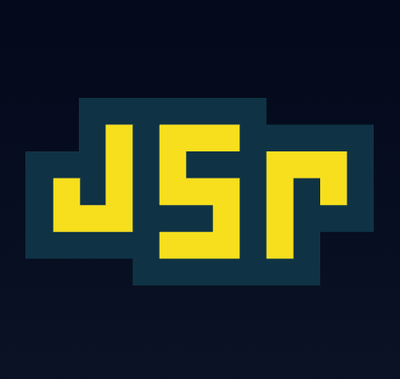
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
crc32-stream
Advanced tools
The crc32-stream npm package is designed for generating CRC32 checksums from streams of data. It is particularly useful for validating the integrity of data being transferred or stored. The package provides a way to compute CRC32 checksums efficiently on-the-fly for both streams of data and individual files.
CRC32 Checksum for Streams
This feature allows you to create a CRC32 checksum for data streams. The code sample demonstrates how to pipe a file stream through the CRC32Stream to compute its checksum.
const {CRC32Stream} = require('crc32-stream');
const fs = require('fs');
const source = fs.createReadStream('path/to/file');
const crc32Stream = new CRC32Stream();
source.pipe(crc32Stream).on('finish', function() {
console.log('CRC32 Checksum:', crc32Stream.digest().toString(16));
});
CRC32 Checksum for Files
This feature demonstrates how to compute and append a CRC32 checksum for files. It shows the process of reading a file, computing the checksum as the file is being processed, and then writing the output to another file.
const {CRC32Stream} = require('crc32-stream');
const fs = require('fs');
const crc32Stream = new CRC32Stream();
const output = fs.createWriteStream('output.file');
fs.createReadStream('input.file')
.pipe(crc32Stream)
.pipe(output)
.on('finish', function() {
console.log('File processed with CRC32 Checksum:', crc32Stream.digest().toString(16));
});
The 'crc' package offers a collection of CRC (Cyclic Redundancy Check) algorithms for strings and buffers. Unlike crc32-stream, which is stream-focused, 'crc' provides a more general approach to CRC calculation, supporting various CRC algorithms but without native stream support.
This package is designed to validate streams using various hash algorithms, including CRC32. It is similar to crc32-stream in its stream-oriented approach but offers more flexibility by supporting additional hash algorithms like MD5 and SHA-1.
crc32-stream is a streaming CRC32 checksumer. It uses buffer-crc32 behind the scenes to reliably handle binary data and fancy character sets. Data is passed through untouched.
npm install crc32-stream --save
You can also use npm install https://github.com/archiverjs/node-crc32-stream/archive/master.tar.gz
to test upcoming versions.
Inherits Transform Stream options and methods.
var CRC32Stream = require('crc32-stream');
var source = fs.createReadStream('file.txt');
var checksum = new CRC32Stream();
checksum.on('end', function(err) {
// do something with checksum.digest() here
});
// either pipe it
source.pipe(checksum);
// or write it
checksum.write('string');
checksum.end();
Inherits zlib.DeflateRaw options and methods.
var DeflateCRC32Stream = require('crc32-stream').DeflateCRC32Stream;
var source = fs.createReadStream('file.txt');
var checksum = new DeflateCRC32Stream();
checksum.on('end', function(err) {
// do something with checksum.digest() here
});
// either pipe it
source.pipe(checksum);
// or write it
checksum.write('string');
checksum.end();
Returns the checksum digest in unsigned form.
Returns the hexadecimal representation of the checksum digest. (ie E81722F0)
Returns the raw size/length of passed-through data.
If compressed
is true
, it returns compressed length instead. (DeflateCRC32Stream)
FAQs
a streaming CRC32 checksumer
The npm package crc32-stream receives a total of 7,394,087 weekly downloads. As such, crc32-stream popularity was classified as popular.
We found that crc32-stream demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.