delorean.js
Advanced tools
Comparing version 0.5.0 to 0.6.0
{ | ||
"name": "delorean.js", | ||
"version": "0.5.0", | ||
"version": "0.6.0", | ||
"homepage": "http://deloreanjs.com", | ||
@@ -5,0 +5,0 @@ "authors": [ |
@@ -1,20 +0,30 @@ | ||
/*! delorean.js - v0.4.9 - 2014-08-25 */ | ||
/*! delorean.js - v0.5.0-18 - 2014-09-01 */ | ||
(function (DeLorean) { | ||
'use strict'; | ||
var Dispatcher, Store; | ||
// Helper functions | ||
function _generateActionName(name) { | ||
return "action:" + name; | ||
function __hasOwn(object, prop) { | ||
return Object.prototype.hasOwnProperty.call(object, prop); | ||
} | ||
function _hasOwn(object, prop) { | ||
return Object.prototype.hasOwnProperty.call(object, prop); | ||
function __generateActionName(name) { | ||
return 'action:' + name; | ||
} | ||
function _argsShift(args, from) { | ||
function __argsShift(args, from) { | ||
return Array.prototype.slice.call(args, from); | ||
} | ||
function __findDispatcher(view) { | ||
if (!view.props.dispatcher) { | ||
return __findDispatcher(view._owner); | ||
} else { | ||
return view.props.dispatcher; | ||
} | ||
} | ||
// Dispatcher | ||
var Dispatcher = (function () { | ||
Dispatcher = (function () { | ||
function Dispatcher(stores) { | ||
@@ -27,8 +37,8 @@ var self = this; | ||
Dispatcher.prototype.dispatch = function (actionName, data) { | ||
var self = this; | ||
var self = this, stores, deferred; | ||
var stores = (function () { | ||
var stores = []; | ||
stores = (function () { | ||
var stores = [], store; | ||
for (var storeName in self.stores) { | ||
var store = self.stores[storeName]; | ||
store = self.stores[storeName]; | ||
if (!store instanceof Store) { | ||
@@ -42,6 +52,6 @@ throw 'Given store is not a store instance'; | ||
var deferred = this.waitFor(stores); | ||
deferred = this.waitFor(stores); | ||
for (var storeName in self.stores) { | ||
self.stores[storeName].dispatchAction(actionName, data); | ||
}; | ||
} | ||
return deferred; | ||
@@ -51,14 +61,15 @@ }; | ||
Dispatcher.prototype.waitFor = function (stores) { | ||
var self = this; | ||
var promises = (function () { | ||
var _promises = []; | ||
var self = this, promises; | ||
promises = (function () { | ||
var __promises = [], __promiseGenerator, promise; | ||
__promiseGenerator = function (store) { | ||
return new DeLorean.Promise(function (resolve, reject) { | ||
store.listener.once('change', resolve); | ||
}); | ||
}; | ||
for (var i in stores) { | ||
var promise = (function (store) { | ||
return new DeLorean.Promise(function (resolve, reject) { | ||
store.listener.once('change', resolve); | ||
}); | ||
})(stores[i]); | ||
_promises.push(promise); | ||
promise = __promiseGenerator(stores[i]); | ||
__promises.push(promise); | ||
} | ||
return _promises; | ||
return __promises; | ||
}()); | ||
@@ -74,3 +85,3 @@ return DeLorean.Promise.all(promises).then(function () { | ||
} else { | ||
throw 'Action callback should be a function.' | ||
throw 'Action callback should be a function.'; | ||
} | ||
@@ -91,3 +102,3 @@ }; | ||
// Store | ||
var Store = (function () { | ||
Store = (function () { | ||
@@ -103,3 +114,3 @@ function Store(store) { | ||
if (typeof store.initialize === 'function') { | ||
var args = _argsShift(arguments, 1); | ||
var args = __argsShift(arguments, 1); | ||
store.initialize.apply(this.store, args); | ||
@@ -110,2 +121,4 @@ } | ||
Store.prototype.bindActions = function () { | ||
var callback; | ||
this.store.emit = this.listener.emit.bind(this.listener); | ||
@@ -115,8 +128,8 @@ this.store.listenChanges = this.listenChanges.bind(this); | ||
for (var actionName in this.store.actions) { | ||
if (_hasOwn(this.store.actions, actionName)) { | ||
var callback = this.store.actions[actionName]; | ||
if (__hasOwn(this.store.actions, actionName)) { | ||
callback = this.store.actions[actionName]; | ||
if (typeof this.store[callback] !== 'function') { | ||
throw 'Callback should be a method!'; | ||
} | ||
this.listener.on(_generateActionName(actionName), | ||
this.listener.on(__generateActionName(actionName), | ||
this.store[callback].bind(this.store)); | ||
@@ -128,3 +141,3 @@ } | ||
Store.prototype.dispatchAction = function (actionName, data) { | ||
this.listener.emit(_generateActionName(actionName) , data) | ||
this.listener.emit(__generateActionName(actionName), data); | ||
}; | ||
@@ -137,2 +150,3 @@ | ||
Store.prototype.listenChanges = function (object) { | ||
var self = this, observer; | ||
if (!Object.observe) { | ||
@@ -142,5 +156,4 @@ console.error('Store#listenChanges method uses Object.observe, you should fire changes manually.'); | ||
} | ||
var self = this; | ||
var observer = Array.isArray(object) ? Array.observe : Object.observe; | ||
observer = Array.isArray(object) ? Array.observe : Object.observe; | ||
@@ -160,14 +173,16 @@ observer(object, function () { | ||
return new Store(factoryDefinition); | ||
} | ||
}; | ||
}, | ||
createDispatcher: function (actionsToDispatch) { | ||
var actionsOfStores, dispatcher, callback; | ||
if (typeof actionsToDispatch.getStores === 'function') { | ||
var actionsOfStores = actionsToDispatch.getStores(); | ||
actionsOfStores = actionsToDispatch.getStores(); | ||
} | ||
var dispatcher = new Dispatcher(actionsOfStores || {}); | ||
dispatcher = new Dispatcher(actionsOfStores || {}); | ||
for (var actionName in actionsToDispatch) { | ||
if (_hasOwn(actionsToDispatch, actionName)) { | ||
if (__hasOwn(actionsToDispatch, actionName)) { | ||
if (actionName !== 'getStores') { | ||
var callback = actionsToDispatch[actionName]; | ||
callback = actionsToDispatch[actionName]; | ||
dispatcher.registerAction(actionName, callback.bind(dispatcher)); | ||
@@ -198,18 +213,22 @@ } | ||
componentDidMount: function () { | ||
var self = this; | ||
var self = this, store, __changeHandler; | ||
__changeHandler = function (store, storeName) { | ||
return function () { | ||
var state; | ||
// call the components `storeDidChanged` method | ||
if (self.storeDidChange) { | ||
self.storeDidChange(storeName); | ||
} | ||
// change state | ||
if (typeof store.store.getState === 'function') { | ||
state = store.store.getState(); | ||
self.state.stores[storeName] = state; | ||
self.forceUpdate(); | ||
} | ||
}; | ||
}; | ||
for (var storeName in this.stores) { | ||
if (_hasOwn(this.stores, storeName)) { | ||
var store = this.stores[storeName]; | ||
store.onChange(function () { | ||
// call the components `storeDidChanged` method | ||
if (self.storeDidChange) { | ||
self.storeDidChange(storeName); | ||
} | ||
// change state | ||
if (typeof store.store.getState === 'function') { | ||
var state = store.store.getState(); | ||
self.state.stores[storeName] = state; | ||
self.forceUpdate(); | ||
} | ||
}); | ||
if (__hasOwn(this.stores, storeName)) { | ||
store = this.stores[storeName]; | ||
store.onChange(__changeHandler(store, storeName)); | ||
} | ||
@@ -219,13 +238,6 @@ } | ||
getInitialState: function () { | ||
var self = this; | ||
function _findDispatcher(view) { | ||
if (!view.props.dispatcher) { | ||
return _findDispatcher(view._owner); | ||
} else { | ||
return view.props.dispatcher; | ||
} | ||
} | ||
var self = this, state; | ||
