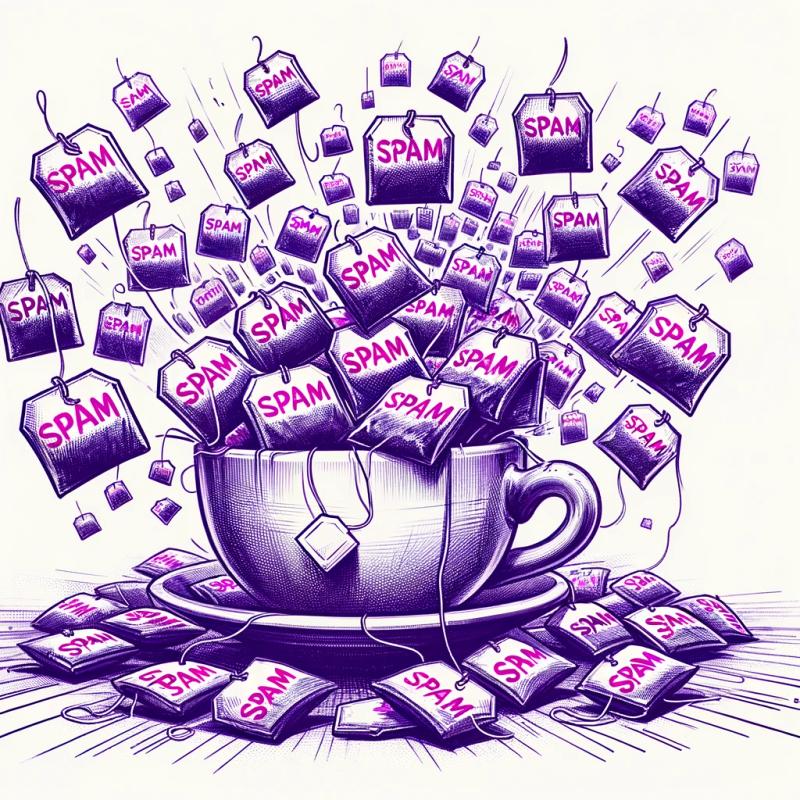
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
datagovsg-plottable-charts
Advanced tools
Readme
Generating a chart is easy, making it looks beautiful requires much more effort. Numerous charting libraries have been written to solves the basic problem of converting data to chart objects. Regardless of the library you choose, out-of-the-box defaults hardly produces the look you want.
Charts on Data.gov.sg are rendered using the Plottable library. Based on D3, it is highly flexible and gives you many low level controls to fine-tune every single detail. However, this power comes at the price of additional configurations. We want to abstract away these configurations by creating wrappers that pre-apply all the styles we want on our component. That's why we created this library.
npm install --save datagovsg-plottable-charts
<!-- html -->
<link rel="stylesheet" href="lib/plottable.css">
<link rel="stylesheet" href="lib/datagovsg-charts.css">
<!-- ... -->
<script src="lib/d3.min.js"></script>
<script src="lib/plottable.min.js"></script>
/* js */
import {SimplePie} from 'datagovsg-plottable-charts'
// Instantiate the chart component
const pie = new SimplePie(props)
// Mount component
pie.mount(document.getElementById('ctn'))
// Update chart
pie.update(newProps)
import {
highlightOnHover,
setupOuterLabel
} from 'datagovsg-plottable-charts/dist/plugins'
highlightOnHover(pie)
setupOuterLabel(pie)
<!-- html -->
<script src="lib/datagovsg-charts.min.js"></script>
/* js */
const {SimplePie, plugins} = window.DatagovsgCharts
const {highlightOnHover, setupOuterLabel} = plugins
const pie = new SimplePie(props)
highlightOnHover(pie)
setupOuterLabel(pie)
import {DatagovsgLine} from 'datagovsg-plottable-charts'
import PivotTable, {
filterItems,
filterGroups,
groupItems,
aggregate
} from 'datagovsg-plottable-charts/dist/PivotTable'
const pivotTable = new PivotTable(data)
pivotTable.push(
filterItems('income', {type: 'exclude', values: ['-', 'na']}),
groupItems('gender'),
filterGroups('gender', {type: 'exclude', values: ['Total']})
aggregate('year', 'income')
)
const processedData = pivotTable.transform()
const series = processedData.map(g => ({
label: g._group.gender,
series: g._summaries[0].series
}))
const chart = new DatagovsgLine({data: series})
chart.mount(document.getElementById('chart'))
Original data
year | gender | income |
---|---|---|
2006 | Total | 2042 |
2016 | Total | 3250 |
2017 | Total | - |
2006 | Male | 2213 |
2016 | Male | 3500 |
2006 | Female | 1875 |
2016 | Female | 2979 |
Transform into custom data structure
pivotTable.transform()
// returns
[
{
_group: {},
_items: [
{year: 2006, gender: 'Total', income: 2042},
{year: 2016, gender: 'Total', income: 3250},
{year: 2016, gender: 'Total', income: '-'},
{year: 2006, gender: 'Male', income: 2213},
{year: 2016, gender: 'Male', income: 3500},
{year: 2006, gender: 'Female', income: 1875},
{year: 2016, gender: 'Female', income: 2979}
