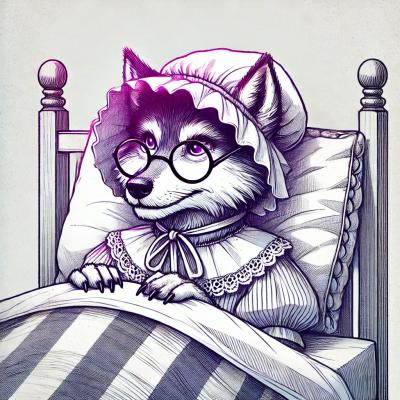
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
cosmiconfig
Advanced tools
Find and load configuration from a package.json property, rc file, or CommonJS module
The cosmiconfig npm package is a utility for finding and loading configuration from a variety of file formats and locations. It is commonly used in Node.js projects to create flexible configuration systems for tools and libraries.
Searching for configuration files
This feature allows you to search for configuration files with a given name in various locations, including package.json properties, rc files, and regular JSON or YAML files.
const cosmiconfig = require('cosmiconfig');
const explorer = cosmiconfig('yourModuleName');
explorer.search()
.then((result) => {
const config = result ? result.config : {};
// do something with config
})
.catch((error) => {
// handle error
});
Loading configuration files directly
This feature allows you to load a configuration file directly from a specified path, regardless of the file's format.
const cosmiconfig = require('cosmiconfig');
const explorer = cosmiconfig('yourModuleName');
explorer.load('/path/to/config').then((result) => {
const config = result ? result.config : {};
// do something with config
}).catch((error) => {
// handle error
});
Creating a custom explorer
This feature allows you to create a custom explorer with specific search places and loaders, enabling you to define where and how configuration files should be found and interpreted.
const cosmiconfig = require('cosmiconfig');
const explorer = cosmiconfig('yourModuleName', {
searchPlaces: ['custom.config.js', 'package.json'],
loaders: {
'.js': cosmiconfig.loadJs,
'.json': cosmiconfig.loadJson
}
});
The 'rc' package is similar to cosmiconfig in that it also searches for and loads configuration files. However, 'rc' focuses on a specific pattern of '.rc' files and does not support as many file formats as cosmiconfig.
The 'config' package is another configuration loader that supports multiple file formats. Unlike cosmiconfig, 'config' is designed for application configuration and has a different approach to file discovery, defaulting to a 'config' directory.
Convict is a configuration management library that includes schema definitions. It is more opinionated than cosmiconfig and includes validation and environment variable support out of the box, which cosmiconfig does not do without additional setup.
Find and load a configuration object from
package.json
property (anywhere down the file tree).config.js
CommonJS module (anywhere down the file tree)For example, if your module's name is "soursocks," cosmiconfig will search out configuration in the following places:
soursocks
property in package.json
(anywhere down the file tree).soursocksrc
file in JSON or YAML format (anywhere down the file tree)soursocks.config.js
file exporting a JS object (anywhere down the file tree)cosmiconfig continues to search in these places all the way down the file tree until it finds acceptable configuration (or hits the home directory).
Additionally, all of these search locations are configurable: you can customize filenames or turn off any location.
You can also look for rc files with extensions, e.g. .soursocksrc.json
or .soursocksrc.yaml
.
You may like extensions on your rc files because you'll get syntax highlighting and linting in text editors.
npm install cosmiconfig
Tested in Node 4+.
var cosmiconfig = require('cosmiconfig');
var explorer = cosmiconfig(yourModuleName[, options]);
explorer.load(yourSearchPath)
.then((result) => {
// result.config is the parsed configuration object
// result.filepath is the path to the config file that was found
})
.catch((parsingError) => {
// do something constructive
});
The function cosmiconfig()
searches for a configuration object and returns a Promise,
which resolves with an object containing the information you're looking for.
You can also pass option sync: true
to load the config synchronously, returning the config itself.
So let's say var yourModuleName = 'goldengrahams'
— here's how cosmiconfig will work:
process.cwd()
(or some other directory defined by the searchPath
argument to load()
), it looks for configuration objects in three places, in this order:
goldengrahams
property in a package.json
file (or some other property defined by options.packageProp
);.goldengrahamsrc
file with JSON or YAML syntax (or some other filename defined by options.rc
);goldengrahams.config.js
JS file exporting the object (or some other filename defined by options.js
)../
, ../
, ../../
, ../../../
, etc., checking those three locations in each directory.options.stopDir
).cosmiconfig()
Promise resolves with its result object.cosmiconfig()
Promise resolves with null
.cosmiconfig()
Promise rejects and shares that error (so you should .catch()
it).All this searching can be short-circuited by passing options.configPath
to specify a file.
cosmiconfig will read that file and try parsing it as JSON, YAML, or JS.
