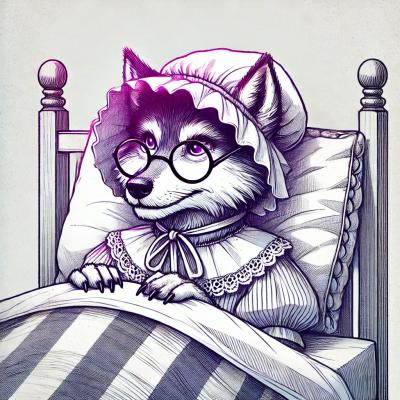
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
date-and-time
Advanced tools
The date-and-time npm package is a lightweight library for date and time manipulation in JavaScript. It provides a variety of functions for parsing, formatting, adding, subtracting, and comparing dates and times.
Formatting Dates
This feature allows you to format dates into various string representations. The format method takes a date object and a format string as arguments.
const date = new Date(2023, 9, 10);
const dateAndTime = require('date-and-time');
const formattedDate = dateAndTime.format(date, 'YYYY/MM/DD HH:mm:ss');
console.log(formattedDate); // Output: 2023/10/10 00:00:00
Parsing Dates
This feature allows you to parse date strings into JavaScript Date objects. The parse method takes a date string and a format string as arguments.
const dateAndTime = require('date-and-time');
const dateString = '2023/10/10 00:00:00';
const parsedDate = dateAndTime.parse(dateString, 'YYYY/MM/DD HH:mm:ss');
console.log(parsedDate); // Output: Tue Oct 10 2023 00:00:00 GMT+0000 (Coordinated Universal Time)
Adding and Subtracting Time
This feature allows you to add or subtract time units (days, months, years, etc.) from a date. The addDays and addMonths methods are used in this example.
const dateAndTime = require('date-and-time');
let date = new Date(2023, 9, 10);
date = dateAndTime.addDays(date, 5);
console.log(date); // Output: Sun Oct 15 2023 00:00:00 GMT+0000 (Coordinated Universal Time)
date = dateAndTime.addMonths(date, -1);
console.log(date); // Output: Fri Sep 15 2023 00:00:00 GMT+0000 (Coordinated Universal Time)
Comparing Dates
This feature allows you to compare dates. The isSameDay method checks if two dates fall on the same day.
const dateAndTime = require('date-and-time');
const date1 = new Date(2023, 9, 10);
const date2 = new Date(2023, 9, 15);
const isSameDay = dateAndTime.isSameDay(date1, date2);
console.log(isSameDay); // Output: false
Moment.js is a widely-used library for date and time manipulation. It offers extensive functionality for parsing, validating, manipulating, and formatting dates. Compared to date-and-time, Moment.js is more feature-rich but also larger in size.
date-fns is a modern JavaScript date utility library that provides a comprehensive set of functions for date manipulation. It is modular, allowing you to import only the functions you need, making it more lightweight compared to Moment.js. date-fns is similar to date-and-time in terms of functionality but offers a more functional programming approach.
Luxon is a modern library for working with dates and times in JavaScript. It is built by one of the Moment.js developers and offers a more modern API with better support for internationalization. Luxon is more feature-rich than date-and-time and is designed to be a more modern alternative to Moment.js.
Moment.js, the most famous DateTime utility, is very useful. But it's also the bloated module (20.7k gz). If you are looking for a similar and smaller one, this would be a good solution.
via npm:
$ npm install date-and-time --save
via Bower:
$ bower install date-and-time
directly:
<script src="date-and-time.min.js"></script>
Node.js:
var date = require('date-and-time');
AMD:
require(['date-and-time'], function (date) {
});
the browser:
window.date; // global object
parse
NaN
object in case of parse error.1970
.locale
var now = new Date();
date.format(now, 'YYYY/MM/DD HH:mm:ss'); // => '2015/01/02 23:14:05'
date.format(now, 'ddd MMM DD YYYY'); // => 'Fri Jan 02 2015'
date.format(now, 'hh:mm A [GMT]Z'); // => '11:14 p.m. GMT-0800'
date.format(now, 'hh:mm A [GMT]Z', true); // => '07:14 a.m. GMT+0000'
token | meaning | example |
---|---|---|
YYYY | year | 0999, 2015 |
YY | year | 15, 99 |
Y | year | 999, 2015 |
MMMM | month | January, December |
MMM | month | Jan, Dec |
MM | month | 01, 12 |
M | month | 1, 12 |
DD | day | 02, 31 |
D | day | 2, 31 |
dddd | day of week | Friday, Sunday |
ddd | day of week | Fri, Sun |
dd | day of week | Fr, Su |
HH | hour-24 | 23, 08 |
H | hour-24 | 23, 8 |
A | meridiem | a.m., p.m. |
hh | hour-12 | 11, 08 |
h | hour-12 | 11, 8 |
mm | minute | 14, 07 |
m | minute | 14, 7 |
ss | second | 05, 10 |
s | second | 5, 10 |
SSS | millisecond | 753, 022 |
SS | millisecond | 75, 02 |
S | millisecond | 7, 0 |
Z | timezone | +0100, -0800 |
[...]