// some shortcuts | ||
this.dispatcher = _findDispatcher(this); | ||
this.dispatcher = __findDispatcher(this); | ||
if (this.storesDidChange) { | ||
@@ -239,9 +251,9 @@ this.dispatcher.on('change:all', function () { | ||
var state = {stores: {}}; | ||
state = {stores: {}}; | ||
// more shortcuts for the state | ||
for (var storeName in this.stores) { | ||
if (_hasOwn(this.stores, storeName)) { | ||
if (this.stores[storeName] | ||
&& this.stores[storeName].store | ||
&& this.stores[storeName].store.getState) { | ||
if (__hasOwn(this.stores, storeName)) { | ||
if (this.stores[storeName] && | ||
this.stores[storeName].store && | ||
this.stores[storeName].store.getState) { | ||
state.stores[storeName] = this.stores[storeName].store.getState(); | ||
@@ -263,3 +275,3 @@ } | ||
if (typeof define === 'function' && define.amd) { | ||
define([], function() { | ||
define([], function () { | ||
return DeLorean; | ||
@@ -273,3 +285,3 @@ }); | ||
})({}); | ||
;(function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);throw new Error("Cannot find module '"+o+"'")}var f=n[o]={exports:{}};t[o][0].call(f.exports,function(e){var n=t[o][1][e];return s(n?n:e)},f,f.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s})({1:[function(require,module,exports){ | ||
;(function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s})({1:[function(require,module,exports){ | ||
"use strict"; | ||
@@ -437,4 +449,4 @@ var Promise = require("./promise/promise").Promise; | ||
exports.asap = asap; | ||
}).call(this,require("JkpR2F"),typeof self !== "undefined" ? self : typeof window !== "undefined" ? window : {}) | ||
},{"JkpR2F":12}],4:[function(require,module,exports){ | ||
}).call(this,require('_process'),typeof global !== "undefined" ? global : typeof self !== "undefined" ? self : typeof window !== "undefined" ? window : {}) | ||
},{"_process":12}],4:[function(require,module,exports){ | ||
"use strict"; | ||
@@ -495,3 +507,3 @@ var config = { | ||
exports.polyfill = polyfill; | ||
}).call(this,typeof self !== "undefined" ? self : typeof window !== "undefined" ? window : {}) | ||
}).call(this,typeof global !== "undefined" ? global : typeof self !== "undefined" ? self : typeof window !== "undefined" ? window : {}) | ||
},{"./promise":6,"./utils":10}],6:[function(require,module,exports){ | ||
@@ -1262,2 +1274,2 @@ "use strict"; | ||
},{"es6-promise":1,"events":11}]},{},[13]); | ||
},{"es6-promise":1,"events":11}]},{},[13]); |
@@ -1,2 +0,2 @@ | ||
!function(DeLorean){"use strict";function a(a){return"action:"+a}function b(a,b){return Object.prototype.hasOwnProperty.call(a,b)}function c(a,b){return Array.prototype.slice.call(a,b)}var Dispatcher=function(){function Dispatcher(a){this.listener=new DeLorean.EventEmitter,this.stores=a}return Dispatcher.prototype.dispatch=function(a,b){var c=this,d=function(){var a=[];for(var b in c.stores){var d=c.stores[b];if(!d instanceof Store)throw"Given store is not a store instance";a.push(d)}return a}(),e=this.waitFor(d);for(var f in c.stores)c.stores[f].dispatchAction(a,b);return e},Dispatcher.prototype.waitFor=function(a){var b=this,c=function(){var b=[];for(var c in a){var d=function(a){return new DeLorean.Promise(function(b){a.listener.once("change",b)})}(a[c]);b.push(d)}return b}();return DeLorean.Promise.all(c).then(function(){b.listener.emit("change:all")})},Dispatcher.prototype.registerAction=function(a,b){if("function"!=typeof b)throw"Action callback should be a function.";this[a]=b.bind(this.stores)},Dispatcher.prototype.on=function(){return this.listener.on.apply(this.listener,arguments)},Dispatcher.prototype.off=function(){return this.listener.removeListener.apply(this.listener,arguments)},Dispatcher}(),Store=function(){function Store(a){if("object"!=typeof a)throw"Stores should be defined by passing the definition to the constructor";if(this.listener=new DeLorean.EventEmitter,this.store=a,this.bindActions(),"function"==typeof a.initialize){var b=c(arguments,1);a.initialize.apply(this.store,b)}}return Store.prototype.bindActions=function(){this.store.emit=this.listener.emit.bind(this.listener),this.store.listenChanges=this.listenChanges.bind(this);for(var c in this.store.actions)if(b(this.store.actions,c)){var d=this.store.actions[c];if("function"!=typeof this.store[d])throw"Callback should be a method!";this.listener.on(a(c),this.store[d].bind(this.store))}},Store.prototype.dispatchAction=function(b,c){this.listener.emit(a(b),c)},Store.prototype.onChange=function(a){this.listener.on("change",a)},Store.prototype.listenChanges=function(a){if(!Object.observe)return void console.error("Store#listenChanges method uses Object.observe, you should fire changes manually.");var b=this,c=Array.isArray(a)?Array.observe:Object.observe;c(a,function(){b.listener.emit("change")})},Store}();DeLorean.Flux={createStore:function(a){return function(){return new Store(a)}},createDispatcher:function(a){if("function"==typeof a.getStores)var c=a.getStores();var d=new Dispatcher(c||{});for(var e in a)if(b(a,e)&&"getStores"!==e){var f=a[e];d.registerAction(e,f.bind(d))}return d},define:function(a,b){DeLorean[a]=b}},DeLorean.Dispatcher=Dispatcher,DeLorean.Store=Store,DeLorean.Flux.mixins={storeListener:{componentDidMount:function(){var a=this;for(var c in this.stores)if(b(this.stores,c)){var d=this.stores[c];d.onChange(function(){if(a.storeDidChange&&a.storeDidChange(c),"function"==typeof d.store.getState){var b=d.store.getState();a.state.stores[c]=b,a.forceUpdate()}})}},getInitialState:function(){function a(b){return b.props.dispatcher?b.props.dispatcher:a(b._owner)}var c=this;this.dispatcher=a(this),this.storesDidChange&&this.dispatcher.on("change:all",function(){c.storesDidChange()}),this.stores=this.dispatcher.stores;var d={stores:{}};for(var e in this.stores)b(this.stores,e)&&this.stores[e]&&this.stores[e].store&&this.stores[e].store.getState&&(d.stores[e]=this.stores[e].store.getState());return d}}},"undefined"!=typeof module&&"undefined"!=typeof module.exports?(DeLorean.Flux.define("EventEmitter",require("events").EventEmitter),DeLorean.Flux.define("Promise",require("es6-promise").Promise),module.exports=DeLorean):"function"==typeof define&&define.amd?define([],function(){return DeLorean}):window.DeLorean=DeLorean}({}),function a(b,c,d){function e(g,h){if(!c[g]){if(!b[g]){var i="function"==typeof require&&require;if(!h&&i)return i(g,!0);if(f)return f(g,!0);throw new Error("Cannot find module '"+g+"'")}var j=c[g]={exports:{}};b[g][0].call(j.exports,function(a){var c=b[g][1][a];return e(c?c:a)},j,j.exports,a,b,c,d)}return c[g].exports}for(var f="function"==typeof require&&require,g=0;g<d.length;g++)e(d[g]);return e}({1:[function(a,b,c){"use strict";var d=a("./promise/promise").Promise,e=a("./promise/polyfill").polyfill;c.Promise=d,c.polyfill=e},{"./promise/polyfill":5,"./promise/promise":6}],2:[function(a,b,c){"use strict";function d(a){var b=this;if(!e(a))throw new TypeError("You must pass an array to all.");return new b(function(b,c){function d(a){return function(b){e(a,b)}}function e(a,c){h[a]=c,0===--i&&b(h)}var g,h=[],i=a.length;0===i&&b([]);for(var j=0;j<a.length;j++)g=a[j],g&&f(g.then)?g.then(d(j),c):e(j,g)})}var e=a("./utils").isArray,f=a("./utils").isFunction;c.all=d},{"./utils":10}],3:[function(a,b,c){(function(a,b){"use strict";function d(){return function(){a.nextTick(g)}}function e(){var a=0,b=new k(g),c=document.createTextNode("");return b.observe(c,{characterData:!0}),function(){c.data=a=++a%2}}function f(){return function(){l.setTimeout(g,1)}}function g(){for(var a=0;a<m.length;a++){var b=m[a],c=b[0],d=b[1];c(d)}m=[]}function h(a,b){var c=m.push([a,b]);1===c&&i()}var i,j="undefined"!