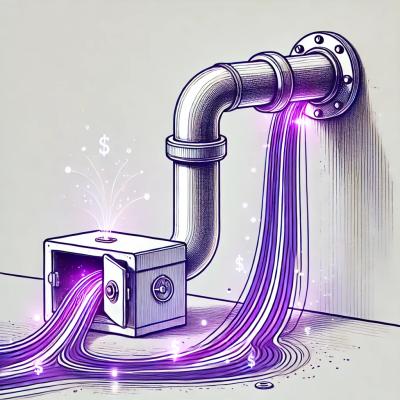
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
couchdb-promises
Advanced tools
All Functions return a Promise object whose fulfillment or failure handler receives an object of 5 properties:
The promise is resolved if the CouchDB status code is < 400 otherwise rejected.
npm install couchdb-promises
const db = require('couchdb-promises')({
baseUrl: 'http://localhost:5984', // required
requestTimeout: 10000
})
const dbName = 'testdb'
db.getInfo()
.then(console.log)
// { headers: { ... },
// data:
// { couchdb: 'Welcome',
// version: '2.0.0',
// vendor: { name: 'The Apache Software Foundation' } },
// status: 200,
// message: 'OK - Request completed successfully'
// duration: 36 }
db.createDatabase(dbName)
.then(console.log)
// { headers: { ... },
// data: { ok: true },
// status: 201,
// message: 'Created - Database created successfully',
// duration: 131 }
.then(() => db.getDatabaseHead(dbName))
.then(console.log)
// { headers: { ... },
// data: {},
// status: 200,
// message: 'OK - Database exists',
// duration: 4 }
.then(() => db.listDatabases())
.then(console.log)
// { headers: { ... },
// data: [ '_replicator', '_users', 'testdb' ],
// status: 200,
// message: 'OK - Request completed successfully',
// duration: 4 }
.then(() => db.createDocument(dbName, {name: 'Bob'}))
.then(console.log)
// { headers: { ... },
// data:
// { ok: true,
// id: 'daae0752c6909d7ca4cd833f46014605',
// rev: '1-5a26fa4b20e40bc9e2d3e47b168be460' },
// status: 201,
// message: 'Created – Document created and stored on disk',
// duration: 42 }
.then(() => db.createDocument(dbName, {name: 'Alice'}, 'doc2'))
.then(console.log)
// { headers: { ... },
// data:
// { ok: true,
// id: 'doc2',
// rev: '1-88b10f13383b5d1e34d1d66d296f061f' },
// status: 201,
// message: 'Created – Document created and stored on disk',
// duration: 38 }
.then(() => db.getDocumentHead(dbName, 'doc2'))
.then(console.log)
// { 'cache-control': 'must-revalidate',
// connection: 'close',
// 'content-length': '74',
// 'content-type': 'application/json',
// date: 'Sun, 06 Nov 2016 08:56:47 GMT',
// etag: '"1-88b10f13383b5d1e34d1d66d296f061f"',
// server: 'CouchDB/2.0.0 (Erlang OTP/17)',
// 'x-couch-request-id': '041e46071b',
// 'x-couchdb-body-time': '0' },
// data: {},
// status: 200,
// message: 'OK - Document exists',
// duration: 6 }
.then(() => db.getDocument(dbName, 'doc2'))
.then(response => { console.log(response); return response.data })
// { headers: { ... },
// data:
// { _id: 'doc2',
// _rev: '1-88b10f13383b5d1e34d1d66d296f061f',
// name: 'Alice' },
// status: 200,
// message: 'OK - Request completed successfully',
// duration: 11 }
.then((doc) => {
doc.age = 42
return db.createDocument(dbName, doc, 'doc2')
})
.then(console.log)
// { headers: { ... },
// data:
// { ok: true,
// id: 'doc2',
// rev: '2-ee5ea9ac0bb1bec913a9b5e7bc11b113' },
// status: 201,
// message: 'Created – Document created and stored on disk',
// duration: 36 }
.then(() => db.getAllDocuments(dbName, {
descending: true,
include_docs: true
}))
.then(console.log)
// { headers: { ... },
// data: { total_rows: 2, offset: 0, rows: [ [Object], [Object] ] },
// status: 200,
// message: 'OK - Request completed successfully',
// duration: 9 }
.then(() => db.deleteDocument(dbName, 'doc2', '2-ee5ea9ac0bb1bec913a9b5e7bc11b113'))
.then(console.log)
// { headers: { ... },
// data:
// { ok: true,
// id: 'doc2',
// rev: '3-ec0a86a95c5e98a5cd52c29b79b66372' },
// status: 200,
// message: 'OK - Document successfully removed',
// duration: 39 }
.then(() => db.copyDocument(dbName, 'doc', 'docCopy'))
.then(console.log)
// { headers: { ... },
// data:
// { ok: true,
// id: 'doc3',
// rev: '1-4c6114c65e295552ab1019e2b046b10e' },
// status: 201,
// message: 'Created – Document created and stored on disk',
