csv-exporter-utility
Advanced tools
Comparing version 1.0.2 to 1.1.0
@@ -5,3 +5,8 @@ /** | ||
* @param {string} [filename='data'] - The filename for the downloaded CSV file. | ||
* @param {string[]} [omitProperties=[]] - Array of properties to omit, supports dot notation for nested properties. | ||
* | ||
* | ||
* @author Arslan Ameer | ||
* @see https://www.arslanameer.com | ||
*/ | ||
export declare function downloadCSVFile(data: object[], filename?: string): void; | ||
export declare function downloadCSVFile(data: object[], filename?: string, omitProperties?: string[]): void; |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.downloadCSVFile = void 0; | ||
exports.downloadCSVFile = downloadCSVFile; | ||
/** | ||
@@ -8,8 +8,13 @@ * Downloads a CSV file from given JSON data. | ||
* @param {string} [filename='data'] - The filename for the downloaded CSV file. | ||
* @param {string[]} [omitProperties=[]] - Array of properties to omit, supports dot notation for nested properties. | ||
* | ||
* | ||
* @author Arslan Ameer | ||
* @see https://www.arslanameer.com | ||
*/ | ||
function downloadCSVFile(data, filename = 'data') { | ||
// Get headers from the data | ||
const headers = Object.keys(data[0]); | ||
function downloadCSVFile(data, filename = 'data', omitProperties = []) { | ||
// Get headers from the data, excluding omitted properties | ||
const headers = Object.keys(data[0]).filter(header => !shouldOmit(header, omitProperties)); | ||
// Convert JSON data to CSV format | ||
const csvData = convertJsonToCSV(data, headers); | ||
const csvData = convertJsonToCSV(data, headers, omitProperties); | ||
// Create a Blob with the CSV data and appropriate MIME type | ||
@@ -35,3 +40,2 @@ const blob = new Blob(['\ufeff' + csvData], { type: 'text/csv;charset=utf-8;' }); | ||
} | ||
exports.downloadCSVFile = downloadCSVFile; | ||
/** | ||
@@ -41,5 +45,6 @@ * Converts JSON data to CSV format. | ||
* @param {string[]} headerList - The list of headers for the CSV file. | ||
* @param {string[]} omitProperties - Array of properties to omit. | ||
* @returns {string} - The CSV formatted string. | ||
*/ | ||
function convertJsonToCSV(objArray, headerList) { | ||
function convertJsonToCSV(objArray, headerList, omitProperties) { | ||
// Ensure objArray is an object array | ||
@@ -51,5 +56,32 @@ const array = typeof objArray !== 'object' ? JSON.parse(objArray) : objArray; | ||
array.forEach((row, rowIndex) => { | ||
csv += `${rowIndex + 1},${headerList.map(header => row[header]).join(',')}\r\n`; | ||
const csvRow = headerList.map(header => { | ||
if (shouldOmit(header, omitProperties)) { | ||
return ''; | ||
} | ||
return getNestedProperty(row, header); | ||
}).join(','); | ||
csv += `${rowIndex + 1},${csvRow}\r\n`; | ||
}); | ||
return csv; | ||
} | ||
/** | ||
* Determines if a property should be omitted based on the omit list. | ||
* @param {string} property - The property name to check. | ||
* @param {string[]} omitProperties - The list of properties to omit. | ||
* @returns {boolean} - True if the property should be omitted, false otherwise. | ||
*/ | ||
function shouldOmit(property, omitProperties) { | ||
return omitProperties.some(omitProp => { | ||
const regex = new RegExp(`^${omitProp.replace('.', '\\.')}`); | ||
return regex.test(property); | ||
}); | ||
} | ||
/** | ||
* Retrieves a nested property value from an object based on dot notation. | ||
* @param {object} obj - The object to retrieve the property from. | ||
* @param {string} path - The path to the property in dot notation. | ||
* @returns {any} - The value of the nested property, or undefined if not found. | ||
*/ | ||
function getNestedProperty(obj, path) { | ||
return path.split('.').reduce((acc, part) => acc && acc[part], obj); | ||
} |
{ | ||
"name": "csv-exporter-utility", | ||
"version": "1.0.2", | ||
"version": "1.1.0", | ||
"description": "Downloads a CSV file from given JSON data", | ||
@@ -21,3 +21,4 @@ "main": "dist/index.js", | ||
"file-generation", | ||
"data-export" | ||
"data-export", | ||
"browser" | ||
], | ||
@@ -24,0 +25,0 @@ "author": "Arslan Ameer", |
@@ -12,2 +12,6 @@ <p align="center" float="left"> | ||
[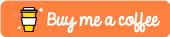](https://www.buymeacoffee.com/arslanameer) | ||
