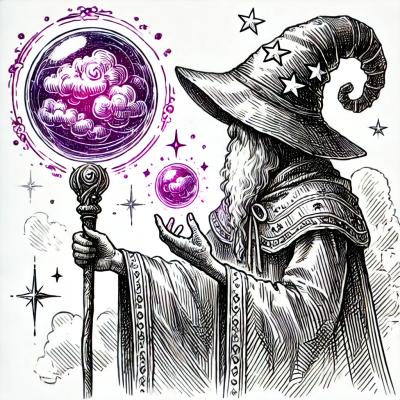
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
CSSO (CSS Optimizer) is a CSS minifier. It performs three kinds of optimizations: structural optimizations, reducing CSS size by merging blocks with identical properties, removing overridden properties, etc.; cleaning (removing unused @media rules, cutting out the comments, etc.); and compressing (transforming values to shorter forms, merging identical selectors, etc.). It can be used as a command-line tool or as a library.
Minification
Minifies CSS by removing whitespace, comments, and making other optimizations to reduce file size.
const csso = require('csso');
const minifiedCss = csso.minify('.test { color: #ff0000; }').css;
Structural Optimization
Optimizes CSS structure by merging blocks with identical properties and removing overridden properties.
const csso = require('csso');
const optimizedCss = csso.minify('.test { color: red; } .test { font-size: 16px; }', { restructure: true }).css;
Source Map Generation
Generates a source map that can be used to debug the minified CSS by mapping it back to the original sources.
const csso = require('csso');
const result = csso.minify('.test { color: red; }', { sourceMap: true });
const minifiedCss = result.css;
const map = result.map.toString();
clean-css is a fast and efficient CSS optimizer for Node.js and the Web. It provides similar minification capabilities as CSSO but also offers advanced optimizations like restructuring.
uglifycss is a CSS minifier that aims to be fast and simple. It doesn't have as many features as CSSO, focusing mainly on removing whitespace and comments to compress CSS files.
purifycss is a tool to remove unused CSS. Unlike CSSO, which focuses on optimizing existing CSS, purifycss analyzes your content and CSS files to remove unused selectors.
CSSO (CSS Optimizer) is a CSS minimizer unlike others. In addition to usual minification techniques it can perform structural optimization of CSS files, resulting in smaller file size compared to other minifiers.
npm install -g csso
csso [input] [output] [options]
Options:
--debug Output intermediate state of CSS during compression
-h, --help Output usage information
-i, --input <filename> Input file
-o, --output <filename> Output file (result outputs to stdout if not set)
--restructure-off Turns structure minimization off
-v, --version Output the version
Some examples:
> csso in.css out.css
> csso in.css
...output result in stdout...
> echo ".test { color: #ff0000; }" | csso
.test{color:red}
> cat source1.css source2.css | csso | gzip -9 -c > production.css.gz
var csso = require('csso');
var compressed = csso.minify('.test { color: #ff0000; }');
console.log(compressed);
// .test{color:red}
// you may do it step by step
var ast = csso.parse('.test { color: #ff0000; }');
ast = csso.compress(ast);
var compressed = csso.translate(ast, true);
console.log(compressed);
// .test{color:red}
May be outdated
FAQs
CSS minifier with structural optimisations
The npm package csso receives a total of 12,056,742 weekly downloads. As such, csso popularity was classified as popular.
We found that csso demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.