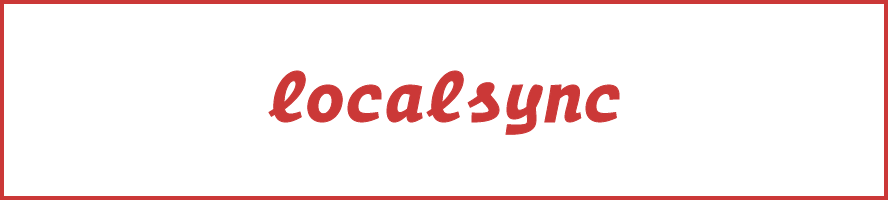
serversync
a lightweight module to sync JS objects in realtime across tabs / windows of a browser COOKIE FALLBACK VERSION.
Features
- Uses local storage event emitters to sync objects in realtime across tabs.
- Never calls the tab that the event occurred on.
- Falls back to cookie polling internally if using an unsupported browser (IE 9+ / Edge).
- Isomorphic.
- Tested with mocha.


Install
npm i -S cookiesync
How to use
import cookiesync from 'cookiesync'
const action = (userID, first, last) => ({ userID, first, last })
const handler = (value, old, url) => {
console.info(`Another tab at url ${url} switched user from "${old.first} ${old.last}" to "${value.first} ${value.last}".`)
}
const usersync = cookiesync('user', action, handler)
usersync.start()
if(usersync.isFallback)
console.warn('browser doesnt support local storage synchronization, falling back to cookie synchronization.')
usersync.trigger(1, 'jimmy', 'john')
setTimeout(() => {
usersync.trigger(2, 'jane', 'wonka')
}, 5000)
setTimeout(() => {
if(usersync.isRunning)
usersync.stop()
}, 10000)
Documentation
cookiesync(key: string, action: (...args) => payload, handler: payload => {}, [opts: Object]): { start, stop, trigger, isRunning, isFallback }
opts
name | type | default | description |
---|
tracing | boolean | false | toggles tracing for debugging purposes |
logger | Object | console | the logger object to trace to |
loglevel | string | 'info' | the log level to use when tracing (error , warn , info , trace ) |
IE / Edge fallback props for cookiesync
name | type | default | description |
---|
pollFrequency | number | 3000 | the number in milliseconds that should be used for cookie polling |
idLength | number | 8 | the number of characters to use for tracking the current instance (tab) |
path | string | '/' | The path to use for cookies |
secure | boolean | false | Whether to set the secure flag on cookies or not (not recommended) |
httpOnly | boolean | false | Whether to set the http only flag on cookies or not |