As of v2, cosmiconfig uses a few caches to reduce the need for repetitious reading of the filesystem. Every new cosmiconfig instance (created with cosmiconfig()
) has its own caches.
To avoid or work around caching, you can
cache: false
in your options.var explorer = cosmiconfig(moduleName[, options])
Creates a cosmiconfig instance (i.e. explorer) configured according to the arguments, and initializes its caches.
Type: string
You module name. This is used to create the default filenames that cosmiconfig will look for.
Type: string
or false
Default: '[moduleName]'
Name of the property in package.json
to look for.
If false
, cosmiconfig will not look in package.json
files.
Type: string
or false
Default: '.[moduleName]rc'
Name of the "rc file" to look for, which can be formatted as JSON or YAML.
If false
, cosmiconfig will not look for an rc file.
If rcExtensions: true
, the rc file can also have extensions that specify the syntax, e.g. .[moduleName]rc.json
.
You may like extensions on your rc files because you'll get syntax highlighting and linting in text editors.
Also, with rcExtensions: true
, you can use JS modules as rc files, e.g. .[moduleName]rc.js
.
Type: string
or false
Default: '[moduleName].config.js'
Name of a JS file to look for, which must export the configuration object.
If false
, cosmiconfig will not look for a JS file.
Type: boolean
Default: false
If true
, cosmiconfig will expect rc files to be strict JSON. No YAML permitted, and no sloppy JSON.
By default, rc files are parsed with js-yaml, which is more permissive with punctuation than standard strict JSON.
Type: boolean
Default: false
If true
, cosmiconfig will look for rc files with extensions, in addition to rc files without.
This adds a few steps to the search process.
Instead of just looking for .goldengrahamsrc
(no extension), it will also look for the following, in this order:
.goldengrahamsrc.json
.goldengrahamsrc.yaml
.goldengrahamsrc.yml
.goldengrahamsrc.js
Type: string
Default: Absolute path to your home directory
Directory where the search will stop.
Type: boolean
Default: true
If false
, no caches will be used.
Type: boolean
Default: false
If true
, config will be loaded synchronously.
Type: Function
A function that transforms the parsed configuration. Receives the result object with config
and filepath
properties.
If the option sync
is false
(default), the function must return a Promise that resolves with the transformed result.
If the option sync
is true
, though, transform
should be a synchronous function which returns the transformed result.
The reason you might use this option instead of simply applying your transform function some other way is that the transformed result will be cached. If your transformation involves additional filesystem I/O or other potentially slow processing, you can use this option to avoid repeating those steps every time a given configuration is loaded.
Type: string
If provided, cosmiconfig will load and parse a config from this path, and will not perform its usual search.
explorer
)load([searchPath, configPath])
Find and load a configuration file. Returns a Promise that resolves with null
, if nothing is found, or an object with two properties:
config
: The loaded and parsed configuration.filepath
: The filepath where this configuration was found.You should provide either searchPath
or configPath
. Use configPath
if you know the path of the configuration file you want to load. Otherwise, use searchPath
.
explorer.load('start/search/here');
explorer.load('start/search/at/this/file.css');
explorer.load(null, 'load/this/file.json');
If you provide searchPath
, cosmiconfig will start its search at searchPath
and continue to search up the file tree, as documented above.
If you provide configPath
(i.e. you already know where the configuration is that you want to load), cosmiconfig will try to read and parse that file.
clearFileCache()
Clears the cache used when you provide a configPath
argument to load
.
clearDirectoryCache()
Clears the cache used when you provide a searchPath
argument to load
.
clearCaches()
Performs both clearFileCache()
and clearDirectoryCache()
.
rc serves its focused purpose well. cosmiconfig differs in a few key ways — making it more useful for some projects, less useful for others:
package.json
property, an rc file, a .config.js
file, and rc files with extensions.Please note that this project is released with a Contributor Code of Conduct. By participating in this project you agree to abide by its terms.
And please do participate!
3.0.1
require-from-string
.FAQs
Find and load configuration from a package.json property, rc file, TypeScript module, and more!
The npm package cosmiconfig receives a total of 39,317,792 weekly downloads. As such, cosmiconfig popularity was classified as popular.
We found that cosmiconfig demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.