d3-ease
Easing is a method of distorting time to control apparent motion in animation. It is most commonly used for slow-in, slow-out. By easing time, animated transitions are smoother and exhibit more plausible motion.
An easing function takes a single argument: the time t, typically in the normalized range [0,1]. The easing function returns the “eased” time tʹ, typically in the same range [0,1]. (Though note that some easing methods, such as easeElastic, may return eased values somewhat outside the range [0,1].) A good easing function should return 0 if t = 0 and 1 if t = 1. See the easing explorer for a visual demonstration.
These easing functions are largely based on work by Robert Penner.
Installing
If you use NPM, npm install d3-ease
. Otherwise, download the latest release. The released bundle supports AMD, CommonJS, and vanilla environments. Create a custom build using Rollup or your preferred bundler. You can also load directly from d3js.org:
<script src="https://d3js.org/d3-ease.v0.5.min.js"></script>
In a vanilla environment, a d3_ease
global is exported. Try d3-ease in your browser.
API Reference
# d3.easeBind(type[, parameters…])
A convenience function for binding zero or more parameters to the specified easing function type. If no parameters are specified, this function simply returns type. The returned function takes a single argument t and passes any optional parameters to the underlying function type. For example, the following statements are equivalent:
d3.easeBind(d3.easePolyIn, 3)(0.5);
d3.easePolyIn(0.5, 3);
d3.easeBind(d3.easeCubicIn)(0.5);
d3.easeCubicIn(0.5);
# d3.easeLinearIn(t)
# d3.easeLinearOut(t)
# d3.easeLinearInOut(t)
Linear easing; the identity function. Returns t.

# d3.easePolyIn(t[, e])
Polynomial easing; raises t to the specified power e. If e is not specified, it defaults to 3, equivalent to easeCubicIn.
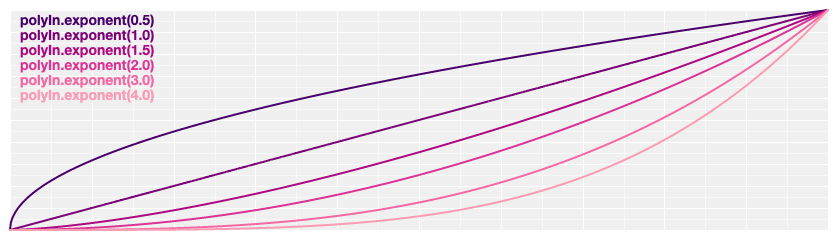
# d3.easePolyOut(t[, e])
Reverse polynomial easing; equivalent to 1 - easePolyIn(1 - t, e). If e is not specified, it defaults to 3, equivalent to easeCubicOut.
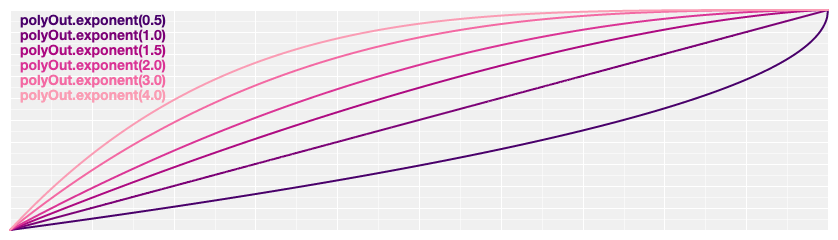
# d3.easePolyInOut(t[, e])
Symmetric polynomial easing; scales easePolyIn for t in [0, 0.5] and easePolyOut for t in [0.5, 1]. If e is not specified, it defaults to 3, equivalent to easeCubicInOut.
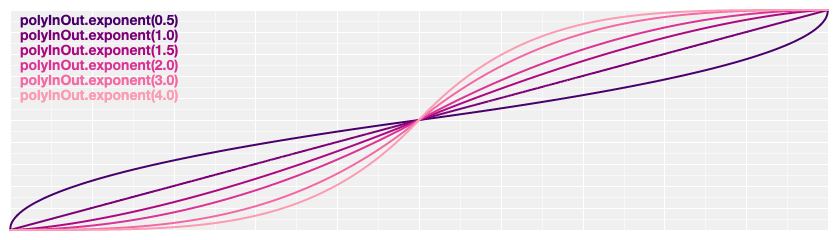
# d3.easeQuadIn(t)
Quadratic easing; equivalent to easePolyIn(t, 2).
