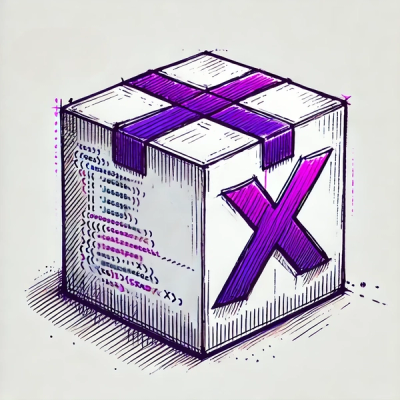
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
distributo
Advanced tools
Distributo is a TypeScript-based framework designed to streamline the distribution of tasks from a master node (the Task Distributor) to multiple worker nodes (the Worker Clients). It provides a simple API for discovering, connecting, and coordinating workers and a master server over a network using Socket.IO and optional Bonjour-based service discovery.
Newly Introduced Components:
The Master (Task Distributor / CoordinatorTaskDistributor)
The master node runs a server that:
The Workers (WorkerClient / EnhancedWorkerClient)
Each worker:
npm install
Make sure you have Node.js and npm installed, as well as TypeScript if you’re working from source.
@types/express
, @types/socket.io
, @types/bonjour
, @types/node
, etc.)Below is an example of setting up the original, simpler TaskDistributor
master node:
import { TaskDistributor } from './TaskDistributor';
interface MyTask {
word: string;
// Add any other fields your task might require
}
function getMyTasks(): MyTask[] {
return [
{ word: "apple" },
{ word: "banana" },
// ... your tasks
];
}
function handleMyResults(results: MyTask[]): void {
// Handle the processed results (e.g., save to DB, write to file)
console.log('Results received:', results.length);
}
const distributor = new TaskDistributor<MyTask>({
port: 4000, // The port to listen on
serviceType: 'eventbus', // The Bonjour service type
batchSize: 5, // Number of tasks to send to each worker per request
getTasks: getMyTasks,
handleResults: handleMyResults,
enableBonjour: true // Enable Bonjour-based service discovery
});
distributor.start();
A basic WorkerClient
:
import { WorkerClient } from './WorkerClient';
interface MyTask {
word: string;
}
interface MyResult {
word: string;
syllableData: any;
}
async function processMyTasks(tasks: MyTask[]): Promise<MyResult[]> {
const results: MyResult[] = [];
for (const task of tasks) {
const data = await scrapeSyllableData(task.word);
if (data) {
results.push({ word: task.word, syllableData: data });
}
}
return results;
}
const worker = new WorkerClient<MyTask, MyResult>({
serviceType: 'eventbus', // Matches the master's serviceType
processTasks: processMyTasks
});
worker.start();
// `scrapeSyllableData(word: string)` should be defined by you.
CoordinatorTaskDistributor
is a more robust version of the master node that:
/addTask
and /addTasks
).taskGenerator
for dynamic task provisioning.EnhancedWorkerClient
.import { CoordinatorTaskDistributor } from './CoordinatorTaskDistributor';
const coordinator = new CoordinatorTaskDistributor({
port: 4000,
httpPort: 5000,
taskFolderPath: '/path/to/projects',
serviceType: 'eventbus',
enableBonjour: true,
onResult: (results) => {
console.log('Handled results:', results);
},
taskGenerator: async () => {
// Dynamically return an array of tasks
return [{ taskId: 'dynamic-task-1', taskType: 'build', type: 'executeCode', ...otherProps }];
}
});
coordinator.start();
You can now POST
tasks to http://localhost:5000/addTask
or http://localhost:5000/addTasks
to feed new tasks into the system.
EnhancedWorkerClient
is designed to handle more complex tasks that require setup or installation phases before execution. It:
import EnhancedWorkerClient from './EnhancedWorkerClient';
const worker = new EnhancedWorkerClient({
serviceType: 'eventbus',
taskTypes: ['build', 'test'], // The tasks this worker can handle
workingDirectory: '/path/to/workdir',
});
worker.start();
With Bonjour enabled, workers (either WorkerClient
or EnhancedWorkerClient
) automatically find the master on the local network by the specified serviceType
. If you prefer a static setup, you can skip Bonjour and provide the masterUrl
directly:
const worker = new EnhancedWorkerClient({
masterUrl: 'http://127.0.0.1:4000', // Direct connection, no Bonjour required
taskTypes: ['build', 'test'],
});
Both the master and the workers come with a built-in logger using winston
. Logs are printed to the console and written to .log
files. You can customize the logger by passing in your own winston.Logger
instance.
EnhancedWorkerClient
reports setup/installation failures back to the coordinator, allowing for re-queueing or custom fallback strategies.Distributo is designed to be flexible:
CoordinatorTaskDistributor
can integrate with a taskGenerator
function to dynamically provision tasks.CoordinatorTaskDistributor
and EnhancedWorkerClient
, you can track tasks through installation, execution, and completion phases.Contributions are welcome! Please submit pull requests with new features or bug fixes, and ensure you run tests and follow the code style guidelines.
This project is released under the MIT License.
Happy distributing!
FAQs
 # Distributo
The npm package distributo receives a total of 23 weekly downloads. As such, distributo popularity was classified as not popular.
We found that distributo demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.