datastore-fs
Advanced tools
Comparing version 8.0.0 to 9.0.0
@@ -0,1 +1,12 @@ | ||
import { Key, KeyQuery, Pair, Query } from 'interface-datastore'; | ||
import { BaseDatastore } from 'datastore-core'; | ||
import type { AwaitIterable } from 'interface-store'; | ||
export interface FsDatastoreInit { | ||
createIfMissing?: boolean; | ||
errorIfExists?: boolean; | ||
extension?: string; | ||
putManyConcurrency?: number; | ||
getManyConcurrency?: number; | ||
deleteManyConcurrency?: number; | ||
} | ||
/** | ||
@@ -6,121 +17,47 @@ * A datastore backed by the file system. | ||
* to the file system as is. | ||
* | ||
* @implements {Datastore} | ||
*/ | ||
export class FsDatastore extends BaseDatastore implements Datastore { | ||
/** | ||
* @param {string} location | ||
* @param {{ createIfMissing?: boolean, errorIfExists?: boolean, extension?: string, putManyConcurrency?: number } | undefined} [opts] | ||
*/ | ||
constructor(location: string, opts?: { | ||
createIfMissing?: boolean; | ||
errorIfExists?: boolean; | ||
extension?: string; | ||
putManyConcurrency?: number; | ||
} | undefined); | ||
export declare class FsDatastore extends BaseDatastore { | ||
path: string; | ||
opts: { | ||
createIfMissing: boolean; | ||
errorIfExists: boolean; | ||
extension: string; | ||
deleteManyConcurrency: number; | ||
getManyConcurrency: number; | ||
putManyConcurrency: number; | ||
} & { | ||
createIfMissing?: boolean | undefined; | ||
errorIfExists?: boolean | undefined; | ||
extension?: string | undefined; | ||
putManyConcurrency?: number | undefined; | ||
}; | ||
private readonly createIfMissing; | ||
private readonly errorIfExists; | ||
private readonly extension; | ||
private readonly deleteManyConcurrency; | ||
private readonly getManyConcurrency; | ||
private readonly putManyConcurrency; | ||
constructor(location: string, init?: FsDatastoreInit); | ||
open(): Promise<void>; | ||
close(): Promise<void>; | ||
/** | ||
* Calculate the directory and file name for a given key. | ||
* | ||
* @private | ||
* @param {Key} key | ||
* @returns {{dir:string, file:string}} | ||
*/ | ||
private _encode; | ||
_encode(key: Key): { | ||
dir: string; | ||
file: string; | ||
}; | ||
/** | ||
* Calculate the original key, given the file name. | ||
* | ||
* @private | ||
* @param {string} file | ||
* @returns {Key} | ||
*/ | ||
private _decode; | ||
_decode(file: string): Key; | ||
/** | ||
* Write to the file system without extension. | ||
* | ||
* @param {Key} key | ||
* @param {Uint8Array} val | ||
* @returns {Promise<void>} | ||
*/ | ||
putRaw(key: Key, val: Uint8Array): Promise<void>; | ||
/** | ||
* Store the given value under the key | ||
* | ||
* @param {Key} key | ||
* @param {Uint8Array} val | ||
* @returns {Promise<void>} | ||
*/ | ||
put(key: Key, val: Uint8Array): Promise<void>; | ||
/** | ||
* @param {AwaitIterable<Pair>} source | ||
* @returns {AsyncIterable<Pair>} | ||
*/ | ||
putMany(source: AwaitIterable<Pair>): AsyncIterable<Pair>; | ||
/** | ||
* Read from the file system without extension. | ||
* | ||
* @param {Key} key | ||
* @returns {Promise<Uint8Array>} | ||
* Read from the file system | ||
*/ | ||
getRaw(key: Key): Promise<Uint8Array>; | ||
/** | ||
* Read from the file system. | ||
* | ||
* @param {Key} key | ||
* @returns {Promise<Uint8Array>} | ||
*/ | ||
get(key: Key): Promise<Uint8Array>; | ||
/** | ||
* @param {AwaitIterable<Key>} source | ||
* @returns {AsyncIterable<Uint8Array>} | ||
*/ | ||
getMany(source: AwaitIterable<Key>): AsyncIterable<Uint8Array>; | ||
/** | ||
* @param {AwaitIterable<Key>} source | ||
* @returns {AsyncIterable<Key>} | ||
*/ | ||
deleteMany(source: AwaitIterable<Key>): AsyncIterable<Key>; | ||
/** | ||
* Check for the existence of the given key. | ||
* | ||
* @param {Key} key | ||
* @returns {Promise<boolean>} | ||
* Check for the existence of the given key | ||
*/ | ||
has(key: Key): Promise<boolean>; | ||
/** | ||
* Delete the record under the given key. | ||
