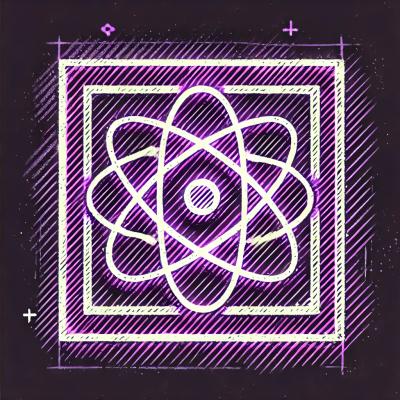
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
dipswitch
Dipswitch is a multi-tenant feature toggle library.
$ npm install dipswitch --save
If you wish to use redis to store this data, also install dipswitch-redis. Otherwise, an in-memory store will be used.
var Dipswitch = require('dipswitch'),
dipswitch = new Dipswitch({
redis: require('redis').createClient(),
getTenantId: function (tenant) { // default is `return tenant.id;`
return tenant.tenantId;
},
getUserId: function (user) { // default is `return user.id;`
return user.userId;
},
getUserGroups: function (user) { // default is `return user.groups || [];`
if (user.isAdmin) {
return ['admins'];
}
return [];
}
});
// ADDING & REMOVING FEATURES --------------------------------------------------
// add & remove a feature
dipswitch.features.add('EXAMPLE_FEATURE').then(function () { /* ... */ });
dipswitch.features.remove('EXAMPLE_FEATURE').then(function () { /* ... */ });
// and another syntax for registering many features at once
dipswitch.features.add(['FEATURE_1', 'FEATURE_2']).then(function () { /* ... */ });
// and unregister many features at once as well
dipswitch.features.remove(['EXAMPLE_FEATURE1', 'EXAMPLE_FEATURE2']).then(function () { /* ... */ });
// retrieve all registered features
dipswitch.features.find().then(function (features) {
/* ... */
});
// ENABLING FEATURES -----------------------------------------------------------
// enable a feature globally
dipswitch.features.enable('EXAMPLE_FEATURE').then(function () { /* ... */ });
// enable a feature for an entire tenant
dipswitch.tenant(tenant).enable('EXAMPLE_FEATURE').then(function () { /* ... */ });
// enable a feature for a group within a tenant
dipswitch.tenant(tenant).group('admins').enable('EXAMPLE_FEATURE').then(function () { /* ... */ });
// enable a feature a group across all tenants
dipswitch.group('admins').enable('EXAMPLE_FEATURE').then(function () { /* ... */ });
// enable a feature for a user within a tenant
dipswitch.tenant(tenant).user(user).enable('EXAMPLE_FEATURE').then(function () { /* ... */ });
// enable a feature for a user across all tenants
dipswitch.user(user).enable('EXAMPLE_FEATURE').then(function () { /* ... */ });
// SCHEDULING FEATURES ---------------------------------------------------------
// when enabling a feature you may optionally pass a `start` and/or `end` date
dipswitch.features.enable('LOL_OMG_CATFACTS', {
start: new Date(2015, 3, 1), // April 1st
end: new Date(2015, 3, 2) // April 2nd
}).then(function () { /* ... */ });
// CHECKING FEATURES -----------------------------------------------------------
// retrieve all features and whether they're enabled or not for a user
dipswitch.tenant(tenant).user(user).features().then(function (features) {
console.log(features.EXAMPLE_FEATURE); // `true`
});
// check a single feature and whether it is enabled or not for a user
dipswitch.tenant(tenant).user(user).feature('EXAMPLE_FEATURE').then(function (isEnabled) {
console.log(isEnabled); // `true`
});
// DISABLING FEATURES ----------------------------------------------------------
// anywhere that you can call #enable, you can also call #disable
dipswitch.tenant(tenant).user(user).disable('EXAMPLE_FEATURE').then(function () { /* ... */ });
Rather than having to have an admin UI for unregistering features you no longer care about, you can use a bit of underscore
/lodash
magic to automate the process:
var _ = require('underscore'),
Dipswitch = require('dipswitch'),
dipswitch = new Dipswitch(options);
// note that FEATURE_2 was removed at some point
var desiredFeatures = ['FEATURE_1', 'FEATURE_3'];
dipswitch.register(desiredFeatures)
.then(function () {
return dipswitch.features(); // lets say this returns ['FEATURE_1', 'FEATURE_2', 'FEATURE_3']
})
.then(function (registeredFeatures) {
var outOfDateFeatures = _.without(registeredFeatures, desiredFeatures);
return dipswitch.unregister(outOfDateFeatures); // will remove ['FEATURE_2']
});
When enabling or disabling a feature that has never been registered, that feature is automatically registered. When testing to see the value of a feature that isn't registered, the result will be false
.
FAQs
Multi-tenant feature toggles backed by Redis.
The npm package dipswitch receives a total of 3 weekly downloads. As such, dipswitch popularity was classified as not popular.
We found that dipswitch demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.