],
_summaries: []
}
]
filterItems( )
pivotTable.push(
filterItems('income', {type: 'exclude', values: ['-', 'na']})
)
pivotTable.transform()
// returns
[
{
_group: {},
_items: [
{year: 2006, gender: 'Total', income: 2042},
{year: 2016, gender: 'Total', income: 3250},
{year: 2006, gender: 'Male', income: 2213},
{year: 2016, gender: 'Male', income: 3500},
{year: 2006, gender: 'Female', income: 1875},
{year: 2016, gender: 'Female', income: 2979}
],
_summaries: []
}
]
groupItems( )
pivotTable.push(
filterItems('income', {type: 'exclude', values: ['Total']}),
groupItems('gender')
)
pivotTable.transform()
// returns
[
{
_group: {gender: 'Total'},
_items: [
{year: 2006, gender: 'Total', income: 2042},
{year: 2016, gender: 'Total', income: 3250},
],
_summaries: []
},
{
_group: {gender: 'Male'},
_items: [
{year: 2006, gender: 'Male', income: 2213},
{year: 2016, gender: 'Male', income: 3500},
],
_summaries: []
},
{
_group: {gender: 'Female'},
_items: [
{year: 2006, gender: 'Female', income: 1875},
{year: 2016, gender: 'Female', income: 2979}
],
_summaries: []
}
]
filterGroups( )
pivotTable.push(
filterItems('income', {type: 'exclude', values: ['-', 'na']}),
groupItems('gender'),
filterGroups('gender', {type: 'exclude', values: ['Total']})
)
pivotTable.transform()
// returns
[
{
_group: {gender: 'Male'},
_items: [
{year: 2006, gender: 'Male', income: 2213},
{year: 2016, gender: 'Male', income: 3500},
],
_summaries: []
},
{
_group: {gender: 'Female'},
_items: [
{year: 2006, gender: 'Female', income: 1875},
{year: 2016, gender: 'Female', income: 2979}
],
_summaries: []
}
]
aggregate( )
pivotTable.push(
filterItems('income', {type: 'exclude', values: ['-', 'na']}),
groupItems('gender'),
filterGroups('gender', {type: 'exclude', values: ['Total']})
aggregate('year', 'income')
)
pivotTable.transform()
// returns
[
{
_group: {gender: 'Male'},
_items: [
{year: 2006, gender: 'Male', income: 2213},
{year: 2016, gender: 'Male', income: 3500},
],
_summaries: [
{
labelField: 'year',
valueField: 'income',
series: [
{label: 2006, value: 2213},
{label: 2016, value: 3500}
]
}
]
},
{
_group: {gender: 'Female'},
_items: [
{year: 2006, gender: 'Female', income: 1875},
{year: 2016, gender: 'Female', income: 2979}
],
_summaries: [
{
labelField: 'year',
valueField: 'income',
series: [
{label: 2006, value: 1875},
{label: 2016, value: 2979}
]
}
]
}
]
<!-- html -->
<script src="lib/pivot-table.min.js"></script>
/* js */
const PivotTable = window.PivotTable
const {filterItems, groupItems, filterGroups, aggregate} = PivotTable
cd
to the cloned reponpm install
"main": "src/index.js"
sudo npm link
cd
to your working directorynpm link datagovsg-plottable-charts
FAQs
Reusable Plottable chart components
The npm package datagovsg-plottable-charts receives a total of 45 weekly downloads. As such, datagovsg-plottable-charts popularity was classified as not popular.
We found that datagovsg-plottable-charts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.