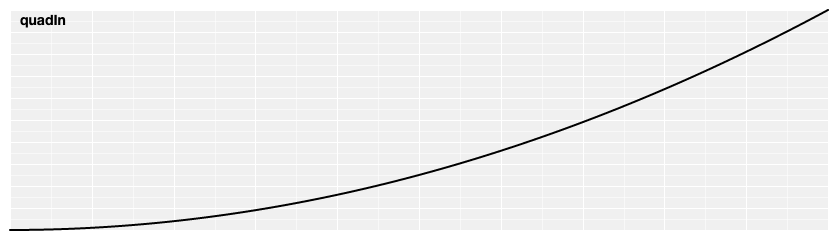
# d3.easeQuadOut(t)
Reverse quadratic easing; equivalent to 1 - easeQuadIn(1 - t). Also equivalent to easePolyOut(t, 2).

# d3.easeQuadInOut(t)
Symmetric quadratic easing; scales easeQuadIn for t in [0, 0.5] and easeQuadOut for t in [0.5, 1]. Also equivalent to easePolyInOut(t, 2).
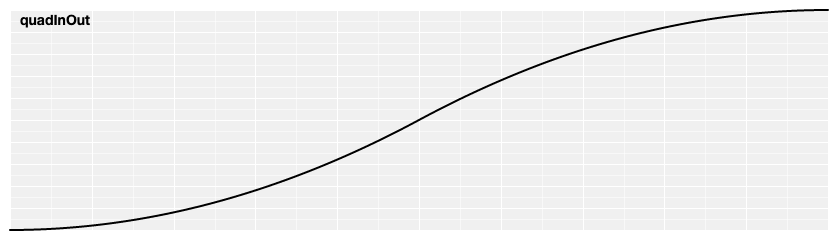
# d3.easeCubicIn(t)
Cubic easing; equivalent to easePolyIn(t, 3).
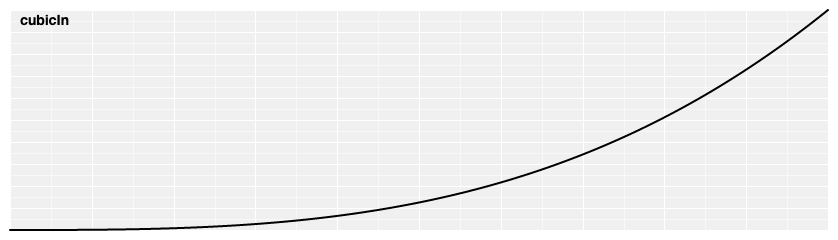
# d3.easeCubicOut(t)
Reverse cubic easing; equivalent to 1 - easeCubicIn(1 - t). Also equivalent to easePolyOut(t, 3).
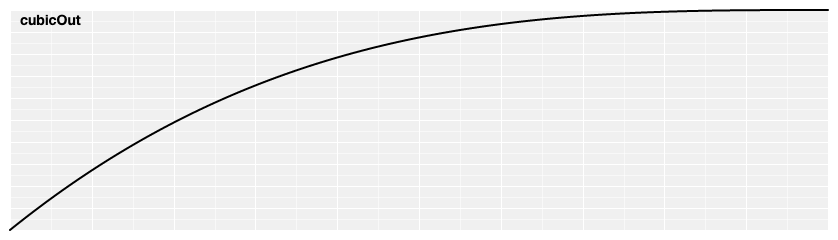
# d3.easeCubicInOut(t)
Symmetric cubic easing; scales easeCubicIn for t in [0, 0.5] and easeCubicOut for t in [0.5, 1]. Also equivalent to easePolyInOut(t, 3).