=typeof window?window:{},k=j.MutationObserver||j.WebKitMutationObserver,l="undefined"!=typeof b?b:void 0===this?window:this,m=[];i="undefined"!=typeof a&&"[object process]"==={}.toString.call(a)?d():k?e():f(),c.asap=h}).call(this,a("JkpR2F"),"undefined"!=typeof self?self:"undefined"!=typeof window?window:{})},{JkpR2F:12}],4:[function(a,b,c){"use strict";function d(a,b){return 2!==arguments.length?e[a]:void(e[a]=b)}var e={instrument:!1};c.config=e,c.configure=d},{}],5:[function(a,b,c){(function(b){"use strict";function d(){var a;a="undefined"!=typeof b?b:"undefined"!=typeof window&&window.document?window:self;var c="Promise"in a&&"resolve"in a.Promise&&"reject"in a.Promise&&"all"in a.Promise&&"race"in a.Promise&&function(){var b;return new a.Promise(function(a){b=a}),f(b)}();c||(a.Promise=e)}var e=a("./promise").Promise,f=a("./utils").isFunction;c.polyfill=d}).call(this,"undefined"!=typeof self?self:"undefined"!=typeof window?window:{})},{"./promise":6,"./utils":10}],6:[function(a,b,c){"use strict";function d(a){if(!q(a))throw new TypeError("You must pass a resolver function as the first argument to the promise constructor");if(!(this instanceof d))throw new TypeError("Failed to construct 'Promise': Please use the 'new' operator, this object constructor cannot be called as a function.");this._subscribers=[],e(a,this)}function e(a,b){function c(a){j(b,a)}function d(a){l(b,a)}try{a(c,d)}catch(e){d(e)}}function f(a,b,c,d){var e,f,g,h,k=q(c);if(k)try{e=c(d),g=!0}catch(m){h=!0,f=m}else e=d,g=!0;i(b,e)||(k&&g?j(b,e):h?l(b,f):a===y?j(b,e):a===z&&l(b,e))}function g(a,b,c,d){var e=a._subscribers,f=e.length;e[f]=b,e[f+y]=c,e[f+z]=d}function h(a,b){for(var c,d,e=a._subscribers,g=a._detail,h=0;h<e.length;h+=3)c=e[h],d=e[h+b],f(b,c,d,g);a._subscribers=null}function i(a,b){var c,d=null;try{if(a===b)throw new TypeError("A promises callback cannot return that same promise.");if(p(b)&&(d=b.then,q(d)))return d.call(b,function(d){return c?!0:(c=!0,void(b!==d?j(a,d):k(a,d)))},function(b){return c?!0:(c=!0,void l(a,b))}),!0}catch(e){return c?!0:(l(a,e),!0)}return!1}function j(a,b){a===b?k(a,b):i(a,b)||k(a,b)}function k(a,b){a._state===w&&(a._state=x,a._detail=b,o.async(m,a))}function l(a,b){a._state===w&&(a._state=x,a._detail=b,o.async(n,a))}function m(a){h(a,a._state=y)}function n(a){h(a,a._state=z)}var o=a("./config").config,p=(a("./config").configure,a("./utils").objectOrFunction),q=a("./utils").isFunction,r=(a("./utils").now,a("./all").all),s=a("./race").race,t=a("./resolve").resolve,u=a("./reject").reject,v=a("./asap").asap;o.async=v;var w=void 0,x=0,y=1,z=2;d.prototype={constructor:d,_state:void 0,_detail:void 0,_subscribers:void 0,then:function(a,b){var c=this,d=new this.constructor(function(){});if(this._state){var e=arguments;o.async(function(){f(c._state,d,e[c._state-1],c._detail)})}else g(this,d,a,b);return d},"catch":function(a){return this.then(null,a)}},d.all=r,d.race=s,d.resolve=t,d.reject=u,c.Promise=d},{"./all":2,"./asap":3,"./config":4,"./race":7,"./reject":8,"./resolve":9,"./utils":10}],7:[function(a,b,c){"use strict";function d(a){var b=this;if(!e(a))throw new TypeError("You must pass an array to race.");return new b(function(b,c){for(var d,e=0;e<a.length;e++)d=a[e],d&&"function"==typeof d.then?d.then(b,c):b(d)})}var e=a("./utils").isArray;c.race=d},{"./utils":10}],8:[function(a,b,c){"use strict";function d(a){var b=this;return new b(function(b,c){c(a)})}c.reject=d},{}],9:[function(a,b,c){"use strict";function d(a){if(a&&"object"==typeof a&&a.constructor===this)return a;var b=this;return new b(function(b){b(a)})}c.resolve=d},{}],10:[function(a,b,c){"use strict";function d(a){return e(a)||"object"==typeof a&&null!==a}function e(a){return"function"==typeof a}function f(a){return"[object Array]"===Object.prototype.toString.call(a)}var g=Date.now||function(){return(new Date).getTime()};c.objectOrFunction=d,c.isFunction=e,c.isArray=f,c.now=g},{}],11:[function(a,b){function c(){this._events=this._events||{},this._maxListeners=this._maxListeners||void 0}function d(a){return"function"==typeof a}function e(a){return"number"==typeof a}function f(a){return"object"==typeof a&&null!==a}function g(a){return void 0===a}b.exports=c,c.EventEmitter=c,c.prototype._events=void 0,c.prototype._maxListeners=void 0,c.defaultMaxListeners=10,c.prototype.setMaxListeners=function(a){if(!e(a)||0>a||isNaN(a))throw TypeError("n must be a positive number");return this._maxListeners=a,this},c.prototype.emit=function(a){var b,c,e,h,i,j;if(this._events||(this._events={}),"error"===a&&(!this._events.error||f(this._events.error)&&!this._events.error.length))throw b=arguments[1],b instanceof Error?b:TypeError('Uncaught, unspecified "error" event.');if(c=this._events[a],g(c))return!1;if(d(c))switch(arguments.length){case 1:c.call(this);break;case 2:c.call(this,arguments[1]);break;case 3:c.call(this,arguments[1],arguments[2]);break;default:for(e=arguments.length,h=new Array(e-1),i=1;e>i;i++)h[i-1]=arguments[i];c.apply(this,h)}else if(f(c)){for(e=arguments.length,h=new Array(e-1),i=1;e>i;i++)h[i-1]=arguments[i];for(j=c.slice(),e=j.length,i=0;e>i;i++)j[i].apply(this,h)}return!0},c.prototype.addListener=function(a,b){var e;if(!d(b))throw TypeError("listener must be a function");if(this._events||(this._events={}),this._events.newListener&&this.emit("newListener",a,d(b.listener)?b.listener:b),this._events[a]?f(this._events[a])?this._events[a].push(b):this._events[a]=[this._events[a],b]:this._events[a]=b,f(this._events[a])&&!this._events[a].warned){var e;e=g(this._maxListeners)?c.defaultMaxListeners:this._maxListeners,e&&e>0&&this._events[a].length>e&&(this._events[a].warned=!0,console.error("(node) warning: possible EventEmitter memory leak detected. %d listeners added. Use emitter.setMaxListeners() to increase limit.",this._events[a].length),"function"==typeof console.trace&&console.trace())}return this},c.prototype.on=c.prototype.addListener,c.prototype.once=function(a,b){function c(){this.removeListener(a,c),e||(e=!0,b.apply(this,arguments))}if(!d(b))throw TypeError("listener must be a function");var e=!1;return c.listener=b,this.on(a,c),this},c.prototype.removeListener=function(a,b){var c,e,g,h;if(!d(b))throw TypeError("listener must be a function");if(!this._events||!this._events[a])return this;if(c=this._events[a],g=c.length,e=-1,c===b||d(c.listener)&&c.listener===b)delete this._events[a],this._events.removeListener&&this.emit("removeListener",a,b);else if(f(c)){for(h=g;h-->0;)if(c[h]===b||c[h].listener&&c[h].listener===b){e=h;break}if(0>e)return this;1===c.length?