in the formatString
will be a comment:
date.format(new Date(), 'DD-[MM]-YYYY'); // => '02-MM-2015'
date.format(new Date(), '[DD-[MM]-YYYY]'); // => 'DD-[MM]-YYYY'
date.parse('2015/01/02 23:14:05', 'YYYY/MM/DD HH:mm:ss'); // => date object
date.parse('02-01-2015', 'DD-MM-YYYY'); // => date object
date.parse('11:14:05 p.m.', 'hh:mm:ss A'); // => (Jan 1 1970 23:14:05 GMT-0800)
date.parse('11:14:05 p.m.', 'hh:mm:ss A', true); // => (Jan 1 1970 15:14:05 GMT-0800)
date.parse('Jam 1 2017', 'MMM D YYYY'); // => NaN
date.parse('Feb 29 2017', 'MMM D YYYY'); // => NaN
token | meaning | example |
---|---|---|
YYYY | year | 2015, 1999 |
YY | year | 15, 99 |
MMMM | month | January, December |
MMM | month | Jan, Dec |
MM | month | 01, 12 |
M | month | 1, 12 |
DD | day | 02, 31 |
D | day | 2, 31 |
HH | hour-24 | 23, 08 |
H | hour-24 | 23, 8 |
hh | hour-12 | 11, 08 |
h | hour-12 | 11, 8 |
A | meridiem | a.m., p.m. |
mm | minute | 14, 07 |
m | minute | 14, 7 |
ss | second | 05, 10 |
s | second | 5, 10 |
SSS | millisecond | 753, 022 |
SS | millisecond | 75, 02 |
S | millisecond | 7, 0 |
The minimum year that can be parsed is year 100, the maximum year is year 9999. Year 69 or less are translated into 2000s, year 70 or more and year 99 or less are translated into 1900s.
date.parse('Dec 31 100', 'MMM d YYYY'); // => (Dec 31 100)
date.parse('Dec 31 9999', 'MMM d YYYY'); // => (Dec 31 9999)
date.parse('Dec 31 0', 'MMM d YYYY'); // => (Dec 31 2000)
date.parse('Dec 31 69', 'MMM d YYYY'); // => (Dec 31 2069)
date.parse('Dec 31 70', 'MMM d YYYY'); // => (Dec 31 1970)
date.parse('Dec 31 99', 'MMM d YYYY'); // => (Dec 31 1999)
When using hh
or h
(hour-12), need to use together A
(meridiem).
date.isValid('2015/01/02 23:14:05', 'YYYY/MM/DD HH:mm:ss'); // => true
date.isValid('29-02-2015', 'DD-MM-YYYY'); // => false
The formatString
you can set is the same as the parse
function's.
var now = new Date();
var next_year = date.addYears(now, 1); // => Date object
var now = new Date();
var next_month = date.addMonths(now, 1); // => Date object
var now = new Date();
var yesterday = date.addDays(now, -1); // => Date object
var now = new Date();
var an_hour_ago = date.addHours(now, -1); // => Date object
var now = new Date();
var two_minutes_later = date.addMinutes(now, 2); // => Date object
var now = new Date();
var three_seconds_ago = date.addSeconds(now, -3); // => Date object
var now = new Date();
var a_millisecond_later = date.addMilliseconds(now, 1); // => Date object
var today = new Date(2015, 0, 2);
var yesterday = new Date(2015, 0, 1);
date.subtract(today, yesterday).toDays(); // => 1 = today - yesterday
date.subtract(today, yesterday).toHours(); // => 24
date.subtract(today, yesterday).toMinutes(); // => 1440
date.subtract(today, yesterday).toSeconds(); // => 86400
date.subtract(today, yesterday).toMilliseconds(); // => 86400000
var date1 = new Date(2015, 0, 2);
var date2 = new Date(2012, 0, 2);
date.isLeapYear(date1); // => false
date.isLeapYear(date2); // => true
var date1 = new Date(2017, 0, 2, 0); // Jan 2 2017 00:00:00
var date2 = new Date(2017, 0, 2, 23, 59); // Jan 2 2017 23:59:00
var date3 = new Date(2017, 0, 1, 23, 59); // Jan 1 2017 23:59:00
date.isSameDay(date1, date2); // => true
date.isSameDay(date1, date3); // => false
It supports the following languages for now:
Month, day of week, and meridiem are displayed in English by default. If you want to use other languages, can switch to them as follows:
Node.js:
var date = require('date-and-time');
date.locale('fr'); // French
date.format(new Date(), 'dddd D MMMM'); // => 'lundi 11 janvier'
AMD:
require(['date-and-time', 'locale/de'], function (date) {
date.locale('de'); // German
date.format(new Date(), 'dddd, D. MMMM'); // => 'Montag, 11. Januar'
});
the browser:
<script src="date-and-time.min.js"></script>
<script src="locale/zh-cn.js"></script>
<script>
date.locale('zh-cn'); // Chinese
date.format(new Date(), 'MMMD日dddd'); // => '1月11日星期一'
</script>
You can not only switch to other languages, but can customize them as you want:
var now = new Date();
date.format(now, 'h:m A'); // => '12:34 p.m.'
date.setLocales('en', {
A: ['AM', 'PM']
});
date.format(now, 'h:m A'); // => '12:34 PM'
Chrome, Firefox, Safari, Opera, and Internet Explorer 6+.
MIT
FAQs
A Minimalist DateTime utility for Node.js and the browser
The npm package date-and-time receives a total of 172,864 weekly downloads. As such, date-and-time popularity was classified as popular.
We found that date-and-time demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.