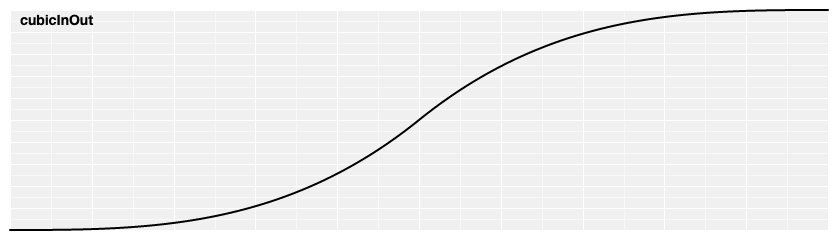
# d3.easeSinIn(t)
Sinusoidal easing; returns sin(t).
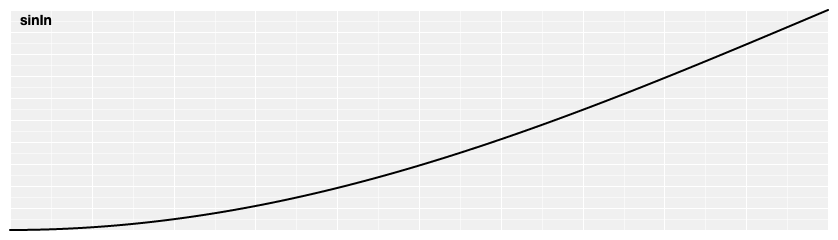
# d3.easeSinOut(t)
Reverse sinusoidal easing; equivalent to 1 - easeSinIn(1 - t).
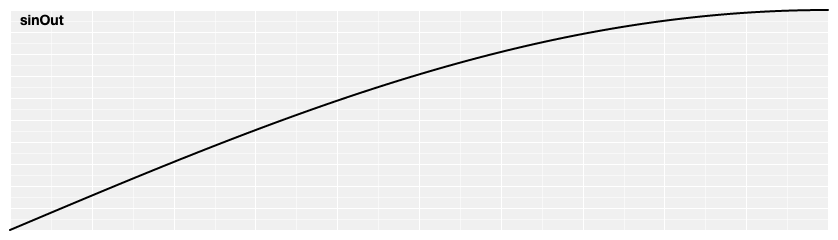
# d3.easeSinInOut(t)
Symmetric sinusoidal easing; scales easeSinIn for t in [0, 0.5] and easeSinOut for t in [0.5, 1].

# d3.easeExpIn(t)
Exponential easing; raises 2 to the power 10 * (t - 1).
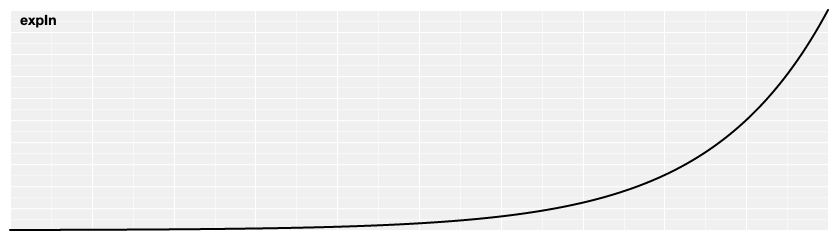
# d3.easeExpOut(t)
Reverse exponential easing; equivalent to 1 - easeExpIn(1 - t).
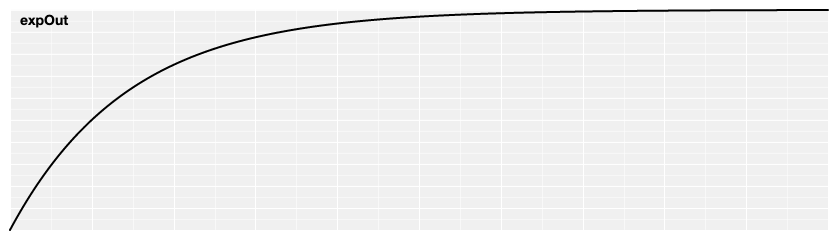
# d3.easeExpInOut(t)
Symmetric exponential easing; scales easeExpIn for t in [0, 0.5] and easeExpOut for t in [0.5, 1].
