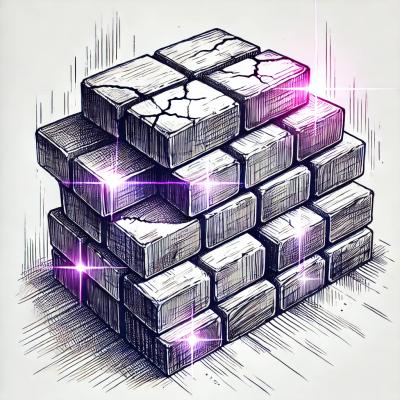
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
degiro-api
Advanced tools
Unofficial DeGiro API for Javascript. Buy and sell in the stock market. See your portfolio and much more
This is an unofficial Node.js API client for DeGiro's trading platform. Using this module you can easily automate your orders (buy and sell) and get information about orders, funds or products.
DeGiro is Europe's fastest growing online stockbroker. DeGiro distinguishes itself by offering institutional fees to retail investors.
⚠️ DeGiro could change their API at any moment, if something is not working, please open an issue.
# using npm
npm install --save degiro-api
# using yarn
yarn add degiro-api
Basic log into DeGiro Platform. All endpoint needs a session key before those can be call them. You can pass credentials to DeGiro constructor or export in your terminal prompt sesion as DEGIRO_USER
and DEGIRO_PWD
const DeGiro = require('degiro-api')
const degiro = new DeGiro({
username: 'username',
pwd: '*****'
})
degiro.login()
.then((accountData) => console.log('Log in success\n', accountData))
.catch(console.error)
// or creating with the static create method
const degiro = DeGiro.create({ username: '*****', pwd: '*****' })
const accountData = await degiro.login()
// or create with env credentials
const degiro = new DeGiro() // <-- Use DEGIRO_USER & DEGIRO_PWD
const accountData = await degiro.login()
$ export DEGIRO_DEBUG=1
$ yarn start
Run the next command and open index.html file inside doc folder.
$ yarn doc
yarn run v1.22.4
$ typedoc --out docs src
Using TypeScript 3.9.2 from ....../degiro-api/node_modules/typescript/lib
Rendering [========================================] 100%
Documentation generated at ....../degiro-api/docs
✨ Done in 3.94s.
Before run the test set you must set DEGIRO_USER & DEGIRO_PWD env export variables to attach an account to the test sets.
$ yarn install && yarn test
yarn run v1.22.4
$ mocha -r ts-node/register tests/**/*.spec.ts
Environment variables
✓ DEGIRO_USER env var should exists
✓ DEGIRO_PWD env var should exists
Create DeGiro instance
✓ should create an instance of DeGiro class from env vars
✓ should create an instance of DeGiro class from constructor params
DeGiro login process
✓ should successfully log in with environment credentials (619ms)
✓ should return a valid account config from server (738ms)
✓ should return a valid account data from server (727ms)
✓ getJSESSIONID should return a valid jsessionId
✓ should login with previous jsessionId
DeGiro logout process
✓ should successfully log out after sign in (685ms)
10 passing (3s)
✨ Done in 5.21s.
The JSessionId is the session browser cookie that DeGiro use to authenticate requests. You could prevent masive login/logout requests reusing a valid jsessionid from previous DeGiro instance. The way to do that is:
getJSESSIONID(): string
import DeGiro from 'degiro-api'
(async () => {
const degiro = new DeGiro({}) // <-- Using ENV variables
await degiro.login()
// Get the jsessionId (LOOK, is not a promise)
const jsessionId = degiro.getJSESSIONID()
})()
import DeGiro from 'degiro-api'
(async () => {
// Create an instance from a previous session
const degiro = new DeGiro({
username: '<your_username_here>',
pwd: '*******',
jsessionId: previousJSESSIONID
})
// Hydrate
// Re-use sessions need to re-hydrate the account config data and could use as a session expiration checker
await degiro.login()
// Do your stuff here...
