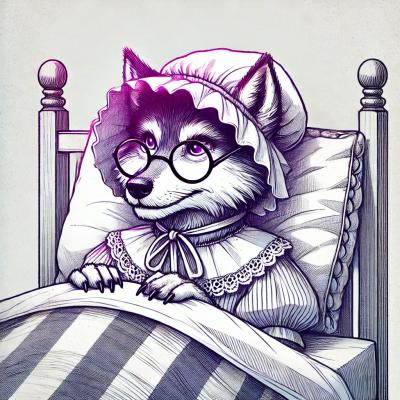
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Micro dom manipulation library. Because jQuery is not needed always.
A lot of this code is taken from http://youmightnotneedjquery.com/
bower install d.js
//Get an array with all .buttons elements
var buttons = d.getAll('.buttons');
//Change css properties
d.css(buttons, {
fontFamily: 'Arial',
color: 'red',
transition: 'all 2s'
});
//Handle events
d.on('click', buttons, function () {
alert('clicked');
});
Returns the first element found:
var container = d.get('.container');
//Use an object to specify the context
var buttonInContainer = d.get({'.button': container});
Returns an array with all elements found:
d.get('.button').forEach(function (el) {
el.classList.add('selected');
});
Returns an array with all siblings of another.
d.getSiblings('li'); //return all siblings
d.getSiblings('li', '.filtered'); //return all siblings with class '.filtered'
Returns if the element matches with the selector:
d.is(document.body, 'h1'); //false
d.is(document.body, 'body'); //true
Attach an event to the elements
Event
function clickAction(e) {
alert('Event ' + e.type);
}
d.on('click', '.button', clickAction);
Delegate an event to the elements
Event
function clickAction(e) {
alert('Event ' + e.type);
}
d.on('click', '.navigation', 'a', clickAction);
Removes an event from the elements
Event
d.off('click', '.button', clickAction);
Trigger an event of the elements
Event
d.trigger('click', '.button');
Removes the elements from the DOM
d.remove('.button');
Set a data-*
attribute. If it's an array or object, is converted to json
d.setData('.button', 'clicked', true);
Get a data-*
attribute. It can detect and convert primitive types like integers, floats and booleans. Json values are automatically parsed.
d.setData('.button', 'clicked'); //true
Insert new elements after other
d.insertAfter('li:last-child', newNodes);
d.insertAfter('li:last-child', '<li>new content</li>');
Insert new elements before other
d.insertBefore('li:first-child', newNodes);
d.insertBefore('li:first-child', '<li>new content</li>');
Insert new elements as first children of other element
d.prepend('ul', newLiNode);
d.prepend('ul', '<li>new content</li>');
Insert new elements as last children of other element
d.append('ul', newLiNode);
d.append('ul', '<li>new content</li>');
Set/get the css properties of the first element. The vendor prefixes are handled automatically.
//Get the value
var color = d.css('.button', 'color');
//Set a new value
d.css('.button', 'color', 'blue');
//Set several values
d.css('.button', {
color: 'red',
backgroundColor: ['blue', 'red', 'green'], //set different values for each element
transform: 'rotate(5deg)' //don't care about vendor prefixes
width: function (el, index) { //use a function to calculate the value for each element
return (100 + (100*index)) + 'px';
}
});
Parses html code. Returns an element or an array of elements
//parse one element
var button = d.parse('<button>Hello</button>');
button.classList.add('active');
//parse a list of elements
var buttons = d.parse('<button>Hello</button><button>World</button>');
buttons.forEach(function (el) {
el.classList.add('active');
});
d.js
allows to create d
instances so you can chain some of these methods. Example:
d('.button')
.css({
color: 'red',
fontFamily: 'Arial'
}).
.on('click', function (e) {
alert('Button clicked');
})
.append('.buttons');
//You can create new elements on the fly:
d('<button>Click me</button>')
.css({
color: 'red',
fontFamily: 'Arial'
})
.on('click', function () {
alert('Hi!');
})
.appendTo('.buttons');
Chainable methods:
Method | Description |
---|---|
.on(event, callback, useCapture) | Attach an event. |
.off(event, callback, useCapture) | Removes an event. |
.delegate(event, target, callback, useCapture) | Delegates an event. |
.trigger(event, data) | Trigger an event |
`.css(props | prop, value)` |
`.insertBefore(content | query)` |
`.insertAfter(content | query)` |
`.prepend(content | query)` |
`.append(content | query)` |
.insertBeforeTo(query) | Insert the element before other element |
.insertAfterTo(query) | Insert the element after other element |
.prependTo(query) | Insert the element as first child of other element |
.appendTo(query) | Insert the element as last child of other element |
1.8.0 - 2017-04-17
d.getData()
and d.setData()
.FAQs
DOM manipulation micro-library
The npm package d_js receives a total of 0 weekly downloads. As such, d_js popularity was classified as not popular.
We found that d_js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.