English | 简体中文
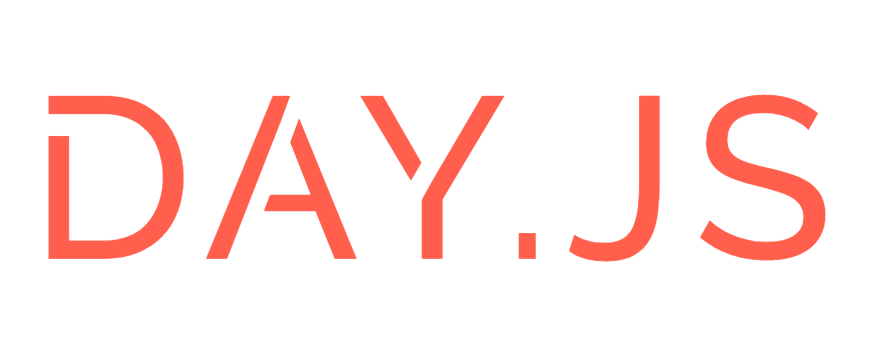
Fast 2kB alternative to Moment.js with the same modern API
Day.js is a minimalist JavaScript library for modern browsers with a largely Moment.js-compatible API. If you use Moment.js, you already know how to use Day.js.
dayjs().startOf('month').add(1, 'day').set('year', 2018).format('YYYY-MM-DD HH:mm:ss');
- 🕒 Familiar Moment.js API & patterns
- 💪 Immutable
- 🔥 Chainable
- 📦 2kb mini library
- 👫 All browsers support
Installation
You have multiple ways of getting Day.js:
npm install dayjs --save
var dayjs = require('dayjs');
dayjs().format();
<script src="https://unpkg.com/dayjs"></script>
<script>
dayjs().format();
</script>
- Via download and self-hosting:
Just download the latest version of Day.js at https://unpkg.com/dayjs/dist/
Getting Started
Instead of modifying the native Date.prototype
, Day.js creates a wrapper for the Date object, called Dayjs
object.
Dayjs
object is immutable, that is to say, all API operation will return a new Dayjs
object.
API
API will always return a new Dayjs
object if not specified.
Parse
Simply call dayjs()
with one of the supported input types.
Now
To get the current date and time, just call dayjs() with no parameters.
dayjs();
String
Creating from a string matches ISO 8601 format.
dayjs(String);
dayjs('1995-12-25');
Unix Timestamp (milliseconds)
Passing an integer value representing the number of milliseconds since the Unix Epoch (Jan 1 1970 12AM UTC).
dayjs(Number);
dayjs(1318781876406);
Date
Passing a pre-existing native Javascript Date object.
dayjs(Date);
dayjs(new Date(2018, 8, 18));
Clone
All Dayjs
are immutable. If you want a copy of the object, just call .clone()
.
Calling dayjs() on a Dayjs
object will also clone it.
dayjs(Dayjs);
dayjs().clone();
Validation
Check whether the Dayjs
object considers the date invalid.
dayjs().isValid();
Get + Set
Get and set date.
Year
Get year.
dayjs().year();
Month
Get month.
dayjs().month();
Date of Month
Get day of the month.
dayjs().date();
Day of Week
Get day of the week.
dayjs().day();
Hour
Get hour.
dayjs().hour();
Minute
Get minute.
dayjs().minute();
Second
Get second.
dayjs().second();
Millisecond
Get millisecond.
dayjs().millisecond();
Set
Date setter.
Units are case insensitive
dayjs().set((unit: String), (value: Int));
dayjs().set('month', 3);
dayjs().set('second', 30);
Manipulate
Once you have a Dayjs
object, you may want to manipulate it in some way like this:
dayjs()
.startOf('month')
.add(1, 'day')
.subtract(1, 'year');
Add
Returns a new Dayjs
object by adding time.
dayjs().add((value: Number), (unit: String));
dayjs().add(7, 'day');
Subtract
Returns a new Dayjs
object by subtracting time. exactly the same as dayjs#add
.
dayjs().subtract((value: Number), (unit: String));
dayjs().subtract(7, 'year');
Start of Time
Returns a new Dayjs
object by by setting it to the start of a unit of time.
dayjs().startOf((unit: String));
dayjs().startOf('year');
End of Time
Returns a new Dayjs
object by by setting it to the end of a unit of time.
dayjs().endOf((unit: String));
dayjs().endOf('month');
Display
Once parsing and manipulation are done, you need some way to display the Dayjs
object.
Format
Takes a string of tokens and replaces them with their corresponding date values.
dayjs().format(String);
dayjs().format();
dayjs().format('{YYYY} MM-DDTHH:mm:ssZ[Z]');
- To escape characters in string, wrap the characters in square brackets (e.g. [Z]).
List of all available formats:
Format | Output | Description |
---|
YY | 18 | Two digit year |
YYYY | 2018 | Four digit year |
M | 1-12 | The month, beginning at 1 |
MM | 01-12 | The month, 2-digits |
MMM | Jan-Dec | The abbreviated month name |
MMMM | January-December | The full month name |
D | 1-31 | The day of the month |
DD | 01-31 | The day of the month, 2-digits |
d | 0-6 | The day of the week, with Sunday as 0 |
dddd | Sunday-Saturday | The name of the day of the week |
H | 0-23 | The hour |
HH | 00-23 | The hour, 2-digits |
h | 1-12 | The hour, 12-hour clock |
hh | 01-12 | The hour, 12-hour clock, 2-digits |
m | 0-59 | The minute |
mm | 00-59 | The minute, 2-digits |
s | 0-59 | The second |
ss | 00-59 | The second, 2-digits |
SSS | 000-999 | The millisecond, 3-digits |
Z | +5:00 | The offset from UTC |
ZZ | +0500 | The offset from UTC, 2-digits |
A | AM PM | |
a | am pm | |
Difference
Get the difference of two Dayjs
object in milliseconds or other unit.
dayjs().diff(Dayjs, unit);
dayjs().diff(dayjs(), 'years');
Unix Timestamp (milliseconds)
Outputs the number of milliseconds since the Unix Epoch
dayjs().valueOf();
Unix Timestamp (seconds)
Outputs a Unix timestamp (the number of seconds since the Unix Epoch).
dayjs().unix();
Days in Month
Get the number of days in the current month.
dayjs().daysInMonth();
As Javascript Date
- returns a Javascript
Date
object
Get copy of the native Date
object from Dayjs
object.
dayjs().toDate();
As Array
Returns an array that mirrors the parameters from new Date().
dayjs().toArray();
As JSON
Serializing an Dayjs
to JSON, will return an ISO8601 string.
dayjs().toJSON();
As ISO 8601 String
Formats a string to the ISO8601 standard.
dayjs().toISOString();
As Object
Returns an object with year, month ... millisecond.
dayjs().toObject();
As String
dayjs().toString();
Query
Is Before
Check if a Dayjs
object is before another Dayjs
object.
dayjs().isBefore(Dayjs);
dayjs().isBefore(dayjs());
Is Same
Check if a Dayjs
object is same as another Dayjs
object.
dayjs().isSame(Dayjs);
dayjs().isSame(dayjs());
Is After
Check if a Dayjs
object is after another Dayjs
object.
dayjs().isAfter(Dayjs);
dayjs().isAfter(dayjs());
Is Leap Year
Check if a year is a leap year.
dayjs().isLeapYear();
dayjs('2000-01-01').isLeapYear();
License
MIT