})()
isLogin(options): boolean
import DeGiro from 'degiro-api'
(async () => {
// Create an instance from a previous session
const degiro = new DeGiro({}) // <-- Using ENV variables
if (!degiro.isLogin()) {
await degiro.login()
if (degiro.isLogin()) {
// AWESOME!! We're in
}
}
})()
The problem with this method is that it only checks if you have the account configuration data set. The only way to verify that the session is still active is make a request.
You can force isLogin method passing it a secure
field set to true. This way the method will return a promise and below it will call a DeGiro API endpoint (usually getAccountConfig)
import DeGiro from 'degiro-api'
(async () => {
// Create an instance from a previous session
const degiro = new DeGiro({}) // <-- Using ENV variables
// Force to make a request and check if session is still alive
if(! await degiro.isLogin({ secure: true })) {
await degiro.login()
}
})()
Get account info using await
:
import DeGiro from 'degiro-api'
(async () => {
const degiro = new DeGiro({
username: 'username',
pwd: '*****'
})
await degiro.login() // Login also returns accountData
const accountData = await degiro.getAccountData()
// console.log(accountData)
})()
getPortfolio(config: GetPorfolioConfigType): Promise<any[]>
getPortfolio
config parameter could have:
type: set the types or positions you want to fetch. Could be:
getProductDetails: if is set to true the positions results will have a productData
field with all the product details.
Get all open positions:
import DeGiro, { DeGiroEnums, DeGiroTypes } from 'degiro-api'
const { PORTFOLIO_POSITIONS_TYPE_ENUM } = DeGiroEnums
(async () => {
const degiro: DeGiro = new DeGiro({
username: 'your_username_here',
pwd: '**********',
})
await degiro.login()
const portfolio = await degiro.getPortfolio({
type: PORTFOLIO_POSITIONS_TYPE_ENUM.ALL,
getProductDetails: true,
})
console.log(JSON.stringify(portfolio, null, 2))
})()
Also you can fetch your portfolio data this way:
import DeGiro, { DeGiroEnums, DeGiroTypes } from 'degiro-api'
const { PORTFOLIO_POSITIONS_TYPE_ENUM } = DeGiroEnums
(async () => {
const degiro: DeGiro = new DeGiro({
username: 'your_username_here',
pwd: '**********',
})
await degiro.login()
const portfolio = await degiro.getPortfolio({ type: PORTFOLIO_POSITIONS_TYPE_ENUM.ALL })
console.log(JSON.stringify(portfolio, null, 2))
})()
And getting product details too
import DeGiro, { DeGiroEnums, DeGiroTypes } from 'degiro-api'
const { PORTFOLIO_POSITIONS_TYPE_ENUM } = DeGiroEnums
(async () => {
const degiro: DeGiro = new DeGiro({
username: 'your_username_here',
pwd: '**********',
})
await degiro.login()
const portfolio = await degiro.getPortfolio({
type: PORTFOLIO_POSITIONS_TYPE_ENUM.ALL,
getProductDetails: true,
})
console.log(JSON.stringify(portfolio, null, 2))
})()
degiro.searchProduct(options): Promise<SearchProductResultType[]>
DeGiroProducTypes
Search the text "AAPL" without any limitation
import DeGiro from 'degiro-api'
(async () => {
const degiro: DeGiro = new DeGiro({
username: 'your_username_here',
pwd: '***********',
})
await degiro.login()
const result = await degiro.searchProduct({ text: 'AAPL' })
console.log(JSON.stringify(result, null, 2))
})()
Search TSLA stock
import DeGiro, { DeGiroEnums, DeGiroTypes } from 'degiro-api'
const { DeGiroProducTypes } = DeGiroEnums
(async () => {
const degiro: DeGiro = new DeGiro({
username: 'your_username_here',
pwd: '***********',
})
await degiro.login()
const result = await degiro.searchProduct({
text: 'TSLA',
type: DeGiroProducTypes.shares,
limit: 1,
})
console.log(JSON.stringify(result, null, 2))
})()
degiro.createOrder(order: OrderType): Promise<CreateOrderResultType>
