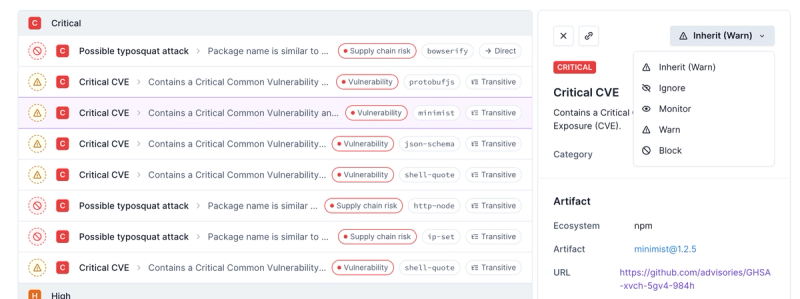
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
conventional-commits-filter
Advanced tools
Package description
The conventional-commits-filter npm package is used to filter out commit messages that do not follow the Conventional Commits specification. This is useful in projects that enforce a specific commit message format for better readability, automation, and tooling.
Filtering valid commits
This feature allows you to filter out commits that do not follow the Conventional Commits specification. In the example, only the commits with headers 'feat: add new feature' and 'fix: fix bug' will be considered valid.
const filter = require('conventional-commits-filter');
const commits = [
{ header: 'feat: add new feature' },
{ header: 'fix: fix bug' },
{ header: 'invalid commit message' }
];
const validCommits = filter(commits);
console.log(validCommits);
Filtering invalid commits
This feature allows you to filter out valid commits and only keep the invalid ones. In the example, only the commit with the header 'invalid commit message' will be considered invalid.
const filter = require('conventional-commits-filter');
const commits = [
{ header: 'feat: add new feature' },
{ header: 'fix: fix bug' },
{ header: 'invalid commit message' }
];
const invalidCommits = filter(commits, { ignore: true });
console.log(invalidCommits);
commitlint checks if your commit messages meet the Conventional Commits format. It is more comprehensive than conventional-commits-filter as it provides linting capabilities and can be integrated into CI/CD pipelines.
conventional-changelog is used to generate changelogs based on commit messages that follow the Conventional Commits specification. While it does not filter commits, it complements conventional-commits-filter by providing a way to generate meaningful changelogs.
validate-commit-msg is a tool to validate commit messages against a set of rules, including the Conventional Commits specification. It is similar to conventional-commits-filter but focuses more on validation rather than filtering.
Readme
Filter out reverted commits parsed by conventional-commits-parser.
# pnpm
pnpm add conventional-commits-filter
# yarn
yarn add conventional-commits-filter
# npm
npm i conventional-commits-filter
import {
filterRevertedCommitsSync,
filterRevertedCommits,
filterRevertedCommitsStream
} from 'conventional-commits-filter'
import { pipeline } from 'stream/promises'
import { Readable } from 'stream'
const commits = [{
type: 'revert',
scope: null,
subject: 'feat(): amazing new module',
header: 'revert: feat(): amazing new module\n',
body: 'This reverts commit 56185b7356766d2b30cfa2406b257080272e0b7a.\n',
footer: null,
notes: [],
references: [],
revert: {
header: 'feat(): amazing new module',
hash: '56185b7356766d2b30cfa2406b257080272e0b7a',
body: null
},
hash: '789d898b5f8422d7f65cc25135af2c1a95a125ac\n',
raw: {
type: 'revert',
scope: null,
subject: 'feat(): amazing new module',
header: 'revert: feat(): amazing new module\n',
body: 'This reverts commit 56185b7356766d2b30cfa2406b257080272e0b7a.\n',
footer: null,
notes: [],
references: [],
revert: {
header: 'feat(): amazing new module',
hash: '56185b7356766d2b30cfa2406b257080272e0b7a',
body: null
},
hash: '789d898b5f8422d7f65cc25135af2c1a95a125ac\n'
}
}, {
type: 'Features',
scope: null,
subject: 'wow',
header: 'amazing new module\n',
body: null,
footer: 'BREAKING CHANGE: Not backward compatible.\n',
notes: [],
references: [],
revert: null,
hash: '56185b',
raw: {
type: 'feat',
scope: null,
subject: 'amazing new module',
header: 'feat(): amazing new module\n',
body: null,
footer: 'BREAKING CHANGE: Not backward compatible.\n',
notes: [],
references: [],
revert: null,
hash: '56185b7356766d2b30cfa2406b257080272e0b7a\n'
}
}, {
type: 'What',
scope: null,
subject: 'new feature',
header: 'feat(): new feature\n',
body: null,
footer: null,
notes: [],
references: [],
revert: null,
hash: '815a3f0',
raw: {
type: 'feat',
scope: null,
subject: 'new feature',
header: 'feat(): new feature\n',
body: null,
footer: null,
notes: [],
references: [],
revert: null,
hash: '815a3f0717bf1dfce007bd076420c609504edcf3\n'
}
}, {
type: 'Chores',
scope: null,
subject: 'first commit',
header: 'chore: first commit\n',
body: null,
footer: null,
notes: [],
references: [],
revert: null,
hash: '74a3e4d6d25',
raw: {
type: 'chore',
scope: null,
subject: 'first commit',
header: 'chore: first commit\n',
body: null,
footer: null,
notes: [],
references: [],
revert: null,
hash: '74a3e4d6d25dee2c0d6483a0a3887417728cbe0a\n'
}
}];
// to filter reverted commits syncronously:
for (const commit of filterRevertedCommitsSync(commits)) {
console.log(commit)
}
// to filter reverted commits in async iterables:
await pipeline(
commits,
filterRevertedCommits,
async function* (filteredCommits) {
for await (const commit of filteredCommits) {
console.log(commit)
}
}
)
// to filter reverted commits in streams:
Readable.from(commits)
.pipe(filterRevertedCommitsStream())
.on('data', commit => console.log(commit))
Output:
{
type: 'What',
scope: null,
subject: 'new feature',
header: 'feat(): new feature\n',
body: null,
footer: null,
notes: [],
references: [],
revert: null,
hash: '815a3f0',
raw: {
type: 'feat',
scope: null,
subject: 'new feature',
header: 'feat(): new feature\n',
body: null,
footer: null,
notes: [],
references: [],
revert: null,
hash: '815a3f0717bf1dfce007bd076420c609504edcf3\n'
}
}
{
type: 'Chores',
scope: null,
subject: 'first commit',
header: 'chore: first commit\n',
body: null,
footer: null,
notes: [],
references: [],
revert: null,
hash: '74a3e4d6d25',
raw: {
type: 'chore',
scope: null,
subject: 'first commit',
header: 'chore: first commit\n',
body: null,
footer: null,
notes: [],
references: [],
revert: null,
hash: '74a3e4d6d25dee2c0d6483a0a3887417728cbe0a\n'
}
}
MIT © Steve Mao
FAQs
Filter out reverted commits parsed by conventional-commits-parser.
The npm package conventional-commits-filter receives a total of 2,993,767 weekly downloads. As such, conventional-commits-filter popularity was classified as popular.
We found that conventional-commits-filter demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.