decode-deps
Advanced tools
Comparing version 1.1.1 to 1.2.0
@@ -1,2 +0,2 @@ | ||
export declare const port = 5001; | ||
export declare const portNumber = 5001; | ||
export declare const maxNodes = 500; |
@@ -1,2 +0,2 @@ | ||
export var port = 5001; | ||
export var portNumber = 5001; | ||
export var maxNodes = 500; |
@@ -1,1 +0,1 @@ | ||
export default function startDepTrack(sourceDir: string[]): void; | ||
export default function startDecodeDeps(sourceDir: string[]): void; |
@@ -12,4 +12,5 @@ import express from "express"; | ||
import { getDependencies } from "./utils/fileUtils.js"; | ||
import { port } from "./constant.js"; | ||
export default function startDepTrack(sourceDir) { | ||
import { portNumber } from "./constant.js"; | ||
export default function startDecodeDeps(sourceDir) { | ||
var _filename = fileURLToPath(import.meta.url); | ||
@@ -20,2 +21,3 @@ var _dirname = dirname(_filename); | ||
app.use(express.static(path.join(_dirname, "/"))); | ||
app.use(express.static(path.join(_dirname, "utils"))); | ||
app.get("/", function (req, res) { | ||
@@ -43,7 +45,7 @@ res.sendFile(path.join(_dirname, "/", "index.html")); | ||
}); | ||
app.listen(port, function () { | ||
app.listen(portNumber, function () { | ||
console.log( | ||
"Module Dependency Graph Ready at http://localhost:".concat(port) | ||
"Module Dependency Graph Ready at http://localhost:".concat(portNumber) | ||
); | ||
}); | ||
} |
@@ -5,7 +5,8 @@ export interface TreeNode { | ||
size: number; | ||
linkType?: "internal" | "external"; | ||
} | ||
export interface NodeType { | ||
id: string; | ||
children?: TreeNode[]; | ||
size: number; | ||
linkType: "internal" | "external"; | ||
} | ||
@@ -15,2 +16,3 @@ export interface LinkType { | ||
target: string; | ||
linkType: "internal" | "external"; | ||
} |
@@ -8,6 +8,14 @@ import { LinkType, NodeType, TreeNode } from "../types/types"; | ||
links: LinkType[]; | ||
warning: [string, string][]; | ||
warning: { | ||
circular: string[]; | ||
}[]; | ||
}; | ||
export declare function detectCircularDeps(links: LinkType[]): [string, string][]; | ||
export declare function detectCircularDeps(links: LinkType[]): { | ||
circular: string[]; | ||
}[]; | ||
export declare function removeCircularDeps(obj: any): any; | ||
export declare function removeDuplicateCircularDeps(dependencies: [string, string][]): [string, string][]; | ||
export declare function removeDuplicateCircularDeps(dependencies: { | ||
circular: string[]; | ||
}[]): { | ||
circular: string[]; | ||
}[]; |
@@ -0,7 +1,40 @@ | ||
var __assign = | ||
(this && this.__assign) || | ||
function () { | ||
__assign = | ||
Object.assign || | ||