OrderType
DeGiroActions
DeGiroMarketOrderTypes
DeGiroTimeTypes
CreateOrderResultType
TransactionFeeType
import DeGiro, { DeGiroEnums, DeGiroTypes } from 'degiro-api'
const { DeGiroActions, DeGiroMarketOrderTypes, DeGiroTimeTypes } = DeGiroEnums
const { OrderType } = DeGiroTypes
(async () => {
const degiro: DeGiro = new DeGiro({
username: 'your_username_here',
pwd: '************'
})
await degiro.login()
const order: OrderType = {
buySell: DeGiroActions.BUY,
orderType: DeGiroMarketOrderTypes.LIMITED,
productId: '331868', // $AAPL - Apple Inc
size: 1,
timeType: DeGiroTimeTypes.DAY,
price: 272, // limit price [Degiro could reject this value]
// stopPrice: 2,
}
const { confirmationId, freeSpaceNew, transactionFees } = await degiro.createOrder(order)
console.log(JSON.stringify({ confirmationId, freeSpaceNew, transactionFees }, null, 2))
})()
import DeGiro, { DeGiroEnums, DeGiroTypes } from 'degiro-api'
const { DeGiroActions, DeGiroMarketOrderTypes, DeGiroTimeTypes } = DeGiroEnums
const { OrderType } = DeGiroTypes
(async () => {
try {
const degiro: DeGiro = new DeGiro({
username: 'nachoogoomezomg',
pwd: <string>process.env.DEGIRO_PWD,
})
await degiro.login()
const order: OrderType = {
buySell: DeGiroActions.BUY,
orderType: DeGiroMarketOrderTypes.LIMITED,
productId: '331868', // $AAPL - Apple Inc
size: 1,
timeType: DeGiroTimeTypes.DAY,
price: 270, // limit price
// stopPrice: 2,
}
const { confirmationId, freeSpaceNew, transactionFees } = await degiro.createOrder(order)
const orderId = await degiro.executeOrder(order, confirmationId)
console.log(`Order executed with id: ${orderId}`)
} catch (error) {
console.error(error)
}
})()
import DeGiro, { DeGiroEnums, DeGiroTypes } from 'degiro-api'
const { DeGiroActions, DeGiroMarketOrderTypes, DeGiroTimeTypes } = DeGiroEnums
const { OrderType } = DeGiroTypes
(async () => {
const degiro: DeGiro = new DeGiro({
username: 'nachoogoomezomg',
pwd: <string>process.env.DEGIRO_PWD,
})
await degiro.login()
const order: OrderType = {
buySell: DeGiroActions.BUY,
orderType: DeGiroMarketOrderTypes.LIMITED,
productId: '331868', // $AAPL - Apple Inc
size: 1,
timeType: DeGiroTimeTypes.DAY,
price: 272, // limit price
// stopPrice: 2,
}
const { confirmationId, freeSpaceNew, transactionFees } = await degiro.createOrder(order)
const orderId = await degiro.executeOrder(order, confirmationId)
console.log(`Order executed with id: ${orderId}`)
// Wait few seconds to avoid "Rate limit for the given request exceeded" error
const TIMEOUT_SECONDS = 2 * 1000
const deleteOrderFunction = async () => {
try {
await degiro.deleteOrder(orderId)
console.log('Order removed')
} catch (error) {
console.error(error)
}
}
setTimeout(deleteOrderFunction, TIMEOUT_SECONDS)
})()
degiro-cli is an usefull command line interface that help us dealing with DeGiro platform through the terminal. You can see your portfolio status, create and execute orders and much more (may in the future)
$ degiro
Usage: DeGiro Command Line Interface [options] [command]
DeGiro CLI provide you access to DeGiro Broker across the terminal
Options:
-V, --version output the version number
-h, --help display help for command
Commands:
login validate credentials with DeGiro platform
search Search products in DeGiro
portfolio show account portfolio in real-time
help [command] display help for command
MIT
FAQs
Unofficial DeGiro API for Javascript. Buy and sell in the stock market. See your portfolio and much more
The npm package degiro-api receives a total of 16 weekly downloads. As such, degiro-api popularity was classified as not popular.
We found that degiro-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.