { | ||
const context = ctx.useX( | ||
'handleCart was called outside of a running context' | ||
); // { cart: { items: [ 'foo', 'bar' ] } } | ||
// This throws an error if we're not within a `run` call. | ||
// You should catch this error and handle it somewhere above this function. | ||
function goToServer() { | ||
console.log('going to server'); | ||
return `You have ${context.cart.items.length} items in your cart.`; | ||
} | ||
function readFile(file) { | ||
console.log('readingFile ' + file); | ||
} | ||
const dReadFile = countIt(readFile); | ||
const dgoToServer = countIt(goToServer); | ||
readFile('important.docx'); | ||
dReadFile('mainfile.js'); | ||
dReadFile('secondary.js'); | ||
console.log(dReadFile.count); | ||
dgoToServer(); | ||
console.log(dgoToServer.count); | ||
console.log(dReadFile.getAll()); | ||
``` | ||
Imagine a situation where you have functions that are trying to reach a server and read a file. | ||
### bind() | ||
We don't want to modify them but create a wrapper function to keep track | ||
of how many times it runs. | ||
Bind a function to a context. It takes an object referencing the data you want to store within your context, and a callback function to run. It returns a function that can be called with the same arguments as the original function. The function will then internally call `run` with the same arguments, and return the result of the callback function, so it is useful if you want to reference a context after it was closed, for example - when running an async function. | ||
So to create our first box we have the counter function. | ||
It stores the values and receives a deferred anonymous function. | ||
We are passing our function the anonymous function which then gets passed to the tracker function. | ||
The tracker function receives our function runs the count returns the result of our function | ||
We assign this wrapper to the variable countIt. | ||
We pass readFile to countIt and create a new function dReadFile | ||
When we run dReadFile it will behave like readFile but will store the count within. | ||
Since context is a singleton anywhere we run this function within our project it will keep correct track of our count. | ||
# Other Examples | ||
```js | ||
// myContext.js | ||
import createContext from 'context'; | ||
// getProductData.js | ||
import ctx from './ctx'; | ||
export default createContext(); | ||
``` | ||
function getProductData(productId) { | ||
return ctx.bind({ productId }, handleProductData); | ||
```js | ||
// framework.js | ||
import context from './myContext.js'; | ||
function suite(id, tests) { | ||
context.run({ suiteId: id }, () => tests()); // ... | ||
fetchProduct(productId).then(data => { | ||
handleProductData(data); | ||
// will run the function handleProductData within our context, even though there is no context running at the moment. | ||
}); | ||
} | ||
function group(name, groupTests) { | ||
const { suiteId } = context.use(); | ||
context.run( | ||
{ | ||
group: name, | ||
}, | ||
() => groupTests() | ||
); | ||
} | ||
function test(message, cb) { | ||
const { suiteId, group } = context.use(); | ||
const testId = Math.random(); // 0.8418151199238901 | ||
const testData = context.run({ test: testId }, () => cb()); // ... | ||
} | ||
export { suite, group, test } from './framework'; | ||
``` | ||
```js | ||
import testFramework from './framwork.js'; | ||
## Troubleshooting | ||
suite('some_id', () => { | ||
/* | ||
context now is: | ||
{ | ||
suiteId: 'some_id' | ||
} | ||
*/ | ||
### Working with async functions/promises | ||
group('some_group_name', () => { | ||
/* | ||
context now is: | ||
{ | ||
group: 'some_group_name', | ||
parentContext: { | ||
suiteId: 'some_id', | ||
} | ||
} | ||
*/ | ||
Working with context inside within async code may lead to unexpected results when we don't fully consider what's happening. Trying to call your context from the async part of your code will probably return `null` instead of your values. | ||
test('blah blah', () => { | ||
/* | ||
context now is: | ||
{ | ||
test: 0.8418151199238901, | ||
parentContext: { | ||
group: 'some_group_name', | ||
parentContext: { | ||
suiteId: 'some_id', | ||
} | ||
} | ||
} | ||
*/ | ||
}); | ||
}); | ||
}); | ||
``` | ||
This is known and expected behavior. Context is a synchronous context propagation tool that completely relies on the synchronous nature of function calls in JS - this is exactly what allows context to run. | ||
## Binding a function with context | ||
#### But my function is still running. Why did the context clear? | ||
The async parts of your function are actually not executed along with your sync code, and even though you "await" it, the browser carries on and allows other code to run in between instead of blocking execution until your async code is complete. | ||
You can bind a function to a context with ctx.bind, this allows you to create a bound function that's when called - will be called with that bound context, even if not in the same scope anymore. | ||
#### Ok, so what do I do? | ||
There are multiple strategies of handling async functions with context. | ||
```js | ||
const boundFunction = ctx.bind(ctxRef, fn, ...args); | ||
1. Pulling your values from context right before your async call | ||
This is the most obvious and easiest to achieve, though not always what you need. The basic idea is that you take whatever you need from the context when it is still available to you. | ||
boundFunction(); // Will run with the context as if you run it directly within ctx.run(); | ||
``` | ||
## Context initialization | ||
You can add an init function to your context creation. The init function will run every time you call context.run, to allow you to set in-flight keys to your context. It accepts two params - the provided ctxRef, and the parent context when nested. | ||
2. context.bind or context.run your async function to the context you extracted | ||
This is the next logical step - you have a function that you know should run later with your context. You can bind your context to it for delayed execution. When your function runs later down the line within your asynchronous code, internally it will still have access to whatever you bound to it. |
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
No v1
QualityPackage is not semver >=1. This means it is not stable and does not support ^ ranges.
Found 1 instance in 1 package
No bug tracker
MaintenancePackage does not have a linked bug tracker in package.json.
Found 1 instance in 1 package
0
15
254
21984
2
138
1