function (t) { | ||
for (var s, i = 1, n = arguments.length; i < n; i++) { | ||
s = arguments[i]; | ||
for (var p in s) | ||
if (Object.prototype.hasOwnProperty.call(s, p)) t[p] = s[p]; | ||
} | ||
return t; | ||
}; | ||
return __assign.apply(this, arguments); | ||
}; | ||
import { getFileSize } from "./fileUtils.js"; | ||
import path from "path"; | ||
import * as fs from "fs"; | ||
var packageJsonPath = path.resolve(process.cwd(), "package.json"); | ||
var packageJson = JSON.parse(fs.readFileSync(packageJsonPath, "utf-8")); | ||
var allDependencies = __assign( | ||
__assign({}, packageJson.dependencies), | ||
packageJson.devDependencies | ||
); | ||
var allDependenciesName = Object.keys(allDependencies); | ||
export var buildTree = function (deps) { | ||
var nodesMap = {}; | ||
var getNode = function (id) { | ||
var fileRoot = id.split("/"); | ||
var fileName = fileRoot[fileRoot.length - 1].split(".")[0]; | ||
if (!nodesMap[id]) { | ||
nodesMap[id] = { id: id, children: [], size: getFileSize(id) }; | ||
nodesMap[id] = { | ||
id: id, | ||
children: [], | ||
size: getFileSize(id), | ||
linkType: allDependenciesName.includes(fileName) | ||
? "external" | ||
: "internal", | ||
}; | ||
} | ||
@@ -30,15 +63,27 @@ return nodesMap[id]; | ||
var warning = []; | ||
var visited = []; | ||
var visited = new Set(); | ||
function getNodes(node) { | ||
var fileRoot = node.id.split("/"); | ||
var fileName = fileRoot[fileRoot.length - 1].split(".")[0]; | ||
var newNode = { | ||
id: node.id, | ||
size: node.size, | ||
children: node.children, | ||
linkType: allDependenciesName.includes(fileName) | ||
? "external" | ||
: "internal", | ||
}; | ||
if (!visited.includes(newNode.id)) { | ||
visited.push(newNode.id); | ||
if (!visited.has(newNode.id)) { | ||
visited.add(newNode.id); | ||
nodes.push(newNode); | ||
if (node.children) { | ||
node.children.forEach(function (child) { | ||
links.push({ source: node.id, target: child.id }); | ||
links.push({ | ||
source: node.id, | ||
target: child.id, | ||
linkType: | ||
allDependenciesName.includes(node.id) || | ||
allDependenciesName.includes(child.id) | ||
? "external" | ||
: "internal", | ||
}); | ||
getNodes(child); | ||
@@ -50,3 +95,3 @@ }); | ||
trees.forEach(function (tree) { | ||
return getNodes(tree); | ||
getNodes(tree); | ||
}); | ||
@@ -57,10 +102,8 @@ return { nodes: nodes, links: links, warning: warning }; | ||
var visited = new Set(); | ||
var stack = new Set(); | ||
var circularDependencies = []; | ||
function visit(node) { | ||
if (stack.has(node)) { | ||
var circularPath = Array.from(stack).concat(node); | ||
for (var i = 0; i < circularPath.length - 1; i++) { | ||