// duration: 42 }
.then(() => db.createBulkDocuments(dbName, [
{name: 'Tick'}, {name: 'Trick'}, {name: 'Track'}
], {all_or_nothing: true}))
.then(console.log)
// { headers: { ... },
// data:
// [ { ok: true,
// id: '5413cf41edaedaec6b63aee93db86e1f',
// rev: '1-d7f23e94e65978ea9252d753fe5dc3f6' },
// { ok: true,
// id: '5413cf41edaedaec6b63aee93db877cc',
// rev: '1-646cd5f84634632f42fee2bdf4ff753a' },
// { ok: true,
// id: '5413cf41edaedaec6b63aee93db87c3d',
// rev: '1-9cc8cf1e775b686ca337f69cd39ff772' } ],
// status: 201,
// message: 'Created – Document(s) have been created or updated',
// duration: 74 }
.then(() => db.findDocuments(dbName, {
selector: {
$or: [{ name: 'Tick' }, {name: 'Track'}]
},
fields: ['_id', 'name']
}))
.then(console.log)
// { headers: { ... },
// data:
// { warning: 'no matching index found, create an index to optimize query time',
// docs: [ [Object], [Object] ] },
// status: 200,
// message: 'OK - Request completed successfully',
// duration: 14 }
.then(() => db.getUuids(3))
.then(console.log)
// { headers: { ... },
// data:
// { uuids:
// [ 'daae0752c6909d7ca4cd833f46014c47',
// 'daae0752c6909d7ca4cd833f460150c5',
// 'daae0752c6909d7ca4cd833f460156c5' ] },
// status: 200,
// message: 'OK - Request completed successfully',
// duration: 4 }
.then(() => db.deleteDatabase(dbName))
.then(console.log)
// { headers: { ... },
// data: { ok: true },
// status: 200,
// message: 'OK - Database removed successfully' }
// duration: 40 }
.then(() => db.getUrlPath('_all_dbs'))
.then(console.log)
// { headers: { ... },
// data: [ '_replicator', '_users' ],
// status: 200,
// message: 'OK',
// duration: 6 }
.then(() => db.getDocument(dbName, 'doc1'))
.catch(console.error)
// { headers: { ... },
// data: { error: 'not_found', reason: 'Database does not exist.' },
// status: 404,
// message: 'Not Found - Document not found',
// duration: 5 }
const ddoc = {
language: 'javascript',
views: { view1: { map: 'function (doc) {emit(doc.name, doc.number)}' } }
}
const docId = 'ddoc1'
db.createDesignDocument(dbName, ddoc, docId)
.then(console.log)
// { headers: { ... },
// data:
// { ok: true,
// id: '_design/ddoc1',
// rev: '1-548c68d8cc2c1fac99964990a58f66fd' },
// status: 201,
// message: 'Created – Document created and stored on disk',
// duration: 271 }
db.getDesignDocument(dbName, docId)
.then(console.log)
// { headers: { ... },
// data:
// { _id: '_design/ddoc1',
// _rev: '1-548c68d8cc2c1fac99964990a58f66fd',
// language: 'javascript',
// views: { view1: [Object] } },
// status: 200,
// message: 'OK - Request completed successfully',
// duration: 5 }
db.getDesignDocumentInfo(dbName, docId)
.then(console.log)
// { headers: { ... },
// data:
// { name: 'ddoc1',
// view_index:
// { updates_pending: [Object],
// waiting_commit: false,
// waiting_clients: 0,
// updater_running: false,
// update_seq: 0,
// sizes: [Object],
// signature: '1e86d92af43c47ef58da4b645dbd47f1',
// purge_seq: 0,
// language: 'javascript',
// disk_size: 408,
// data_size: 0,
// compact_running: false } },
// status: 200,
// message: 'OK - Request completed successfully',
// duration: 54 }
db.getView(dbName, docId, viewName, {limit: 3})
.then(console.log)
// { headers: { ... },
// data:
// { total_rows: 12,
// offset: 0,
// rows: [ [Object], [Object], [Object] ] },
// status: 200,
// message: 'OK - Request completed successfully',
// duration: 834 }
db.deleteDesignDocument(dbName, docId, rev)
.then(console.log)
// { headers: { ... },
// data:
// { ok: true,
// id: '_design/ddoc1',
// rev: '2-fd68157ec3c1915ebe0b248343292d34' },
// status: 200,
// message: 'OK - Document successfully removed',
// duration: 49 }
db.executeUpdateFunction(dbName, ddocId, func, queryObj)
db.executeUpdateFunctionForDocument(dbName, ddocId, func, queryObj, docId)
