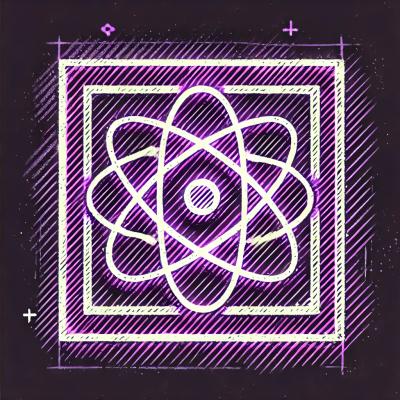
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
data-structures-again
Advanced tools
Light weight javascript data structures library
npm install data-structures-again
const { BST } = require('data-structures-again')
const bst = new BST()
bst.insert(2)
bst.insert(1)
const node = bst.search(1) // { data: 1, left: null, right: null }
bst.delete(1)
const { Stack } = require('data-structures-again')
const stack = new Stack()
stack.push(1)
stack.push(2)
const data = stack.pop() // 2
const top = stack.peek() // 1
const { Queue } = require('data-structures-again')
const queue = new Queue()
queue.enqueue(1)
queue.enqueue(2)
const data = queue.dequeue() // 1
const top = queue.peek() // 2
const { Heap } = require('data-structures-again')
const minHeap = new Heap()
minHeap.push(5)
minHeap.push(2)
minHeap.peek() // 2
const maxHeap = new Heap((a, b) => b - a)
maxHeap.push(4)
maxHeap.push(10)
maxHeap.peek() // 10
const { Graph } = require('data-structures-again')
const graph = new Graph()
/*
a---b
| /
| /
c
*/
graph.addVertex('a')
graph.addVertex('b')
graph.addVertex('c')
graph.addEdge('a', 'c') // add weight using graph.addEdge('a', 'c', 10)
graph.addEdge('c', 'b')
graph.addEdge('a', 'b')
const output = []
graph.dfs('a', vertex => output.push(vertex.name)) // ['a', 'c', 'b']
const { DisjointSet } = require('data-structures-again')
const ds = new DisjointSet()
ds.union('a', 'b')
ds.union('b', 'c')
ds.union('d', 'c')
ds.find('d') // 'a'
ds.isConnected('a', 'd') // true
const { HashSet } = require("data-structures-again");
const set = new HashSet()
set.add(1)
set.add(2)
set.has(1) // true
set.has(2) // true
set.has(3)) // false
const { RedBlackBST } = require('./index')
const tree = new RedBlackBST()
tree.set(1, 'a')
tree.set(2, 'b')
tree.get(1) // 'a'
tree.get(2) // 'b'
const { TwoDTree } = require('./index')
const tree = new TwoDTree()
tree.insert(4, 1)
tree.insert(2.5, 1.5)
tree.insert(2.7, 1.7)
tree.insert(4, 3)
// x1, x2, y1, y2
tree.rangeSearch(2, 3, 1, 2))
/*
[
[2.5, 1.5],
[2.7, 1.7],
[2.6, 1.6]
]
*/
MIT.
FAQs
A Javascript library of simple data structures
The npm package data-structures-again receives a total of 4 weekly downloads. As such, data-structures-again popularity was classified as not popular.
We found that data-structures-again demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.