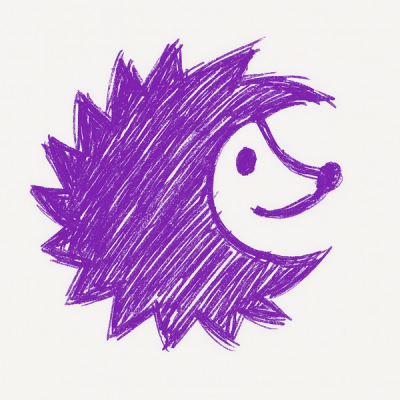
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
The cssstyle npm package is designed to mimic the CSSStyleDeclaration interface provided by browsers, allowing for the manipulation of CSS properties of elements in a JavaScript environment outside of the browser, such as in server-side applications or testing environments. It provides a way to parse, manipulate, and stringify CSS properties programmatically.
Parsing CSS properties
This feature allows the parsing of CSS text into individual properties that can be accessed and manipulated through the CSSStyleDeclaration object.
{"var CSSStyleDeclaration = require('cssstyle');
var css = new CSSStyleDeclaration();
css.cssText = 'color: blue; background-color: white;';
console.log(css.color); // 'blue'"}
Manipulating CSS properties
This feature enables the setting and getting of individual CSS properties using methods like setProperty and getPropertyValue.
{"var CSSStyleDeclaration = require('cssstyle');
var css = new CSSStyleDeclaration();
css.setProperty('color', 'red');
console.log(css.cssText); // 'color: red;'"}
Stringifying CSS properties
This feature allows the CSSStyleDeclaration object to be converted back into a string representation of the CSS, which can be used in HTML or other contexts.
{"var CSSStyleDeclaration = require('cssstyle');
var css = new CSSStyleDeclaration();
css.setProperty('color', 'red');
console.log(css.cssText); // 'color: red;'"}
jsdom is a JavaScript implementation of many web standards, including the DOM and HTML standards. It provides a way to simulate a web page environment, including CSS parsing and manipulation, similar to cssstyle but with a broader scope including the full DOM API.
styled-components is a library for React and React Native that allows developers to write CSS in JavaScript, using tagged template literals. It offers a different approach to styling components compared to cssstyle, focusing on component-level styles in a React ecosystem.
JSS is an authoring tool for CSS which allows you to use JavaScript to describe styles in a declarative, conflict-free and reusable way. It is similar to cssstyle in that it allows manipulation of CSS in JavaScript, but it is more focused on generating CSS using JavaScript objects and functions.
CSSStyleDeclaration is a work-a-like to the CSSStyleDeclaration class in Nikita Vasilyev's CSSOM. I made it so that when using jQuery in node setting css attributes via $.fn.css() would work. node-jquery uses jsdom to create a DOM to use in node. jsdom uses CSSOM for styling, and CSSOM's implementation of the CSSStyleDeclaration doesn't support CSS2Properties, which is how jQuery's $.fn.css() operates.
Well, NV wants to keep CSSOM fast (which I can appreciate) and CSS2Properties aren't required by the standard (though every browser has the interface). So I figured the path of least resistence would be to just modify this one class, publish it as a node module (that requires CSSOM) and then make a pull request of jsdom to use it.
FAQs
CSSStyleDeclaration Object Model implementation
The npm package cssstyle receives a total of 27,140,329 weekly downloads. As such, cssstyle popularity was classified as popular.
We found that cssstyle demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.