## Why use CSV Exporter Utility | ||
@@ -19,2 +23,4 @@ | ||
- Automatically generates CSV headers from JSON keys | ||
- Allows omission of specific properties from the CSV export | ||
- Supports nested properties omission with dot notation | ||
- Cross-browser compatibility | ||
@@ -37,3 +43,3 @@ - User-friendly file download experience | ||
3. Use the `downloadCSVFile` function in your application to convert and download JSON data as a CSV file: | ||
3. Use the `downloadCSVFile` function in your application to convert and download JSON data as a CSV file. You can also pass an array of property names to omit certain fields from the CSV: | ||
@@ -46,7 +52,19 @@ ```typescript | ||
downloadCSVFile(jsonData, "users"); | ||
// Omit the "email" field from the CSV | ||
downloadCSVFile(jsonData, "users", ["email"]); | ||
``` | ||
The above example will download a CSV file named `users.csv` containing the data from the `jsonData` array. | ||
In the above example, the `email` field is omitted from the downloaded `users.csv` file. | ||
### Additional support: Dot notation for nested properties | ||
You can also omit nested properties using dot notation: | ||
```typescript | ||
// Omit the "user.age" field from the CSV | ||
downloadCSVFile(jsonData, "users", ["user.age"]); | ||
``` | ||
This will omit the `age` field inside the `user` object, allowing more control over complex JSON data structures. | ||
## When to use CSV Exporter Utility | ||
@@ -59,2 +77,3 @@ | ||
- Convert JSON data to CSV for further processing or analysis | ||
- Omit specific properties (including nested ones) from the CSV output | ||
- Integrate a lightweight CSV export solution without external dependencies | ||
@@ -93,2 +112,19 @@ | ||
Thank you for your interest in contributing to CSV Exporter Utility! | ||
Thank you for your interest in contributing to CSV Exporter Utility! | ||
[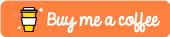](https://www.buymeacoffee.com/arslanameer) | ||
# Changelog | ||
### v1.1.0 - [September 7, 2024] | ||
- **New Features**: | ||
- Added support to omit specific properties from CSV output using the `omitProperties` parameter. | ||
- Enhanced functionality to omit nested properties using dot notation (e.g., `"user.age"` will omit the `age` field from the `user` object). | ||
- **Improvements**: | ||
- Refined CSV formatting logic to handle omitted properties more efficiently. | ||
- Improved error handling for scenarios with missing or invalid property paths in dot notation. | ||
- **Bug Fixes**: | ||
- Resolved an issue where certain fields with nested structures were not properly converted to CSV. |
140
src/index.ts
@@ -5,56 +5,90 @@ /** | ||
* @param {string} [filename='data'] - The filename for the downloaded CSV file. | ||
* @param {string[]} [omitProperties=[]] - Array of properties to omit, supports dot notation for nested properties. | ||
* | ||
* | ||
* @author Arslan Ameer | ||
* @see https://www.arslanameer.com | ||
*/ | ||
export function downloadCSVFile(data: object[], filename = 'data') { | ||
// Get headers from the data | ||
const headers = Object.keys(data[0]); | ||
// Convert JSON data to CSV format | ||
const csvData = convertJsonToCSV(data, headers); | ||
// Create a Blob with the CSV data and appropriate MIME type | ||
const blob = new Blob(['\ufeff' + csvData], { type: 'text/csv;charset=utf-8;' }); | ||
// Create a download link | ||
const dwldLink = document.createElement("a"); | ||
const url = URL.createObjectURL(blob); | ||
// Check if the browser is Safari | ||
const isSafariBrowser = navigator.userAgent.indexOf('Safari') !== -1 && navigator.userAgent.indexOf('Chrome') === -1; | ||
if (isSafariBrowser) { | ||
// If Safari, open in a new window to save the file with a random filename | ||
dwldLink.setAttribute("target", "_blank"); | ||
} | ||
// Set download attributes | ||
dwldLink.setAttribute("href", url); | ||
dwldLink.setAttribute("download", `${filename}.csv`); | ||
dwldLink.style.visibility = "hidden"; | ||
// Add the link to the DOM, click it, and remove it | ||
document.body.appendChild(dwldLink); | ||
dwldLink.click(); | ||
document.body.removeChild(dwldLink); | ||
export function downloadCSVFile(data: object[], filename = 'data', omitProperties: string[] = []) { | ||
// Get headers from the data, excluding omitted properties | ||