(c.length=0,delete this._events[a]):c.splice(e,1),this._events.removeListener&&this.emit("removeListener",a,b)}return this},c.prototype.removeAllListeners=function(a){var b,c;if(!this._events)return this;if(!this._events.removeListener)return 0===arguments.length?this._events={}:this._events[a]&&delete this._events[a],this;if(0===arguments.length){for(b in this._events)"removeListener"!==b&&this.removeAllListeners(b);return this.removeAllListeners("removeListener"),this._events={},this}if(c=this._events[a],d(c))this.removeListener(a,c);else for(;c.length;)this.removeListener(a,c[c.length-1]);return delete this._events[a],this},c.prototype.listeners=function(a){var b;return b=this._events&&this._events[a]?d(this._events[a])?[this._events[a]]:this._events[a].slice():[]},c.listenerCount=function(a,b){var c;return c=a._events&&a._events[b]?d(a._events[b])?1:a._events[b].length:0}},{}],12:[function(a,b){function c(){}var d=b.exports={};d.nextTick=function(){var a="undefined"!=typeof window&&window.setImmediate,b="undefined"!=typeof window&&window.postMessage&&window.addEventListener;if(a)return function(a){return window.setImmediate(a)};if(b){var c=[];return window.addEventListener("message",function(a){var b=a.source;if((b===window||null===b)&&"process-tick"===a.data&&(a.stopPropagation(),c.length>0)){var d=c.shift();d()}},!0),function(a){c.push(a),window.postMessage("process-tick","*")}}return function(a){setTimeout(a,0)}}(),d.title="browser",d.browser=!0,d.env={},d.argv=[],d.on=c,d.addListener=c,d.once=c,d.off=c,d.removeListener=c,d.removeAllListeners=c,d.emit=c,d.binding=function(){throw new Error("process.binding is not supported")},d.cwd=function(){return"/"},d.chdir=function(){throw new Error("process.chdir is not supported")}},{}],13:[function(a){DeLorean.Flux.define("EventEmitter",a("events").EventEmitter),DeLorean.Flux.define("Promise",a("es6-promise").Promise)},{"es6-promise":1,events:11}]},{},[13]); | ||
!function(DeLorean){"use strict";function a(a,b){return Object.prototype.hasOwnProperty.call(a,b)}function b(a){return"action:"+a}function c(a,b){return Array.prototype.slice.call(a,b)}function d(a){return a.props.dispatcher?a.props.dispatcher:d(a._owner)}var Dispatcher,Store;Dispatcher=function(){function Dispatcher(a){this.listener=new DeLorean.EventEmitter,this.stores=a}return Dispatcher.prototype.dispatch=function(a,b){var c,d,e=this;c=function(){var a,b=[];for(var c in e.stores){if(a=e.stores[c],!a instanceof Store)throw"Given store is not a store instance";b.push(a)}return b}(),d=this.waitFor(c);for(var f in e.stores)e.stores[f].dispatchAction(a,b);return d},Dispatcher.prototype.waitFor=function(a){var b,c=this;return b=function(){var b,c,d=[];b=function(a){return new DeLorean.Promise(function(b){a.listener.once("change",b)})};for(var e in a)c=b(a[e]),d.push(c);return d}(),DeLorean.Promise.all(b).then(function(){c.listener.emit("change:all")})},Dispatcher.prototype.registerAction=function(a,b){if("function"!=typeof b)throw"Action callback should be a function.";this[a]=b.bind(this.stores)},Dispatcher.prototype.on=function(){return this.listener.on.apply(this.listener,arguments)},Dispatcher.prototype.off=function(){return this.listener.removeListener.apply(this.listener,arguments)},Dispatcher}(),Store=function(){function Store(a){if("object"!=typeof a)throw"Stores should be defined by passing the definition to the constructor";if(this.listener=new DeLorean.EventEmitter,this.store=a,this.bindActions(),"function"==typeof a.initialize){var b=c(arguments,1);a.initialize.apply(this.store,b)}}return Store.prototype.bindActions=function(){var c;this.store.emit=this.listener.emit.bind(this.listener),this.store.listenChanges=this.listenChanges.bind(this);for(var d in this.store.actions)if(a(this.store.actions,d)){if(c=this.store.actions[d],"function"!=typeof this.store[c])throw"Callback should be a method!";this.listener.on(b(d),this.store[c].bind(this.store))}},Store.prototype.dispatchAction=function(a,c){this.listener.emit(b(a),c)},Store.prototype.onChange=function(a){this.listener.on("change",a)},Store.prototype.listenChanges=function(a){var b,c=this;return Object.observe?(b=Array.isArray(a)?Array.observe:Object.observe,void b(a,function(){c.listener.emit("change")})):void console.error("Store#listenChanges method uses Object.observe, you should fire changes manually.")},Store}(),DeLorean.Flux={createStore:function(a){return function(){return new Store(a)}},createDispatcher:function(b){var c,d,e;"function"==typeof b.getStores&&(c=b.getStores()),d=new Dispatcher(c||{});for(var f in b)a(b,f)&&"getStores"!==f&&(e=b[f],d.registerAction(f,e.bind(d)));return d},define:function(a,b){DeLorean[a]=b}},DeLorean.Dispatcher=Dispatcher,DeLorean.Store=Store,DeLorean.Flux.mixins={storeListener:{componentDidMount:function(){var b,c,d=this;c=function(a,b){return function(){var c;d.storeDidChange&&d.storeDidChange(b),"function"==typeof a.store.getState&&(c=a.store.getState(),d.state.stores[b]=c,d.forceUpdate())}};for(var e in this.stores)a(this.stores,e)&&(b=this.stores[e],b.onChange(c(b,e)))},getInitialState:function(){var b,c=this;this.dispatcher=d(this),this.storesDidChange&&this.dispatcher.on("change:all",function(){c.storesDidChange()}),this.stores=this.dispatcher.stores,b={stores:{}};for(var e in this.stores)a(this.stores,e)&&this.stores[e]&&this.stores[e].store&&this.stores[e].store.getState&&(b.stores[e]=this.stores[e].store.getState());return b}}},"undefined"!=typeof module&&"undefined"!=typeof module.exports?(DeLorean.Flux.define("EventEmitter",require("events").EventEmitter),DeLorean.Flux.define("Promise",require("es6-promise").Promise),module.exports=DeLorean):"function"==typeof define&&define.amd?define([],function(){return DeLorean}):window.DeLorean=DeLorean}({}),function a(b,c,d){function e(g,h){if(!c[g]){if(!b[g]){var i="function"==typeof require&&require;if(!h&&i)return i(g,!0);if(f)return f(g,!0);var j=new Error("Cannot find module '"+g+"'");throw j.code="MODULE_NOT_FOUND",j}var k=c[g]={exports:{}};b[g][0].call(k.exports,function(a){var c=b[g][1][a];return e(c?c:a)},k,k.exports,a,b,c,d)}return c[g].exports}for(var f="function"==typeof require&&require,g=0;g<d.length;g++)e(d[g]);return e}({1:[function(a,b,c){"use strict";var d=a("./promise/promise").Promise,e=a("./promise/polyfill").polyfill;c.Promise=d,c.polyfill=e},{"./promise/polyfill":5,"./promise/promise":6}],2:[function(a,b,c){"use strict";function d(a){var b=this;if(!e(a))throw new TypeError("You must pass an array to all.");return new b(function(b,c){function d(a){return function(b){e(a,b)}}function e(a,c){h[a]=c,0===--i&&b(h)}var g,h=[],i=a.length;0===i&&b([]);for(var j=0;j<a.length;j++)g=a[j],g&&f(g.then)?g.then(d(j),c):e(j,g)})}var e=a("./utils").isArray,f=a("./utils").isFunction;c.all=d},{"./utils":10}],3:[function(a,b,c){(function(a,b){"use strict";function d(){return function(){a.nextTick(g)}}function e(){var a=0,b=new k(g),c=document.createTextNode("");return b.observe(c,{characterData:!0}),function(){c.data=a=++a%2}}function f(){return function(){l.setTimeout(g,1)}}function g(){for(var a=0;a<m.length;a++){var b=m[a],c=b[0],d=b[1];c(d)}m=[]}function h(a,b){var c=m.push([a,b]);1===c&&i()}var i,j="undefined"!