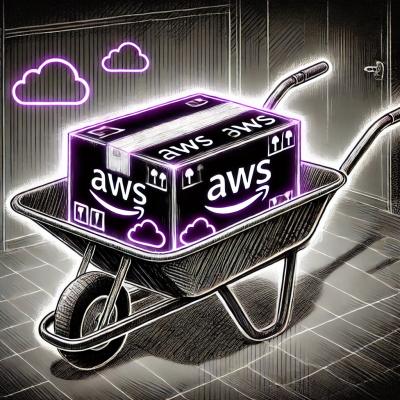
Security News
Research
Malicious Python Package Typosquats Popular 'fabric' SSH Library, Exfiltrates AWS Credentials
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.