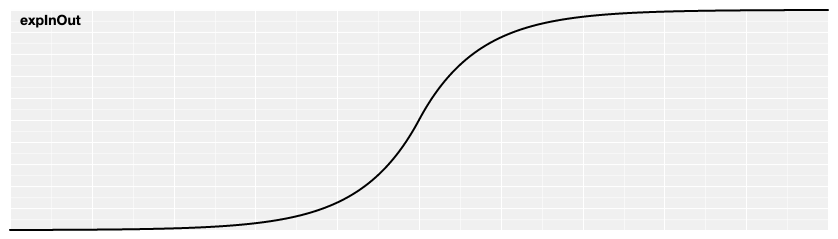
# d3.easeCircleIn(t)
Circular easing.
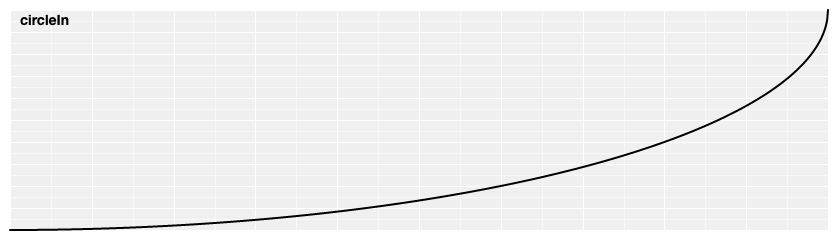
# d3.easeCircleOut(t)
Reverse circular easing; equivalent to 1 - easeCircleIn(1 - t).
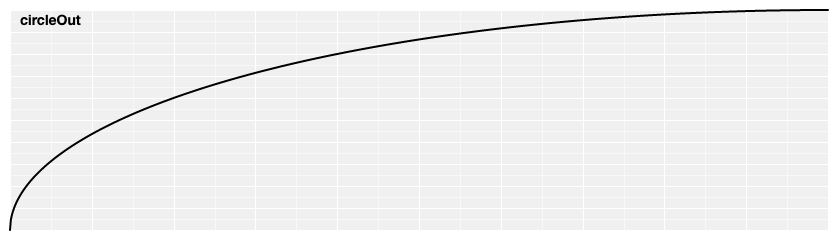
# d3.easeCircleInOut(t)
Symmetric circular easing; scales easeCircleIn for t in [0, 0.5] and easeCircleOut for t in [0.5, 1].
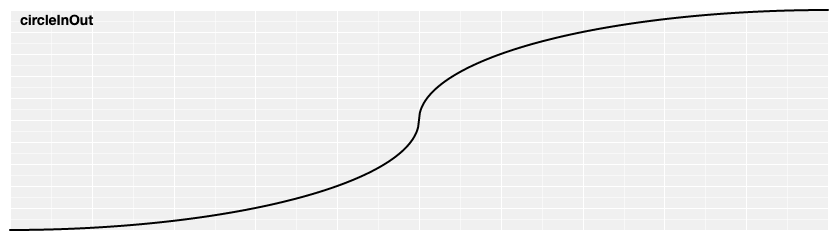
# d3.easeElasticIn(t[, a[, p]])
Elastic easing, like a rubber band. The parameters a and p control the amplitude and period of the oscillation; if not specified, they default to 1 and 0.3, respectively.
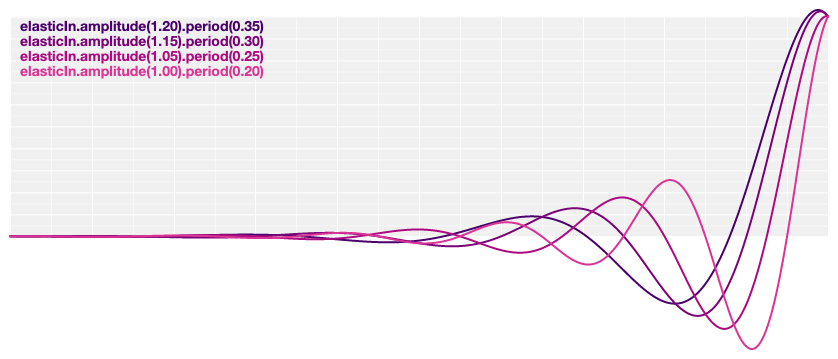
# d3.easeElasticOut(t[, a[, p]])
Reverse elastic easing; equivalent to 1 - easeElasticIn(1 - t).