=typeof window?window:{},k=j.MutationObserver||j.WebKitMutationObserver,l="undefined"!=typeof b?b:void 0===this?window:this,m=[];i="undefined"!=typeof a&&"[object process]"==={}.toString.call(a)?d():k?e():f(),c.asap=h}).call(this,a("_process"),"undefined"!=typeof global?global:"undefined"!=typeof self?self:"undefined"!=typeof window?window:{})},{_process:12}],4:[function(a,b,c){"use strict";function d(a,b){return 2!==arguments.length?e[a]:void(e[a]=b)}var e={instrument:!1};c.config=e,c.configure=d},{}],5:[function(a,b,c){(function(b){"use strict";function d(){var a;a="undefined"!=typeof b?b:"undefined"!=typeof window&&window.document?window:self;var c="Promise"in a&&"resolve"in a.Promise&&"reject"in a.Promise&&"all"in a.Promise&&"race"in a.Promise&&function(){var b;return new a.Promise(function(a){b=a}),f(b)}();c||(a.Promise=e)}var e=a("./promise").Promise,f=a("./utils").isFunction;c.polyfill=d}).call(this,"undefined"!=typeof global?global:"undefined"!=typeof self?self:"undefined"!=typeof window?window:{})},{"./promise":6,"./utils":10}],6:[function(a,b,c){"use strict";function d(a){if(!q(a))throw new TypeError("You must pass a resolver function as the first argument to the promise constructor");if(!(this instanceof d))throw new TypeError("Failed to construct 'Promise': Please use the 'new' operator, this object constructor cannot be called as a function.");this._subscribers=[],e(a,this)}function e(a,b){function c(a){j(b,a)}function d(a){l(b,a)}try{a(c,d)}catch(e){d(e)}}function f(a,b,c,d){var e,f,g,h,k=q(c);if(k)try{e=c(d),g=!0}catch(m){h=!0,f=m}else e=d,g=!0;i(b,e)||(k&&g?j(b,e):h?l(b,f):a===y?j(b,e):a===z&&l(b,e))}function g(a,b,c,d){var e=a._subscribers,f=e.length;e[f]=b,e[f+y]=c,e[f+z]=d}function h(a,b){for(var c,d,e=a._subscribers,g=a._detail,h=0;h<e.length;h+=3)c=e[h],d=e[h+b],f(b,c,d,g);a._subscribers=null}function i(a,b){var c,d=null;try{if(a===b)throw new TypeError("A promises callback cannot return that same promise.");if(p(b)&&(d=b.then,q(d)))return d.call(b,function(d){return c?!0:(c=!0,void(b!==d?j(a,d):k(a,d)))},function(b){return c?!0:(c=!0,void l(a,b))}),!0}catch(e){return c?!0:(l(a,e),!0)}return!1}function j(a,b){a===b?k(a,b):i(a,b)||k(a,b)}function k(a,b){a._state===w&&(a._state=x,a._detail=b,o.async(m,a))}function l(a,b){a._state===w&&(a._state=x,a._detail=b,o.async(n,a))}function m(a){h(a,a._state=y)}function n(a){h(a,a._state=z)}var o=a("./config").config,p=(a("./config").configure,a("./utils").objectOrFunction),q=a("./utils").isFunction,r=(a("./utils").now,a("./all").all),s=a("./race").race,t=a("./resolve").resolve,u=a("./reject").reject,v=a("./asap").asap;o.async=v;var w=void 0,x=0,y=1,z=2;d.prototype={constructor:d,_state:void 0,_detail:void 0,_subscribers:void 0,then:function(a,b){var c=this,d=new this.constructor(function(){});if(this._state){var e=arguments;o.async(function(){f(c._state,d,e[c._state-1],c._detail)})}else g(this,d,a,b);return d},"catch":function(a){return this.then(null,a)}},d.all=r,d.race=s,d.resolve=t,d.reject=u,c.Promise=d},{"./all":2,"./asap":3,"./config":4,"./race":7,"./reject":8,"./resolve":9,"./utils":10}],7:[function(a,b,c){"use strict";function d(a){var b=this;if(!e(a))throw new TypeError("You must pass an array to race.");return new b(function(b,c){for(var d,e=0;e<a.length;e++)d=a[e],d&&"function"==typeof d.then?d.then(b,c):b(d)})}var e=a("./utils").isArray;c.race=d},{"./utils":10}],8:[function(a,b,c){"use strict";function d(a){var b=this;return new b(function(b,c){c(a)})}c.reject=d},{}],9:[function(a,b,c){"use strict";function d(a){if(a&&"object"==typeof a&&a.constructor===this)return a;var b=this;return new b(function(b){b(a)})}c.resolve=d},{}],10:[function(a,b,c){"use strict";function d(a){return e(a)||"object"==typeof a&&null!==a}function e(a){return"function"==typeof a}function f(a){return"[object Array]"===Object.prototype.toString.call(a)}var g=Date.now||function(){return(new Date).getTime()};c.objectOrFunction=d,c.isFunction=e,c.isArray=f,c.now=g},{}],11:[function(a,b){function c(){this._events=this._events||{},this._maxListeners=this._maxListeners||void 0}function d(a){return"function"==typeof a}function e(a){return"number"==typeof a}function f(a){return"object"==typeof a&&null!==a}function g(a){return void 0===a}b.exports=c,c.EventEmitter=c,c.prototype._events=void 0,c.prototype._maxListeners=void 0,c.defaultMaxListeners=10,c.prototype.setMaxListeners=function(a){if(!e(a)||0>a||isNaN(a))throw TypeError("n must be a positive number");return this._maxListeners=a,this},c.prototype.emit=function(a){var b,c,e,h,i,j;if(this._events||(this._events={}),"error"===a&&(!this._events.error||f(this._events.error)&&!this._events.error.length))throw b=arguments[1],b instanceof Error?b:TypeError('Uncaught, unspecified "error" event.');if(c=this._events[a],g(c))return!1;if(d(c))switch(arguments.length){case 1:c.call(this);break;case 2:c.call(this,arguments[1]);break;case 3:c.call(this,arguments[1],arguments[2]);break;default:for(e=arguments.length,h=new Array(e-1),i=1;e>i;i++)h[i-1]=arguments[i];c.apply(this,h)}else if(f(c)){for(e=arguments.length,h=new Array(e-1),i=1;e>i;i++)h[i-1]=arguments[i];for(j=c.slice(),e=j.length,i=0;e>i;i++)j[i].apply(this,h)}return!0},c.prototype.addListener=function(a,b){var e;if(!d(b))throw TypeError("listener must be a function");if(this._events||(this._events={}),this._events.newListener&&this.emit("newListener",a,d(b.listener)?b.listener:b),this._events[a]?f(this._events[a])?this._events[a].push(b):this._events[a]=[this._events[a],b]:this._events[a]=b,f(this._events[a])&&!this._events[a].warned){var e;e=g(this._maxListeners)?c.defaultMaxListeners:this._maxListeners,e&&e>0&&this._events[a].length>e&&(this._events[a].warned=!0,console.error("(node) warning: possible EventEmitter memory leak detected. %d listeners added. Use emitter.setMaxListeners() to increase limit.",this._events[a].length),"function"==typeof console.trace&&console.trace())}return this},c.prototype.on=c.prototype.addListener,c.prototype.once=function(a,b){function c(){this.removeListener(a,c),e||(e=!0,b.apply(this,arguments))}if(!d(b))throw TypeError("listener must be a function");var e=!1;return c.listener=b,this.on(a,c),this},c.prototype.removeListener=function(a,b){var c,e,g,h;if(!d(b))throw TypeError("listener must be a function");if(!this._events||!this._events[a])return this;if(c=this._events[a],g=c.length,e=-1,c===b||d(c.listener)&&c.listener===b)delete this._events[a],this._events.removeListener&&this.emit("removeListener",a,b);else if(f(c)){for(h=g;h-->0;)if(c[h]===b||c[h].listener&&c[h].listener===b){e=h;break}if(0>e)return this;1===c.length?(c.length=0,delete this._events[a]):c.splice(e,1),this._events.removeListener&&this.emit("removeListener",a,b)}return this},c.prototype.removeAllListeners=function(a){var b,c;if(!this._events)return this;if(!this._events.removeListener)return 0===arguments.length?this._events={}:this._events[a]&&delete this._events[a],this;if(0===arguments.length){for(b in this._events)"removeListener"!==b&&this.removeAllListeners(b);return this.removeAllListeners("removeListener"),this._events={},this}if(c=this._events[a],d(c))this.removeListener(a,c);else for(;c.length;)this.removeListener(a,c[c.length-1]);return delete this._events[a],this},c.prototype.listeners=function(a){var b;return b=this._events&&this._events[a]?d(this._events[a])?