circularDependencies.push([circularPath[i], circularPath[i + 1]]); | ||
} | ||
function visit(node, path) { | ||
if (path.includes(node)) { | ||
var cycleStartIndex = path.indexOf(node); | ||
var circularPath = path.slice(cycleStartIndex, cycleStartIndex + 2); | ||
circularDependencies.push({ circular: circularPath }); | ||
return true; | ||
@@ -70,10 +113,10 @@ } | ||
visited.add(node); | ||
stack.add(node); | ||
path.push(node); | ||
for (var _i = 0, links_2 = links; _i < links_2.length; _i++) { | ||
var link = links_2[_i]; | ||
if (link.source === node) { | ||
visit(link.target); | ||
visit(link.target, path); | ||
} | ||
} | ||
stack.delete(node); | ||
path.pop(); | ||
return false; | ||
@@ -84,3 +127,3 @@ } | ||
if (!visited.has(link.source)) { | ||
visit(link.source); | ||
visit(link.source, []); | ||
} | ||
@@ -111,8 +154,8 @@ } | ||
var result = []; | ||
dependencies.forEach(function (pair) { | ||
var sortedPair = pair.slice().sort(); | ||
var key = "".concat(sortedPair[0], "-").concat(sortedPair[1]); | ||
dependencies.forEach(function (dep) { | ||
var sortedDep = dep.circular.slice().sort(); | ||
var key = sortedDep.join("-"); | ||
if (!uniqueDeps.has(key)) { | ||
uniqueDeps.add(key); | ||
result.push(pair); | ||
result.push(dep); | ||
} | ||
@@ -119,0 +162,0 @@ }); |
{ | ||
"name": "decode-deps", | ||
"version": "1.1.1", | ||
"version": "1.2.0", | ||
"type": "module", | ||
"scripts": { | ||
"dev": "tsx test/test.ts", | ||
"build": "rm -fr dist && tsc --project tsconfig.json && cp -R public/* dist/" | ||
"dev": "tsx debug/index.ts", | ||
"vdev": "vite", | ||
"build": "rm -fr dist && tsc --project tsconfig.json && cp -R public/* dist/", | ||
"compile": "tsc --project tsconfig.json" | ||
}, | ||
@@ -17,5 +19,7 @@ "bin": { | ||
"@types/express": "^5.0.0", | ||
"cors": "^2.8.5", | ||
"d3": "^7.9.0", | ||
"tsx": "^4.19.2", | ||
"typescript": "^5.6.3" | ||
"typescript": "^5.6.3", | ||
"vite": "^5.4.11" | ||
}, | ||
@@ -22,0 +26,0 @@ "author": { |
@@ -1,19 +0,17 @@ | ||
# DecodeDeps | ||
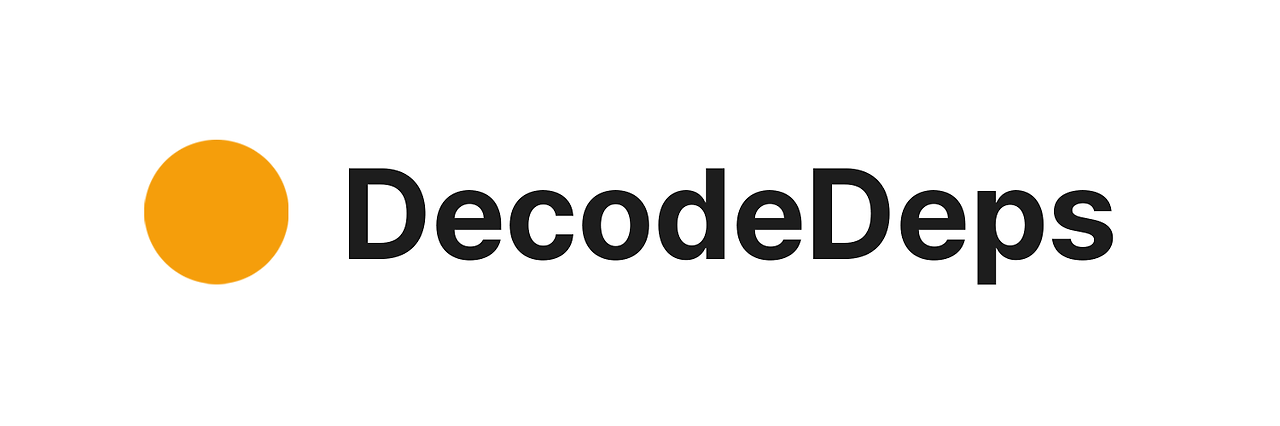 | ||
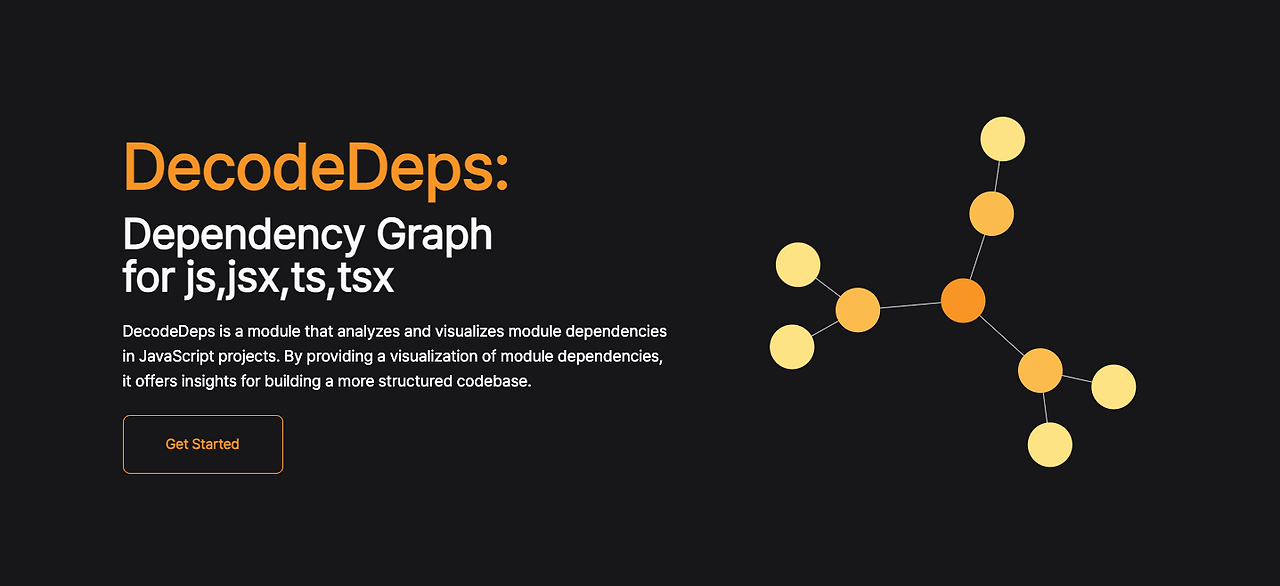 | ||
**DecodeDeps** is a dev-tool that analyzes and visualizes module dependencies in js, jsx, ts, tsx projects. It identifies modules using `import` and `require` statements and generates a graph to illustrate these relationships. By providing a visualization of module dependencies, it offers insights for building a more structured codebase. | ||
## 🚀 Last Update v.1.1.1 (November 5, 2024) | ||
## 🚀 Last Update v.1.2.0 (November 13, 2024) | ||
- Fix Circular Dependency Error. | ||
- Update new UI about Circulr Dependency Error. | ||