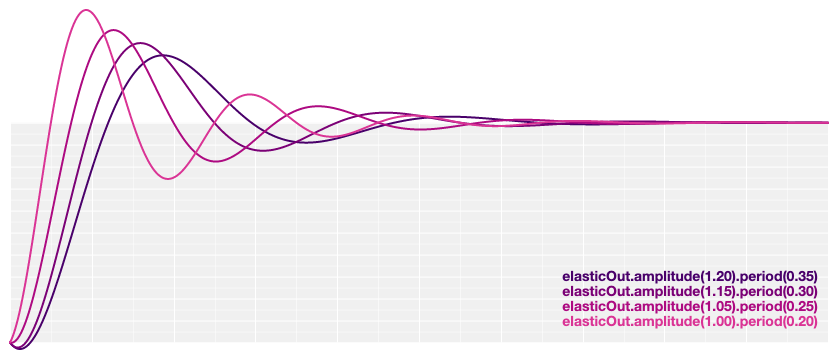
# d3.easeElasticInOut(t[, a[, p]])
Symmetric elastic easing; scales easeElasticIn for t in [0, 0.5] and easeElasticOut for t in [0.5, 1].
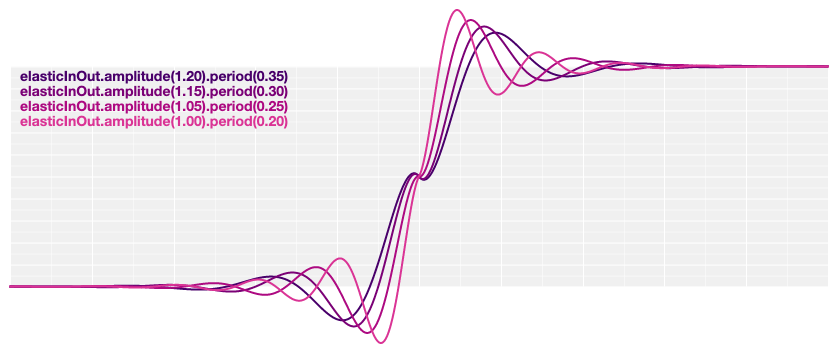
# d3.easeBackIn(t[, s])
Anticipatory easing, like a dancer bending his knees before jumping off the floor. The amount of anticipation (“backing up”) is determined by the parameter s; it not specified, it defaults to 1.70158.
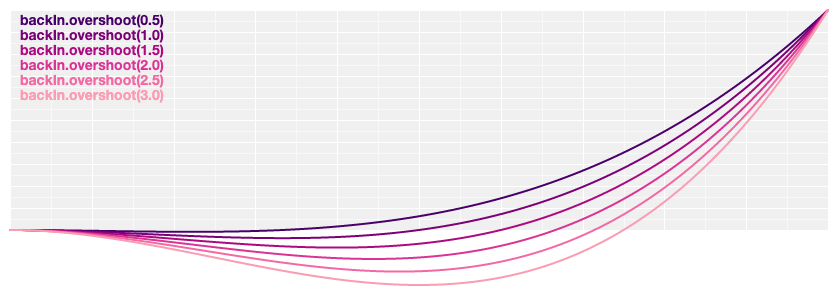
# d3.easeBackOut(t[, s])
Reverse anticipatory easing; equivalent to 1 - easeBackIn(1 - t).