[this._events[a]]:this._events[a].slice():[]},c.listenerCount=function(a,b){var c;return c=a._events&&a._events[b]?d(a._events[b])?1:a._events[b].length:0}},{}],12:[function(a,b){function c(){}var d=b.exports={};d.nextTick=function(){var a="undefined"!=typeof window&&window.setImmediate,b="undefined"!=typeof window&&window.postMessage&&window.addEventListener;if(a)return function(a){return window.setImmediate(a)};if(b){var c=[];return window.addEventListener("message",function(a){var b=a.source;if((b===window||null===b)&&"process-tick"===a.data&&(a.stopPropagation(),c.length>0)){var d=c.shift();d()}},!0),function(a){c.push(a),window.postMessage("process-tick","*")}}return function(a){setTimeout(a,0)}}(),d.title="browser",d.browser=!0,d.env={},d.argv=[],d.on=c,d.addListener=c,d.once=c,d.off=c,d.removeListener=c,d.removeAllListeners=c,d.emit=c,d.binding=function(){throw new Error("process.binding is not supported")},d.cwd=function(){return"/"},d.chdir=function(){throw new Error("process.chdir is not supported")}},{}],13:[function(a){DeLorean.Flux.define("EventEmitter",a("events").EventEmitter),DeLorean.Flux.define("Promise",a("es6-promise").Promise)},{"es6-promise":1,events:11}]},{},[13]); | ||
//# sourceMappingURL=delorean.min.js.map |
@@ -5,3 +5,3 @@ ## What is Flux | ||
 | ||
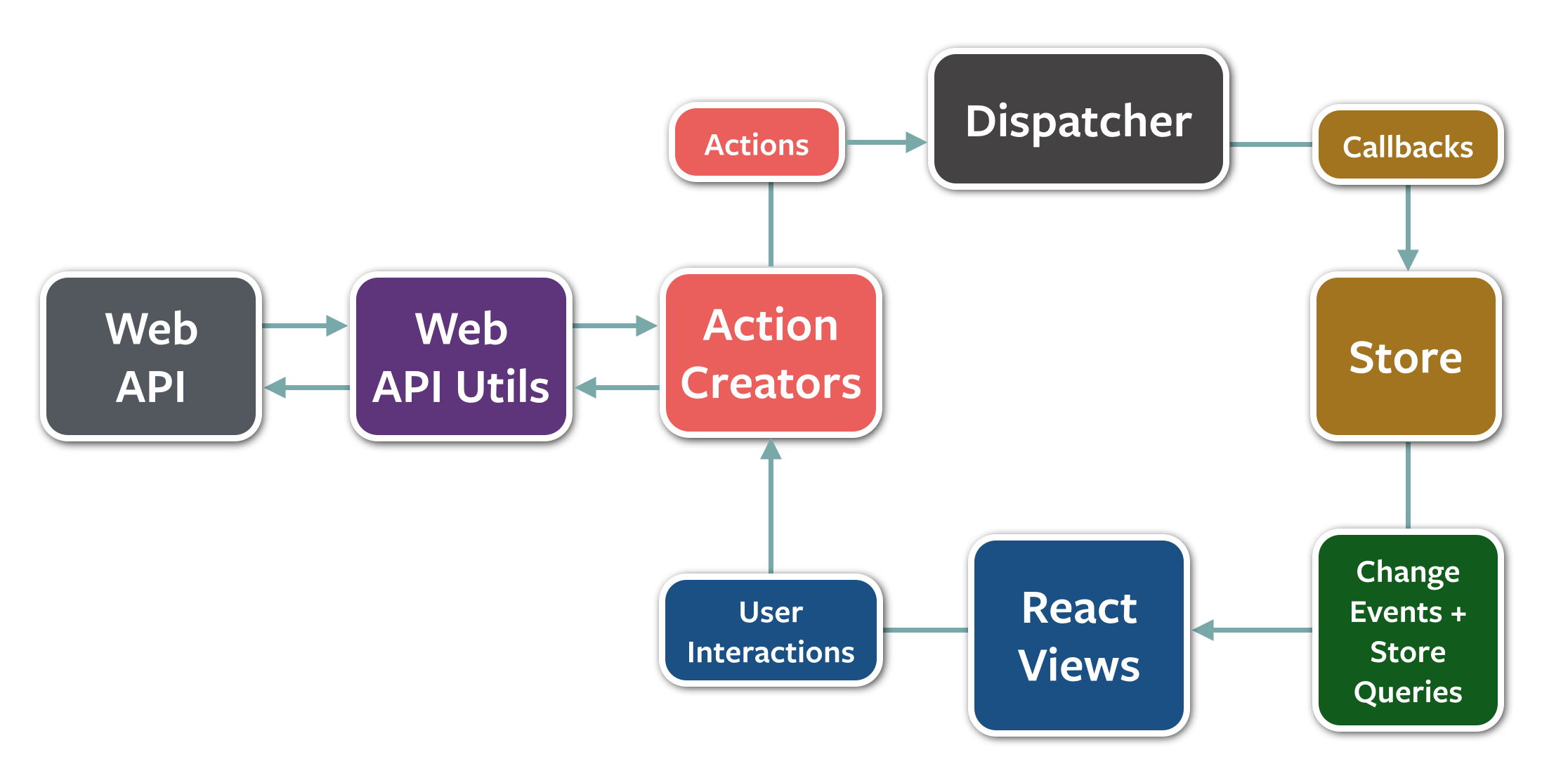 | ||
@@ -12,1 +12,3 @@ - [**Store**: A postbox](./store.md) | ||
- [**Action Creator**: The post office, manages postmans](./actions.md) | ||
[Read the tutorial now.](./tutorial.md) |
@@ -1,1 +0,126 @@ | ||
## Soon... | ||
# Tutorial | ||
You can easily start using **DeLorean.js**. | ||
First of all, let's create the DOM (view) we'll need. | ||
```html | ||
<!doctype html> | ||
<html> | ||
<head> | ||
<script src="//rawgit.com/f/delorean/master/dist/delorean.min.js"></script> | ||
</head> | ||
<body> | ||
<ul id="list"></ul> | ||
<button id="addItem">Add Random Item</button> | ||
<script src="js/app.js"></script> | ||
</body> | ||
</html> | ||
``` | ||
Now, let's create an `app.js` file. | ||
### Step 1: Action Creation | ||
And then let's handle the events: | ||
```javascript | ||
document.getElementById('addItem').onclick = function () { | ||
ActionCreator.addItem(); | ||
}; | ||
``` | ||
Sure, it will create an error when you click the button: | ||
``` | ||
ReferenceError: ActionCreator is not defined | ||
``` | ||
Then let's create an **Action Creator** called `ActionCreator`. Action Creators | ||
are simple JavaScript objects. You can use them as **controllers**. | ||
```javascript | ||
var ActionCreator = { | ||
addItem: function () { | ||
// We'll going to call dispatcher methods. | ||
MyAppDispatcher.addItem({random: Math.random()}); | ||
} | ||
}; | ||
``` | ||
### Step 2: Dispatching Actions to the Stores | ||
But now, we'll need a dispatcher. Now we'll use DeLorean's dispatcher. | ||
```javascript | ||
var MyAppDispatcher = DeLorean.Flux.createDispatcher({ | ||
addItem: function (data) { | ||
this.dispatch('addItem', data); | ||
} | ||
}); | ||
``` | ||
It's now looking like actually another action creator but dispatchers are | ||
units that informs stores one by one. So we need to define the special function | ||
called `getStores`. | ||
```javascript | ||
var MyAppDispatcher = DeLorean.Flux.createDispatcher({ | ||
addItem: function (data) { | ||
this.dispatch('addItem', data); | ||
}, | ||
getStores: function () { | ||
return { | ||
myStore: myStore | ||
} | ||
} | ||
}); | ||
``` | ||
### Step 3: Stores to Manage Data | ||
Now let's create the store. You need to define an object called `actions`. It's | ||
simply forwarders for dispatcher. When a dispatcher connected to the store | ||
dispatches an event, the `actions` object will forward it to the related method | ||
with arguments. | ||
Also you have to call `this.emit('change')` when you update your data. | ||
```javascript | ||
var MyAppStore = DeLorean.Flux.createStore({ | ||
list: [], | ||
actions: { | ||
// Remember the `dispatch('addItem')` | ||
'addItem': 'addItemMethod' | ||
}, | ||
addItemMethod: function (data) { | ||
this.list.push('ITEM: ' + data.random); | ||
// You need to say your store is changed. | ||
this.emit('change'); | ||
} | ||
}); | ||
var myStore = new MyAppStore(); | ||
``` | ||
### Step 4: Completing the Cycle: Views | ||
Now everything seems okay, but **we didn't completed the cycle yet**. | ||
```javascript | ||
var list = document.getElementById('list'); | ||
// Store emits the `change` event when changed. | ||
myStore.onChange(function () { | ||
list.innerHTML = ''; // Best thing for this example. | ||
myStore.store.list.forEach(function (item) { | ||
var listItem = document.createElement('li'); | ||
listItem.innerHTML = item; | ||
list.appendChild(listItem); | ||
}); | ||
}); | ||
``` |
@@ -8,2 +8,4 @@ module.exports = function (grunt) { | ||
grunt.loadNpmTasks('grunt-contrib-watch'); | ||
grunt.loadNpmTasks('grunt-jscs-checker'); | ||
grunt.loadNpmTasks('grunt-contrib-jshint'); | ||
grunt.loadNpmTasks('grunt-release'); | ||
@@ -15,6 +17,19 @@ | ||
jshint: { | ||
all: ['Gruntfile.js', 'src/**/*.js', 'test/**/*.js', '!test/vendor/**/*.js'] | ||
}, | ||
jscs: { | ||
src: ['Gruntfile.js', 'src/**/*.js', 'test/**/*.js', '!test/vendor/**/*.js'], | ||
options: { | ||
config: '.jscsrc', | ||
validateIndentation: 2, | ||
disallowDanglingUnderscores: null, | ||
disallowMultipleVarDecl: null, | ||
requireMultipleVarDecl: null | ||
} | ||
}, | ||
karma: { | ||
unit: { | ||
configFile: 'test/karma.conf.js', | ||
}, | ||
configFile: 'test/karma.conf.js' | ||
} | ||
}, | ||
@@ -36,3 +51,3 @@ browserify: { | ||
src: ['src/delorean.js', 'dist/.tmp/delorean-requires.js'], | ||
dest: 'dist/delorean.js', | ||
dest: 'dist/delorean.js' | ||
} | ||
@@ -68,4 +83,4 @@ }, | ||
livereload: true | ||
}, | ||
}, | ||
} | ||
} | ||
}, | ||
@@ -79,5 +94,5 @@ release: { | ||
grunt.registerTask('default', ['browserify', 'concat', 'uglify']); | ||
grunt.registerTask('default', ['jscs', 'jshint', 'browserify', 'concat', 'uglify']); | ||
grunt.registerTask('dev', ['connect', 'watch']); | ||
}; |
{ | ||
"name": "delorean.js", | ||
"version": "0.5.0", | ||
"version": "0.6.0", | ||
"description": "Flux Library", | ||
@@ -14,6 +14,9 @@ "main": "src/delorean.js", | ||
"grunt-browserify": "^2.1.4", | ||
"grunt-cli": "^0.1.13", | ||
"grunt-contrib-concat": "^0.5.0", | ||
"grunt-contrib-connect": "^0.8.0", | ||
"grunt-contrib-jshint": "^0.10.0", | ||
"grunt-contrib-uglify": "^0.5.1", | ||
"grunt-contrib-watch": "^0.6.1", | ||
"grunt-jscs-checker": "^0.6.2", | ||
"grunt-karma": "^0.8.3", | ||
@@ -20,0 +23,0 @@ "grunt-livereload": "^0.1.3", |
@@ -1,7 +0,6 @@ | ||
 | ||
# DeLorean.js | ||
[](http://badge.fury.io/js/delorean.js) | ||
 | ||
[](https://travis-ci.org/deloreanjs/delorean) | ||
[](http://badge.fury.io/js/delorean.js) | ||
 | ||
@@ -13,9 +12,12 @@ DeLorean is a tiny Flux pattern implementation. | ||
- Makes data more **consistent** in your whole application, | ||
