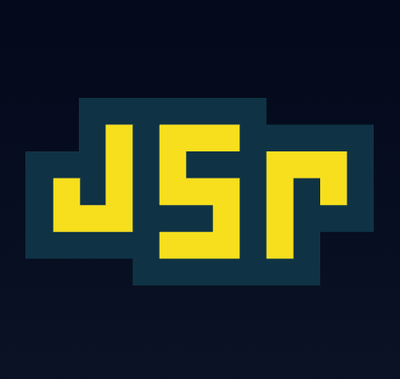
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
csv-stringify
Advanced tools
The csv-stringify npm package is a module that provides the ability to convert arrays or objects into a CSV (Comma-Separated Values) string. It can be used to generate CSV files or strings from JavaScript data structures, supporting both the Node.js stream API and a simple callback-based API.
Stringifying an array of records
This feature allows you to convert an array of arrays (representing records) into a CSV string. Each sub-array is a row in the CSV output.
const stringify = require('csv-stringify');
const records = [['1', '2', '3'], ['a', 'b', 'c']];
stringify(records, (err, output) => {
if (err) throw err;
console.log(output);
});
Stringifying from a stream
This feature demonstrates how to use csv-stringify with Node.js streams. Data can be piped into the stringify function, which then outputs a CSV formatted stream.
const stringify = require('csv-stringify');
const { Readable } = require('stream');
const records = new Readable({
objectMode: true,
read() {}
});
records.push(['1', '2', '3']);
records.push(['a', 'b', 'c']);
records.push(null);
records.pipe(stringify()).pipe(process.stdout);
Stringifying objects with column headers
This feature allows you to convert an array of objects into a CSV string, including column headers as the first row in the output.
const stringify = require('csv-stringify');
const records = [{ id: '1', name: 'John Doe' }, { id: '2', name: 'Jane Doe' }];
stringify(records, { header: true }, (err, output) => {
if (err) throw err;
console.log(output);
});
PapaParse is a robust and powerful CSV (character-separated values) parser with a focus on ease of use and performance. It can parse CSV files or strings, convert them to JSON, and provide many advanced features like auto-detection of delimiters. Compared to csv-stringify, PapaParse offers both parsing and stringifying capabilities, whereas csv-stringify is focused only on stringifying.
fast-csv is an npm package that provides parsing and formatting capabilities for CSV data. It is designed to be fast and flexible, allowing for both stream and callback-based APIs. While csv-stringify is specifically for stringifying JavaScript data into CSV format, fast-csv offers both parsing and formatting, making it a more comprehensive solution for working with CSV data.
Part of the [CSV module][csv_home], this project is a stringifier converting
arrays or objects input into a CSV text. It implements the Node.js
[stream.Transform
API][stream_transform]. It also provides a simple
callback-based API for convenience. It is both extremely easy to use and
powerful. It was first released in 2010 and is used against big data sets by a
large community.
Documentation for the "csv-stringify" package is available here.
csv-generate
, csv-parse
and stream-transform
Refer to the project webpage for an exhaustive list of options and some usage examples.
The module is built on the Node.js Stream API. For the sake of simplify, a simple callback API is also provided. To give you a quick look, here's an example of the callback API:
var stringify = require('csv-stringify');
input = [ [ '1', '2', '3', '4' ], [ 'a', 'b', 'c', 'd' ] ];
stringify(input, function(err, output){
output.should.eql('1,2,3,4\na,b,c,d');
});
Tests are executed with mocha. To install it, simple run npm install
followed by npm test
. It will install mocha and its dependencies in your
project "node_modules" directory and run the test suite. The tests run
against the CoffeeScript source files.
To generate the JavaScript files, run npm run coffee
.
The test suite is run online with Travis against the versions 0.10, 0.11 and 0.12 of Node.js.
FAQs
CSV stringifier implementing the Node.js `stream.Transform` API
The npm package csv-stringify receives a total of 2,973,132 weekly downloads. As such, csv-stringify popularity was classified as popular.
We found that csv-stringify demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.