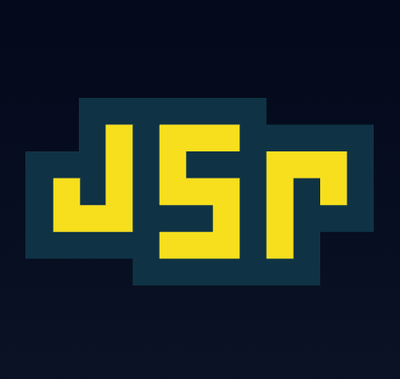
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The devcert npm package is used to generate locally-trusted development SSL certificates. It simplifies the process of creating and managing SSL certificates for local development environments, ensuring that your local development server can run over HTTPS without browser warnings.
Generate a development SSL certificate
This feature allows you to generate a locally-trusted SSL certificate for a specified domain. The code sample demonstrates how to generate a certificate for the domain 'my-app.test' and log the resulting SSL object.
const devcert = require('devcert');
(async () => {
const ssl = await devcert.certificateFor('my-app.test');
console.log(ssl);
})();
Generate a certificate with custom options
This feature allows you to generate a certificate with additional options, such as installing certutil if it's not already installed. The code sample shows how to generate a certificate for 'my-app.test' with the 'installCertutil' option set to true.
const devcert = require('devcert');
(async () => {
const ssl = await devcert.certificateFor('my-app.test', { installCertutil: true });
console.log(ssl);
})();
Retrieve existing certificate
This feature allows you to retrieve an existing certificate without modifying the hosts file. The code sample demonstrates how to retrieve a certificate for 'my-app.test' with the 'skipHostsFile' option set to true.
const devcert = require('devcert');
(async () => {
const ssl = await devcert.certificateFor('my-app.test', { skipHostsFile: true });
console.log(ssl);
})();
mkcert is a simple tool for making locally-trusted development certificates. It automatically creates and installs a local CA in the system root store, and generates certificates for your local development domains. Compared to devcert, mkcert is a standalone tool that can be used outside of a Node.js environment, whereas devcert is an npm package designed for use within Node.js projects.
local-ssl-proxy is a simple SSL proxy for local development. It allows you to run an HTTPS server that forwards requests to your local HTTP server. Unlike devcert, which focuses on generating SSL certificates, local-ssl-proxy provides a way to run an HTTPS server that proxies requests to an existing HTTP server.
selfsigned is an npm package for generating self-signed certificates. It provides a simple API for creating certificates, but does not handle the installation of certificates in the system trust store like devcert does. selfsigned is more focused on the certificate generation aspect, while devcert provides a more comprehensive solution for local development.
So, running a local HTTPS server usually sucks. There's a range of approaches, each with their own tradeoff. The common one, using self-signed certificates, means having to ignore scary browser warnings for each project.
devcert makes the process easy. Want a private key and certificate file to use with your server? Just ask:
import { createServer } from 'https';
import * as express from 'express';
import getDevelopmentCertificate from 'devcert';
async function buildMyApp() {
let app = express();
app.get('/', function (req, res) {
res.send('Hello Secure World!');
});
let ssl;
if (process.env.NODE_ENV === 'development') {
ssl = await getDevelopmentCertificate('my-app', { installCertutil: true });
} else {
ssl = // load production ssl ...
}
return createServer(ssl, app).listen(3000);
}
Now open https://localhost:3000 and voila - your page loads with no scary warnings or hoops to jump through.
Certificates are cached by name, so two calls for
getDevelopmentCertificate('foo')
will return the same key and certificate.
devcert currently takes a single option: installCertutil
. If true, devcert
will attempt to install some software necessary to tell Firefox (and Chrome on
Linux) to trust your development certificates. This is not required, but without
it, you'll need to tell Firefox to trust these certificates manually:
Firefox provides a point-and-click wizard for importing and trusting a
certificate, so if you don't provide installCertutil: true
to devcert, we'll
instead open Firefox and kick off this wizard for you. Simply follow the prompts
to trust the certificate. Reminder: you'll only need to do this once per
machine
Note: Chrome on Linux requires installCertutil: true
, or else you'll
face the scary browser warnings every time. Unfortunately, there's no way to
tell Chrome on Linux to trust a certificate without install certutil.
The software installed varies by OS:
brew install nss
apt install libnss3-tools
When you ask for a development certificate, devcert will first check to see if it has run on this machine before. If not, it will create a root certificate authority and add it to your OS and various browser trust stores. You'll likely see password prompts from your OS at this point to authorize the new root CA. This is the only time you'll see these prompts.
This root certificate authority allows devcert to create a new SSL certificate whenever you want without needing to ask for elevated permissions again. It also ensures that browsers won't show scary warnings about untrusted certificates, since your OS and browsers will now trust devcert's certificates. The root CA certificate is unique to your machine only, and is generated on-the-fly when it is installed.
MIT © Dave Wasmer
FAQs
Generate trusted local SSL/TLS certificates for local SSL development
The npm package devcert receives a total of 308,314 weekly downloads. As such, devcert popularity was classified as popular.
We found that devcert demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.