- Too easy to use with **React.js**; just add a mixin, | ||
- Too easy to use with **Flight.js**; see the example | ||
- It's platform agnostic, completely. There's no dependency. | ||
- It's framework agnostic, completely. There's **no view framework dependency**. | ||
- Too small, just **4K** gzipped. | ||
- Built-in **React.js** integration, easy to use with **Flight.js** and **Ractive.js** and probably all others. | ||
## Overview | ||
### Tutorial | ||
You can learn Flux and DeLorean.js in minutes. [Read the tutorial](./docs/tutorial.md) | ||
## Using with Frameworks | ||
- [Try **React.js** example on JSFiddle](http://jsfiddle.net/fkadev/a2ms7rcc/) | ||
@@ -43,8 +45,78 @@ - [Try **Flight.js** example on JSFiddle](http://jsfiddle.net/fkadev/1cw9Leau/) | ||
Hipster way: | ||
```js | ||
var Flux = require('delorean.js').Flux; | ||
// ... | ||
``` | ||
Old-skool way: | ||
```html | ||
<script src="//rawgit.com/f/delorean/master/dist/delorean.min.js"></script> | ||
<script> | ||
var Flux = DeLorean.Flux; | ||
// ... | ||
</script> | ||
``` | ||
## Overview | ||
```javascript | ||
/* | ||
* Stores are simple data buckets which manages data. | ||
*/ | ||
var Store = Flux.createStore({ | ||
data: null, | ||
setData: function (data) { | ||
this.data = data; | ||
this.emit('change'); | ||
}, | ||
actions: { | ||
'incoming-data': 'setData' | ||
} | ||
}); | ||
var store = new Store(); | ||
/* | ||
* Dispatcher are simple action dispatchers for stores. | ||
* Stores handle the related action. | ||
*/ | ||
var Dispatcher = Flux.createDispatcher({ | ||
setData: function (data) { | ||
this.dispatch('incoming-data', data); | ||
}, | ||
getStores: function () { | ||
return {increment: store}; | ||
} | ||
}); | ||
/* | ||
* Action Creators are simple controllers. They are simple functions. | ||
* They talk to dispatchers. They are not required. | ||
*/ | ||
var Actions = { | ||
setData: function (data) { | ||
Dispatcher.setData(data); | ||
} | ||
}; | ||
// The data cycle. | ||
store.onChange(function () { | ||
// End of data cycle. | ||
document.getElementById('result').innerText = store.store.data; | ||
}); | ||
document.getElementById('dataChanger').onclick = function () { | ||
// Start data cycle: | ||
Actions.setData(Math.random()); | ||
}; | ||
``` | ||
[Run this example on JSFiddle](http://jsfiddle.net/fkadev/40cx3146/) | ||
## Docs | ||
You can read the [tutorial](./docs/tutorial.md) to learn how to start using | ||
**DeLorean.js** with your favorite framework. | ||
### Basic Concepts | ||
@@ -76,1 +148,12 @@ | ||
[MIT License](http://f.mit-license.org) | ||
## Authors | ||
- Fatih Kadir Akin ([@f](/f)) | ||
- Burak Can ([@burakcan](/burakcan)) | ||
## Contributors | ||
- Quang Van ([@quangv](/quangv)) | ||
- James H. Edwards ([@incrediblesound](/incrediblesound)) | ||
- Fehmi Can Sağlam ([@fehmicansaglam](/fehmicansaglam)) |
(function (DeLorean) { | ||
'use strict'; | ||
var Dispatcher, Store; | ||
// Helper functions | ||
function _generateActionName(name) { | ||
return "action:" + name; | ||
function __hasOwn(object, prop) { | ||
return Object.prototype.hasOwnProperty.call(object, prop); | ||
} | ||
function _hasOwn(object, prop) { | ||
return Object.prototype.hasOwnProperty.call(object, prop); | ||
function __generateActionName(name) { | ||
return 'action:' + name; | ||
} | ||
function _argsShift(args, from) { | ||
function __argsShift(args, from) { | ||
return Array.prototype.slice.call(args, from); | ||
} | ||
function __findDispatcher(view) { | ||
if (!view.props.dispatcher) { | ||
return __findDispatcher(view._owner); | ||
} else { | ||
return view.props.dispatcher; | ||
} | ||
} | ||
// Dispatcher | ||
var Dispatcher = (function () { | ||
Dispatcher = (function () { | ||
function Dispatcher(stores) { | ||
@@ -26,8 +36,8 @@ var self = this; | ||
Dispatcher.prototype.dispatch = function (actionName, data) { | ||
var self = this; | ||
var self = this, stores, deferred; | ||
var stores = (function () { | ||
var stores = []; | ||
stores = (function () { | ||
var stores = [], store; | ||
for (var storeName in self.stores) { | ||
var store = self.stores[storeName]; | ||
store = self.stores[storeName]; | ||
if (!store instanceof Store) { | ||
@@ -41,6 +51,6 @@ throw 'Given store is not a store instance'; | ||
var deferred = this.waitFor(stores); | ||
deferred = this.waitFor(stores); | ||
for (var storeName in self.stores) { | ||
self.stores[storeName].dispatchAction(actionName, data); | ||
}; | ||
} | ||
return deferred; | ||
@@ -50,14 +60,15 @@ }; | ||
Dispatcher.prototype.waitFor = function (stores) { | ||
var self = this; | ||
var promises = (function () { | ||
var _promises = []; | ||
var self = this, promises; | ||
promises = (function () { | ||
var __promises = [], __promiseGenerator, promise; | ||
__promiseGenerator = function (store) { | ||
return new DeLorean.Promise(function (resolve, reject) { | ||
store.listener.once('change', resolve); | ||
}); | ||
}; | ||
for (var i in stores) { | ||
var promise = (function (store) { | ||
return new DeLorean.Promise(function (resolve, reject) { | ||
store.listener.once('change', resolve); | ||
}); | ||
})(stores[i]); | ||
_promises.push(promise); | ||
promise = __promiseGenerator(stores[i]); | ||
__promises.push(promise); | ||
} | ||
return _promises; | ||
return __promises; | ||
}()); | ||
@@ -73,3 +84,3 @@ return DeLorean.Promise.all(promises).then(function () { | ||
} else { | ||
throw 'Action callback should be a function.' | ||
throw 'Action callback should be a function.'; | ||
} | ||
@@ -90,3 +101,3 @@ }; | ||
// Store | ||
var Store = (function () { | ||
Store = (function () { | ||
@@ -102,3 +113,3 @@ function Store(store) { | ||
if (typeof store.initialize === 'function') { | ||
var args = _argsShift(arguments, 1); | ||
var args = __argsShift(arguments, 1); | ||
store.initialize.apply(this.store, args); | ||
@@ -109,2 +120,4 @@ } | ||
Store.prototype.bindActions = function () { | ||
var callback; | ||
this.store.emit = this.listener.emit.bind(this.listener); | ||
@@ -114,8 +127,8 @@ this.store.listenChanges = this.listenChanges.bind(this); | ||
for (var actionName in this.store.actions) { | ||
if (_hasOwn(this.store.actions, actionName)) { | ||
var callback = this.store.actions[actionName]; | ||
if (__hasOwn(this.store.actions, actionName)) { | ||
callback = this.store.actions[actionName]; | ||
if (typeof this.store[callback] !== 'function') { | ||
throw 'Callback should be a method!'; | ||
} | ||
this.listener.on(_generateActionName(actionName), | ||
this.listener.on(__generateActionName(actionName), | ||
this.store[callback].bind(this.store)); | ||
@@ -127,3 +140,3 @@ } | ||
Store.prototype.dispatchAction = function (actionName, data) { | ||
this.listener.emit(_generateActionName(actionName) , data) | ||
this.listener.emit(__generateActionName(actionName), data); | ||
}; | ||
@@ -136,2 +149,3 @@ | ||
Store.prototype.listenChanges = function (object) { | ||
var self = this, observer; | ||
if (!Object.observe) { | ||
@@ -141,5 +155,4 @@ console.error('Store#listenChanges method uses Object.observe, you should fire changes manually.'); | ||
} | ||
var self = this; | ||
