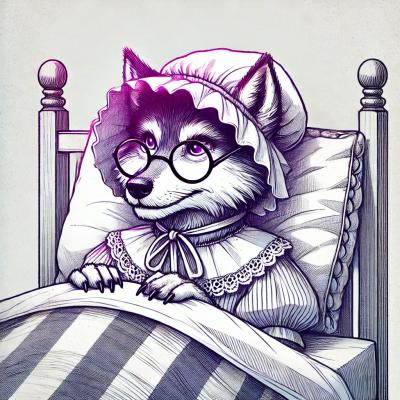
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
deepmerge-ts
Advanced tools
The deepmerge-ts package is a TypeScript library designed to deeply merge two or more objects. It ensures type safety and is particularly useful for complex nested objects where shallow merging would not suffice.
Basic Deep Merge
This feature allows you to deeply merge two objects. In this example, obj1 and obj2 are merged such that the nested properties are combined.
const deepmerge = require('deepmerge-ts').default;
const obj1 = { a: 1, b: { c: 2 } };
const obj2 = { b: { d: 3 } };
const result = deepmerge(obj1, obj2);
console.log(result); // { a: 1, b: { c: 2, d: 3 } }
Array Merge
This feature allows you to merge arrays within objects. In this example, the arrays in obj1 and obj2 are concatenated.
const deepmerge = require('deepmerge-ts').default;
const obj1 = { a: [1, 2, 3] };
const obj2 = { a: [4, 5] };
const result = deepmerge(obj1, obj2);
console.log(result); // { a: [1, 2, 3, 4, 5] }
Custom Merge Function
This feature allows you to define custom merge functions for specific keys. In this example, the custom merge function concatenates arrays for the key 'a'.
const deepmerge = require('deepmerge-ts').default;
const customMerge = (key, options) => {
if (key === 'a') {
return (a, b) => a.concat(b);
}
return undefined;
};
const obj1 = { a: [1, 2, 3], b: { c: 2 } };
const obj2 = { a: [4, 5], b: { d: 3 } };
const result = deepmerge(obj1, obj2, { customMerge });
console.log(result); // { a: [1, 2, 3, 4, 5], b: { c: 2, d: 3 } }
The deepmerge package is a popular library for deep merging objects in JavaScript. It is similar to deepmerge-ts but does not provide TypeScript type safety out of the box.
Lodash is a utility library that offers a wide range of functions, including deep merging with lodash.merge. While it is versatile and widely used, it is a larger library compared to deepmerge-ts, which is more specialized.
The merge-deep package is another library for deep merging objects. It is simpler and less feature-rich compared to deepmerge-ts, focusing solely on deep merging without additional customization options.
Any donations would be much appreciated. 😄
# Install with npm
npm install deepmerge-ts --save-dev
# Install with yarn
yarn add -D deepmerge-ts
// import_map.json
{
"imports": {
"deepmerge-ts": "https://deno.land/x/deepmergets@__version__/dist/deno/index.ts"
}
}
import { deepmerge } from "deepmerge-ts";
const x = {
record: {
prop1: "value1",
prop2: "value2",
},
array: [1, 2, 3],
set: new Set([1, 2, 3]),
map: new Map([
["key1", "value1"],
["key2", "value2"],
]),
};
const y = {
record: {
prop1: "changed",
prop3: "value3",
},
array: [2, 3, 4],
set: new Set([2, 3, 4]),
map: new Map([
["key2", "changed"],
["key3", "value3"],
]),
};
const merged = deepmerge(x, y);
console.log(merged);
// Prettierfied output:
//
// Object {
// "record": Object {
// "prop1": "changed",
// "prop2": "value2",
// "prop3": "value3",
// },
// "array": Array [1, 2, 3, 2, 3, 4],
// "set": Set { 1, 2, 3, 4 },
// "map": Map {
// "key1" => "value1",
// "key2" => "changed",
// "key3" => "value3",
// },
// }
You can try out this example at codesandbox.io.
We use smart merging instead of the classic merging strategy which some alternative libraries use. This vastly improves performance, both in execution time and memory usage.
With classic merging, each input is merged with the next input until all inputs are merged.
This strategy has large performance issues when lots of items need to be merged.
With our smart merging, we look ahead to see what can be merged and only merge those things.
In addition to performance improvements, this strategy merges multiple inputs at once; allowing for benefits such as taking averages of the inputs.
3.0.0 (2022-02-19)
deepmergeCustom
FAQs
Deeply merge 2 or more objects respecting type information.
The npm package deepmerge-ts receives a total of 446,833 weekly downloads. As such, deepmerge-ts popularity was classified as popular.
We found that deepmerge-ts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.