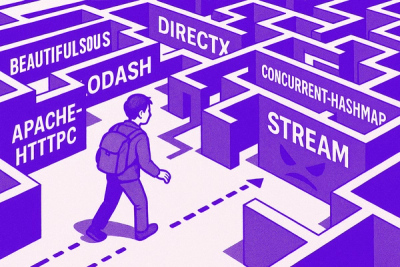
Research
NPM targeted by malware campaign mimicking familiar library names
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
CouchSet is a Couchbase ORM & Automatic GraphQL API code generator (Resolver/Queries) tool
npm i couchset --save
import { couchset } from 'couchset';
const started = await couchset({
connectionString: 'couchbase://localhost',
username: 'admin',
password: '123456',
bucketName: 'stq'
})
import { Model } from 'couchset';
const userModel = new Model('User');
// Create document
const created = await userModel.create({
username: 'ceddy',
password: 'love couchbase',
});
// Find document
const foundData = await userModel.findById(created.id);
// update document
const updatedData = await userModel.updateById(created.id, { ...created, someValue: 'x' });
// delete
const deletedData = await userModel.delete(created.id);
All models come with a method for automatic pagination
const paginationData = await userModel.pagination({
select: ["id", "email", "phone","fullname"],
where: {
userId: { $eq: "ceddy" },
$or: [{ userId: { $eq: "ceddy" } }, { phone: 10 }],
},
limit: 100,
page: 0,
});
which translates to this query
SELECT * FROM `stq` WHERE _type="User" AND userId="ceddy" AND (userId="ceddy" OR phone=10) ORDER BY createdAt DESC LIMIT 100 OFFSET 0
Pagination results
[
{
id: '209d3143-09b7-4b3d-bf7d-f0ccd3f98922',
updatedAt: 2021-01-26T01:51:43.218Z,
createdAt: 2021-01-26T01:51:43.210Z,
_type: 'User',
userId: 'ceddy',
password: '...',
someValue: 'x'
},
{
id: '1392e4f6-ae1e-4e01-b7d5-103bdd0e843f',
updatedAt: 2021-01-26T01:51:29.591Z,
createdAt: 2021-01-26T01:51:29.583Z,
_type: 'User',
userId: 'ceddy',
password: '...',
someValue: 'x'
}
]
Query builder is inspired from node-ottoman, for more examples, please see https://ottomanjs.com/guides/query-builder.html#query-builder
import { Query } from 'couchset';
const params = {
select: [
{
$count: {
$field: {
name: 'type',
},
as: 'odm',
},
},
],
let: [
{ key: 'amount_val', value: 10 },
{ key: 'size_val', value: 20 },
],
where: {
$or: [{ price: { $gt: 'amount_val', $isNotNull: true } }, { auto: { $gt: 10 } }, { amount: 10 }],
$and: [
{ price2: { $gt: 1.99, $isNotNull: true } },
{ $or: [{ price3: { $gt: 1.99, $isNotNull: true } }, { id: '20' }] },
],
$any: {
$expr: [{ $in: { search_expr: 'search', target_expr: 'address' } }],
$satisfied: { address: '10' },
},
$in: { search_expr: 'search', target_expr: ['address'] },
},
groupBy: [{ expr: 'type', as: 'sch' }],
letting: [
{ key: 'amount_v2', value: 10 },
{ key: 'size_v2', value: 20 },
],
having: { type: { $like: '%hotel%' } },
orderBy: { type: 'DESC' },
limit: 10,
offset: 1,
use: ['airlineR_8093', 'airlineR_8094'],
};
const query = new Query(params, 'travel-sample').build();
console.log(query);
which translates to
SELECT COUNT(type) AS odm FROM travel-sample USE KEYS ["airlineR_8093","airlineR_8094"] LET amount_val=10,size_val=20 WHERE ((price>amount_val AND price IS NOT NULL) OR auto>10 OR amount=10) AND ((price2>1.99 AND price2 IS NOT NULL) AND ((price3>1.99 AND price3 IS NOT NULL) OR id="20")) AND ANY search IN address SATISFIES address="10" END AND search IN ["address"] GROUP BY type AS sch LETTING amount_v2=10,size_v2=20 HAVING type LIKE "%hotel%" ORDER BY type DESC LIMIT 10 OFFSET 1
import { QueryCluster } from 'couchset';
const queryresults = await QueryCluster(queryBuilder);
// queryresults = { rows: object[], meta: any}
This is how we automate it to generate code with all methods,schema, queries
const automaticUser = userModel.automate();
After automating the model, automaticUser
will come with Server-side Resolver functions, and client queries, mutations, subscriptions, like below
// Get all automatic generated resolvers and queries/fragments,mutations,subscriptions
const {
resolver: UserResolver, // Server resolver for building GraphQL
modelKeys: UserSelectors, // for any custom queries or exporting
client, // client queries,mutations,subscriptions
} = automaticUser;
TODO
save
, update
, findMany
e.t.c ✅
Couchset is MIT licensed.
FAQs
Couchbase ORM
The npm package couchset receives a total of 43 weekly downloads. As such, couchset popularity was classified as not popular.
We found that couchset demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
Research
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.