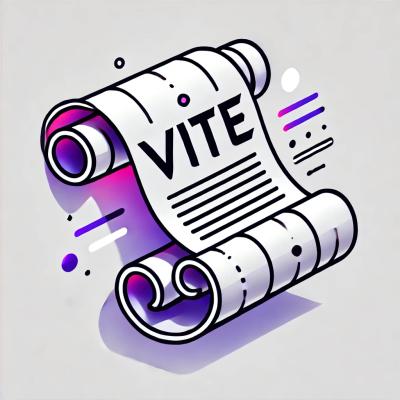
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
dcts-google-places-api
Advanced tools
Node client for Google Places API (placeSearch, placeDetails, placePhoto). Written by dcts.
Node client to run the basic google places API requests (using axios). Published on NPM.
$ npm install dcts-google-places-api
const apiKey = "INSERT_YOUR_API_KEY"; // define API key
const GooglePlacesApi = require('dcts-google-places-api');
const googleapi = new GooglePlacesApi(apiKey); // initialize
const searchQuery = "Pizza New York"; // example search
// with promises
googleapi.runPlaceSearch(searchQuery).then(placeId => {
console.log(placeId); // returns placeId (as string) or null
});
// with async await
(async () => {
let placeId = await googleapi.runPlaceSearch(searchQuery);
console.log(placeId);
})();;
Get place details by place_id
.
const placeId = "ChIJHegKoJUfyUwRjMxaCcviZDA"; // placeId of "Pizza Chicken New York"
// with promises
googleapi.runPlaceDetails(placeId).then(placeDetails => {
console.log(`place found: ${placeDetails.name}`);
})
// with async await
(async () => {
let placeDetails = await googleapi.runPlaceDetails(placeId);
console.log(`place found: ${placeDetails.name}`);
})();
Get place details by cid
. This is a deprecated endpoint and not documented in the official google api documentation, but it still works. See also this answer on stackoverflow
const cid = "10056734717913051463"; // cid of "Brooklyn Boulders Chicago"
// with promises
googleapi.runPlaceDetailsCid(cid).then(placeDetails => {
console.log(`place found: ${placeDetails.name} (${placeDetails.cid})`);
});
// with async await
(async () => {
let placeDetails = await googleapi.runPlaceDetailsCid(cid);
console.log(`place found: ${placeDetails.name} (${placeDetails.cid})`);
})();
⚠️ Be Aware: this function returns only the photoUrl, not the image itself.
const photoReference = 'CmRaAAAAqYV1efHXXLX3UB1msekeprgOUD362n4-8lxwYI3aSFANLw51oE1_KeNziEgnnbr5WQzJtQo9SbNnZFRfymg594T9h7yRWnLQL8w1n_ekN6BbyJzg1k0hadSJ4N0i63TmEhA3NIzf_JWUEZcW3VgXJ5FqGhRq7ij6D2Vl8DOSF2yHY1iuTYuAKA';
// with promises
googleapi.runPlacePhotos(photoReference).then(photoUrl => {
console.log(`photo url: ${photoUrl}`);
});
// with async await
(async () => {
let photoUrl = await googleapi.runPlacePhotos(photoReference);
console.log(`photo url: ${photoUrl}`);
})();
const textSearch = "Nolita Pizza New York";
// with promises
googleapi.runPlaceSearch(textSearch).then(placeId => {
console.log(`place id found: ${placeId}`);
return googleapi.runPlaceDetails(placeId);
}).then(placeDetails => {
console.log(`place details fetched for ${placeDetails.name} (${placeDetails.place_id})`);
const promises = [];
placeDetails.photos.map(photo => {
promises.push(googleapi.runPlacePhotos(photo.photo_reference));
});
return Promise.all(promises);
}).then(placePhotoUrls => {
console.log(`${placePhotoUrls.length} photo urls fetched:`);
console.log(placePhotoUrls);
});
// with async await
(async () => {
let placeId = await googleapi.runPlaceSearch(textSearch)
console.log(`place id found: ${placeId}`);
let placeDetails = await googleapi.runPlaceDetails(placeId);
console.log(`place details fetched for ${placeDetails.name} (${placeDetails.place_id})`);
const placePhotoUrls = [];
for (const photo of placeDetails.photos) {
placePhotoUrls.push(await googleapi.runPlacePhotos(photo.photo_reference));
}
console.log(`${placePhotoUrls.length} photo urls fetched:`);
console.log(placePhotoUrls);
})();
npm run test
npm run test
to see missing tests.encodeURI()
or encodeURIComponent
-> write tests for them first.Bartleby’s Ice Cream Cakes
-> no results from the API, but finds it on webinterface. I think it could be something with the top appostroph.Written by dcts for personal use only. Check my portfolio website. Or go play tetris.
FAQs
Node client for Google Places API (placeSearch, placeDetails, placePhoto). Written by dcts.
The npm package dcts-google-places-api receives a total of 0 weekly downloads. As such, dcts-google-places-api popularity was classified as not popular.
We found that dcts-google-places-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.