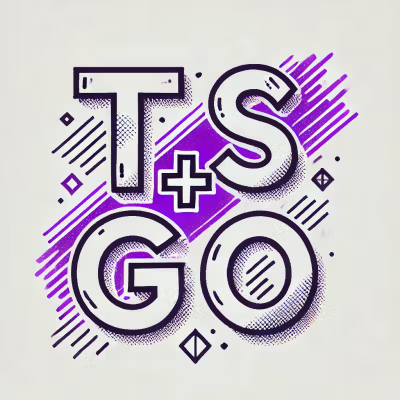
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Ridiculously easy JavaScript state containers with reducer methods. Like Redux without all of the boilerplate.
npm install --save deduce
// reducers.js
export function increment(state, val = 1) {
return state + val;
}
export function decrement(state, val = 1) {
return increment(state, -val);
}
// store.js
import deduce from 'deduce';
import * as reducers from './reducers';
const store = deduce(1, reducers);
store.addListener(() => {
console.log(store.state);
});
store.increment(); // -> 2
store.increment(2); // -> 4
store.decrement(); // -> 3
store.decrement(2); // -> 1
initialState
{*}
reducers
{Object<String,Function>}
Current state of the store.
const store = deduce({ foo: 1 });
console.log(store.state); // -> { foo: 1 }
reducers
{Object<String,Function>}
Registers reducers to modify the state. Chainable.
store.addReducers({
increment(state, val) {
return {
...state,
foo: state.foo + val,
};
},
});
property
{String}
reducers
{Object<String,Function>}
Registers reducers to modify a specific state property. Chainable.
store.addReducersFor('foo', {
increment(state, val) {
return state + val;
},
});
callback
{Function}
Adds a listener to be called any time the state is updated. Returns a function to remove the listener.
const removeListener = store.addListener(() => {
console.log(store.state);
});
store.increment();
The typical Redux patterns entail a lot of boilerplate. The documented and accepted patterns for reducing boilerplate really just swap one kind for another:
Consider the following Redux example that creates a store with two numbers: foo
which may be incremented and bar
which may be decremented.
// foo
const FOO_INCREMENT = 'FOO_INCREMENT';
const fooInitial = 0;
const fooReducers = {
[FOO_INCREMENT]: (state = fooInitial, action) {
return state + action.payload;
}
};
function foo(state = {}, action) {
if (action.type in fooReducers) {
return fooReducers[action.type](state, action);
}
return state;
}
function createFooIncrementAction(payload) {
return {
type: FOO_INCREMENT,
payload
};
}
// bar
const BAR_DECREMENT = 'BAR_DECREMENT';
const barInitial = 0;
const barReducers = {
[BAR_DECREMENT]: (state = barInitial, action) {
return state - action.payload;
}
};
function bar(state, action) {
if (action.type in barReducers) {
return barReducers[action.type](state, action);
}
return state;
}
function createBarDecrementAction(payload) {
return {
type: BAR_DECREMENT,
payload
};
}
// store
import { createStore, combineReducers } from 'redux';
const reducer = combineReducers({ foo, bar });
const store = createStore(reducer, {});
// application
store.dispatch(createFooIncrementAction(1));
store.dispatch(createBarDecrementAction(1));
console.log(store.getState());
// {
// foo: 1,
// bar: -1
// }
Split that up into modules and you can see how new-comers could easily be overwhelmed when the underlying principles are beautifully clean and simple.
Compare the above with this deduce
example that does the same thing:
// foo
const fooInitial = 0;
const fooReducers = {
incrementFoo(state = fooInitial, val) {
return state + val;
}
};
// bar
const barInitial = 0;
const barReducers = {
decrementBar(state = barInitial, val) {
return state - val;
}
};
// store
import deduce from 'deduce';
const store = deduce()
.addReducersFor('foo', fooReducers)
.addReducersFor('bar', barReducers);
// application
store.incrementFoo(1);
store.decrementBar(1);
console.log(store.state);
// {
// foo: 1,
// bar: -1
// }
Standards for this project, including tests, code coverage, and semantics are enforced with a build tool. Pull requests must include passing tests with 100% code coverage and no linting errors.
$ npm test
MIT © Shannon Moeller
FAQs
Ridiculously easy JavaScript state containers with reducer methods.
The npm package deduce receives a total of 1 weekly downloads. As such, deduce popularity was classified as not popular.
We found that deduce demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.