* | ||
* @param {Key} key | ||
* @returns {Promise<void>} | ||
* Delete the record under the given key | ||
*/ | ||
delete(key: Key): Promise<void>; | ||
/** | ||
* @param {Query} q | ||
*/ | ||
_all(q: Query): AsyncGenerator<import("interface-datastore").Pair, void, unknown>; | ||
/** | ||
* @param {KeyQuery} q | ||
*/ | ||
_allKeys(q: KeyQuery): AsyncGenerator<Key, void, undefined>; | ||
_all(q: Query): AsyncIterable<Pair>; | ||
_allKeys(q: KeyQuery): AsyncIterable<Key>; | ||
} | ||
export type Datastore = import('interface-datastore').Datastore; | ||
export type Pair = import('interface-datastore').Pair; | ||
export type Query = import('interface-datastore').Query; | ||
export type KeyQuery = import('interface-datastore').KeyQuery; | ||
export type AwaitIterable<TEntry> = import('interface-store').AwaitIterable<TEntry>; | ||
import { BaseDatastore } from "datastore-core"; | ||
import { Key } from "interface-datastore"; | ||
//# sourceMappingURL=index.d.ts.map |
{ | ||
"name": "datastore-fs", | ||
"version": "8.0.0", | ||
"version": "9.0.0", | ||
"description": "Datastore implementation with file system backend", | ||
@@ -28,18 +28,2 @@ "author": "Friedel Ziegelmayer<dignifiedquire@gmail.com>", | ||
"types": "./dist/src/index.d.ts", | ||
"typesVersions": { | ||
"*": { | ||
"*": [ | ||
"*", | ||
"dist/*", | ||
"dist/src/*", | ||
"dist/src/*/index" | ||
], | ||
"src/*": [ | ||
"*", | ||
"dist/*", | ||
"dist/src/*", | ||
"dist/src/*/index" | ||
] | ||
} | ||
}, | ||
"files": [ | ||
@@ -54,3 +38,3 @@ "src", | ||
"types": "./dist/src/index.d.ts", | ||
"import": "./src/index.js" | ||
"import": "./dist/src/index.js" | ||
} | ||
@@ -152,33 +136,26 @@ }, | ||
"lint": "aegir lint", | ||
"build": "aegir build", | ||
"build": "aegir build --bundle false", | ||
"release": "aegir release", | ||
"test": "aegir test", | ||
"test": "aegir test -t node -t electron-main", | ||
"test:node": "aegir test -t node", | ||
"dep-check": "aegir dep-check -i rimraf" | ||
"test:electron-main": "aegir test -t electron-main", | ||
"dep-check": "aegir dep-check", | ||
"docs": "aegir docs" | ||
}, | ||
"dependencies": { | ||
"datastore-core": "^8.0.1", | ||
"datastore-core": "^9.0.1", | ||
"fast-write-atomic": "^0.2.0", | ||
"interface-datastore": "^7.0.0", | ||
"it-glob": "^1.0.1", | ||
"it-map": "^1.0.5", | ||
"it-parallel-batch": "^1.0.9", | ||
"mkdirp": "^1.0.4" | ||
"interface-datastore": "^8.0.0", | ||
"interface-store": "^4.0.0", | ||
"it-glob": "^2.0.1", | ||
"it-map": "^2.0.1", | ||
"it-parallel-batch": "^2.0.1" | ||
}, | ||
"devDependencies": { | ||
"@types/mkdirp": "^1.0.2", | ||
"@types/rimraf": "^3.0.2", | ||
"aegir": "^37.5.0", | ||
"async-iterator-all": "^1.0.0", | ||
"detect-node": "^2.0.4", | ||
"interface-datastore-tests": "^3.0.0", | ||
"ipfs-utils": "^9.0.4", | ||
"memdown": "^6.0.0", | ||
"rimraf": "^3.0.2" | ||
}, | ||
"browser": { | ||
"fs": false, | ||
"path": false, | ||
"util": false | ||
"@types/mkdirp": "^2.0.0", | ||
"@types/rimraf": "^4.0.5", | ||
"aegir": "^38.1.7", | ||
"interface-datastore-tests": "^4.0.0", | ||
"ipfs-utils": "^9.0.4" | ||
} | ||
} |
# datastore-fs <!-- omit in toc --> | ||
[](http://ipfs.io) | ||
[](http://webchat.freenode.net/?channels=%23ipfs) | ||
[](https://discord.gg/ipfs) | ||
[](https://ipfs.tech) | ||
[](https://discuss.ipfs.tech) | ||
[](https://codecov.io/gh/ipfs/js-datastore-fs) | ||
[](https://github.com/ipfs/js-datastore-fs/actions/workflows/js-test-and-release.yml) | ||
[](https://github.com/ipfs/js-datastore-fs/actions/workflows/js-test-and-release.yml?query=branch%3Amaster) | ||
@@ -15,5 +14,5 @@ > Datastore implementation with file system backend | ||
- [Usage](#usage) | ||
- [API Docs](#api-docs) | ||
- [License](#license) | ||
- [Contribute](#contribute) | ||
- [License](#license) | ||
- [Contribute](#contribute-1) | ||
@@ -34,10 +33,6 @@ ## Install | ||