const headers = Object.keys(data[0]).filter(header => !shouldOmit(header, omitProperties)); | ||
// Convert JSON data to CSV format | ||
const csvData = convertJsonToCSV(data, headers, omitProperties); | ||
// Create a Blob with the CSV data and appropriate MIME type | ||
const blob = new Blob(['\ufeff' + csvData], { type: 'text/csv;charset=utf-8;' }); | ||
// Create a download link | ||
const dwldLink = document.createElement("a"); | ||
const url = URL.createObjectURL(blob); | ||
// Check if the browser is Safari | ||
const isSafariBrowser = navigator.userAgent.indexOf('Safari') !== -1 && navigator.userAgent.indexOf('Chrome') === -1; | ||
if (isSafariBrowser) { | ||
// If Safari, open in a new window to save the file with a random filename | ||
dwldLink.setAttribute("target", "_blank"); | ||
} | ||
/** | ||
* Converts JSON data to CSV format. | ||
* @param {object[]} objArray - The JSON data to be converted. | ||
* @param {string[]} headerList - The list of headers for the CSV file. | ||
* @returns {string} - The CSV formatted string. | ||
*/ | ||
function convertJsonToCSV(objArray: object[], headerList: string[]): string { | ||
// Ensure objArray is an object array | ||
const array = typeof objArray !== 'object' ? JSON.parse(objArray as unknown as string) : objArray; | ||
// Create the header row | ||
let csv = headerList.map((header, index) => index === 0 ? `S.No,${header}` : header).join(',') + '\r\n'; | ||
// Iterate through the data and create CSV rows | ||
array.forEach((row: Record<string, any>, rowIndex: number) => { | ||
csv += `${rowIndex + 1},${headerList.map(header => row[header]).join(',')}\r\n`; | ||
}); | ||
return csv; | ||
} | ||
// Set download attributes | ||
dwldLink.setAttribute("href", url); | ||
dwldLink.setAttribute("download", `${filename}.csv`); | ||
dwldLink.style.visibility = "hidden"; | ||
// Add the link to the DOM, click it, and remove it | ||
document.body.appendChild(dwldLink); | ||
dwldLink.click(); | ||
document.body.removeChild(dwldLink); | ||
} | ||
/** | ||
* Converts JSON data to CSV format. | ||
* @param {object[]} objArray - The JSON data to be converted. | ||
* @param {string[]} headerList - The list of headers for the CSV file. | ||
* @param {string[]} omitProperties - Array of properties to omit. | ||
* @returns {string} - The CSV formatted string. | ||
*/ | ||
function convertJsonToCSV(objArray: object[], headerList: string[], omitProperties: string[]): string { | ||
// Ensure objArray is an object array | ||
const array = typeof objArray !== 'object' ? JSON.parse(objArray as unknown as string) : objArray; | ||
// Create the header row | ||
let csv = headerList.map((header, index) => index === 0 ? `S.No,${header}` : header).join(',') + '\r\n'; | ||
// Iterate through the data and create CSV rows | ||
array.forEach((row: Record<string, any>, rowIndex: number) => { | ||
const csvRow = headerList.map(header => { | ||
if (shouldOmit(header, omitProperties)) { | ||
return ''; | ||
} | ||
return getNestedProperty(row, header); | ||
}).join(','); | ||
csv += `${rowIndex + 1},${csvRow}\r\n`; | ||
}); | ||
return csv; | ||
} | ||
/** | ||
* Determines if a property should be omitted based on the omit list. | ||
* @param {string} property - The property name to check. | ||
* @param {string[]} omitProperties - The list of properties to omit. | ||
* @returns {boolean} - True if the property should be omitted, false otherwise. | ||
*/ | ||
function shouldOmit(property: string, omitProperties: string[]): boolean { | ||
return omitProperties.some(omitProp => { | ||
const regex = new RegExp(`^${omitProp.replace('.', '\\.')}`); | ||
return regex.test(property); | ||
}); | ||
} | ||
/** | ||
* Retrieves a nested property value from an object based on dot notation. | ||
* @param {object} obj - The object to retrieve the property from. | ||
* @param {string} path - The path to the property in dot notation. | ||
* @returns {any} - The value of the nested property, or undefined if not found. | ||
*/ | ||
function getNestedProperty(obj: Record<string, any>, path: string): any { | ||
return path.split('.').reduce((acc, part) => acc && acc[part], obj); | ||
} |
@@ -0,0 +0,0 @@ { |
Sorry, the diff of this file is not supported yet
93779
10
185
124