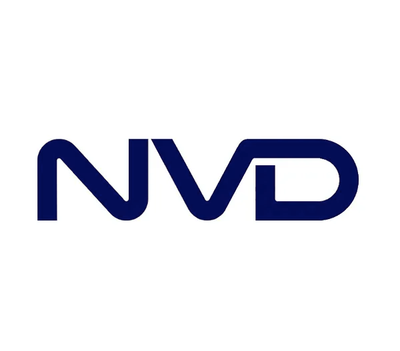
Security News
NIST Misses 2024 Deadline to Clear NVD Backlog
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
discord.js
Advanced tools
discord.js is a powerful Node.js module that allows you to interact with the Discord API very easily. It provides a comprehensive set of features to create bots and manage Discord servers.
Creating a Bot
This code demonstrates how to create a simple Discord bot using discord.js. The bot logs 'Ready!' to the console when it is successfully logged in and ready.
const { Client, GatewayIntentBits } = require('discord.js');
const client = new Client({ intents: [GatewayIntentBits.Guilds] });
client.once('ready', () => {
console.log('Ready!');
});
client.login('your-token-goes-here');
Handling Messages
This code shows how to handle messages in a Discord server. When a user sends a message with the content '!ping', the bot responds with 'Pong!'.
client.on('messageCreate', message => {
if (message.content === '!ping') {
message.channel.send('Pong!');
}
});
Managing Roles
This code demonstrates how to manage roles in a Discord server. When a user sends a message with the content '!addRole', the bot adds a role named 'NewRole' to the user.
client.on('messageCreate', async message => {
if (message.content === '!addRole') {
let role = message.guild.roles.cache.find(r => r.name === 'NewRole');
if (role) {
await message.member.roles.add(role);
message.channel.send('Role added!');
}
}
});
Sending Embeds
This code shows how to send embedded messages in Discord. When a user sends a message with the content '!embed', the bot responds with a rich embed message.
const { MessageEmbed } = require('discord.js');
client.on('messageCreate', message => {
if (message.content === '!embed') {
const embed = new MessageEmbed()
.setTitle('Sample Embed')
.setDescription('This is an example of an embed message')
.setColor(0xff0000);
message.channel.send({ embeds: [embed] });
}
});
Eris is another powerful library for interacting with the Discord API. It is known for being lightweight and efficient, making it a good alternative to discord.js. However, it may have a steeper learning curve for beginners.
discord.io is a small, lightweight library for interfacing with Discord. It is less feature-rich compared to discord.js and Eris, but it can be a good choice for simpler bots or for those who prefer a minimalistic approach.
discord.js is a powerful Node.js module that allows you to easily interact with the Discord API.
Node.js 16.9.0 or newer is required.
npm install discord.js
yarn add discord.js
pnpm add discord.js
npm install zlib-sync
)npm install bufferutil
)bufferutil
for much faster WebSocket processing (npm install utf-8-validate
)npm install @discordjs/voice
)Install discord.js:
npm install discord.js
yarn add discord.js
pnpm add discord.js
Register a slash command against the Discord API:
import { REST, Routes } from 'discord.js';
const commands = [
{
name: 'ping',
description: 'Replies with Pong!',
},
];
const rest = new REST({ version: '10' }).setToken(TOKEN);
try {
console.log('Started refreshing application (/) commands.');
await rest.put(Routes.applicationCommands(CLIENT_ID), { body: commands });
console.log('Successfully reloaded application (/) commands.');
} catch (error) {
console.error(error);
}
Afterwards we can create a quite simple example bot:
import { Client, GatewayIntentBits } from 'discord.js';
const client = new Client({ intents: [GatewayIntentBits.Guilds] });
client.on('ready', () => {
console.log(`Logged in as ${client.user.tag}!`);
});
client.on('interactionCreate', async interaction => {
if (!interaction.isChatInputCommand()) return;
if (interaction.commandName === 'ping') {
await interaction.reply('Pong!');
}
});
client.login(TOKEN);
Before creating an issue, please ensure that it hasn't already been reported/suggested, and double-check the
documentation.
See the contribution guide if you'd like to submit a PR.
If you don't understand something in the documentation, you are experiencing problems, or you just need a gentle nudge in the right direction, please don't hesitate to join our official discord.js Server.
FAQs
A powerful library for interacting with the Discord API
The npm package discord.js receives a total of 175,081 weekly downloads. As such, discord.js popularity was classified as popular.
We found that discord.js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.