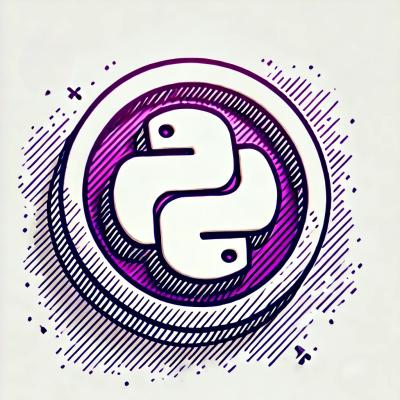
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
cron-validate
Advanced tools
The cron-validate npm package is used to validate and parse cron expressions. It ensures that cron expressions are syntactically correct and can be used to schedule tasks in a reliable manner.
Basic Validation
This feature allows you to validate a basic cron expression. The code sample checks if the cron expression '0 0 * * *' is valid.
const cronValidate = require('cron-validate');
const result = cronValidate('0 0 * * *');
console.log(result.isValid()); // true
Custom Validation Options
This feature allows you to validate a cron expression with custom options. The code sample uses a preset option to validate the cron expression.
const cronValidate = require('cron-validate');
const options = { preset: 'npm-package' };
const result = cronValidate('0 0 * * *', options);
console.log(result.isValid()); // true
Detailed Error Messages
This feature provides detailed error messages for invalid cron expressions. The code sample demonstrates how to get an error message for an invalid cron expression.
const cronValidate = require('cron-validate');
const result = cronValidate('invalid-cron');
console.log(result.getError()); // Detailed error message
The cron-parser package is used to parse and manipulate cron expressions. It provides similar validation capabilities but also includes features for parsing and iterating over cron schedules. Compared to cron-validate, it offers more advanced manipulation of cron expressions.
The node-cron package is a task scheduler in pure JavaScript for node.js based on cron syntax. While it includes validation of cron expressions, its primary focus is on scheduling tasks rather than just validation. It is more feature-rich in terms of task scheduling compared to cron-validate.
The cron package is another task scheduler for node.js that uses cron syntax. It includes validation as part of its scheduling capabilities. Similar to node-cron, it is more focused on task scheduling and execution rather than just validation.
Cron-validate is a cron-expression validator written in TypeScript. The validation options are customizable and cron fields like seconds and years are supported.
Pacakge is available on npm:
npm install -S cron-validate
import cron from 'cron-validate'
const cronResult = cron('* * * * *')
if (cronResult.isValid()) {
// !cronResult.isError()
// valid code
} else {
// error code
}
The cron
function returns a Result-type, which is either Valid<T, E>
or Err<T, E>
.
For checking the returned result, just use result.isValid()
or result.isError()
Both result types contain values:
import cron from 'cron-validate'
const cronResult = cron('* * * * *')
if (cronResult.isValid()) {
const validValue = cronResult.getValue()
// The valid value is a object containing all cron fields
console.log(validValue)
// In this case, it would be:
// { seconds: undefined, minutes: '*', hours: '*', daysOfMonth: '*', months: '*', daysOfWeek: '*', years: undefiend }
} else {
const errorValue = cronResult.getError()
// The error value contains an array of strings, which represent the cron validation errors.
console.log(errorValue) // string[] of error messages
}
Make sure to test the result type beforehand, because getValue()
only works on Valid
and getError()
only works on Err
. If you don't check, it will throw an error.
For further information, you can check out https://github.com/gDelgado14/neverthrow, because I used and modified his code for this package. (Therefor not every documented function on his package is available on this package.)
To configure the validator, cron-validate uses a preset system. There are already defined presets (default, npm-node-cron or aws), but you can also define your own preset to use for your system. You can also use the override property to set certain option on single cron validates.
