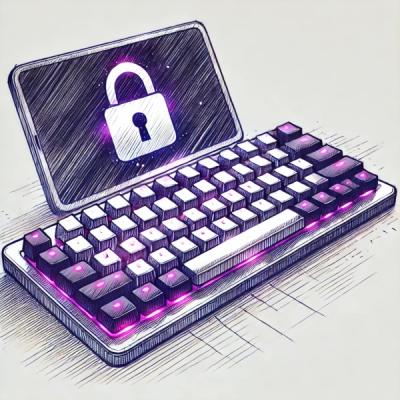
Research
Security News
Malicious npm Package Typosquats react-login-page to Deploy Keylogger
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
debounce-fn
Advanced tools
Package description
The debounce-fn npm package provides a simple and efficient way to debounce functions in JavaScript. Debouncing is a technique used to limit the rate at which a function is executed. This is particularly useful for performance optimization in scenarios where a function is called repeatedly in quick succession, such as during window resizing, scrolling, or keypress events.
Basic Debouncing
This feature allows you to debounce a function with a specified wait time. In this example, the `expensiveFunction` will only be called once every 200 milliseconds, even though `debouncedFunction` is called every 50 milliseconds.
const debounceFn = require('debounce-fn');
const expensiveFunction = () => {
console.log('Expensive function called');
};
const debouncedFunction = debounceFn(expensiveFunction, { wait: 200 });
// Call the debounced function multiple times
setInterval(debouncedFunction, 50);
Immediate Execution
This feature allows the debounced function to be executed immediately on the first call, and then debounced for subsequent calls. In this example, `logMessage` is executed immediately on the first call, and then debounced for 200 milliseconds.
const debounceFn = require('debounce-fn');
const logMessage = () => {
console.log('Function executed immediately');
};
const debouncedLogMessage = debounceFn(logMessage, { wait: 200, immediate: true });
debouncedLogMessage(); // Executes immediately
setTimeout(debouncedLogMessage, 100); // Will not execute
setTimeout(debouncedLogMessage, 300); // Executes after 300ms
Cancel Debounced Function
This feature allows you to cancel a debounced function before it gets executed. In this example, `fetchData` is debounced with a wait time of 300 milliseconds, but the second call to `debouncedFetchData.cancel()` cancels the execution.
const debounceFn = require('debounce-fn');
const fetchData = () => {
console.log('Fetching data');
};
const debouncedFetchData = debounceFn(fetchData, { wait: 300 });
debouncedFetchData();
debouncedFetchData.cancel(); // Cancels the debounced function
The lodash.debounce package is a popular utility for debouncing functions. It is part of the Lodash library, which provides a wide range of utility functions for JavaScript. Compared to debounce-fn, lodash.debounce offers more configuration options and is widely used in the JavaScript community.
The debounce package is a lightweight utility for debouncing functions. It provides a simple API similar to debounce-fn but with fewer configuration options. It is suitable for users who need a minimalistic solution for debouncing functions.
The throttle-debounce package provides both throttling and debouncing utilities. It is useful for scenarios where you need both functionalities. Compared to debounce-fn, throttle-debounce offers a more comprehensive solution for rate-limiting function executions.
Readme
Debounce a function
$ npm install debounce-fn
const debounceFn = require('debounce-fn');
window.onresize = debounceFn(() => {
// Do something on window resize
}, {wait: 100});
Returns a debounced function that delays calling the input
function until after wait
milliseconds have elapsed since the last time the debounced function was called.
It comes with a .cancel()
method to cancel any scheduled input
function calls.
Type: Function
Function to debounce.
Type: Object
Type: number
Default: 0
Time to wait until the input
function is called.
Type: boolean
Default: false
Trigger the function on the leading edge instead of the trailing edge of the wait
interval. For example, can be useful for preventing accidental double-clicks on a "submit" button from firing a second time.
MIT © Sindre Sorhus
FAQs
Unknown package
We found that debounce-fn demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
Security News
The JavaScript community has launched the e18e initiative to improve ecosystem performance by cleaning up dependency trees, speeding up critical parts of the ecosystem, and documenting lighter alternatives to established tools.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.