var observer = Array.isArray(object) ? Array.observe : Object.observe; | ||
observer = Array.isArray(object) ? Array.observe : Object.observe; | ||
@@ -159,14 +172,16 @@ observer(object, function () { | ||
return new Store(factoryDefinition); | ||
} | ||
}; | ||
}, | ||
createDispatcher: function (actionsToDispatch) { | ||
var actionsOfStores, dispatcher, callback; | ||
if (typeof actionsToDispatch.getStores === 'function') { | ||
var actionsOfStores = actionsToDispatch.getStores(); | ||
actionsOfStores = actionsToDispatch.getStores(); | ||
} | ||
var dispatcher = new Dispatcher(actionsOfStores || {}); | ||
dispatcher = new Dispatcher(actionsOfStores || {}); | ||
for (var actionName in actionsToDispatch) { | ||
if (_hasOwn(actionsToDispatch, actionName)) { | ||
if (__hasOwn(actionsToDispatch, actionName)) { | ||
if (actionName !== 'getStores') { | ||
var callback = actionsToDispatch[actionName]; | ||
callback = actionsToDispatch[actionName]; | ||
dispatcher.registerAction(actionName, callback.bind(dispatcher)); | ||
@@ -197,18 +212,22 @@ } | ||
componentDidMount: function () { | ||
var self = this; | ||
var self = this, store, __changeHandler; | ||
__changeHandler = function (store, storeName) { | ||
return function () { | ||
var state; | ||
// call the components `storeDidChanged` method | ||
if (self.storeDidChange) { | ||
self.storeDidChange(storeName); | ||
} | ||
// change state | ||
if (typeof store.store.getState === 'function') { | ||
state = store.store.getState(); | ||
self.state.stores[storeName] = state; | ||
self.forceUpdate(); | ||
} | ||
}; | ||
}; | ||
for (var storeName in this.stores) { | ||
if (_hasOwn(this.stores, storeName)) { | ||
var store = this.stores[storeName]; | ||
store.onChange(function () { | ||
// call the components `storeDidChanged` method | ||
if (self.storeDidChange) { | ||
self.storeDidChange(storeName); | ||
} | ||
// change state | ||
if (typeof store.store.getState === 'function') { | ||
var state = store.store.getState(); | ||
self.state.stores[storeName] = state; | ||
self.forceUpdate(); | ||
} | ||
}); | ||
if (__hasOwn(this.stores, storeName)) { | ||
store = this.stores[storeName]; | ||
store.onChange(__changeHandler(store, storeName)); | ||
} | ||
@@ -218,13 +237,6 @@ } | ||
getInitialState: function () { | ||
var self = this; | ||
function _findDispatcher(view) { | ||
if (!view.props.dispatcher) { | ||
return _findDispatcher(view._owner); | ||
} else { | ||
return view.props.dispatcher; | ||
} | ||
} | ||
var self = this, state; | ||
// some shortcuts | ||
this.dispatcher = _findDispatcher(this); | ||
this.dispatcher = __findDispatcher(this); | ||
if (this.storesDidChange) { | ||
@@ -238,9 +250,9 @@ this.dispatcher.on('change:all', function () { | ||
var state = {stores: {}}; | ||
state = {stores: {}}; | ||
// more shortcuts for the state | ||
for (var storeName in this.stores) { | ||
if (_hasOwn(this.stores, storeName)) { | ||
if (this.stores[storeName] | ||
&& this.stores[storeName].store | ||
&& this.stores[storeName].store.getState) { | ||
if (__hasOwn(this.stores, storeName)) { | ||
if (this.stores[storeName] && | ||
this.stores[storeName].store && | ||
this.stores[storeName].store.getState) { | ||
state.stores[storeName] = this.stores[storeName].store.getState(); | ||
@@ -262,3 +274,3 @@ } | ||
if (typeof define === 'function' && define.amd) { | ||
define([], function() { | ||
define([], function () { | ||
return DeLorean; | ||
@@ -265,0 +277,0 @@ }); |
@@ -1,2 +0,2 @@ | ||
module.exports = function(config) { | ||
module.exports = function (config) { | ||
config.set({ | ||
@@ -8,2 +8,4 @@ basePath: '..', | ||
files: [ | ||
'test/spec/common.js', | ||
'test/vendor/react-0.11.1.js', | ||
'src/delorean.js', | ||
@@ -10,0 +12,0 @@ 'dist/.tmp/delorean-requires.js', |
@@ -1,14 +0,101 @@ | ||
var Flux = DeLorean.Flux; | ||
describe('Flux', function () { | ||
describe("Flux", function() { | ||
it('should generate a store', function () { | ||
var store = Flux.createStore(); | ||
expect(store instanceof DeLorean.Store).toBeTruthy(); | ||
var initializeSpy = jasmine.createSpy('construction'); | ||
var listenerSpy = jasmine.createSpy('change'); | ||
var listenerSpy2 = jasmine.createSpy('change'); | ||
var MyAppStore = DeLorean.Flux.createStore({ | ||
list: [], | ||
initialize: initializeSpy, | ||
actions: { | ||
// Remember the `dispatch('addItem')` | ||
addItem: 'addItemMethod' | ||
}, | ||
addItemMethod: function (data) { | ||
this.list.push('ITEM: ' + data.random); | ||
// You need to say your store is changed. | ||
this.emit('change'); | ||
} | ||
}); | ||
var myStore = new MyAppStore(); | ||
it('should generate a dispatcher', function () { | ||
var dispatcher = Flux.createDispatcher(); | ||
expect(dispatcher instanceof DeLorean.Dispatcher).toBeTruthy(); | ||
var MyAppStore2 = DeLorean.Flux.createStore({ | ||
list: [], | ||
initialize: initializeSpy, | ||
actions: { | ||
// Remember the `dispatch('addItem')` | ||
addItem: 'addItemMethod' | ||
}, | ||
addItemMethod: function (data) { | ||
this.list.push('ANOTHER: ' + data.random); | ||
// You need to say your store is changed. | ||
this.emit('change'); | ||
} | ||
}); | ||
var myStore2 = new MyAppStore2(); | ||
var MyAppDispatcher = DeLorean.Flux.createDispatcher({ | ||
addItem: function (data) { | ||
this.dispatch('addItem', data); | ||
}, | ||
getStores: function () { | ||
return { | ||
myStore: myStore, | ||
myStore2: myStore2 | ||
}; | ||
} | ||
}); | ||
var ActionCreator = { | ||
addItem: function () { | ||
// We'll going to call dispatcher methods. | ||
MyAppDispatcher.addItem({random: 'hello world'}); | ||
} | ||
}; | ||
myStore.onChange(listenerSpy); | ||
myStore2.onChange(listenerSpy2); | ||
ActionCreator.addItem(); | ||
it('store should be initialized', function () { | ||
expect(initializeSpy).toHaveBeenCalled(); | ||
}); | ||
it('should call run action creator', function () { | ||
expect(listenerSpy).toHaveBeenCalled(); | ||
expect(listenerSpy2).toHaveBeenCalled(); | ||
}); | ||
it('should change data', function () { | ||
expect(myStore.store.list.length).toBe(1); | ||
expect(myStore2.store.list.length).toBe(1); | ||
ActionCreator.addItem(); | ||
expect(myStore.store.list.length).toBe(2); | ||
expect(myStore2.store.list.length).toBe(2); | ||
expect(myStore.store.list[0]).toBe('ITEM: hello world'); | ||
expect(myStore2.store.list[0]).toBe('ANOTHER: hello world'); | ||
}); | ||
it('dispatcher can listen events', function () { | ||
var spy = jasmine.createSpy('dispatcher listener'); | ||
MyAppDispatcher.on('hello', spy); | ||
MyAppDispatcher.listener.emit('hello'); | ||
expect(spy).toHaveBeenCalled(); | ||
}); | ||
it('dispatcher can listen events', function () { | ||
var spy = jasmine.createSpy('dispatcher listener'); | ||
MyAppDispatcher.on('hello', spy); | ||
MyAppDispatcher.off('hello', spy); | ||
MyAppDispatcher.listener.emit('hello'); | ||
expect(spy).not.toHaveBeenCalled(); | ||
}); | ||
}); |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is too big to display
Mixed license
License(Experimental) Package contains multiple licenses.
Found 1 instance in 1 package
1576966
41
37338
157
19
1
186