The following presets are already defined by cron-validate:
To select a preset for your validation, you can simply do this:
cron('* * * * *', {
preset: 'npm-cron-schedule',
})
To define your own preset, use this:
registerOptionPreset('YOUR-PRESET-ID', {
presetId: 'YOUR-PRESET-ID',
useSeconds: false,
useYears: false,
useAliases: false, // optional, default to false
useBlankDay: false,
allowOnlyOneBlankDayField: false,
mustHaveBlankDayField: false, // optional, default to false
useLastDayOfMonth: false, // optional, default to false
useLastDayOfWeek: false, // optional, default to false
useNearestWeekday: false, // optional, default to false
useNthWeekdayOfMonth: false, // optional, default to false
seconds: {
minValue: 0,
maxValue: 59,
lowerLimit: 0, // optional, default to minValue
upperLimit: 59, // optional, default to maxValue
},
minutes: {
minValue: 0,
maxValue: 59,
lowerLimit: 0, // optional, default to minValue
upperLimit: 59, // optional, default to maxValue
},
hours: {
minValue: 0,
maxValue: 23,
lowerLimit: 0, // optional, default to minValue
upperLimit: 23, // optional, default to maxValue
},
daysOfMonth: {
minValue: 1,
maxValue: 31,
lowerLimit: 1, // optional, default to minValue
upperLimit: 31, // optional, default to maxValue
},
months: {
minValue: 0,
maxValue: 12,
lowerLimit: 0, // optional, default to minValue
upperLimit: 12, // optional, default to maxValue
},
daysOfWeek: {
minValue: 1,
maxValue: 7,
lowerLimit: 1, // optional, default to minValue
upperLimit: 7, // optional, default to maxValue
},
years: {
minValue: 1970,
maxValue: 2099,
lowerLimit: 1970, // optional, default to minValue
upperLimit: 2099, // optional, default to maxValue
},
})
The preset properties explained:
presetId: string
useSeconds: boolean
useYears: boolean
useAliases: boolean
useBlankDay: boolean
allowOnlyOneBlankDayField: boolean
mustHaveBlankDayField: boolean
allowOnlyOneBlankDayField
, it means that there will always be either day or day of week as ?
useLastDayOfMonth: boolean
L-2
would me the 2nd to last day of the month.useLastDayOfWeek: boolean
5L
would mean the last friday of the month.useNearestWeekday: boolean
15W
would mean the weekday (mon-fri) closest to the 15th when the 15th is on sat-sun.useNthWeekdayOfMonth: boolean
6#3
would mean the 3rd friday of the month (assuming 6 = friday).minValue: number
maxValue: number
lowerLimit?: number
upperLimit?: number
If you want to override a option for single cron validations, you can use the override
property:
console.log(cron('* * * * * *', {
preset: 'default' // second field not supported in default preset
override: {
useSeconds: true // override preset option
}
}))
console.log(cron('* 10-20 * * * *', {
preset: 'default'
override: {
minutes: {
lowerLimit: 10, // override preset option
upperLimit: 20 // override preset option
}
}
}))
import cron from 'cron-validate'
console.log(cron('* * * * *').isValid()) // true
console.log(cron('* * * * *').isError()) // false
console.log(cron('* 2,3,4 * * *').isValid()) // true
console.log(cron('0 */2 */5 * *').isValid()) // true
console.log(cron('* * * * * *', { override: { useSeconds: true } }).isValid()) // true
console.log(cron('* * * * * *', { override: { useYears: true } }).isValid()) // true
console.log(
cron('30 * * * * *', {
override: {
useSeconds: true,
seconds: {
lowerLimit: 20,
upperLimit: 40,
},
},
}).isValid()
) // true
console.log(
cron('* 3 * * *', {
override: {
hours: {
lowerLimit: 0,
upperLimit: 2,
},
},
}).isValid()
) // false
console.log(
cron('* * ? * *', {
override: {
useBlankDay: true,
},
}).isValid()
) // true
console.log(
cron('* * ? * ?', {
override: {
useBlankDay: true,
allowOnlyOneBlankDayField: true,
},
}).isValid()
) // false
FAQs
cron-validate is a cron-expression validator written in TypeScript.
We found that cron-validate demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.