// { headers: { ... },
// status: 201,
// data: { text: 'added 10' },
// message: 'Created - Document was created or updated',
// duration: 49 }
db.createIndex(dbName, {
index: {
fields: ['foo']
},
name: 'foo-index'
})
.then(console.log)
// { headers: { ... },
// data:
// { result: 'exists',
// id: '_design/a5f4711fc9448864a13c81dc71e660b524d7410c',
// name: 'foo-index' },
// status: 200,
// message: 'OK - Index created successfully or already exists',
// duration: 14 }
db.getIndex(dbName)
.then(console.log)
// { headers: { ... },
// data: { total_rows: 2, indexes: [ [Object], [Object] ] },
// status: 200,
// message: 'OK - Success',
// duration: 6 }
db.getIndex(dbName)
.then(response => {
const docId = response.data.indexes.find(e => e.name === 'foo-index').ddoc
return db.deleteIndex(dbName, docId, 'foo-index')
})
.then(console.log)
// { headers: { ... },
// data: { ok: true },
// status: 200,
// message: 'OK - Success',
// duration: 45 }
See examples for details.
The options object has the following properties:
create new database
[CouchDB API]
[example]
get information about the specified database
[CouchDB API]
[example]
get minimal amount of information about the specified database
[CouchDB API]
[example]
delete the specified database
[CouchDB API]
[example]
get list of all databases
[CouchDB API]
[example]
find documents using a declarative JSON querying syntax (CouchDB >= 2.0)
[CouchDB API]
[example]
returns a JSON structure of all of the documents in a given database
[CouchDB API]
[example]
create a new document
[CouchDB API]
[example]
create a new document or a new revision of the existing document.
[CouchDB API]
[example]
marks the specified document as deleted
[CouchDB API]
[example]
get document by the specified docId
[CouchDB API]
[example]
get a minimal amount of information about the specified document
[CouchDB API]
[example]
copy an existing document to a new document
[CouchDB API]
create database index
(CouchDB >= 2.0)
[CouchDB API]
get all database indexes
(CouchDB >= 2.0)
[CouchDB API]
delete database index
(CouchDB >= 2.0)
[CouchDB API]
upload the supplied data as an attachment to the specified document
[CouchDB API]
[example]
get the attachment associated with the document
[CouchDB API] [example]
get minimal amount of information about the specified attachment
[CouchDB API]
[example]
deletes the attachment attachment of the specified doc
[CouchDB API]
[example]
create new design document or new revision of an existing design document
[CouchDB API]
[example]
delete design document
[CouchDB API]
[example]
get the contents of the design document
[CouchDB API]
[example]
obtain information about the specified design document, including the index, index size and current status of the design document and associated index information
[CouchDB API]
[example]
execute the specified view function from the specified design document
[CouchDB API]
[example]
create or update multiple documents at the same time within a single request
[CouchDB API]
[example]
get meta information about the CouchDB server
[CouchDB API]
[example]
get one or more Universally Unique Identifiers (UUIDs) from the CouchDB server
[CouchDB API]
[example]
generic http GET request function
[example]
3.0.0 - 2017-02-21
old:
const db = require('couchdb-promises')({})
const baseUrl = 'http://localhost:5984'
db.getDocument(baseUrl, dbName, 'mydoc')
new:
const db = require('couchdb-promises')({
baseUrl: 'http://localhost:5984'
})
db.getDocument(dbName, 'mydoc')
FAQs
ES6 promises-based CouchDB client
The npm package couchdb-promises receives a total of 25 weekly downloads. As such, couchdb-promises popularity was classified as not popular.
We found that couchdb-promises demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.