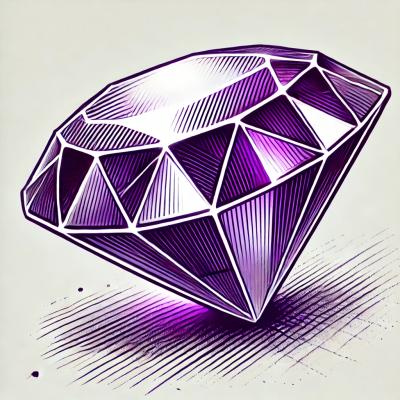
Security News
Highlights from the 2024 Rails Community Survey
A record 2,709 developers participated in the 2024 Ruby on Rails Community Survey, revealing key tools, practices, and trends shaping the Rails ecosystem.
curvy-tabs
Advanced tools
See the demo.
<div class="curvy-tabs-container">
<div style="background-color:lightblue" name="Tab A">
Content for Tab A goes here.
</div>
<div style="background-color:lightgreen" name="Tab B">
Content for Tab B goes here.
</div>
</div>
As npm module:
var CurvyTabs = require('curvy-tab');
From CDN:
<script src="https://joneit.github.io/curvy-tabs/2.0.1/curvy-tabs.min.js">
The following instantiates the controller object, collecting all the content divs into a sub-div, and adds the tab bar (a canvas
element) above it:
var container = document.querySelector('.curvy-tabs-container'); // or whatever
var tabBar = new CurvyTabs(container);
tabBar.paint();
The tabs are named after the content element names.
container
propertyThe element that contains both the tab bar itself (a <canvas>
element) and a <div>
containing the content elements.
contents
propertyThe <div>
containing the content elements.
getTab
methodConvenience method to get a content element by index or name.
For example, to get a reference to the 2nd tab’s content element:
var tab = tabBar.getTab(1); // by index (zero-based, so 1 means 2nd tab)
// or:
var tab = tabBar.getTab('Tab B'); // by name
selected
property and select
methodThe first tab is selected by default.
To programmatically specify some other tab, set selected
to a specific content div, either of the following works:
var tabB = tabBar.contents.children[1];
// or:
var tabB = tabBar.contents.querySelector('[name="Tab B"]');
tabBar.selected = tabToSelect; // but see tabBar.select() method
To select an alternative tab on instantiation:
var tabBar = new CurvyTabs(container, tabB);
Or, use the select
convenience method for setting selected
property by tab index or name:
tabBar.select(1);
// or:
tabBar.select('Tab B');
clear
methodTo "clear" (removes all child elements from) the 2nd tab’s content element:
tabBar.select(1); // by index
// or:
tabBar.select('Tab B'); // by name
To hide the 2nd tab, set 2nd content element’s display
style:
<style>
.curvy-tabs-content > div > *:nth-child(1) { display: none } /* zero-based */
/* or: */
.curvy-tabs-content > div > *[name="Tab B"] { display: none }
</style>
display
style property of the content div:
tabBar.contents.querySelector(1).style.display = 'none'; // default is 'block'
// or:
tabBar.contents.querySelector('[name="Tab B"]').style.display = 'none';
tabBar.paint();
Or, use the hide
, show
, convenience methods to set a tab’s display
property by index or name and call paint
for you:
var indexOrName = 1; // by index
// or:
var indexOrName = 'Tab B'; // by name
// to hide:
tabBar.hide(indexOrName);
tabBar.toggle(indexOrName, false);
// to hide:
tabBar.show(indexOrName);
tabBar.toggle(indexOrName, true);
// to flip:
tabBar.toggle(indexOrName); // hides if visible or shows if hidden
curviness
propertyTo change the curviness of the tab outlines:
tabBar.curviness = 0; // no curves at all (looks exactly like Chrome's tabs)
tabBar.curviness = 0.5; // somewhat flattened curves
tabBar.curviness = 1; // full curviness (default)
minWidth
propertyTabs are sized proportional to their labels. To make all tabs the same width:
tabBar.minWidth = 100; // Tabs whose text exceeds 100 pixels are widened to accommodate
font
propertyTo change the tab font:
tabBar.font = '12pt cursive'; // accepts full CSS font spec
To set the size (i.e., height) of the tabs (for example to accommodate outsized fonts):
CurvyTabs.size = 40;
tabBar.size = 40;
width
and height
propertyThe container must have a width and height. The default is 500 × 500 pixels.
<style>
.curvy-tabs-container { width: 750px; height: 1050px; }
</style>
tabBar.width = 750; // sets both the tab bar width and the container width
tabBar.height = 1050;
css
methodThe tab bar’s background color, border color, and border width affect both the tab bar and content area and can be set as follows:
<style>
.curvy-tabs-container > div {
border: 2x solid red;
background-color: yellow;
}
</style>
css
method (works like jQuery's css
method):
tabBar.css('borderColor', 'red'); // sets border color
tabBar.css('borderColor'); // returns border color
tabBar.css({ borderColor: 'yellow', backgroundColor: 'red' }); // sets both style properties
tabBar.css(['borderColor', 'backgroundColor']); // returns style dictionary
(Note that the tab bar’s background color is only visible through transparent tabs; there is no point in setting this if all your tabs have defined colors.)
contentCss
methodTo set styles on all the content divs at once:
<style>
.curvy-tabs-content > div > * { padding: 3px }
</style>
contentCss
method (also like jQuery's css
method):
tabBar.contentCss('padding', '2px');
tabBar.onclick
If defined as a function, this event handler will be fired on every click of any tab. The event object contains content
(a reference to the content element to be displayed, whose name
attribute is used as the tab label), left
(horizontal pixel location of left edge of tab, and width
(width of tab). For example:
tabBar.onclick = function(event) {
console.log('tab clicked:', event.content.getAttribute('name'));
};
event.preventDefault()
Calling event.preventDefault()
from this handler will prevent the clicked tab from being selected. Therefore, this is a way of disabling all tabs.
tab.onclick
In addition, each tab may also define its own event handler, fired only when that tab is clicked on. For example:
var content = this.tabBar.container.querySelector('.curvy-tabs-content'); // eg, first tab
tabBar.tabs.get(content).onclick = function(event) { ... }; // tabs is a WeakMap
event.preventDefault()
As above, calling event.preventDefault()
from within will prevent the tab from being selected. This is a way of disabling just this specific tab.
event.stopPropagation()
The event will be propagated to the tabBar.onclick
handler (if defined) unless you call event.stopPropagation()
from within.
1.0.0
— Initial version2.0.0
height
is now size
containerHeight
is now height
contents
, contentDivs
, css, and
contentCss`2.0.1
2.1.0
getTab
, select
, and clear
convenience methodsdisplay
propdisplay
prophide
, show
, and toggle
convenience methodsFAQs
Tab bar with fancy tabs
The npm package curvy-tabs receives a total of 1 weekly downloads. As such, curvy-tabs popularity was classified as not popular.
We found that curvy-tabs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A record 2,709 developers participated in the 2024 Ruby on Rails Community Survey, revealing key tools, practices, and trends shaping the Rails ecosystem.
Security News
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.