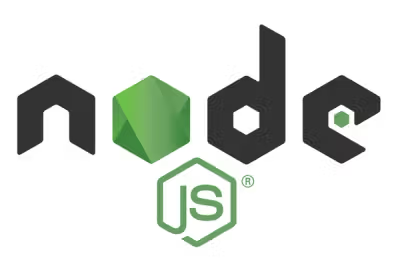
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
deckar01-di
Advanced tools
There are two things - Dependency Injection pattern (aka Inversion of Control) and Dependency Injection framework.
The Dependency Injection pattern is about separating the instantiation of objects from the actual logic and behavior that they encapsulate. This pattern has many benefits such as:
Following this pattern is, of course, possible without any framework.
However, if you do follow the Dependency Injection pattern, you typically end up with some kind of nasty main()
method, where you instantiate all the objects and wire them together. The Dependency Injection framework saves you from this boilerplate. It makes wiring the application declarative rather than imperative. Each component declares its dependencies and the framework does transitively resolve these dependencies...
var Car = function(engine) {
this.start = function() {
engine.start();
};
};
var createPetrolEngine = function(power) {
return {
start: function() {
console.log('Starting engine with ' + power + 'hp');
}
};
};
// a module is just a plain JavaScript object
// it is a recipe for the injector, how to instantiate stuff
var module = {
// if an object asks for 'car', the injector will call new Car(...) to produce it
'car': ['type', Car],
// if an object asks for 'engine', the injector will call createPetrolEngine(...) to produce it
'engine': ['factory', createPetrolEngine],
// if an object asks for 'power', the injector will give it number 1184
'power': ['value', 1184] // probably Bugatti Veyron
};
var di = require('di');
var injector = new di.Injector(module);
injector.invoke(function(car) {
car.start();
});
For more examples, check out the tests. You can also check out Karma and its plugins for more complex examples.
To produce the instance, Constructor
will be called with new
operator.
var module = {
'engine': ['type', DieselEngine]
};
To produce the instance, factoryFn
will be called (without any context) and its result will be used.
var module = {
'engine': ['factory', createDieselEngine]
};
Register the final value.
var module = {
'power': ['value', 1184]
};
The injector looks up tokens based on argument names:
var Car = function(engine, license) {
// will inject objects bound to 'engine' and 'license' tokens
};
You can also use comments:
var Car = function(/* engine */ e, /* x._weird */ x) {
// will inject objects bound to 'engine' and 'x._weird' tokens
};
Sometimes it is helpful to inject only a specific property of some object:
var Engine = function(/* config.engine.power */ power) {
// will inject 1184 (config.engine.power),
// assuming there is no direct binding for 'config.engine.power' token
};
var module = {
'config': ['value', {engine: {power: 1184}, other : {}}]
};
Made for Karma. Heavily influenced by AngularJS. Also inspired by Guice and Pico Container.
FAQs
Dependency Injection for Node.js. Heavily inspired by AngularJS.
The npm package deckar01-di receives a total of 2 weekly downloads. As such, deckar01-di popularity was classified as not popular.
We found that deckar01-di demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.