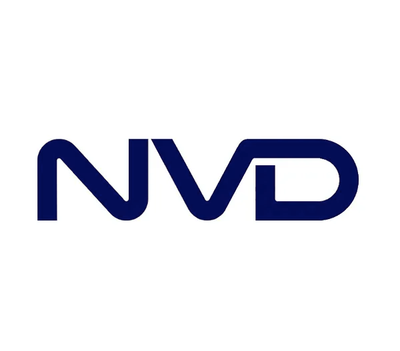
Security News
NIST Misses 2024 Deadline to Clear NVD Backlog
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
cypress-react-unit-test
Advanced tools
A little helper to unit test React components in the open source Cypress.io E2E test runner ALPHA
What is this? This package allows you to use Cypress test runner to unit test your React components with zero effort.
How is this different from Enzyme? It is similar in functionality BUT runs the component in the real browser with full power of Cypress E2E test runner: live GUI, full API, screen recording, CI support, cross-platform.
See issues labeled v2
Requires Node version 8 or above.
npm install --save-dev cypress cypress-react-unit-test
Include this plugin from your project's cypress/support/index.js
require('cypress-react-unit-test/dist/hooks')
Then turn the experimental component support on in your cypress.json
. You can also specify where component spec files are located. For exampled to have them located in src
folder use:
{
"experimentalComponentTesting": true,
"componentFolder": "src"
}
import React from 'react'
import { mount } from 'cypress-react-unit-test'
import { HelloWorld } from './hello-world.jsx'
describe('HelloWorld component', () => {
it('works', () => {
mount(<HelloWorld />)
// now use standard Cypress commands
cy.contains('Hello World!').should('be.visible')
})
})
If you component imports its own style, the style should be applied during the Cypress test. But sometimes you need more power.
You can 3 options to load additional styles:
mount(<Component />, {
style: string, // load inline style CSS
cssFiles: string | string[], // load a single or a list of local CSS files
stylesheets: string | string[] // load external stylesheets
})
You can add individual style to the mounted component by passing its text as an option
it('can be passed as an option', () => {
const style = `
.component-button {
display: inline-flex;
width: 25%;
flex: 1 0 auto;
}
.component-button.orange button {
background-color: #F5923E;
color: white;
}
`
cy.mount(<Button name="Orange" orange />, { style })
cy.get('.orange button').should(
'have.css',
'background-color',
'rgb(245, 146, 62)',
)
})
const cssFiles = 'cypress/integration/Button.css'
cy.mount(<Button name="Orange" orange />, { cssFiles })
See cypress/integration/inject-style-spec.js for more examples.
mount(<Todo todo={todo} />, {
stylesheets: [
'https://cdnjs.cloudflare.com/ajax/libs/bulma/0.7.2/css/bulma.css',
],
})
If your React and React DOM libraries are installed in non-standard paths (think monorepo scenario), you can tell this plugin where to find them. In cypress.json
specify paths like this:
{
"env": {
"cypress-react-unit-test": {
"react": "node_modules/react/umd/react.development.js",
"react-dom": "node_modules/react-dom/umd/react-dom.development.js"
}
}
}
How can we use features that require transpilation? By using @cypress/webpack-preprocessor - see the plugin configuration in cypress/plugins/index.js
If you are using plugins/cra-v3 it instruments the code on the fly using babel-plugin-istanbul
and generates report using dependency cypress-io/code-coverage (included). If you want to disable code coverage instrumentation and reporting, use --env coverage=false
or CYPRESS_coverage=false
or set in your cypress.json
file
{
"env": {
"coverage": false
}
}
If you are using Create-React-App v3 or react-scripts
, and want to reuse the built in webpack before ejecting, this module ships with Cypress preprocessor in plugins folder. From the cypress.json
point at the shipped plugin in the node_modules
.
{
"pluginsFile": "node_modules/cypress-react-unit-test/plugins/cra-v3",
"supportFile": "node_modules/cypress-react-unit-test/support"
}
See example repo bahmutov/try-cra-with-unit-test, typical full config with specs and source files in src
folder (before ejecting the app):
{
"fixturesFolder": false,
"pluginsFile": "node_modules/cypress-react-unit-test/plugins/cra-v3",
"supportFile": "node_modules/cypress-react-unit-test/support",
"testFiles": "**/*.spec.js",
"experimentalComponentTesting": true,
"componentFolder": "src"
}
If you already have a plugins file, you can use a file preprocessor that points at CRA's webpack
// your project's Cypress plugin file
const craFilePreprocessor = require('cypress-react-unit-test/plugins/cra-v3/file-preprocessor')
module.exports = on => {
on('file:preprocessor', craFilePreprocessor())
}
Bonus: re-using the config means if you create your application using create-react-app --typescript
, then TypeScript transpile just works out of the box. See bahmutov/try-cra-app-typescript.
If you have your own webpack config, you can use included plugins file to load it. Here is the configuration using the included plugins file and passing the name of the config file via env
variable in the cypress.json
file
{
"pluginsFile": "node_modules/cypress-react-unit-test/plugins/load-webpack",
"env": {
"webpackFilename": "demo/config/webpack.dev.js"
}
}
Look at the examples in cypress/component folder.
Repo | description |
---|---|
try-cra-with-unit-test | Hello world initialized with CRAv3 |
try-cra-app-typescript | Hello world initialized with CRAv3 --typescript |
react-todo-with-hooks | Modern web application using hooks |
test-redux-examples | Example apps copies from official Redux repo and tested as components |
test-react-hooks-animations | Testing React springs fun blob animation |
test-mdx-example | Example testing MDX components using Cypress |
test-apollo | Component testing an application that uses Apollo GraphQL library |
test-xstate-react | XState component testing using Cypress |
test-react-router-v5 | A few tests of React Router v5 |
test-material-ui | Testing Material UI components: date pickers, lists, autocomplete |
test-d3-react-gauge | Testing React D3 gauges |
To find more examples, see GitHub topic cypress-react-unit-test-example
To get started with this repo, compile the plugin's code and the examples code
npm run transpile
npm run build
npm run cy:open
npx tsc -w
npx cypress open
and select the spec you want to work withlib/index.ts
where all the magic happensUses Percy.io visual diffing service as a GitHub pull request check.
Same feature for unit testing components from other frameworks using Cypress
FAQs
Unit test React components using Cypress
The npm package cypress-react-unit-test receives a total of 1,586 weekly downloads. As such, cypress-react-unit-test popularity was classified as popular.
We found that cypress-react-unit-test demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.