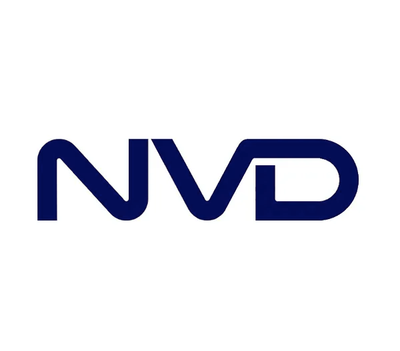
Security News
NIST Misses 2024 Deadline to Clear NVD Backlog
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
The cross-zip npm package is a utility for zipping and unzipping files and directories in a cross-platform manner. It is designed to work seamlessly on both Windows and Unix-like systems, making it a versatile tool for handling zip files in Node.js applications.
Zip a directory
This feature allows you to zip an entire directory. The code sample demonstrates how to zip a directory named 'sourceDir' into a file named 'output.zip'.
const crossZip = require('cross-zip');
crossZip.zip('sourceDir', 'output.zip', (err) => {
if (err) {
console.error('Error zipping directory:', err);
} else {
console.log('Directory zipped successfully');
}
});
Unzip a file
This feature allows you to unzip a zip file into a specified directory. The code sample demonstrates how to unzip a file named 'input.zip' into a directory named 'destinationDir'.
const crossZip = require('cross-zip');
crossZip.unzip('input.zip', 'destinationDir', (err) => {
if (err) {
console.error('Error unzipping file:', err);
} else {
console.log('File unzipped successfully');
}
});
ADM-ZIP is a pure JavaScript implementation for zip data compression for NodeJS. It allows you to create, read, and extract zip files. Compared to cross-zip, ADM-ZIP offers more advanced features like adding files to an existing zip and extracting specific files from a zip.
Yazl is a zip file creation library with a focus on performance and streaming. It allows you to create zip files with a streaming API, which can be more efficient for large files. Unlike cross-zip, yazl does not provide unzipping functionality; it focuses solely on creating zip files.
Archiver is a streaming interface for archive generation, supporting zip and other formats. It provides a comprehensive API for creating archives and is highly configurable. Archiver is more feature-rich compared to cross-zip, offering support for multiple archive formats and advanced options for compression.
npm install cross-zip
var zip = require('cross-zip')
var inPath = path.join(__dirname, 'myFolder') // folder to zip
var outPath = path.join(__dirname, 'myFile.zip') // name of output zip file
zip.zipSync(inPath, outPath)
zip.zip(inPath, outPath, [callback])
Zip the folder at inPath
and save it to a .zip file at outPath
. If a callback
is passed, then it is called with an Error
or null
.
zip.zipSync(inPath, outPath)
Sync version of zip.zip
.
zip.unzip(inPath, outPath, [callback])
Unzip the .zip file at inPath
into the folder at outPath
. If a callback
is
passed, then it is called with an Error
or null
.
zip.unzipSync(inPath, outPath)
Sync version of zip.unzip
.
This package requires .NET Framework 4.5 or later and Powershell 3. These come pre-installed on Windows 8 or later.
On Windows 7 or earlier, you will need to install these manually in order for
cross-zip
to function correctly.
MIT. Copyright (c) Feross Aboukhadijeh.
FAQs
Cross-platform .zip file creation
The npm package cross-zip receives a total of 136,493 weekly downloads. As such, cross-zip popularity was classified as popular.
We found that cross-zip demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.