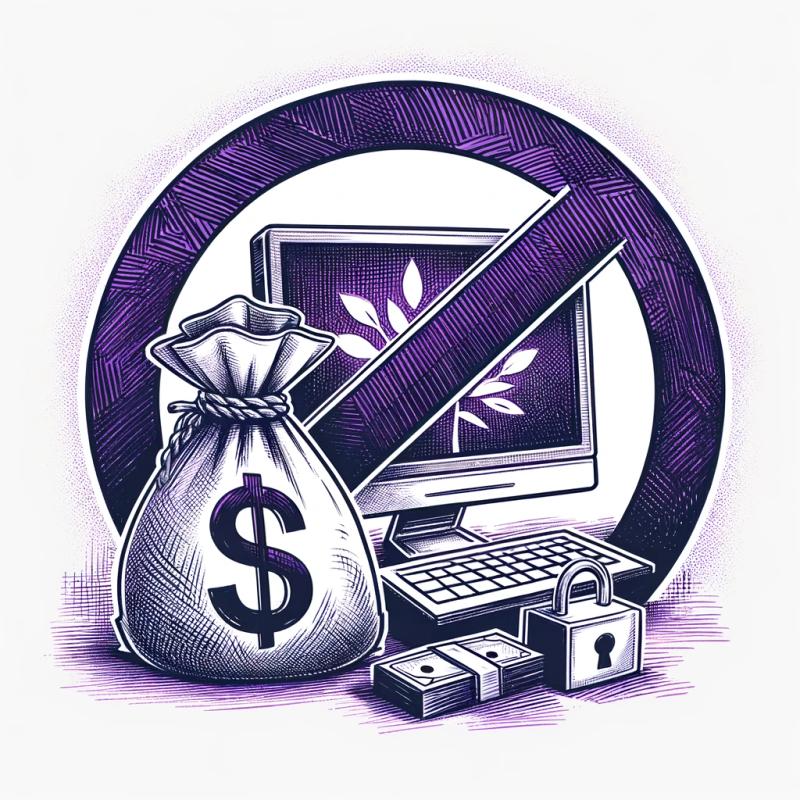
Security News
The Push to Ban Ransom Payments Is Gaining Momentum
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
data-transfer-object
Advanced tools
Changelog
0.3.0 (2021-01-27)
types: skip function types properly (6c1a7d4)
The type of the object returned by toJSON()
was erroneously including function names in its return type. This has been fixed.
import { DataTransferObject, IsString } from 'data-transfer-object';
class MyDto extends DataTransferObject {
@IsString()
key!: string;
}
const input = new MyDto({ key: 'value' });
const data = input.toJSON();
// before 0.3.0
typeof data = {
key: string;
toJSON: never;
validate: never;
validateAsync: never;
}
// 0.3.0 onwards
typeof data = {
key: string;
}
Readme
Data Transfer Object class built on TypeStacks's class-validator
. Allows you to build a DTO class that automatically validates input from outside sources and ensures its shape is correct.
Table of contents
npm i data-transfer-object
# or
yarn add data-transfer-object
UMD builds aren't available yet. Rollup builds are planned for the future.
Create a DTO class by importing DataTransferObject
, extending it with your custom properties, and using the appropriate decorators. This package re-exports all decorators and functions from TypeStack's class-validator
.
import { DataTransferObject, IsString } from 'data-transfer-object';
class MyDto extends DataTransferObject {
// Insert decorated properties here
@IsString()
myString: string;
}
Once your class is defined, you can instantiate it, pass your input into it, and validate it:
const input = new MyDto({ myString: 12 });
const errors = input.validate();
// `errors` will be an array of `ValidationError` objects.
console.log(errors);
// outputs:
[
{
target: { myString: 12 },
value: 12,
property: 'myString',
children: [],
constraints: { isString: 'myString must be a string' },
},
];
Validating it will also silently drop all unknown properties from the input:
const input = new MyDto({ myString: 'a', myOtherString: 'b' });
const errors = input.validate();
console.log(errors); // []
console.log(input); // { myString: 'a' }
Take a look at class-validator
's documentation to get information on all available validators and validation options.
Note that when using TypeScript with "strict": true
, you must use non-null assertions (!:
) when declaring class properties. Also, experimentalDecorators
and emitDecoratorMetadata
must be set to true
in your tsconfig.json
.
The most up-to-date documentation for all exported items from this package is automatically generated from code and available at https://danielegarciav.github.io/data-transfer-object/.
In the following example, we have an Express application that lets us sign up users. In order to validate input from the web app client, we create a data transfer object representing the input data, and attach our desired validators:
// input-dtos.ts
import { DataTransferObject, IsString, Length, MinLength } from 'data-transfer-object';
export class UserSignupInput extends DataTransferObject {
@IsString()
@Length(2, 36, {
message: 'username must be 2-36 characters long',
})
username!: string;
@IsString()
@MinLength(8, {
message: 'password must be at least 8 characters long',
})
password!: string;
}
The Express request handler in our example:
// signup-controller.ts
import { Request, Response } from 'express';
import { User } from './models';
import { UserSignupInput } from './input-dtos';
export async function signup(req: Request, res: Response): {
// Construct a new DTO instance passing starting state as argument.
const input = new UserSignupInput(req.body);
// Run validation. Only synchronous validators are run by default, which covers most use cases.
const errors = input.validate();
// You may also use `.validateAsync()` to run both sync + async validators.
const asyncErrors = await input.validateAsync();
// Result will be an array of `ValidationError[]`
if (errors.length) {
// Handle validation errors here, for example:
return res.status(400).json(errors);
}
// Your input is guaranteed to be validated at this point.
await User.register(input);
// Use `.toJSON()` to get a plain object with just your data.
// `.toJSON()` is automatically called when a DTO instance is stringified.
const data = input.toJSON();
typeof input.validate === 'function';
typeof data.validate === 'undefined';
return res.status(200).json({ success: true });
}
Check package.json to find scripts related to installing dependencies, building, testing, linting and generating documentation.
MIT
FAQs
Data Transfer Object class built on `class-validator`.
We found that data-transfer-object demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
Application Security
New SEC disclosure rules aim to enforce timely cyber incident reporting, but fear of job loss and inadequate resources lead to significant underreporting.
Security News
The Python Software Foundation has secured a 5-year sponsorship from Fastly that supports PSF's activities and events, most notably the security and reliability of the Python Package Index (PyPI).