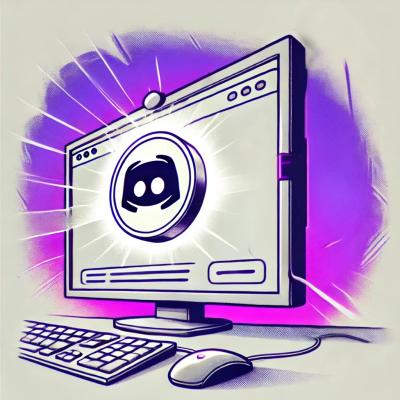
Research
Security News
Malicious PyPI Package ‘pycord-self’ Targets Discord Developers with Token Theft and Backdoor Exploit
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
date-period
Advanced tools
Time period iterator.
A time period in this context is an array of Date objects, recurring at regular intervals, over a given period. Based on ISO 8601's duration and repeating interval format.
Mimics PHP's excellent DatePeriod class.
$ npm install date-period --save
createPeriod({ start: Date, duration: String, end: Date }) ⇒ Array<Date>
createPeriod({ start: Date, duration: String, recurrence: Number }) ⇒ Array<Date>
createPeriod({ iso: String }) ⇒ Array<Date>
Note: the date
and duration
parameters above can be objects which have, respectively, toDate
and toString
methods. This way moment objects are supported.
Require the module
const createPeriod = require('date-period');
let start = new Date('2014-01-01T00:00:00Z'),
duration = 'P1D',
end = new Date('2014-01-05T00:00:00Z'), // not included in result
period;
period = createPeriod({ start, duration, end });
// or, with the number of recurrences instead of an end date:
period = createPeriod({ start, duration, recurrence: 3 });
// or, with a string formatted as an ISO 8601 repeating interval:
period = createPeriod({ iso: 'R3/2014-01-01T00:00:00Z/P1D' });
FAQs
Time period iterator
We found that date-period demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.