- Add functionality to distinguish and display **external** and **internal** modules | ||
- Update UI for the side menu | ||
## 🌟 Features | ||
## 🌟 Key Features | ||
- **JS, JSX, TS, TSX Support**: Works seamlessly with projects using JavaScript, JSX, TypeScript, and TSX. Visualizes module dependencies by analyzing `import` and `export` statements, providing a comprehensive graph view. | ||
- **Import & Require Support**: Analyzes both `import` and `require` statements to capture all dependencies across ES and CommonJS modules. | ||
- **Flexible Folder Input**: Input folders as an array to scan one or multiple directories, making it easy to analyze entire projects or specific subfolders. | ||
- **Dynamic Node Color**: Node colors vary based on module size, offering a quick visual indication of module weight. Hover over a node to view precise module size information. | ||
- **Interactive Graph Customization**: Adjust node size and link distance with intuitive sliders. Supports drag-and-drop functionality to explore dependencies interactively. | ||
- **For js, jsx, ts and tsx files**: Visualizes module dependencies by analyzing `import` and `require` statements, providing a graph view. | ||
- **Multiple folders**: Make it easy to analyze entire projects or specific subfolders. | ||
- **Detect circular dependencies**: Automatically identifies circular dependencies within your modules. | ||
- **Various Node Color**: Node colors vary based on module size, offering a quick visual indication. | ||
- **Interactive Graph**: Navigate and explore the dependency graph with zoom and pan features, as well as adjustable node sizes and link distances, providing a fully interactive visualization | ||
@@ -24,3 +22,3 @@ ## 👀 Preview | ||
 | ||
 | ||
@@ -33,3 +31,3 @@ Enter the command. | ||
 | ||
 | ||
@@ -71,3 +69,3 @@ ## 📥 Installation | ||
- **Junior Developers.** Quickly understand the overall code structure. | ||
- **Performance-Critical Project Teams.** Optimize build performance with improved bundling and loading speeds. | ||
- **Performance-Critical Project Teams.** Optimize build performance with improved bundling. | ||
@@ -82,12 +80,4 @@ ## 💬 Contributing | ||
## 🛠 Planned Updates | ||
The following features are planned for **future updates**: | ||
- Duplicate Dependency Tracking | ||
- File/Folder Search Function | ||
- Unused Code Detection | ||
## 📝 License | ||
This project is licensed under the **MIT License**. |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
Network access
Supply chain riskThis module accesses the network.
Found 1 instance in 1 package
59359
29
1313
6
80
2