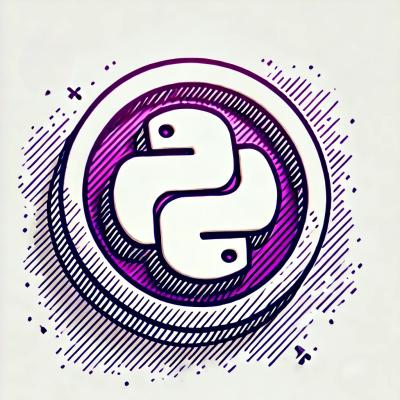
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
The dequal npm package is a library for performing deep equality checks. It is a lightweight and fast utility for comparing objects, arrays, and other nested data structures to determine if they are equivalent in value.
Deep equality check for objects
This feature allows you to compare two objects deeply to check if they are equal in value, regardless of whether they are different instances.
const { dequal } = require('dequal');
let obj1 = { a: 1, b: { c: 2 } };
let obj2 = { a: 1, b: { c: 2 } };
console.log(dequal(obj1, obj2)); // true
Deep equality check for arrays
This feature allows you to compare two arrays deeply to check if they are equal in value, including nested arrays.
const { dequal } = require('dequal');
let arr1 = [1, [2, 3]];
let arr2 = [1, [2, 3]];
console.log(dequal(arr1, arr2)); // true
Deep equality check for mixed structures
This feature allows you to compare mixed data structures like objects containing arrays or arrays containing objects to check for deep equality.
const { dequal } = require('dequal');
let mix1 = { a: [1, { b: 2 }] };
let mix2 = { a: [1, { b: 2 }] };
console.log(dequal(mix1, mix2)); // true
Lodash's isEqual method is a popular utility for performing deep equality checks. It is part of the larger Lodash library, which provides a wide range of utilities for working with JavaScript data types. Compared to dequal, lodash.isequal is part of a larger bundle and may not be as lightweight if you only need the deep equality functionality.
The deep-equal package is another library that provides deep comparison functionality. It is similar to dequal in that it focuses on deep equality checks, but it may have different performance characteristics and API design.
fast-deep-equal is a package that claims to be the fastest deep equality checker. It is similar to dequal in its purpose but may have different implementation details that could affect performance and compatibility in various use cases.
A tiny (304B to 489B) utility to check for deep equality
This module supports comparison of all types, including Function
, RegExp
, Date
, Set
, Map
, TypedArray
s, DataView
, null
, undefined
, and NaN
values. Complex values (eg, Objects, Arrays, Sets, Maps, etc) are traversed recursively.
Important:
- key order within Objects does not matter
- value order within Arrays does matter
- values within Sets and Maps use value equality
- keys within Maps use value equality
$ npm install --save dequal
There are two "versions" of dequal
available:
dequal
Size (gzip): 489 bytes
Availability: CommonJS, ES Module, UMD
dequal/lite
IE9+ | Number | String | Date | RegExp | Object | Array | Class | Set | Map | ArrayBuffer | TypedArray | DataView | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
dequal | :x: | :white_check_mark: | :white_check_mark: | :white_check_mark: | :white_check_mark: | :white_check_mark: | :white_check_mark: | :white_check_mark: | :white_check_mark: | :white_check_mark: | :white_check_mark: | :white_check_mark: | :white_check_mark: |
dequal/lite | :+1: | :white_check_mark: | :white_check_mark: | :white_check_mark: | :white_check_mark: | :white_check_mark: | :white_check_mark: | :white_check_mark: | :x: | :x: | :x: | :x: | :x: |
Note: Table scrolls horizontally!
import { dequal } from 'dequal';
dequal(1, 1); //=> true
dequal({}, {}); //=> true
dequal('foo', 'foo'); //=> true
dequal([1, 2, 3], [1, 2, 3]); //=> true
dequal(dequal, dequal); //=> true
dequal(/foo/, /foo/); //=> true
dequal(null, null); //=> true
dequal(NaN, NaN); //=> true
dequal([], []); //=> true
dequal(
[{ a:1 }, [{ b:{ c:[1] } }]],
[{ a:1 }, [{ b:{ c:[1] } }]]
); //=> true
dequal(1, '1'); //=> false
dequal(null, undefined); //=> false
dequal({ a:1, b:[2,3] }, { a:1, b:[2,5] }); //=> false
dequal(/foo/i, /bar/g); //=> false
Returns: Boolean
Both foo
and bar
can be of any type.
A Boolean
is returned indicating if the two were deeply equal.
Running Node v10.13.0
The benchmarks can be found in the /bench
directory. They are separated into two categories:
basic
– compares an object comprised of String
, Number
, Date
, Array
, and Object
values.complex
– like basic
, but adds RegExp
, Map
, Set
, and Uint8Array
values.Note: Only candidates that pass validation step(s) are listed.
For example,fast-deep-equal/es6
handlesSet
andMap
values, but uses referential equality while those listed use value equality.
Load times:
assert 0.109ms
util 0.006ms
fast-deep-equal 0.479ms
lodash/isequal 22.826ms
nano-equal 0.417ms
dequal 0.396ms
dequal/lite 0.264ms
Benchmark :: basic
assert.deepStrictEqual x 325,262 ops/sec ±0.57% (94 runs sampled)
util.isDeepStrictEqual x 318,812 ops/sec ±0.87% (94 runs sampled)
fast-deep-equal x 1,332,393 ops/sec ±0.36% (93 runs sampled)
lodash.isEqual x 269,129 ops/sec ±0.59% (95 runs sampled)
nano-equal x 1,122,053 ops/sec ±0.36% (96 runs sampled)
dequal/lite x 1,700,972 ops/sec ±0.31% (94 runs sampled)
dequal x 1,698,972 ops/sec ±0.63% (97 runs sampled)
Benchmark :: complex
assert.deepStrictEqual x 124,518 ops/sec ±0.64% (96 runs sampled)
util.isDeepStrictEqual x 125,113 ops/sec ±0.24% (96 runs sampled)
lodash.isEqual x 58,677 ops/sec ±0.49% (96 runs sampled)
dequal x 345,386 ops/sec ±0.27% (96 runs sampled)
MIT © Luke Edwards
FAQs
A tiny (304B to 489B) utility for check for deep equality
The npm package dequal receives a total of 13,381,464 weekly downloads. As such, dequal popularity was classified as popular.
We found that dequal demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.