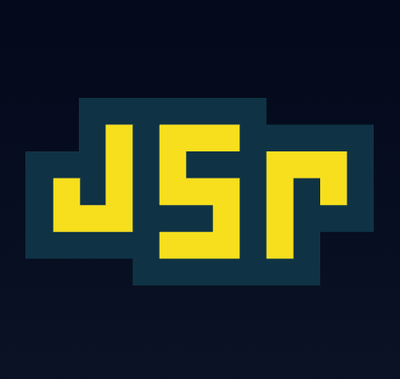
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Generate trusted local SSL/TLS certificates for local SSL development
The devcert npm package is used to generate locally-trusted development SSL certificates. It simplifies the process of creating and managing SSL certificates for local development environments, ensuring that your local development server can run over HTTPS without browser warnings.
Generate a development SSL certificate
This feature allows you to generate a locally-trusted SSL certificate for a specified domain. The code sample demonstrates how to generate a certificate for the domain 'my-app.test' and log the resulting SSL object.
const devcert = require('devcert');
(async () => {
const ssl = await devcert.certificateFor('my-app.test');
console.log(ssl);
})();
Generate a certificate with custom options
This feature allows you to generate a certificate with additional options, such as installing certutil if it's not already installed. The code sample shows how to generate a certificate for 'my-app.test' with the 'installCertutil' option set to true.
const devcert = require('devcert');
(async () => {
const ssl = await devcert.certificateFor('my-app.test', { installCertutil: true });
console.log(ssl);
})();
Retrieve existing certificate
This feature allows you to retrieve an existing certificate without modifying the hosts file. The code sample demonstrates how to retrieve a certificate for 'my-app.test' with the 'skipHostsFile' option set to true.
const devcert = require('devcert');
(async () => {
const ssl = await devcert.certificateFor('my-app.test', { skipHostsFile: true });
console.log(ssl);
})();
mkcert is a simple tool for making locally-trusted development certificates. It automatically creates and installs a local CA in the system root store, and generates certificates for your local development domains. Compared to devcert, mkcert is a standalone tool that can be used outside of a Node.js environment, whereas devcert is an npm package designed for use within Node.js projects.
local-ssl-proxy is a simple SSL proxy for local development. It allows you to run an HTTPS server that forwards requests to your local HTTP server. Unlike devcert, which focuses on generating SSL certificates, local-ssl-proxy provides a way to run an HTTPS server that proxies requests to an existing HTTP server.
selfsigned is an npm package for generating self-signed certificates. It provides a simple API for creating certificates, but does not handle the installation of certificates in the system trust store like devcert does. selfsigned is more focused on the certificate generation aspect, while devcert provides a more comprehensive solution for local development.
So, running a local HTTPS server usually sucks. There's a range of approaches, each with their own tradeoff. The common one, using self-signed certificates, means having to ignore scary browser warnings for each project.
devcert makes the process easy. Want a private key and certificate file to use with your server? Just ask:
let { key, cert } = await devcert.certificateFor('my-app.dev');
https.createServer({ key, cert }, app).listen(3000);
});
Now open https://my-app.dev:3000 and voila - your page loads with no scary warnings or hoops to jump through.
Certificates are cached by name, so two calls for
certificateFor('foo')
will return the same key and certificate.
If you supply a custom domain name (i.e. any domain other than localhost
) when requesting a certificate from devcert, it will attempt to modify your system to redirect requests for that domain to your local machine (rather than to the real domain). It does this by modifying your /etc/hosts
file (or the equivalent file for Windows).
If you pass in the skipHostsFile
option, devcert will skip this step. This means that if you ask for certificates for my-app.dev
(for example), and don't have some other DNS redirect method in place, that you won't be able to access your app at my-app.dev
.
This option will tell devcert to avoid installing certutil
tooling.
certutil
is a tooling package used to automated the installation of SSL certificates in certain circumstances; specifically, Firefox (for every OS) and Chrome (on Linux only). Without it, the install process changes for those two scenarios:
Firefox: Thankully, Firefox makes this easy. There's a point-and-click wizard for importing and trusting a certificate, so if you don't provide installCertutil: true
to devcert, devcert will instead automatically open Firefox and kick off this wizard for you. Simply follow the prompts to trust the certificate. Reminder: you'll only need to do this once per machine
Chrome on Linux: Unfortunately, it appears that the only way to get Chrome to trust an SSL certificate on Linux is via the certutil
tooling - there is no manual process for it. Thus, if you are using Chrome on Linux, do not supply skipCertuil: true
.
The certutil
tooling is installed in OS-specific ways:
brew install nss
apt install libnss3-tools
When you ask for a development certificate, devcert will first check to see if it has run on this machine before. If not, it will create a root certificate authority and add it to your OS and various browser trust stores. You'll likely see password prompts from your OS at this point to authorize the new root CA. Once this root CA is trusted by your machine, devcert will safely store the root CA credentials used to sign certificates in the operating system's secret store. This prevents malicious processes from access those keys to generated trusted certificates.
Since your machine now trusts this root CA, it will trust any certificates signed by it. So when you ask for a certificate, devcert will pull the root CA credentials out of the operating system secret storage (triggering a root password as it does). It then uses those credentials to generate a certificate specific to the domain you requested, and returns the new certificate to you.
If you request a domain that has already had certificates generated for it, devcert will simply return the cached certificates - no additional root password prompting needed.
This setup ensures that browsers won't show scary warnings about untrusted certificates, since your OS and browsers will now trust devcert's certificates. The root CA certificate is unique to your machine only, is generated on-the-fly when it is first installed, and stored in the system secret storage, so attackers should not be able to compromise it to generate their own certificates.
The root certificate authority makes it slightly simpler to manage which domains are configured for SSL by devcert. The alternative is to generate and trust self-signed certificates for each domain. The problem is that while devcert is able to add a certificate to your machine's trust stores, the tooling to remove a certificate doesn't cover every case.
By trusting only a single root CA, devcert is able to guarantee that when you want to disable SSL for a domain, it can do so with no manual intervention - we just delete the certificate files and that's it.
If you want to test a contribution to devcert, it comes packaged with a Vagrantfile to help make testing easier. The Vagrantfile will spin up three virtual machines, one for each supported platform: macOS, Linux, and Windows.
Launch the VMs with vagrant up
, which should start all three in GUI mode. Each VM is a snapshot, with instructions for testing on screen already. Just follow the instructions to test each.
You can also use snapshots of the VMs to roll them back to a pristine state for another round of testing. Just vagrant snapshot push
on the intial bootup of the VMs, and vagrant snapshot pop
to roll it back to the pristine state later.
Note: Be aware that the macOS license terms prohibit running it on non-Apple hardware, so you must own a Mac to test that platform. If you don't own a Mac - that's okay, just mention in the PR that you were unable to test on a Mac and we're happy to test it for you.
MIT © Dave Wasmer
FAQs
Generate trusted local SSL/TLS certificates for local SSL development
The npm package devcert receives a total of 308,314 weekly downloads. As such, devcert popularity was classified as popular.
We found that devcert demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.