## Contribute | ||
## API Docs | ||
Feel free to join in. All welcome. Open an [issue](https://github.com/ipfs/js-datastore-fs/issues)! | ||
- <https://ipfs.github.io/js-datastore-fs> | ||
This repository falls under the IPFS [Code of Conduct](https://github.com/ipfs/community/blob/master/code-of-conduct.md). | ||
[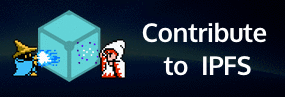](https://github.com/ipfs/community/blob/master/CONTRIBUTING.md) | ||
## License | ||
@@ -52,6 +47,10 @@ | ||
Feel free to join in. All welcome. Open an [issue](https://github.com/ipfs/js-ipfs-unixfs-importer/issues)! | ||
Contributions welcome! Please check out [the issues](https://github.com/ipfs/js-datastore-fs/issues). | ||
This repository falls under the IPFS [Code of Conduct](https://github.com/ipfs/community/blob/master/code-of-conduct.md). | ||
Also see our [contributing document](https://github.com/ipfs/community/blob/master/CONTRIBUTING_JS.md) for more information on how we work, and about contributing in general. | ||
Please be aware that all interactions related to this repo are subject to the IPFS [Code of Conduct](https://github.com/ipfs/community/blob/master/code-of-conduct.md). | ||
Unless you explicitly state otherwise, any contribution intentionally submitted for inclusion in the work by you, as defined in the Apache-2.0 license, shall be dual licensed as above, without any additional terms or conditions. | ||
[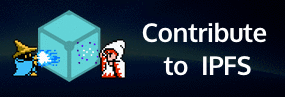](https://github.com/ipfs/community/blob/master/CONTRIBUTING.md) |
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
New author
Supply chain riskA new npm collaborator published a version of the package for the first time. New collaborators are usually benign additions to a project, but do indicate a change to the security surface area of a package.
Found 1 instance in 1 package
Filesystem access
Supply chain riskAccesses the file system, and could potentially read sensitive data.
Found 1 instance in 1 package
5
9
0
1
29709
525
54
+ Addedinterface-store@^4.0.0
+ Added@libp2p/interface@1.7.0(transitive)
+ Added@libp2p/logger@4.0.20(transitive)
+ Addedbrace-expansion@2.0.1(transitive)
+ Addeddatastore-core@9.2.9(transitive)
+ Addedinterface-store@4.1.05.1.8(transitive)
+ Addedit-all@3.0.6(transitive)
+ Addedit-batch@2.0.1(transitive)
+ Addedit-drain@3.0.7(transitive)
+ Addedit-filter@3.1.1(transitive)
+ Addedit-glob@2.0.7(transitive)
+ Addedit-map@3.1.1(transitive)
+ Addedit-merge@3.0.5(transitive)
+ Addedit-parallel-batch@2.0.1(transitive)
+ Addedit-peekable@3.0.5(transitive)
+ Addedit-pipe@3.0.1(transitive)
+ Addedit-sort@3.0.6(transitive)
+ Addedit-stream-types@2.0.2(transitive)
+ Addedit-take@3.0.6(transitive)
+ Addedminimatch@9.0.5(transitive)
+ Addedms@3.0.0-canary.1(transitive)
+ Addedsupports-color@9.4.0(transitive)
+ Addedweald@1.0.4(transitive)
- Removedmkdirp@^1.0.4
- Removed@libp2p/interface-peer-id@2.0.2(transitive)
- Removed@libp2p/logger@2.1.1(transitive)
- Removed@types/minimatch@3.0.5(transitive)
- Removedbrace-expansion@1.1.11(transitive)
- Removedconcat-map@0.0.1(transitive)
- Removeddatastore-core@8.0.4(transitive)
- Removeddebug@4.3.7(transitive)
- Removedinterface-datastore@7.0.4(transitive)
- Removedinterface-store@3.0.4(transitive)
- Removedit-all@2.0.1(transitive)
- Removedit-batch@1.0.9(transitive)
- Removedit-drain@2.0.1(transitive)
- Removedit-filter@2.0.2(transitive)
- Removedit-glob@1.0.2(transitive)
- Removedit-map@1.0.6(transitive)
- Removedit-merge@2.0.1(transitive)
- Removedit-parallel-batch@1.0.11(transitive)
- Removedit-pipe@2.0.5(transitive)
- Removedit-stream-types@1.0.5(transitive)
- Removedit-take@2.0.1(transitive)
- Removedminimatch@3.1.2(transitive)
- Removedmkdirp@1.0.4(transitive)
- Removedms@2.1.3(transitive)
- Removedmultiformats@11.0.212.1.3(transitive)
- Removednanoid@4.0.2(transitive)
- Removeduint8arrays@4.0.10(transitive)
Updateddatastore-core@^9.0.1
Updatedinterface-datastore@^8.0.0
Updatedit-glob@^2.0.1
Updatedit-map@^2.0.1
Updatedit-parallel-batch@^2.0.1