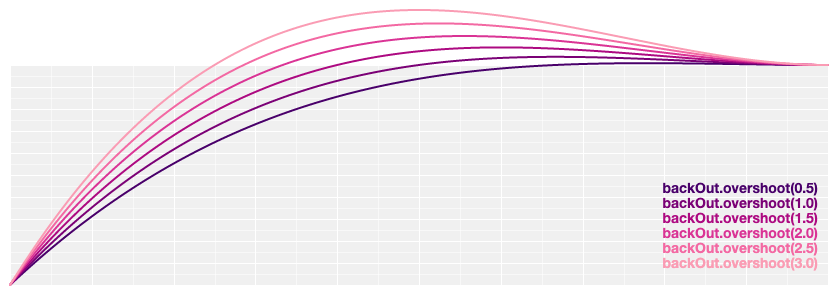
# d3.easeBackInOut(t[, s])
Symmetric anticipatory easing; scales easeBackIn for t in [0, 0.5] and easeBackOut for t in [0.5, 1].
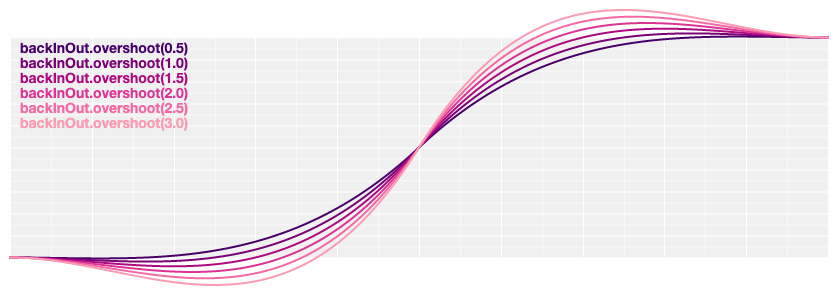
# d3.easeBounceIn(t)
Bounce easing, like a rubber ball.
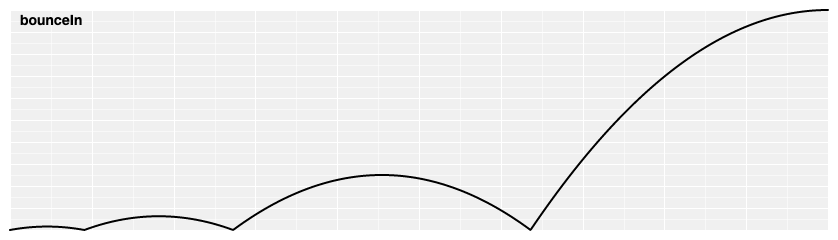
# d3.easeBounceOut(t)
Reverse bounce easing; equivalent to 1 - easeBounceIn(1 - t).
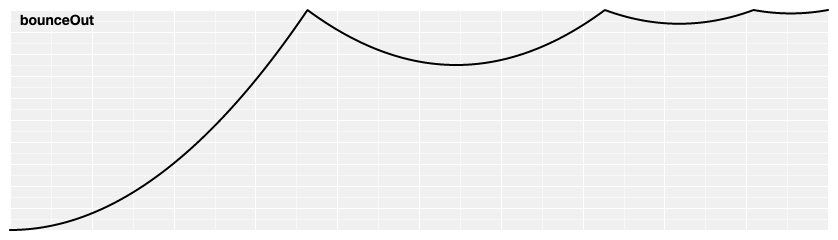
# d3.easeBounceInOut(t)
Symmetric bounce easing; scales easeBounceIn for t in [0, 0.5] and easeBounceOut for t in [0.5, 1].
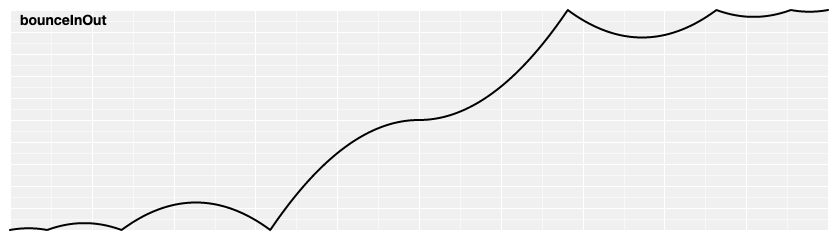